Golang (or Go) is an open-source programming language developed by Google that has gained immense popularity over the last decade. It's known for its simplicity, concurrency support, scalability, and more. One of the key components in Go is structs - composite data types that allow related data to be grouped together.
Here we will provide an in-depth overview of Go structs, the various types, things to consider when creating structs, and how to visualize them with flowcharts.
In this article
Part 1: What is a Golang Struct?
A struct in Go is a user-defined type that allows you to combine multiple different types of data into a single structured data type. Structs are useful for grouping related data together so you can treat the data as a cohesive whole.
Structs are extremely useful in Go for organizing related data and defining custom data types with different behaviors. Properly initializing them is key.
Part 2: Overview of Golang Struct to Map
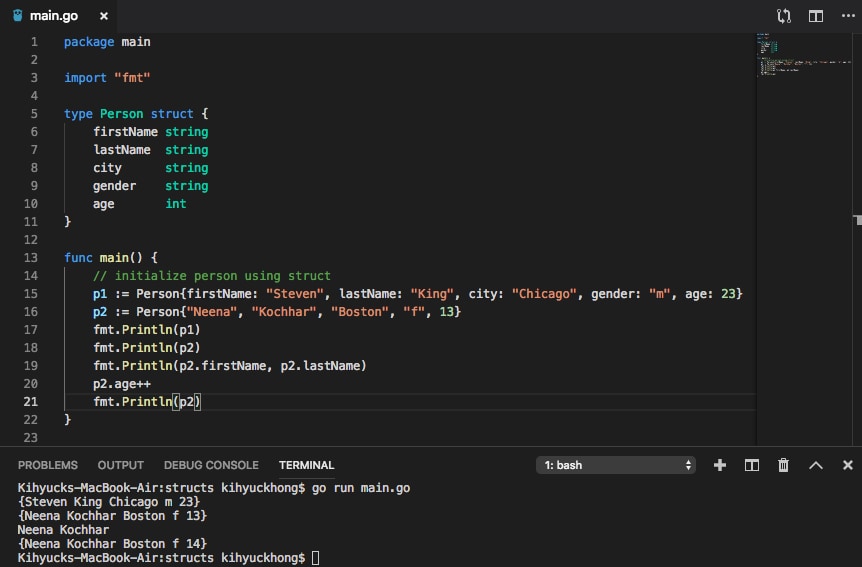
Before we look at initializing a struct, it's important to understand the relationship between structs and maps in Go.
Maps are built-in associative data structures in Go that let you store data as key-value pairs. A key maps to a value. Structs and maps support many of the same operations for getting/setting data based on a key.
But structs provide additional behavior that makes them more useful for data modeling:
- Field names - Struct fields have named keys defined in the struct type.
- Validation - The struct type defines the exact data types for each field.
- Methods - Methods can be added to struct types to define behaviors.
So structs provide data modeling capabilities similar to classes in OOP languages. A struct type defines the shape of the data.
Under the hood, structs actually map field names to values in an underlying map structure. So there is a relationship between structs and maps.
This is important when initializing structs because map syntax can be used to set initial field values.
Part 3: Steps in the Golang Struct Initialization Process
The process of Golang Structs Initialization process involves the following steps:
- Definition: You start by defining a struct type. This defines the blueprint of the structure, specifying its fields and their types.
- Declaration: To create an instance of the struct, you declare a variable of that struct type. This reserves memory for the struct in which you can store values for its fields.
- Initialization: Once the variable is declared, you can initialize the fields of the struct with values. This can be done either during the declaration or by assigning values to the fields afterward.
- Accessing and Modifying: After initialization, you can access the fields of the struct using the dot notation. This allows you to read the values stored in the fields or modify them as needed.
- Constructor Functions (Optional): Sometimes, constructor functions are used to initialize structs with specific values or perform additional setups before returning the initialized struct.
In essence, struct initialization in Go involves defining the structure, declaring variables of that type, assigning values to its fields, and then interacting with those fields as required.
Part 4: Golang Struct Example
Let's look at a full example to see struct initialization in practice.
We'll define a struct to represent blog posts:
type Post struct {
Title string
Content string
Author User
Comments []Comment
}
type User struct {
Name string
}
type Comment struct {
Body string
User User
}
Here Post has a few different fields, including nested User and Comment structs.
Part 5: Creating a Programming Diagram Using EdrawMax
When dealing with complex nested structs, visually diagramming out the relationships can be extremely helpful. That's where a visualization tool like EdrawMax comes in very useful for software development.
EdrawMax makes it easy to diagram programming constructs through simple drag-and-drop shapes and connections. For structs, you could create boxes for the different struct types with fields listed within them.
EdrawMax has robust features focused specifically on programming and software diagrams. Building programming diagrams helps improve documentation and communication for developers and architects. Especially for teaching Go struct syntax and initialization to others, a simple reference diagram can help grasp concepts faster.
Here are the steps to create a simple programming diagram using EdrawMax:
Step 1:
Launch the EdrawMax application on your computer. Look for the "Flowchart" or "Software and Database" category. You might find programming-related diagram templates there.
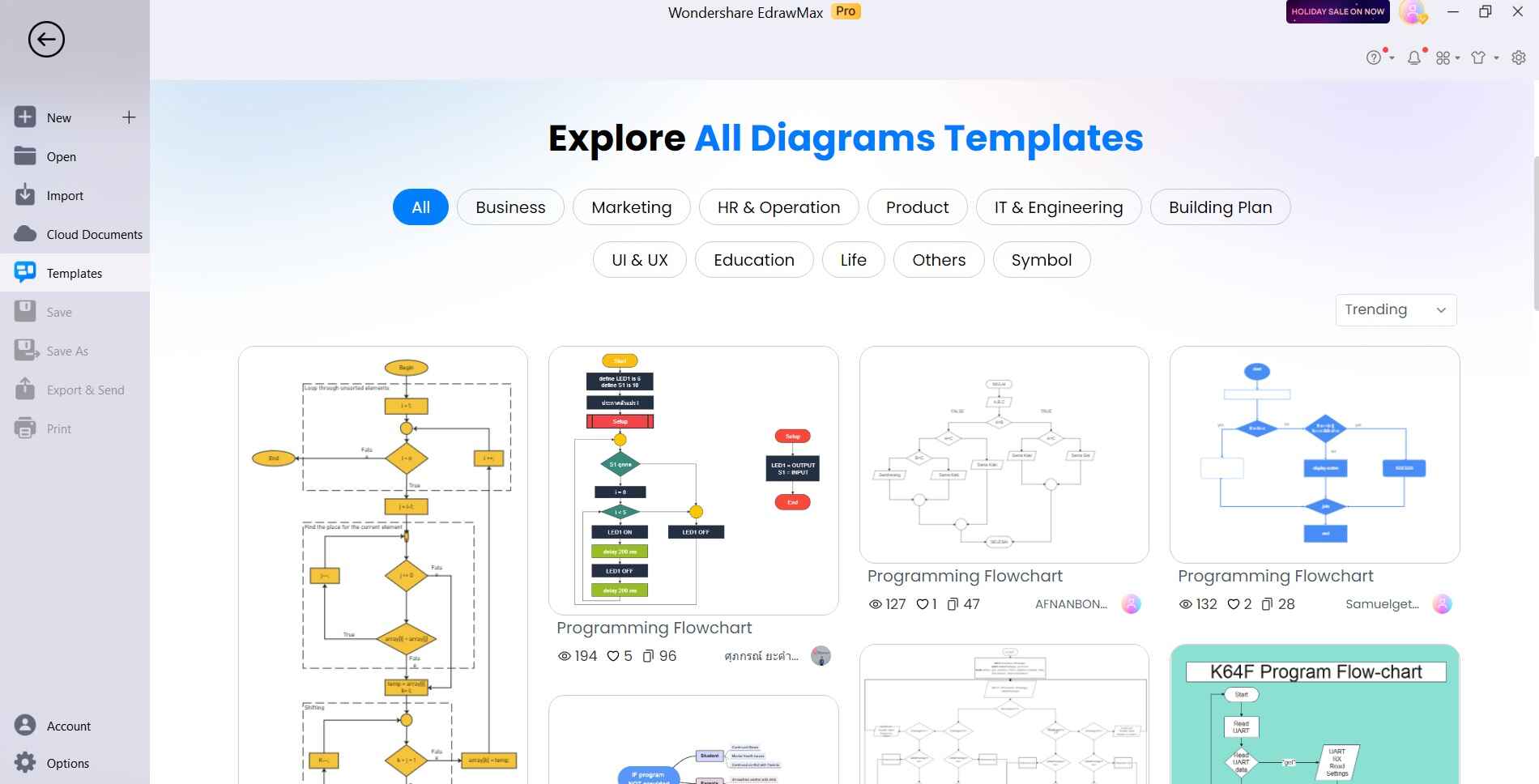
Step 2:
Drag and drop shapes/symbols onto the canvas to represent different programming elements such as processes, decisions, loops, inputs, outputs, etc.
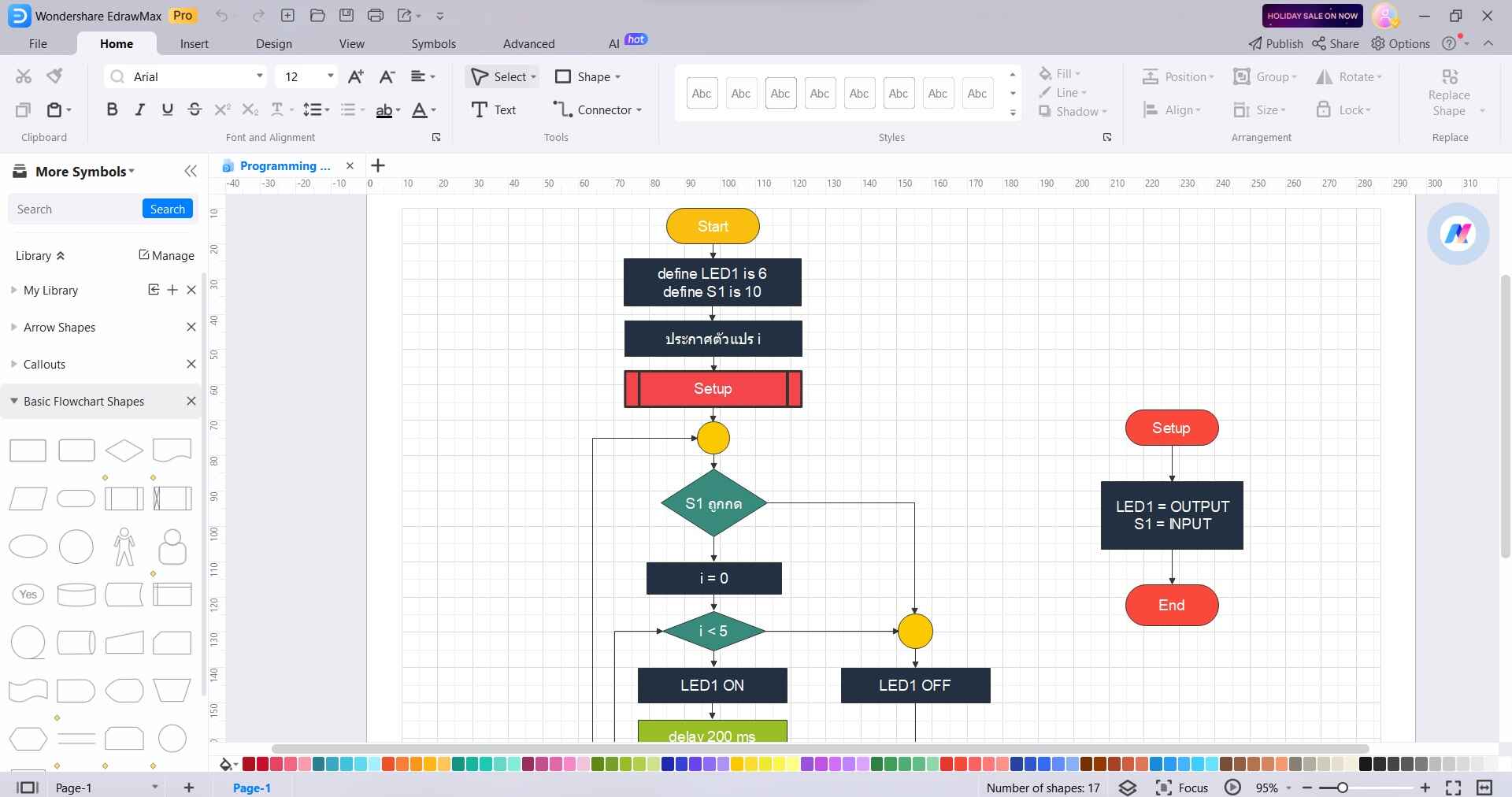
Step 3:
Use connectors or lines to link the shapes in the sequence you desire, illustrating the flow of the program.
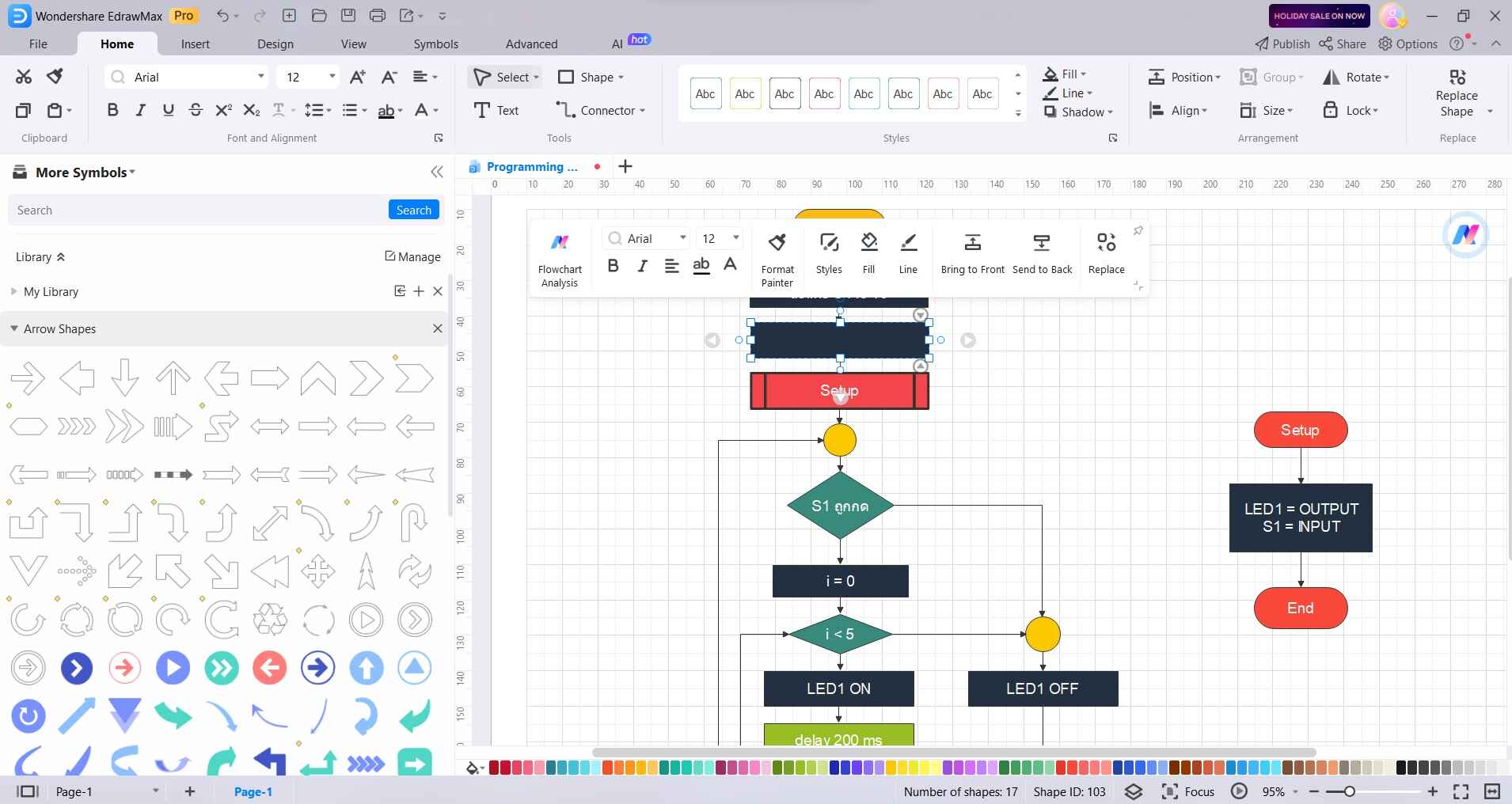
Step 4:
Customize the shapes, lines, and text by changing colors, fonts, sizes, and styles to make the diagram visually appealing and understandable.
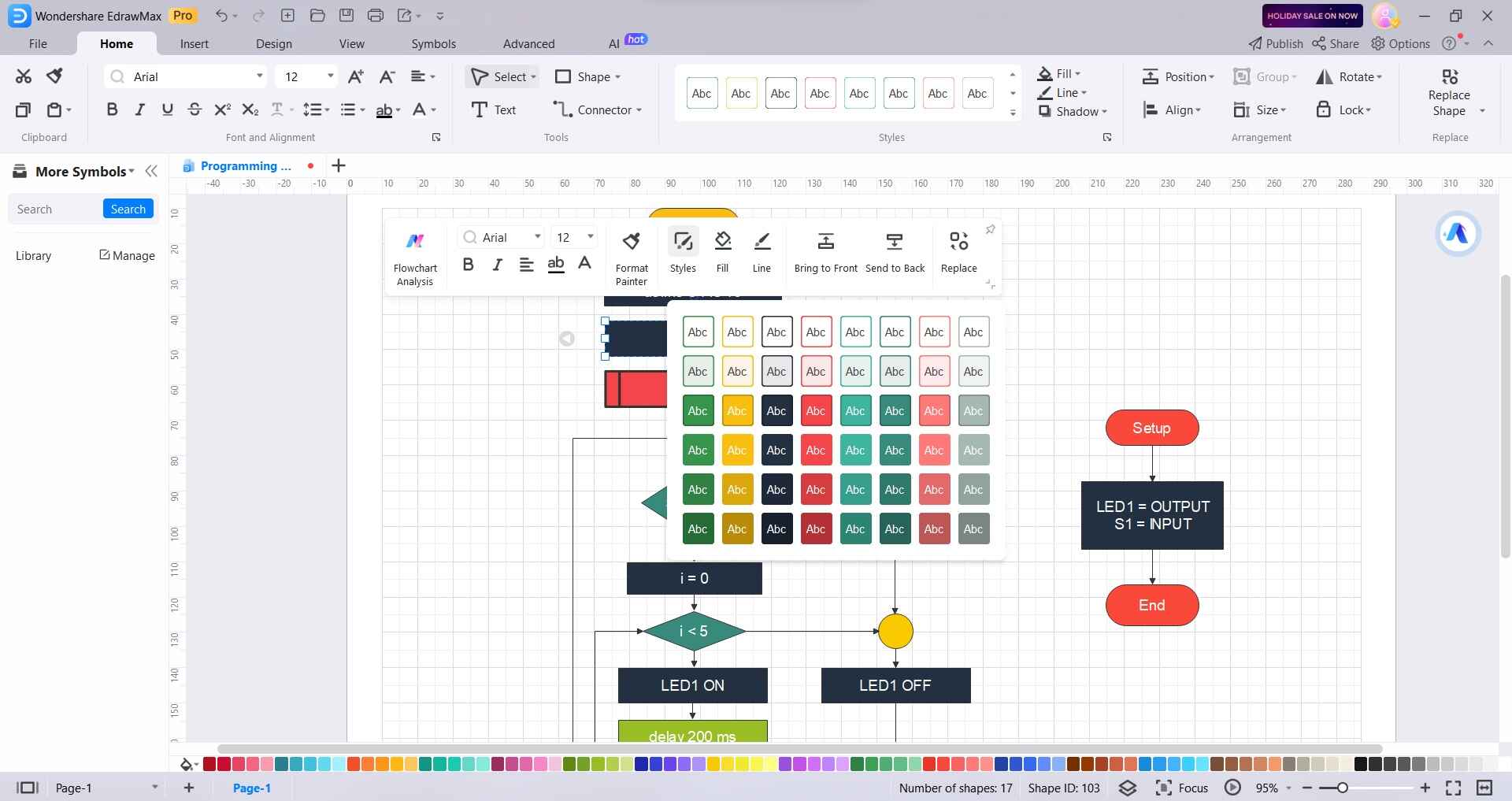
Step 5:
Once your diagram is complete, save your work in EdrawMax's format (.eddx) or export it to common formats like PNG, JPG, PDF, etc., based on your needs.
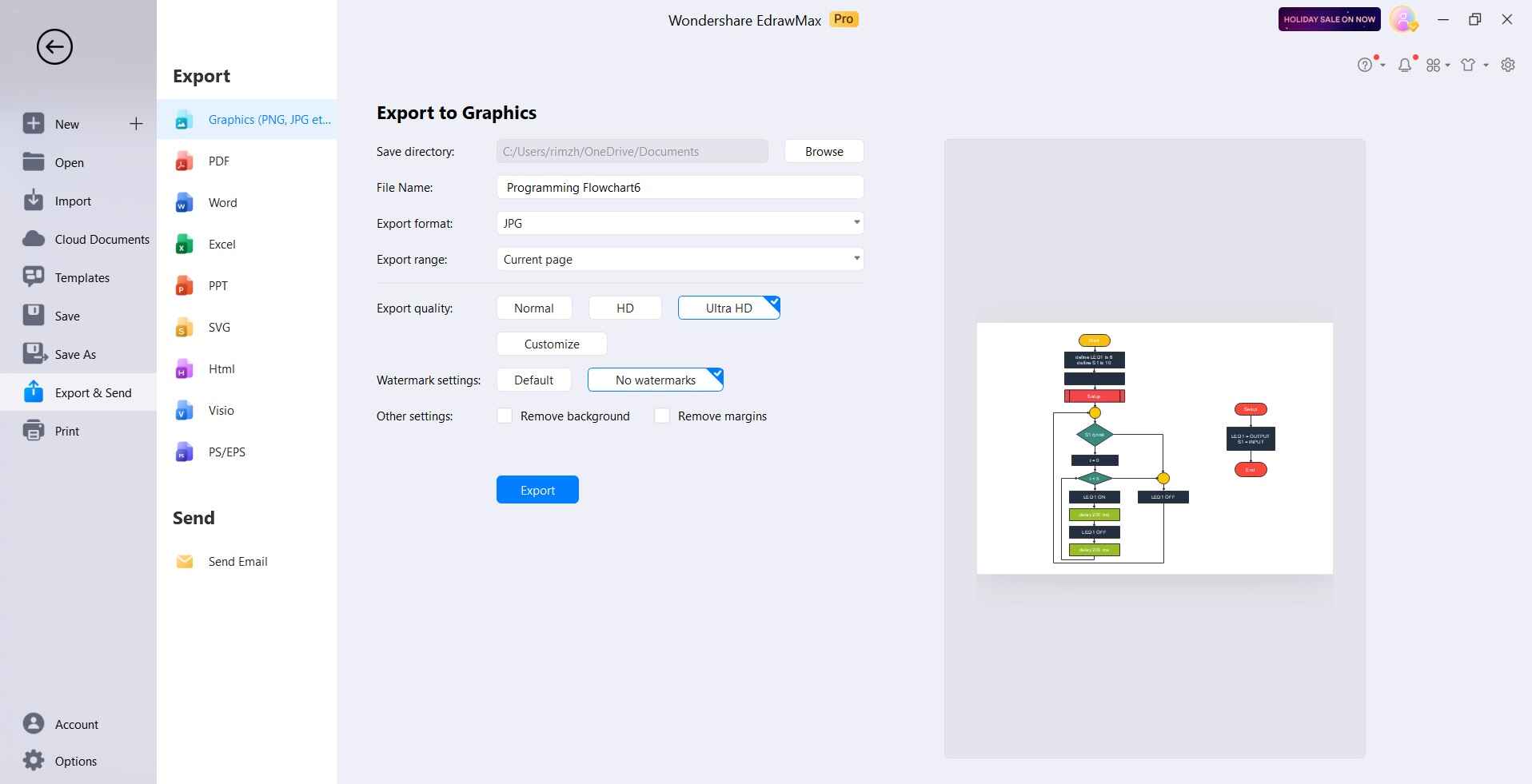
EdrawMax provides a user-friendly interface with a wide variety of shapes, symbols, and customization options to create programming diagrams easily.
Conclusion
Structs allow developers to define custom data structures that combine multiple data types. Initializing them properly when creating new struct instances is important for effectively grouping related data.
Visualizing struct relationships in diagrams makes initialization easier even for nested struct combinations. Tools like EdrawMax simplify software diagramming for structs and other programming concepts.