Golang (or Go) is an open-source programming language developed by Google that has gained immense popularity over the last decade. It's known for its simplicity, concurrency support, scalability, and more. One of the key components in Go is structs - composite data types that allow related data to be grouped together.
Here we will provide an in-depth overview of Go structs, the various types, things to consider when creating structs, and how to visualize them with flowcharts.
In this article
Part 1: What is Golang?
Golang is a statically typed, compiled programming language that makes it easy to build simple, reliable, and efficient software. It was started in 2007 at Google and combined useful elements of other languages like Python, C++, and Pascal/Modula. Some key features of Go:
- Simplicity - easy to learn with a small set of keywords and clean syntax
- Fast compile times
- Built-in concurrency features using goroutines and channels
- Garbage collected
- Scalable and performant
- Supports interfaces and reflection
- Produces standalone binary executables
These attributes make Go a great choice for building web services, backends, distributed systems, networking tools, and more. Major companies using Go include Google, Uber, Dropbox, Twitch, and Cloudflare.
Part 2: Overview of Structs in Golang
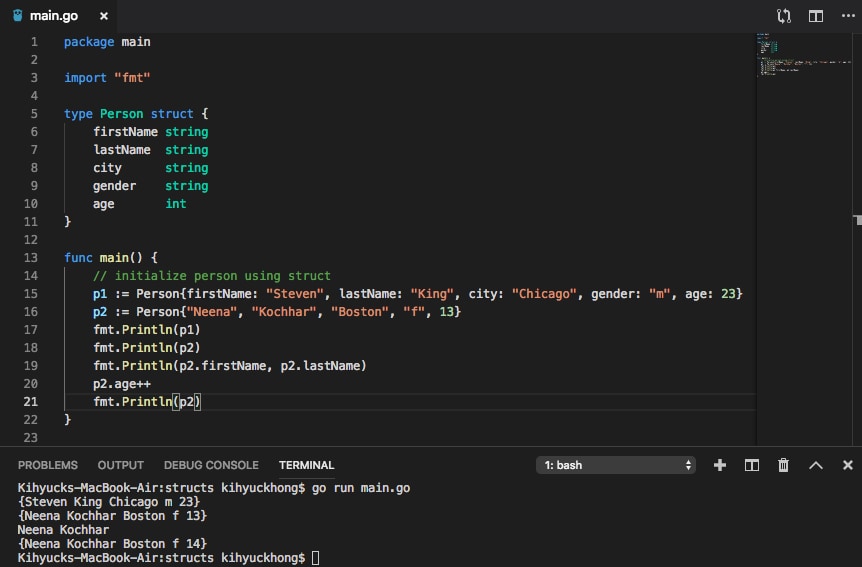
Structs in Golang represent custom data types that allow you to group related fields together. They act like templates for creating object instances with predefined properties that you set.
Here’s an example Golang struct:
type User struct {
ID int
FirstName string
LastName string
Email string
}
This defines a User struct with 4 fields - ID, FirstName, LastName, and Email.
Part 3: Types of Golang Structs
There are several special or advanced struct types in Golang:
#1 Golang Anonymous Structs
These are unnamed structs created directly within functions or code blocks, handy for one-time use without declaring a specific type. They offer a concise way to structure data without defining a named type explicitly.
#2 Golang Generic Struct
A generic struct in Golang serves as a template for creating other specific structs by defining placeholders for types or fields. It allows for writing flexible and reusable code by defining structures that can adapt to different data types.
#3 Golang Extend Struct
Extending structs involves adding fields or methods of one struct to another, enabling the creation of new structs with combined functionalities. It promotes code reusability and extends the capabilities of existing structures.
#4 Golang Embedded Struct
Embedded structs facilitate composition in Go, allowing a struct to inherit the fields and methods of another struct. They help create more complex data structures by combining multiple structs, enhancing code modularity and readability.
#5 Golang Empty Struct
An empty struct in Golang has no fields, yet it can be used as a signal or placeholder in channels, sync mechanisms, or to signify certain conditions. Despite having no data, its presence holds significance in synchronizing goroutines or signaling events.
Part 4: Things to Consider When Creating a Golang Structure Project
Here are some key considerations when working with structs on a Golang project:
- Encapsulation - bundle related fields into meaningful groups.
- Reusability - make structs that can be instantiated anywhere.
- Names - use PascalCase for struct names and camelCase for fields.
- Composition - use embedding wisely to reuse vs inheritance.
- Generic vs Specific - parameterize general-purpose structs.
- Zero value - understand default values with new structs.
- Methods - add helper methods to struct types.
- Validation - check for valid data when creating new instances.
- Mutable vs Immutable - design structs to prefer immutability.
- Documentation - comment behavior of struct fields and methods.
Thinking through these areas will help craft a struct that functions properly.
Part 5: Creating an Algorithm Flowchart Using EdrawMax
EdrawMax offers a comprehensive and intuitive platform for creating programming algorithm flowcharts, crucial in visualizing and designing complex algorithms. Its extensive library of shapes, symbols, and templates tailored for programming allows seamless representation of logical sequences, decision trees, and iterative processes.
With its user-friendly interface and drag-and-drop functionality, EdrawMax simplifies the visualization of algorithms, aiding programmers in presenting their logic clearly, fostering better comprehension, easier debugging, and efficient communication among team members, ultimately enhancing the development process.
Here are the steps to create a simple programming algorithm flowchart using EdrawMax:
Step 1:
Launch the EdrawMax application on your device. Navigate to the "Flowchart" category or search for "Flowchart" in the template search bar. Choose a suitable template or start with a blank canvas.
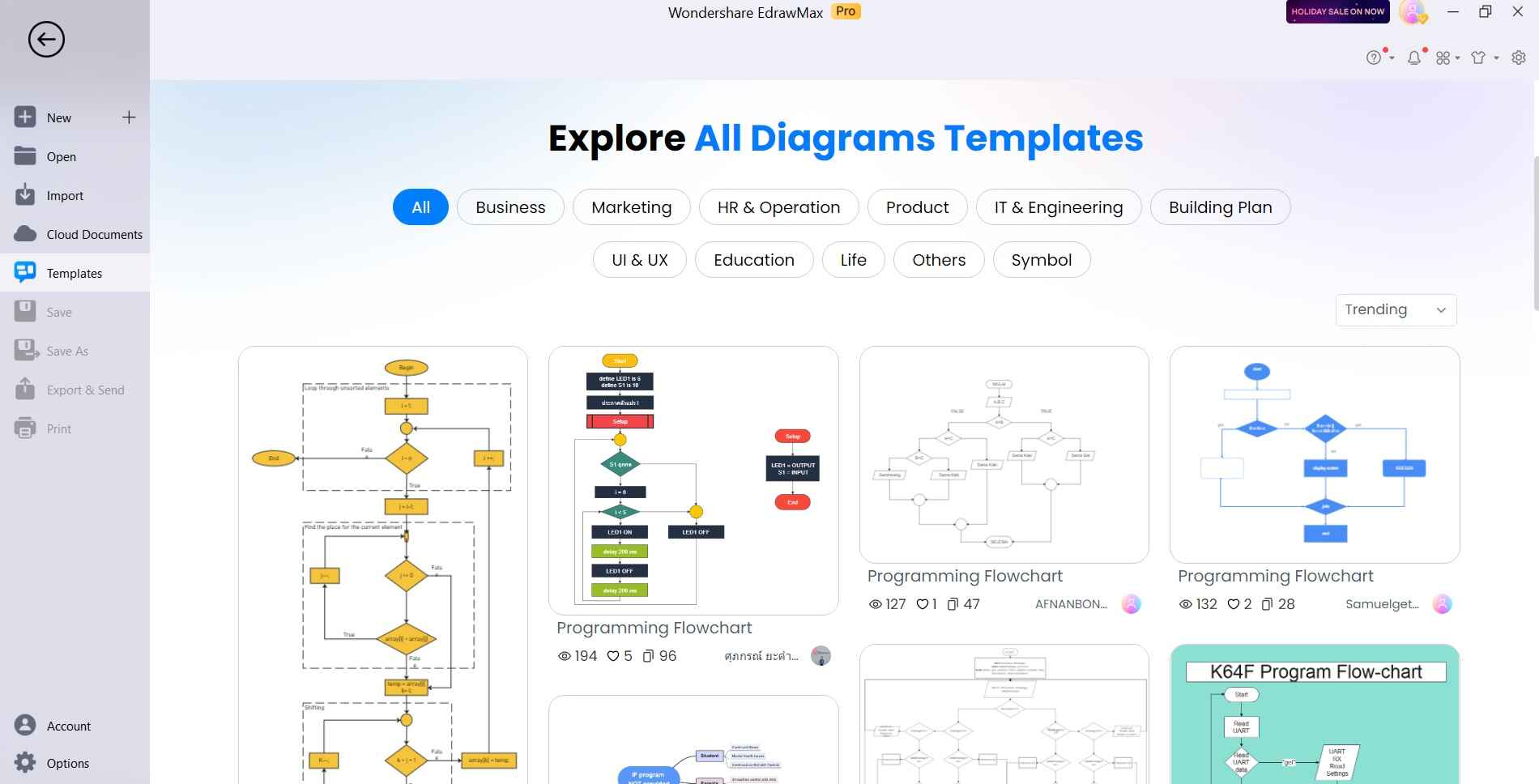
Step 2:
Use the various shapes and symbols available in the toolbox (e.g., start/end, process, decision, input/output) and drag them onto the canvas.
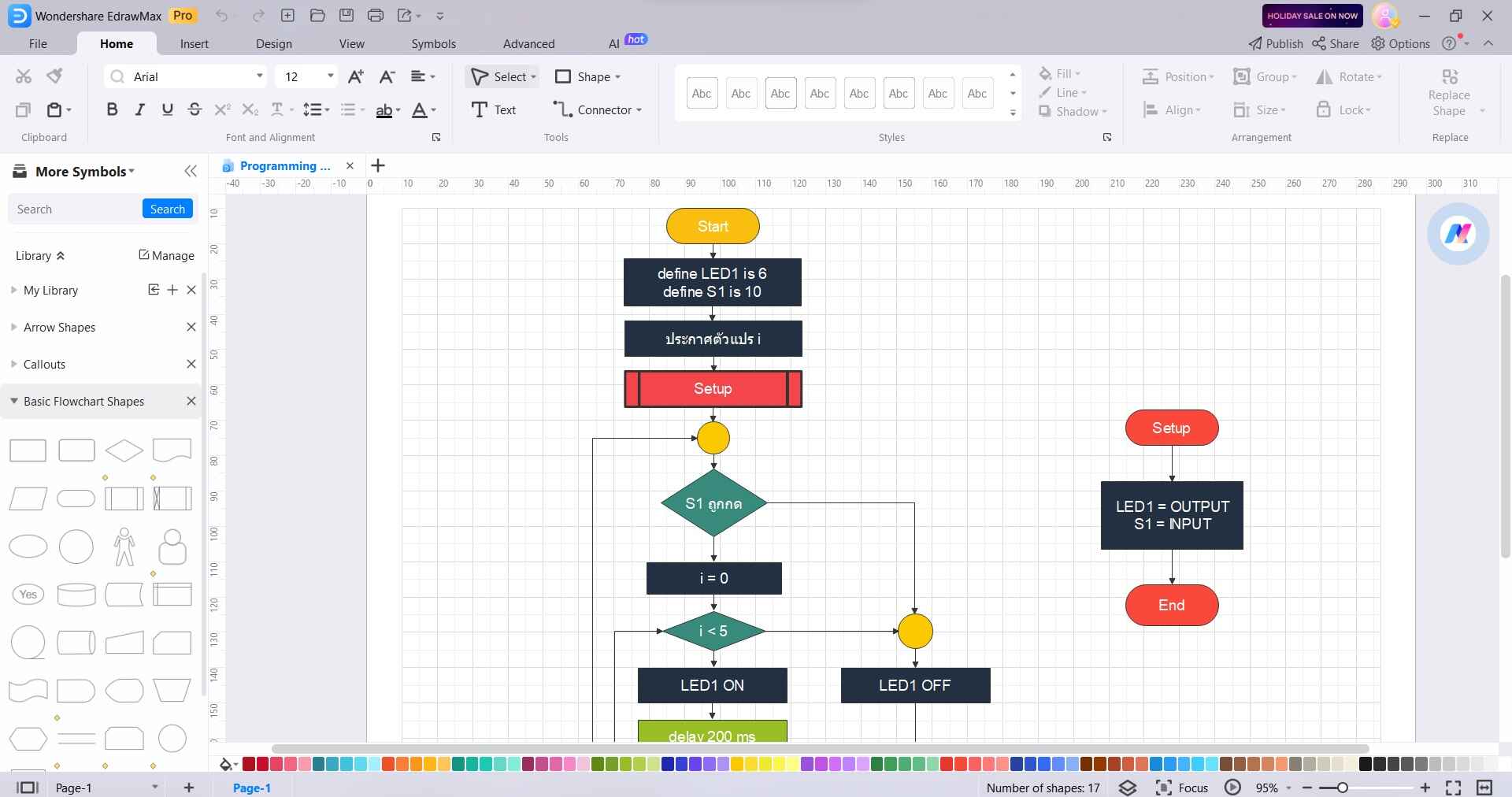
Step 3:
Connect the shapes using arrows or connectors to illustrate the flow of the algorithm.
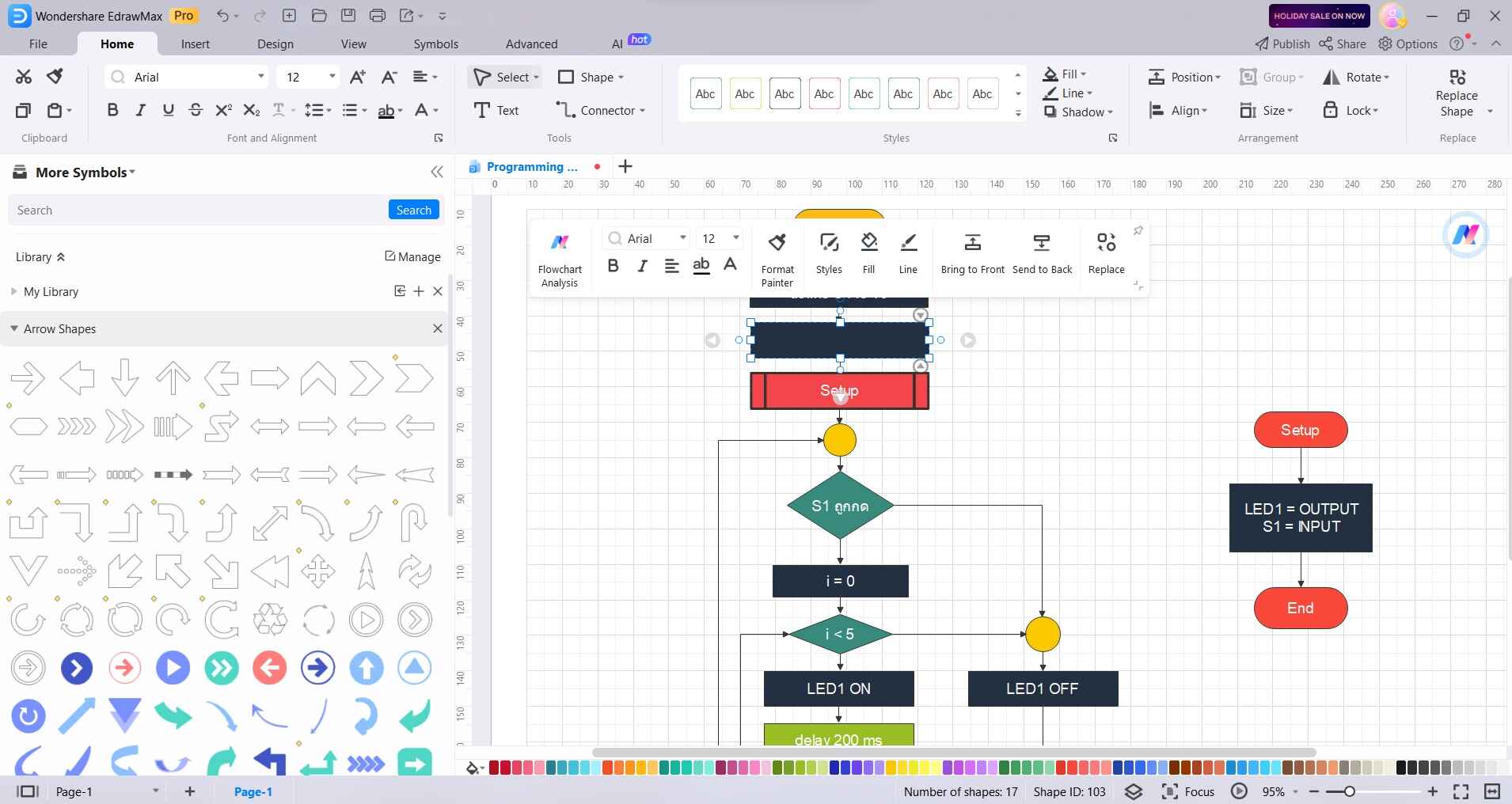
Step 4:
Organize the flowchart elements in a logical sequence, adjusting the layout and formatting (colors, styles, fonts) to enhance clarity and readability.
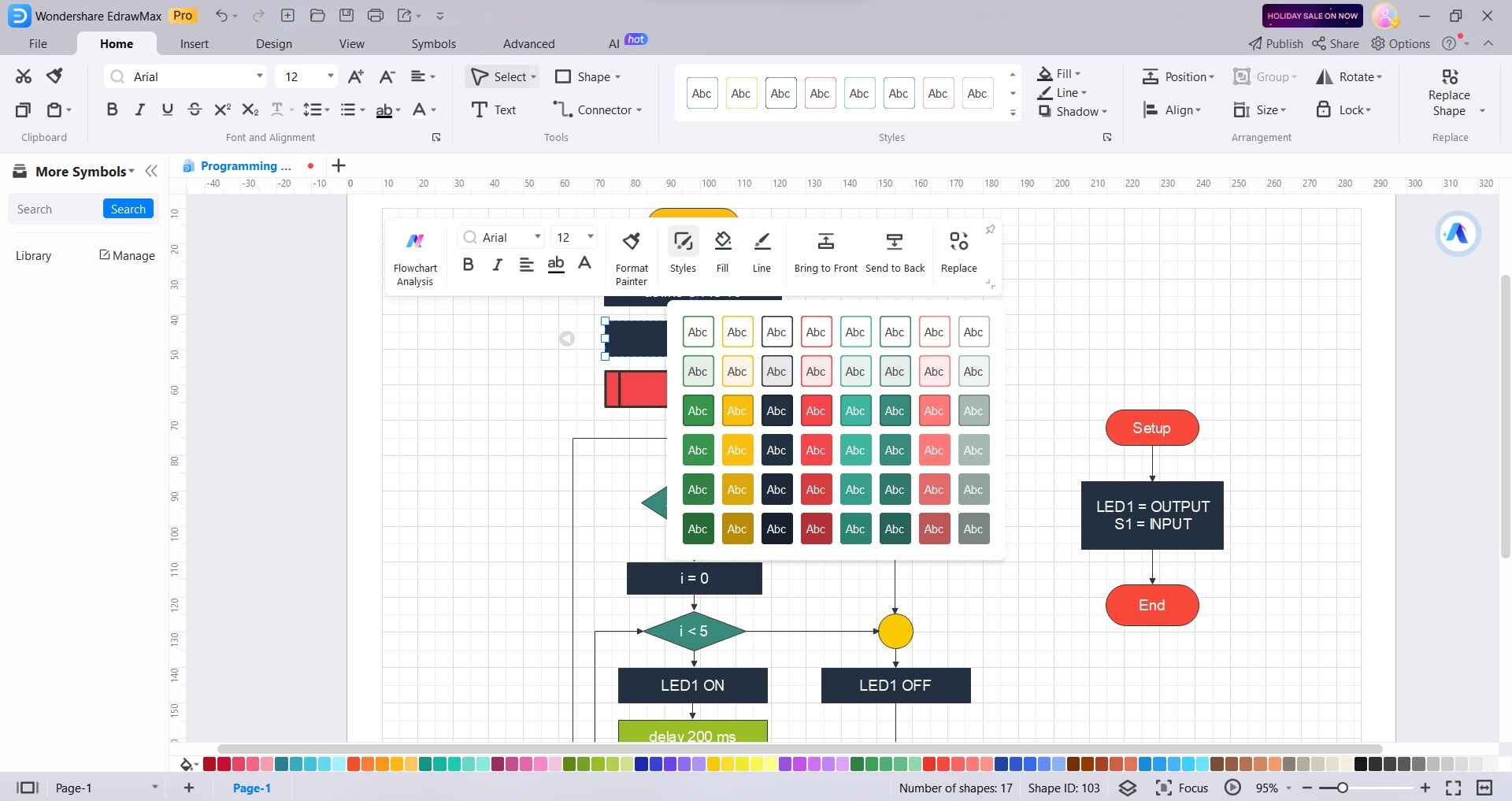
Step 5:
Once satisfied, save your work in the desired format (e.g., .eddx, .pdf, .png) to retain and share your programming algorithm flowchart.
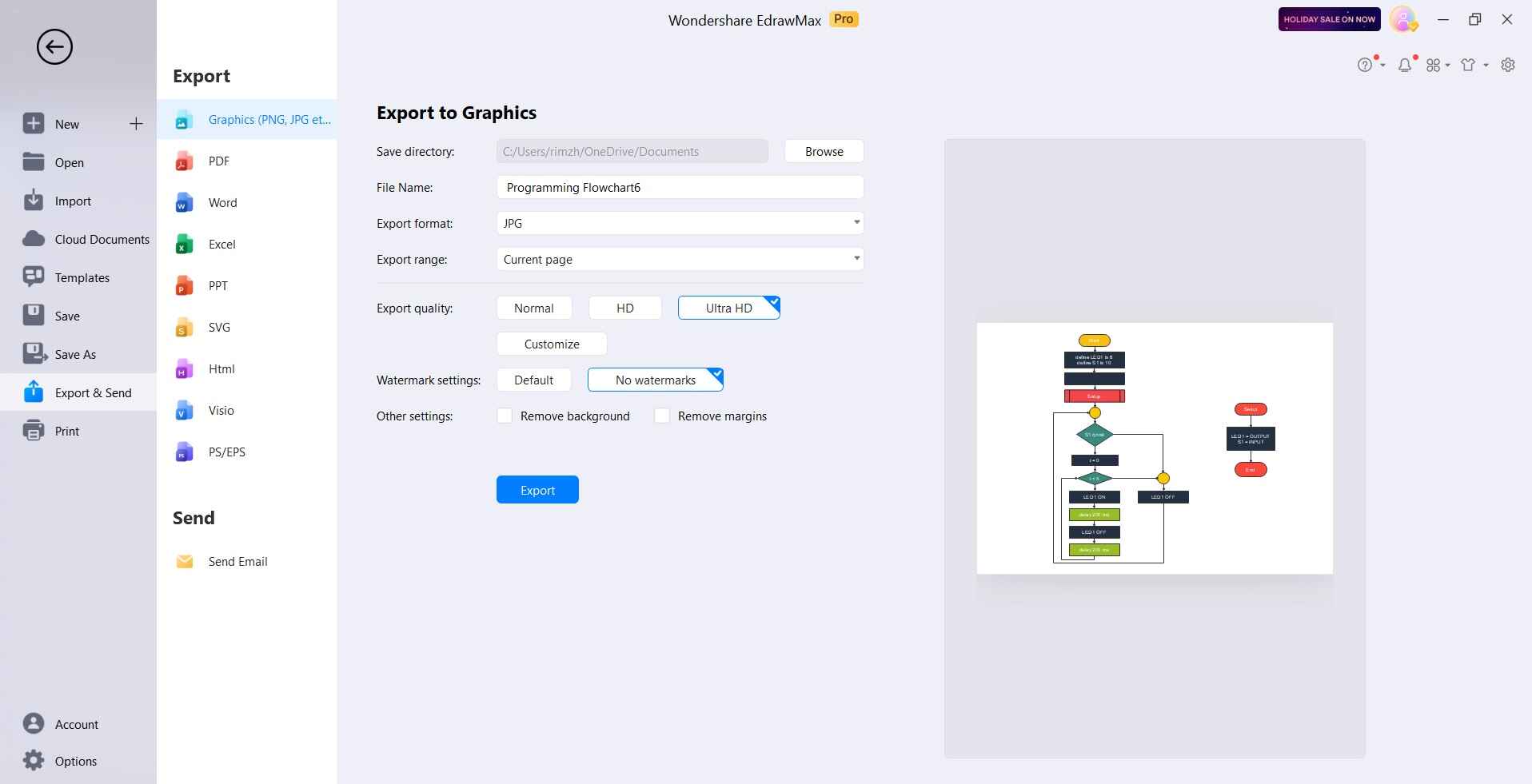
EdrawMax simplifies the process with its user-friendly interface and versatile tools, allowing for the creation of clear and comprehensive programming algorithm flowcharts.
Conclusion
Golang's struct construct allows for easy organization of related data into reusable types. Anonymous, generic, and embedded structs provide further functionality. When planning out Golang structs for a project, considerations around encapsulation, naming, composition, and documentation help create robust data structures. Tools like EdrawMax facilitate nice program visualizations showing how different structs connect and interact across the codebase.