Linked lists are among the most fundamental and versatile data structures in computer science. They provide an efficient and flexible way to store sequential data. Mastering linked lists in Python is key for any aspiring programmer to elevate their skills.
In this comprehensive tutorial, we will walk through the step-by-step process for implementing linked lists in Python. We will cover the basics of linked list data structures, writing out the Python code from scratch, overviewing sorted linked lists, and providing tips for creating clean linked list code.
We’ll also look at how creating a program flowchart in EdrawMax can help plan out your linked list algorithm. By the end, you’ll have a strong foundation for leveraging linked lists in your own Python projects.
In this article
Part 1: What is a Linked List in Python?
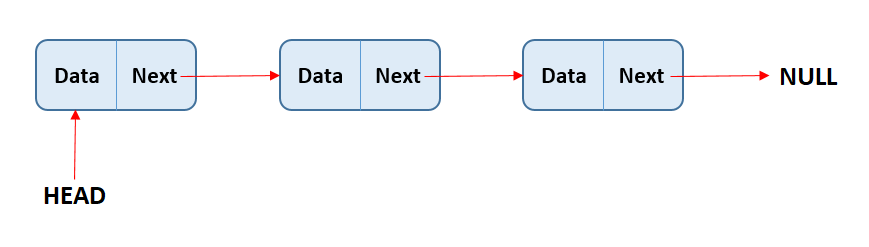
A linked list is a linear data structure consisting of a sequence of “nodes” that are randomly stored in memory. Each node contains data and a pointer that links to the next node in the sequence. This makes linked lists very memory efficient as they only use the exact amount of memory required at any given time.
Part 2: Steps for Implementing a Linked List Python Code
Let’s now walk through the key steps to implement a basic linked list in Python:
- Create a Node Class: We need to define a Node class that contains an element and a reference (next_node) to the next node. This forms the basic building block of our list.
- Initialize the Head Node: We’ll create a Linked List class that contains the key operations (insert, remove, etc). The head node is the starting point for any linked list.
- Insert a New Node: We define an insert method that creates a new node with the given value and inserts it in the correct order in the existing chain of nodes.
- Print Out Contents of List: We need a print method that iterates through the nodes and prints out the value in each node - checking for None as the next_node indicates the end.
- Add Other Methods like Remove, Reverse Operations: Populating other helper methods will allow different manipulations like removing a node with a given value, reversing the list order, etc.
Part 3: Overview of Sorted Linked List Python
A sorted linked list automatically keeps nodes in ascending sorted order upon insertion, removing the need to explicitly sort later. This requires changing the insert method to include:
- Check if the new value being inserted is less than or greater than the current node value.
- Traverse to the appropriate next node until the right sorted position is found.
- Insert it before or after this node maintaining ascending order.
The benefit is to get a pre-sorted list on every insert without needing to call sort. The tradeoff is slightly longer insertion times as the pointers traverse the list to find the correct insertion point.
Part 4: Tips for Creating a Linked List in Python
Here are some key tips for writing clean, effective linked list code in Python:
- Modularize Different Operations: Split code into methods for each operation like insert, remove, etc rather than a long single method.
- Use Descriptive Variable Names: Use names like head_node rather than a to improve readability and understanding.
- Validate Inputs: Check for valid input types and values being inserted to handle edge cases.
- Use a Print Method to Debug: Debugging each step is easier with a dedicated print report showing list order.
- Use Comments and Docstrings: Effective comments explain parts of implementation to yourself and others.
Part 5: Creating a Programming Algorithm Flowchart Using EdrawMax
Visualizing program logic before coding helps structure thoughts into the proper step order. EdrawMax is a cross-platform desktop app for creating all kinds of diagrams and charts.
Having this bird's eye view enables catching logical gaps or edge cases before writing lengthy code. Debugging is also faster by comparing against planned flow.
Here are the steps to create a simple programming flowchart using EdrawMax:
Step 1:
Launch the EdrawMax application on your computer. Choose the "Flowchart" category from the template library or use the search bar to find flowchart templates.
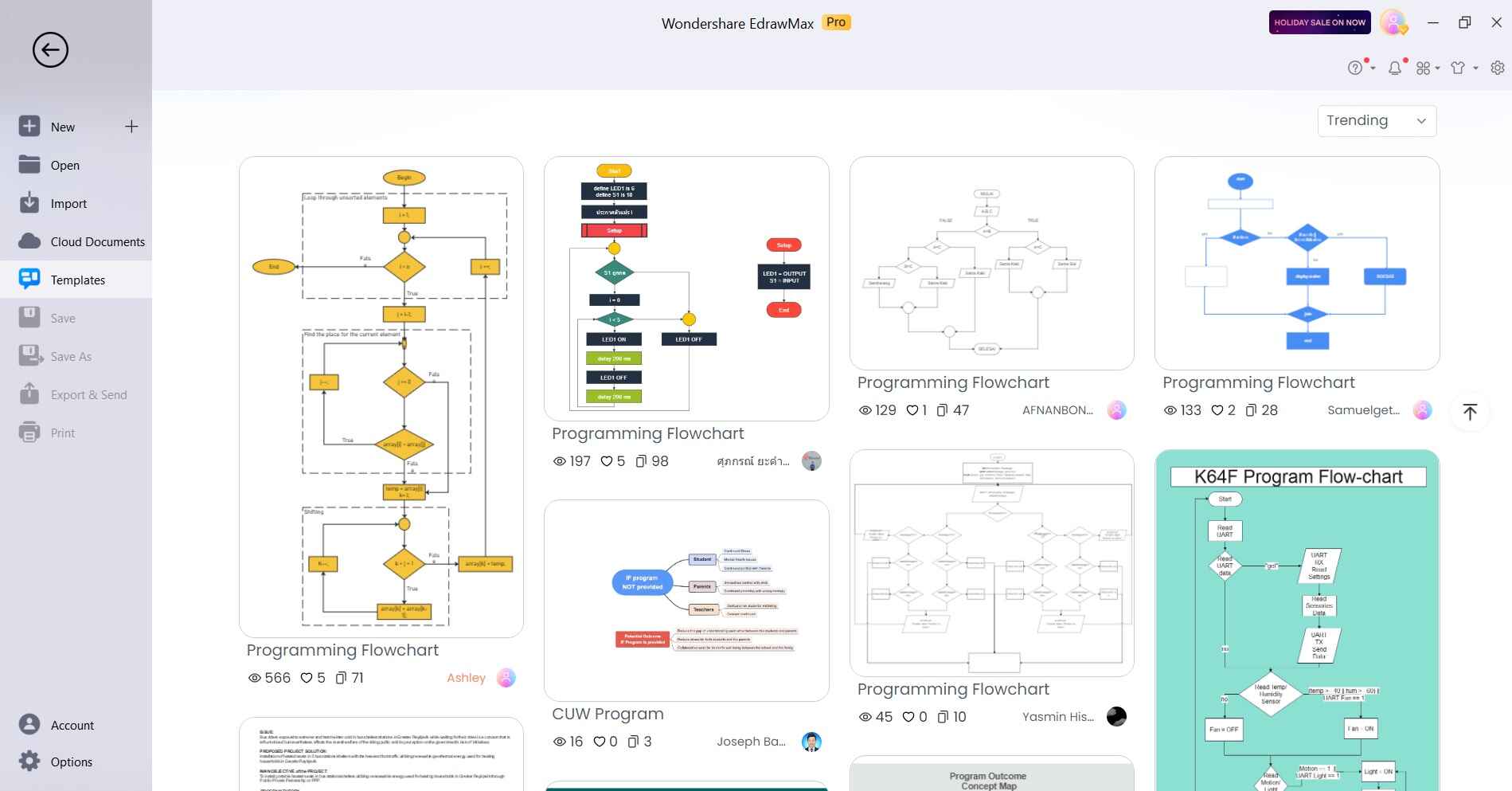
Step 2:
Drag and drop various flowchart symbols or shapes from the left-hand side panel onto the canvas. Common symbols include start/end symbols, process symbols, decision symbols (diamond), input/output symbols, etc.
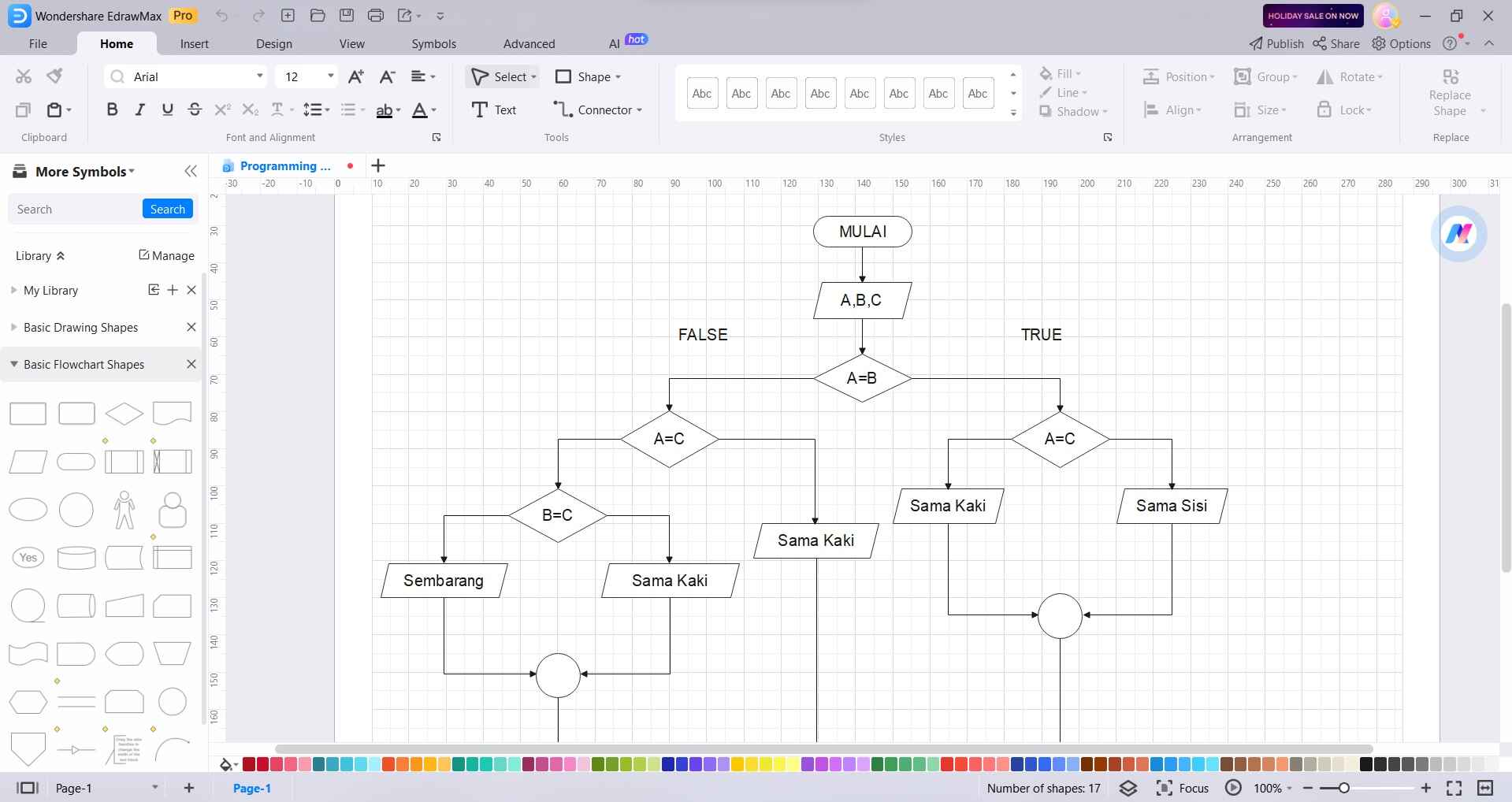
Step 3:
Double-click on the symbols to add text or labels to describe the actions, decisions, inputs, or outputs associated with each symbol.
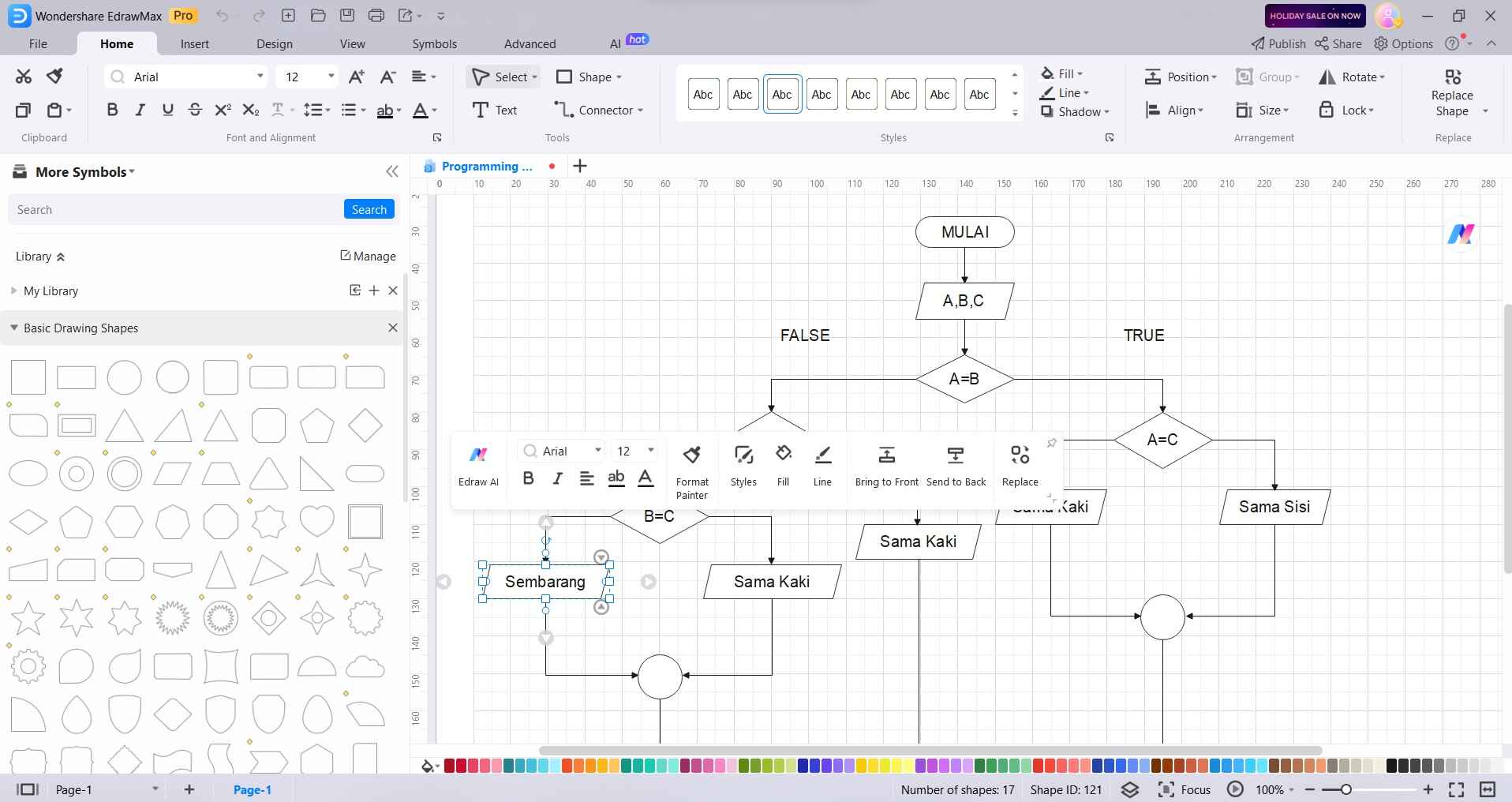
Step 4:
Organize the flowchart elements neatly on the canvas. You can resize symbols, change colors, add formatting, or adjust the layout as needed.
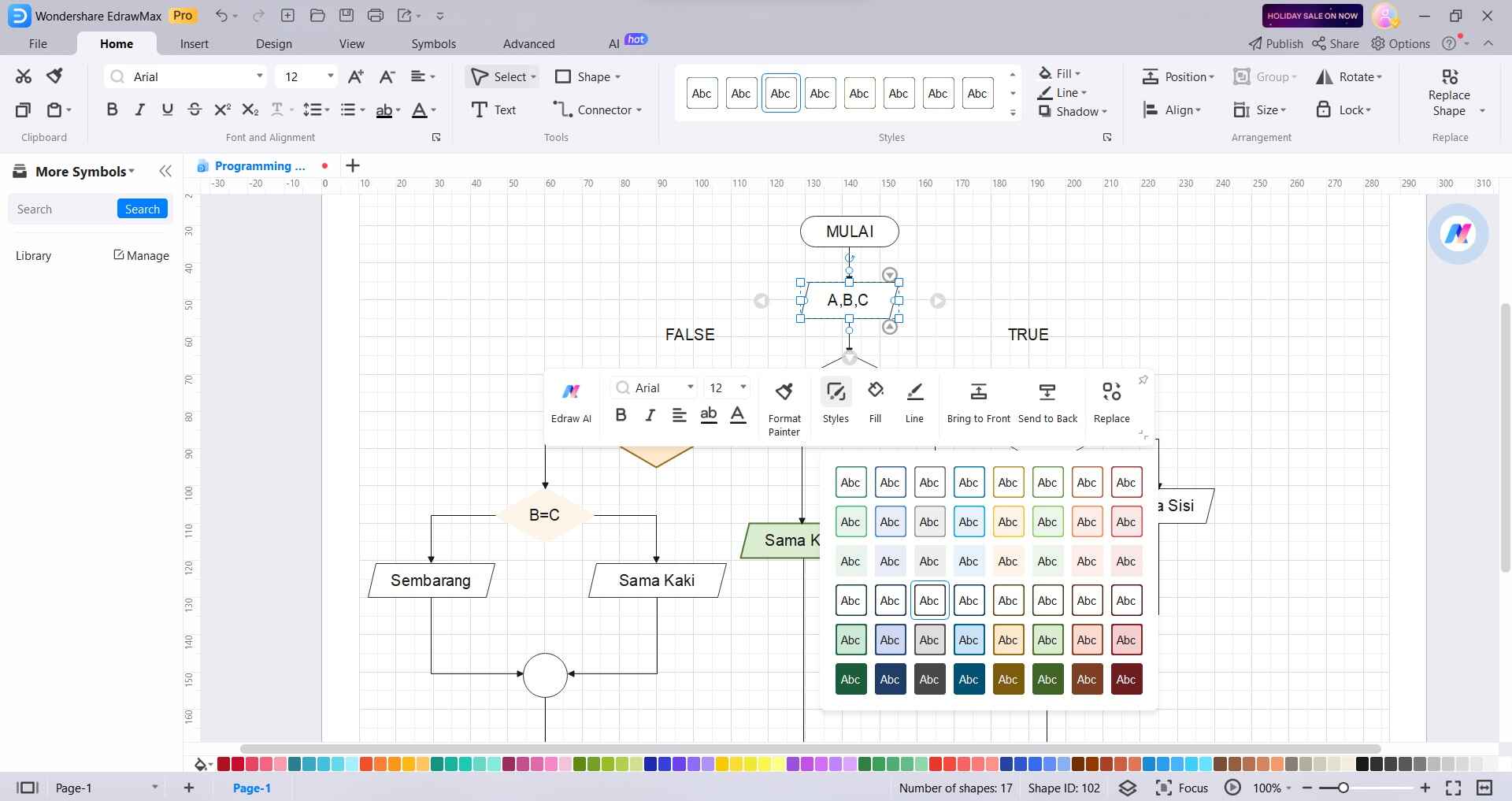
Step 5:
Once you've completed your programming flowchart, save your work within EdrawMax.
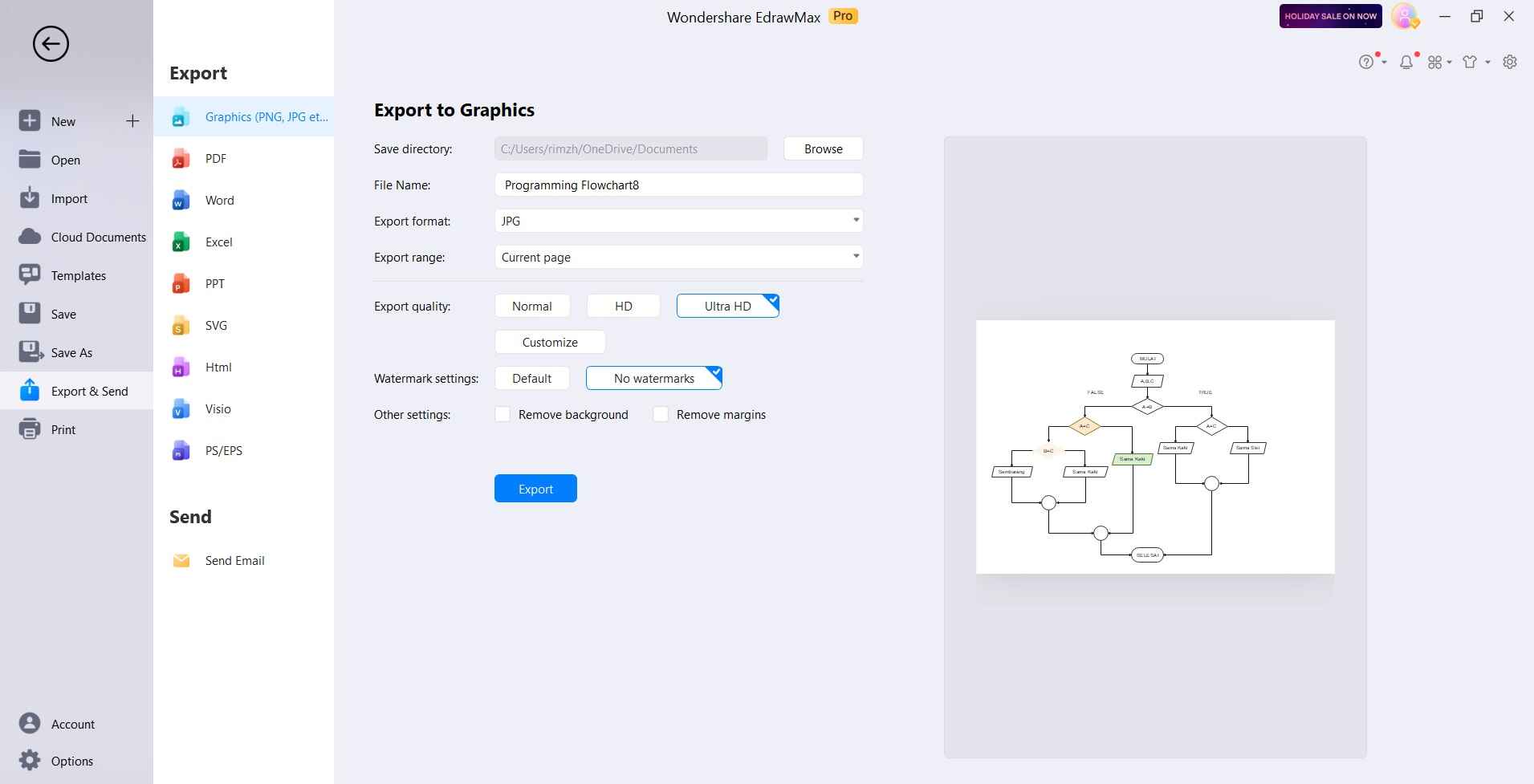
EdrawMax offers various customization options, including different symbols, themes, and styling features to create professional-looking flowcharts. Take advantage of these features to make your programming flowchart visually appealing and easy to understand.
Conclusion
And there you have it - a complete guide to implementing linked lists in Python. We covered the core concepts, step-by-step coding guidelines, tips, and techniques for robust linked list code as well as the importance of visually mapping algorithms first. Mastering these foundational data structures will equip you to handle more advanced projects like graphs, queues, and binary trees.
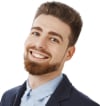