Efficient code optimization is crucial for software performance and scalability. Two key areas to focus on are the stack and heap data structures used by a program. Optimizing usage of the stack and heap can greatly improve code efficiency.
In this article, we will provide an overview of the stack and heap and discuss techniques to optimize them.
In this article
Part 1: Overview of Stack and Heap
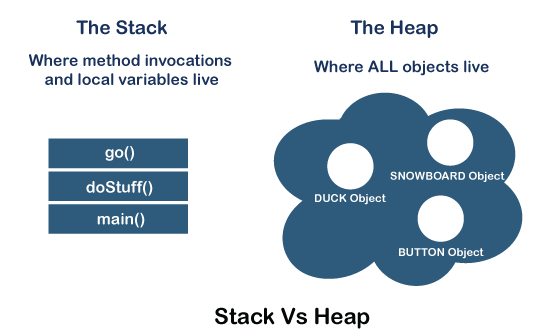
The stack and heap are two memory areas allocated for a running program. The stack stores temporary scalar variables and function parameters, while the heap stores larger, more dynamic objects that live beyond the scope of a function.
Properly utilizing the stack and heap can optimize performance. The stack uses much faster sequential memory access compared to the heap's slower, more fragmented access. Minimizing heap allocations/deallocations and avoiding unnecessary copies between the stack/heap are two key optimization techniques.
Part 2: What is a Heap Tree?
A heap tree is a specialized tree-based data structure that satisfies the "heap" property - the value stored at each node must be greater than or equal to (or less than or equal to) the values stored at child nodes.
Heaps are commonly implemented using arrays or binary trees. In a max binary heap, each node's value is greater than or equal to its two children. Heaps provide efficient constant time lookup of extreme values - useful for implementing priority queues.
Part 3: Steps to Implement Heap in C#
Here are the key steps to implement a basic max heap in C#:
- Create a Heap class with properties for the array of elements, current count/size, and max capacity
- Write a GetParentIndex method to fetch the parent node index from a child index
- Implement SiftUp to place a new element in the correct spot to maintain heap ordering
- Implement SiftDown to reorder elements after removing the root to maintain heap ordering
- Create a peek method to fetch the maximum element at the root
- Implement insert and remove methods to add/delete elements per max heap rules
- Include other useful methods like count property, is empty check, to string override, etc
- Create a Main method to test core functionality like insert, remove, and print heap
Using the above steps allows the creation of a functional heap for use in priority queues, graph algorithms, and optimization problems in C#.
Part 4: Difference Between Sorted Heap and Fibonacci Heap Python
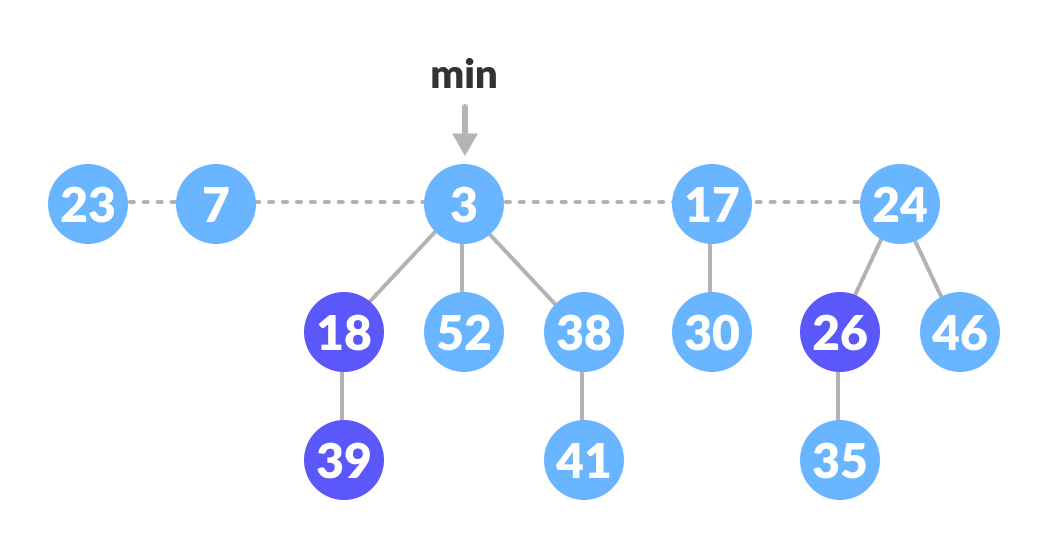
Though both sorted heaps and Fibonacci heaps satisfy the heap property in Python, there are some key differences:
- Sorted heaps are heaps stored in sorted order - child nodes are sorted relative to siblings. Fibonacci heaps store elements without any sorting order.
- Sorted heaps support faster lookup of extreme values but slower insertion/removal in O(n) compared to Fibonacci heaps with O(1) insertion and O(log n) removal.
- Fibonacci heaps were created to optimize the speed of these dynamic operations by using a more complex tree structure compared to typical sorted heaps.
So in summary - sorted heaps provide the fastest read performance while Fibonacci heaps provide the fastest update performance. The choice depends on whether a dynamic priority queue is needed vs mainly extracting extreme values.
Part 5: Creating a Programming Flowchart Using EdrawMax
EdrawMax is a comprehensive diagramming and charting software that can help create programming flowcharts that document code structure and logic flow.
Here are some benefits of using EdrawMax:
- User-friendly interface - Easy drag-and-drop toolbox makes creating flowcharts intuitive
- Customization - Flexible styling options allow customizing shapes, fonts, colors
- Integration - Charts can be exported to various document formats like Word, Excel
- Symbols - Provides standard flowchart symbols for common programming constructs
- Automation - Features like auto-alignment streamline diagram creation
- Sharing - Enables collaboration with a team by exporting editable diagrams
With the rich flowcharting capabilities EdrawMax provides, programmers can quickly diagram complex program logic to aid development and maintenance. The integration potential also helps share these diagrams with stakeholders in readable formats. These features make EdrawMax an essential toolkit item for any programmer.
Here are the steps to create a simple Fibonacci series algorithm flowchart using EdrawMax:
Step 1:
Launch the EdrawMax software on your computer. Look for the "Flowchart" category or search for "Flowchart" in the search bar. Select a template or a blank canvas to start creating your flowchart.
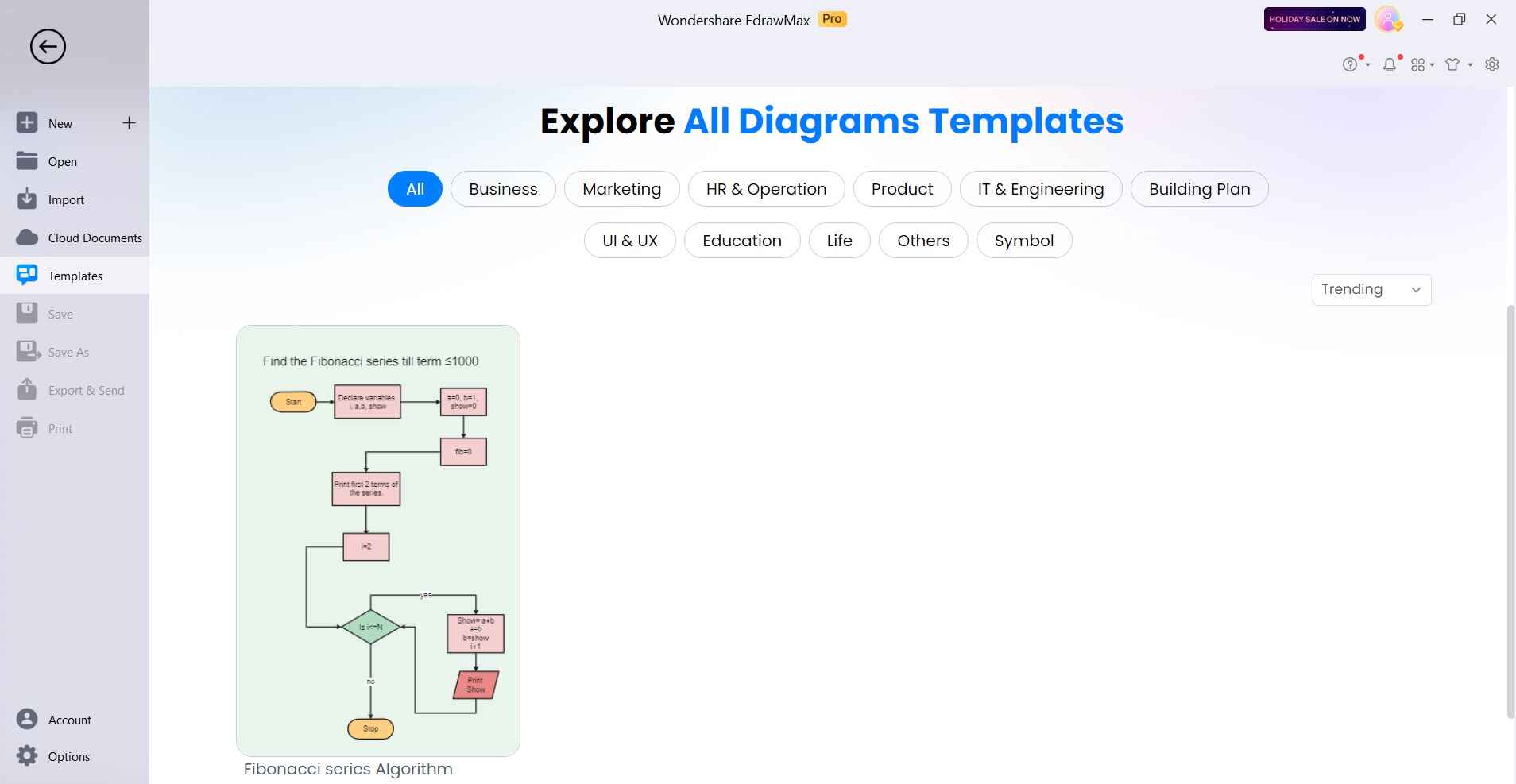
Step 2:
Drag and drop shapes from the left-hand side panel onto the canvas.
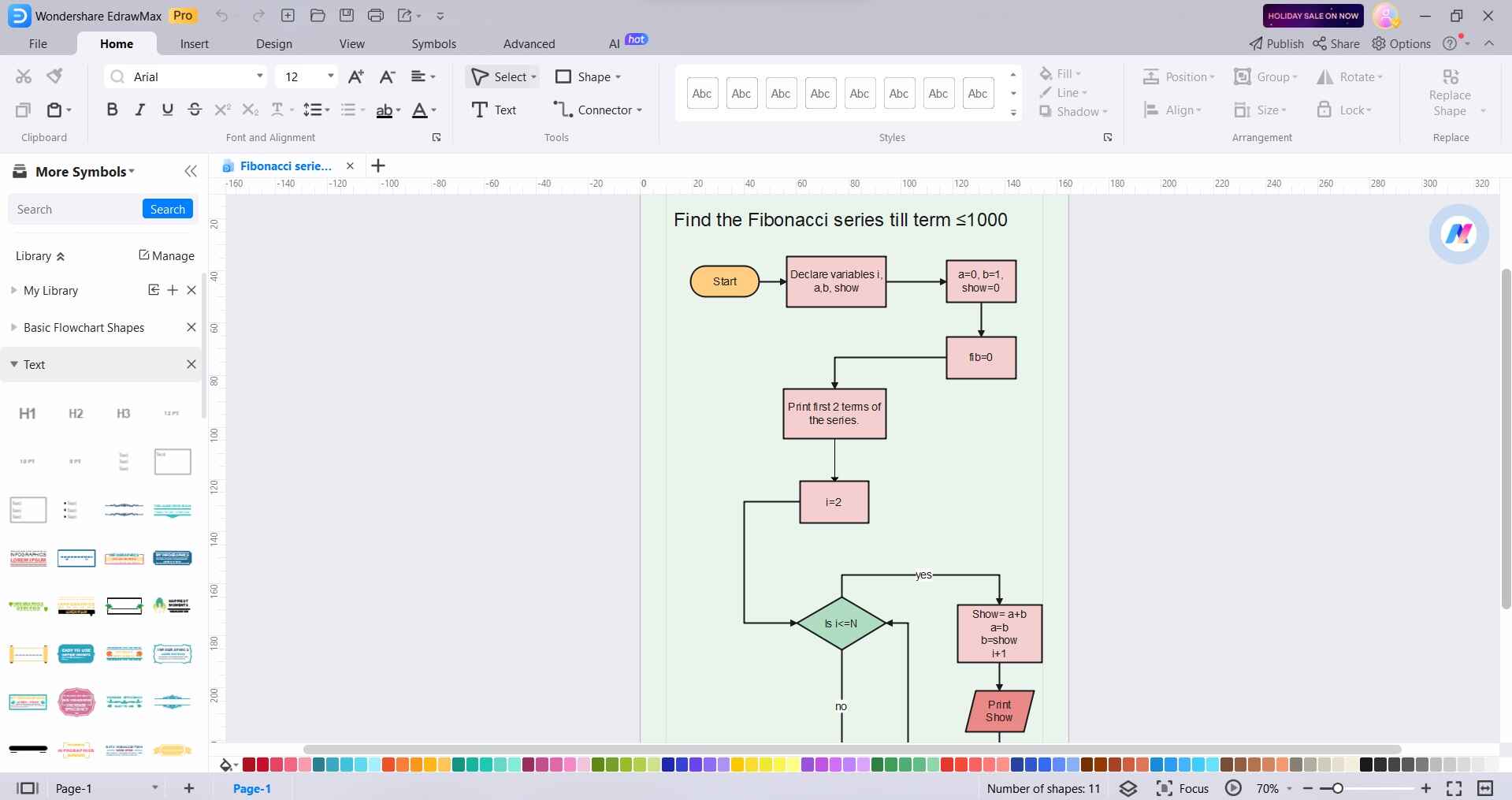
Step 3:
Double-click on each shape to add text or labels indicating actions or decisions. Include clear and concise descriptions for each step in your program.
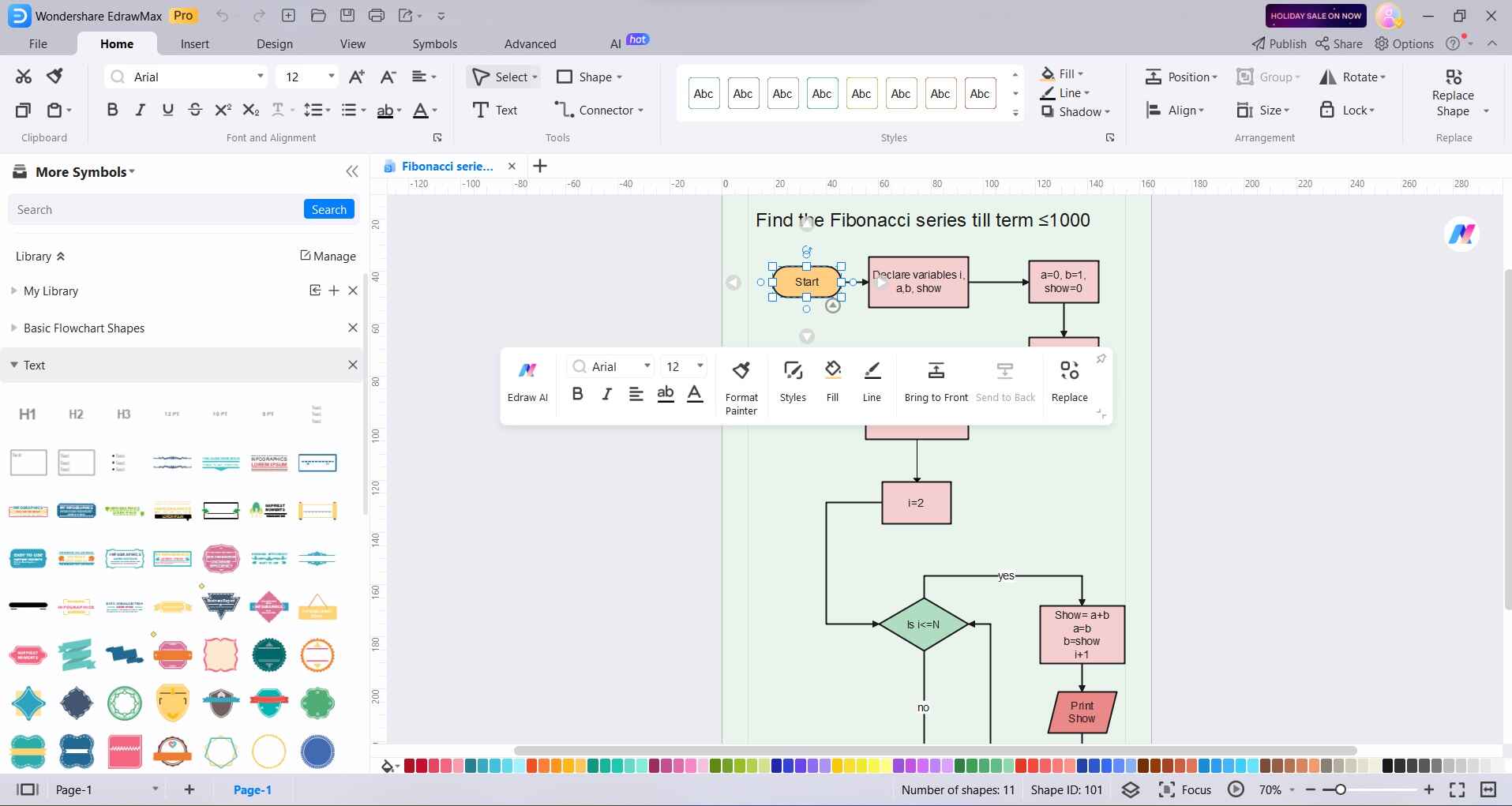
Step 4:
Modify the flowchart's appearance by adjusting colors, dimensions, fonts, and designs, enhancing its visual appeal and clarity.
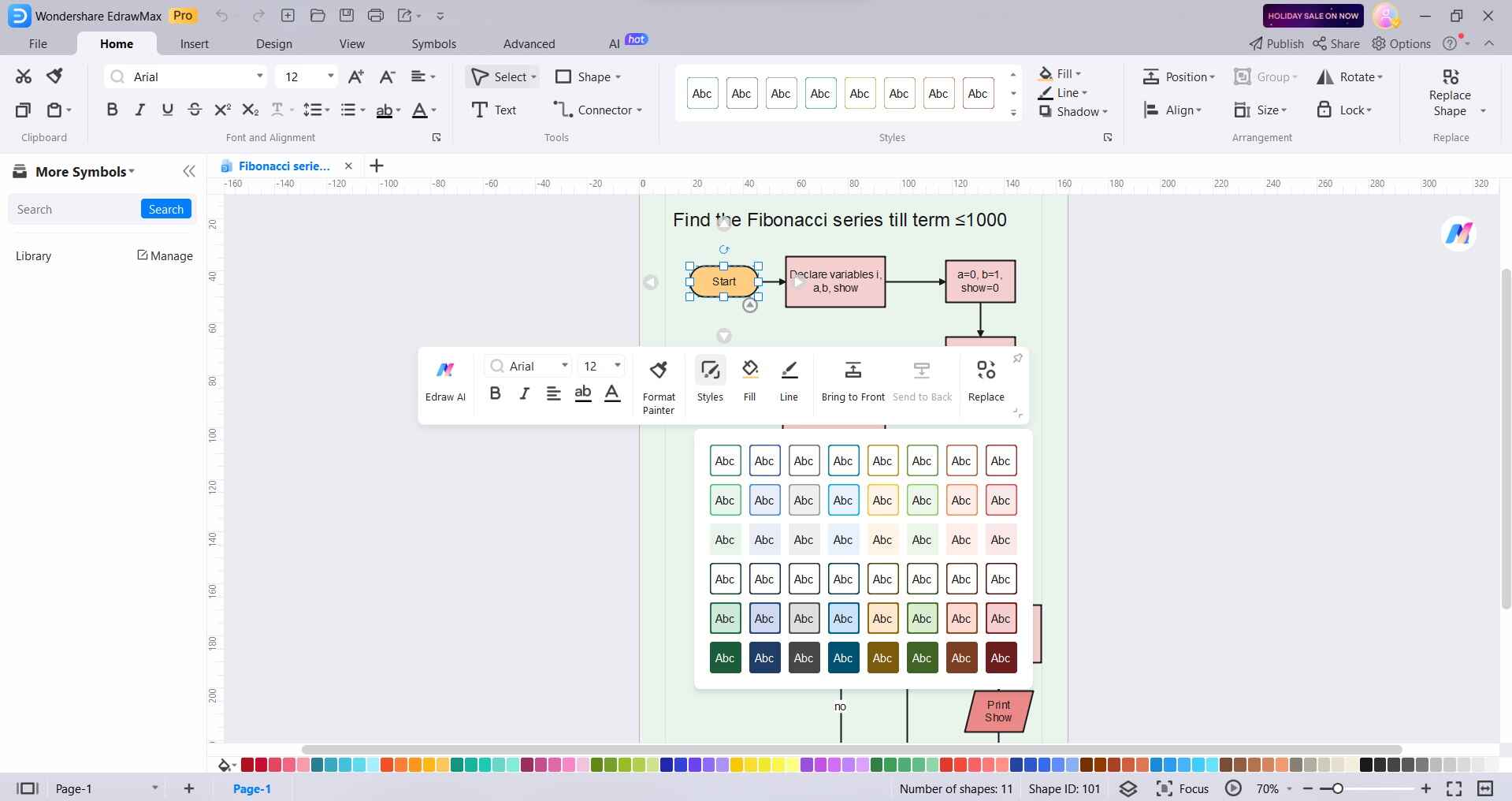
Step 5:
Once you're satisfied with your flowchart, save your work. EdrawMax allows you to save your flowchart in various formats such as JPEG, PNG, PDF, etc., making it easy to share or use in presentations or documents.
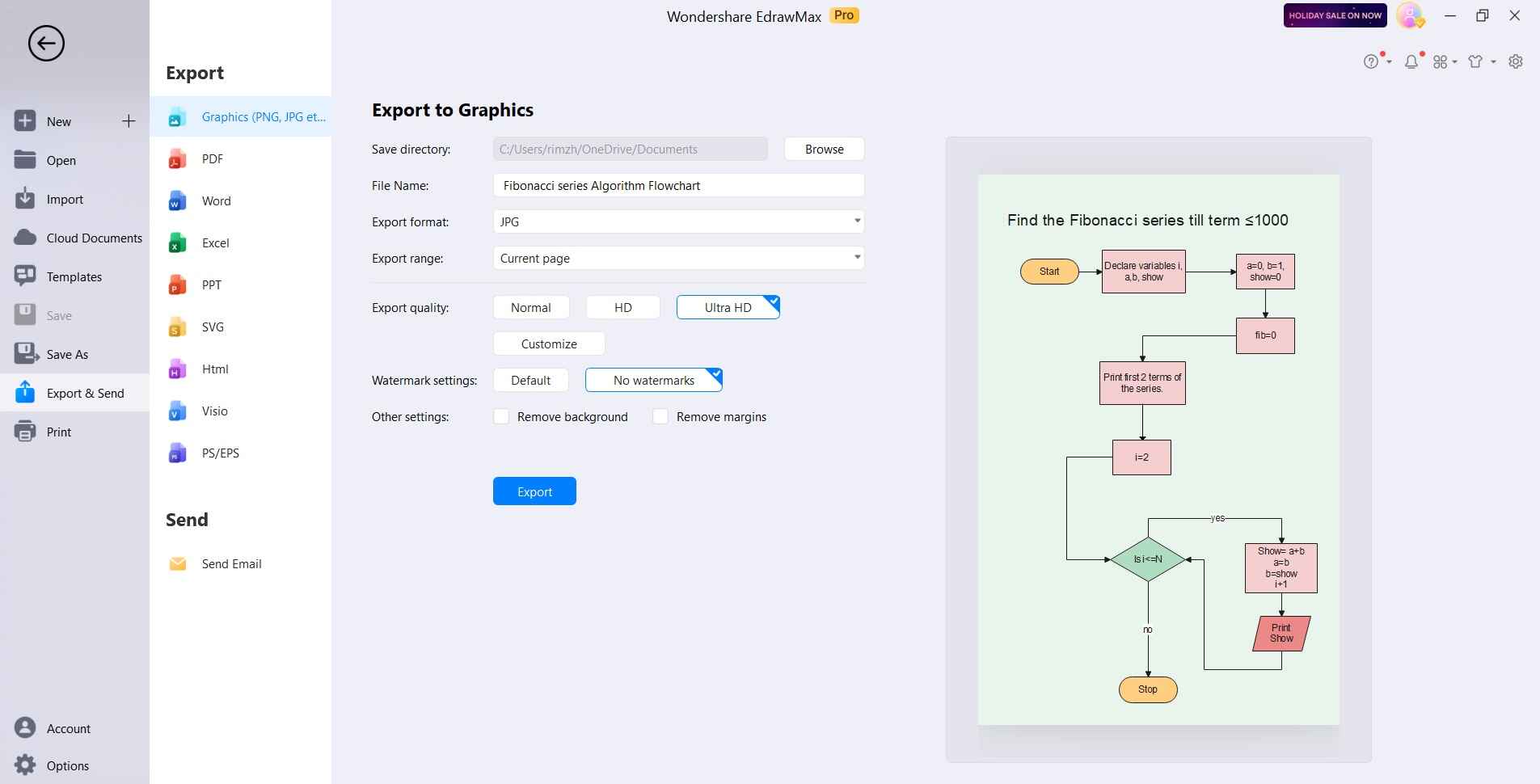
Following these steps will help you create a simple programming flowchart using EdrawMax, allowing you to visually represent the logic and structure of your program.
Conclusion
Optimizing code efficiency involves techniques like minimizing heap allocations, avoiding unnecessary stack/heap copies, and using efficient data structures like heaps. Heaps provide fast access to extreme values and underlie structures like Fibonacci heaps that optimize updates. Diagramming code logic using tools like EdrawMax also aids development.