Linked lists are one of the fundamental data structures used in computer programming. They provide an efficient and flexible way to store and organize data. In this article, we will dive into the details of linked lists in data structure programs – how they work, their key components, different types, and some best practices when implementing them.
By the end, you will have a strong conceptual understanding of this important data structure.
In this article
Part 1: What is a Linked List in Data Structure Program?
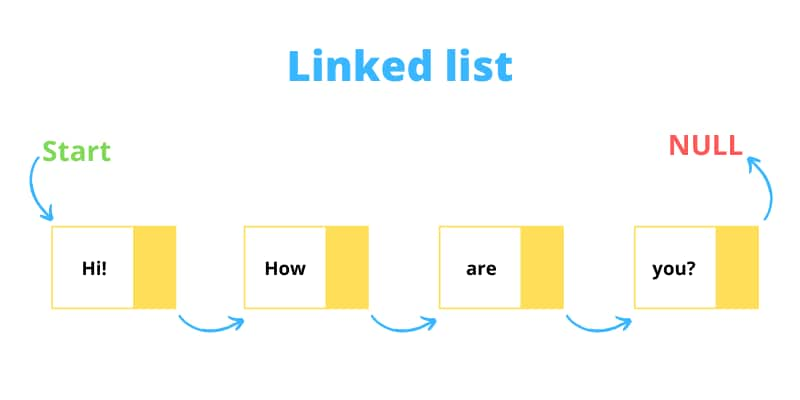
A linked list is a linear data structure consisting of nodes that are connected via pointers. Each node contains data and a pointer that points to the next node in the sequence. The first node is called the head while the last node points to null instead of another node. Linked lists do not require contiguous memory allocation like arrays, making them dynamic and efficient for inserting and removing data.
Overall, linked lists allow programmers to organize data in ways that arrays cannot.
Part 2: Key Components in Linked List in Data Structure Program
Now that we understand what linked lists are, let’s go through the key components that make them up:
- Data – Each node in a linked list holds some data. This can be any data type like int, string, object, etc. The power of linked lists comes from being able to string together nodes holding different kinds of data.
- Pointer to next node – Nodes connect through a pointer reference, commonly called “next.” Each node stores a pointer or address that leads to the next node. The last node holds a null pointer to indicate there are no more nodes after it.
- Head pointer – The head node is the very first node in a linked list. It is important to keep track of the head to be able to traverse the full list starting there.
- Tail pointer – The last or tail node allows easy appending to the linked list since you can change what it points to quickly.
- Size – Tracking the size or length of the linked list can be useful to quickly determine how many elements you have. Size helps write algorithms that rely on the number of nodes.
The connections created by pointers between nodes give linked lists flexibility in organizing data dynamically. Understanding how to navigate those connections is key.
Part 3: Types of Linked Lists in Data Structure in C
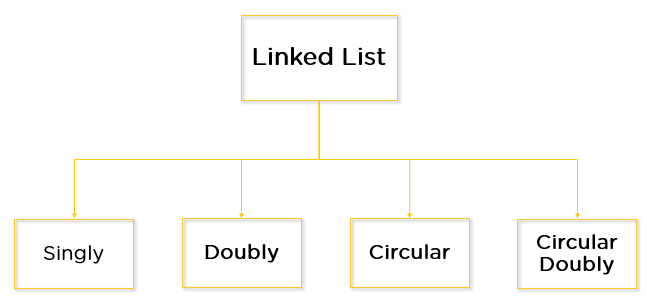
There are a few different types of linked lists commonly used in programming:
- Singly Linked Lists – The singly linked list in data structure programs in C is the most basic version with nodes that store data and point to the next node location. Easy to implement but can only traverse in one direction.
- Doubly Linked Lists – A doubly linked list in data structure programs adds node pointers to both the next and previous nodes. More memory but allows traversing backward.
- Circular Linked Lists – In a circular queue in data structure programs, the final node points back to the head rather than null terminating. Useful for certain cases like buffer implementation.
- Multilinked – Nodes point to multiple other nodes, not just singular next. Allows complex connections beyond the linear next node.
Knowing these types allows you to choose the right linked list for the program’s needs.
Part 4: Queue Data Structure Program In C Vs Selection Sort In Data Structure Program
It can be useful to contrast linked lists with other common data structures. Let’s explore how linked lists compare to the queue and selection sort algorithms.
Queues operate in a first-in, first-out manner (FIFO) which is quite different from linked lists. However, linked lists allow flexibility in node order that queues do not. Linked list nodes can be rearranged and inserted anywhere needed. Queues only remove or dequeue from the front.
On the other hand, a selection sort examines an array, selects the smallest element, and exchanges it into the first position continuing progressively.
Part 5: Creating a Programming Diagram Example Using EdrawMax
Visualizing programming concepts like linked lists through diagrams provides clarity and aids understanding.
EdrawMax simplifies diagramming complex programming constructs through point-and-click ease and powerful customization. The extensive shape libraries make EdrawMax widely used for things like UML, flowcharts, mind maps, and more.
Here are the steps to create a sorted array programming flowchart using EdrawMax:
Step 1: Open the EdrawMax application on your device. Select the "File" menu and choose "New." Or from the templates provided, select "Sorted Array Flowchart".
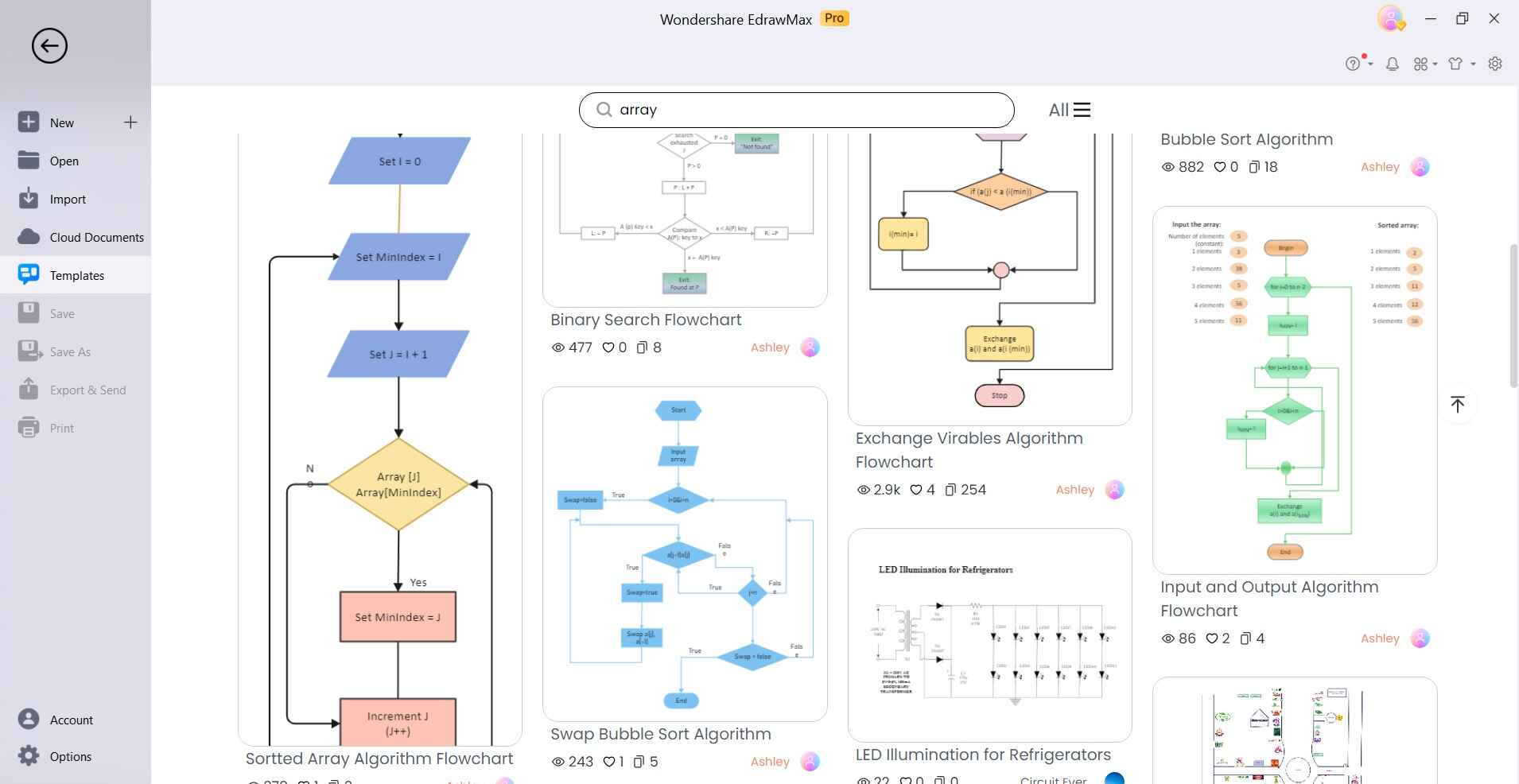
Step 2: Use the toolbar or drag-and-drop interface to add shapes and symbols onto the canvas.
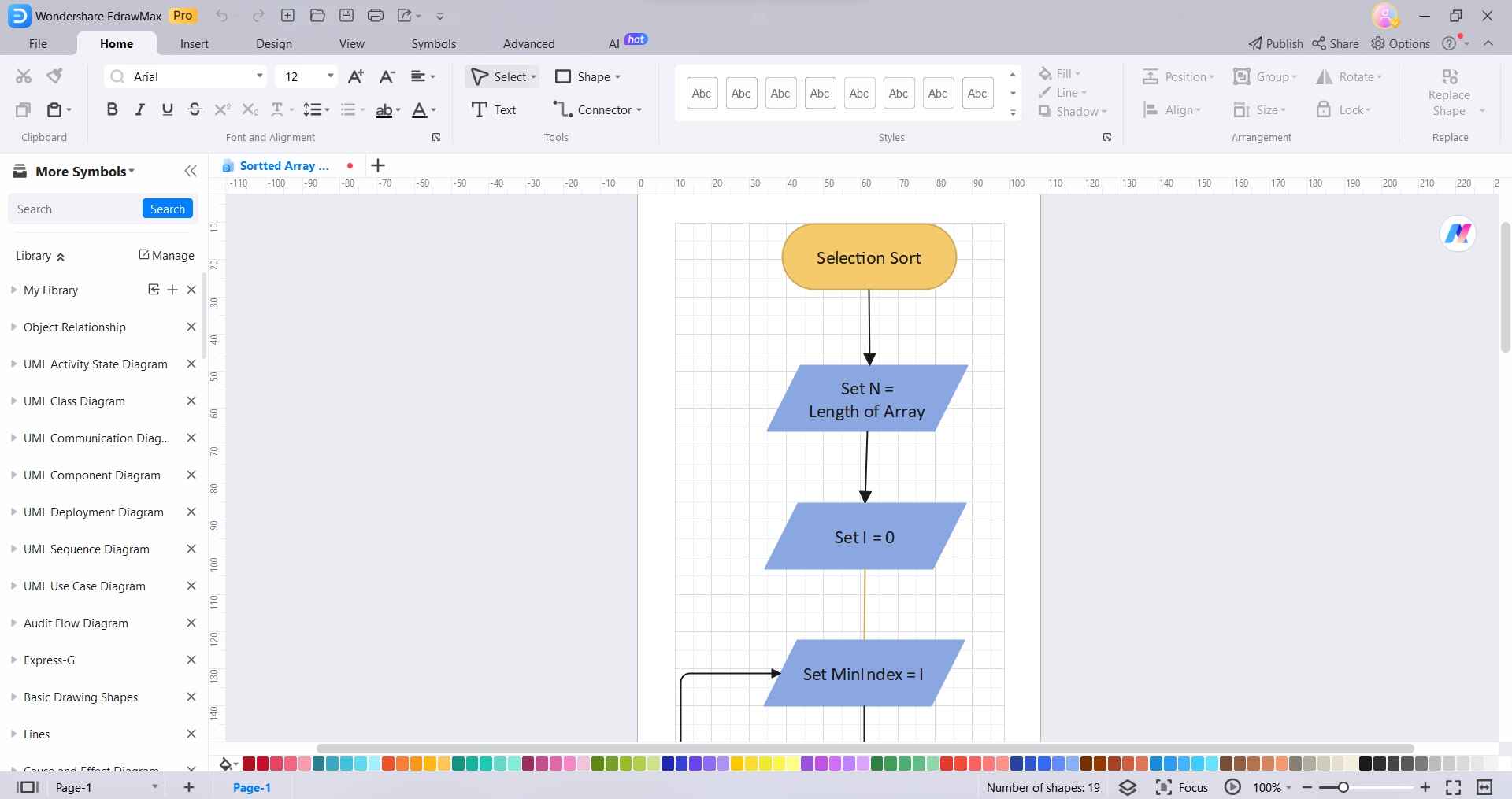
Step 3: Double-click on shapes to add text or labels representing functions, conditions, or actions.
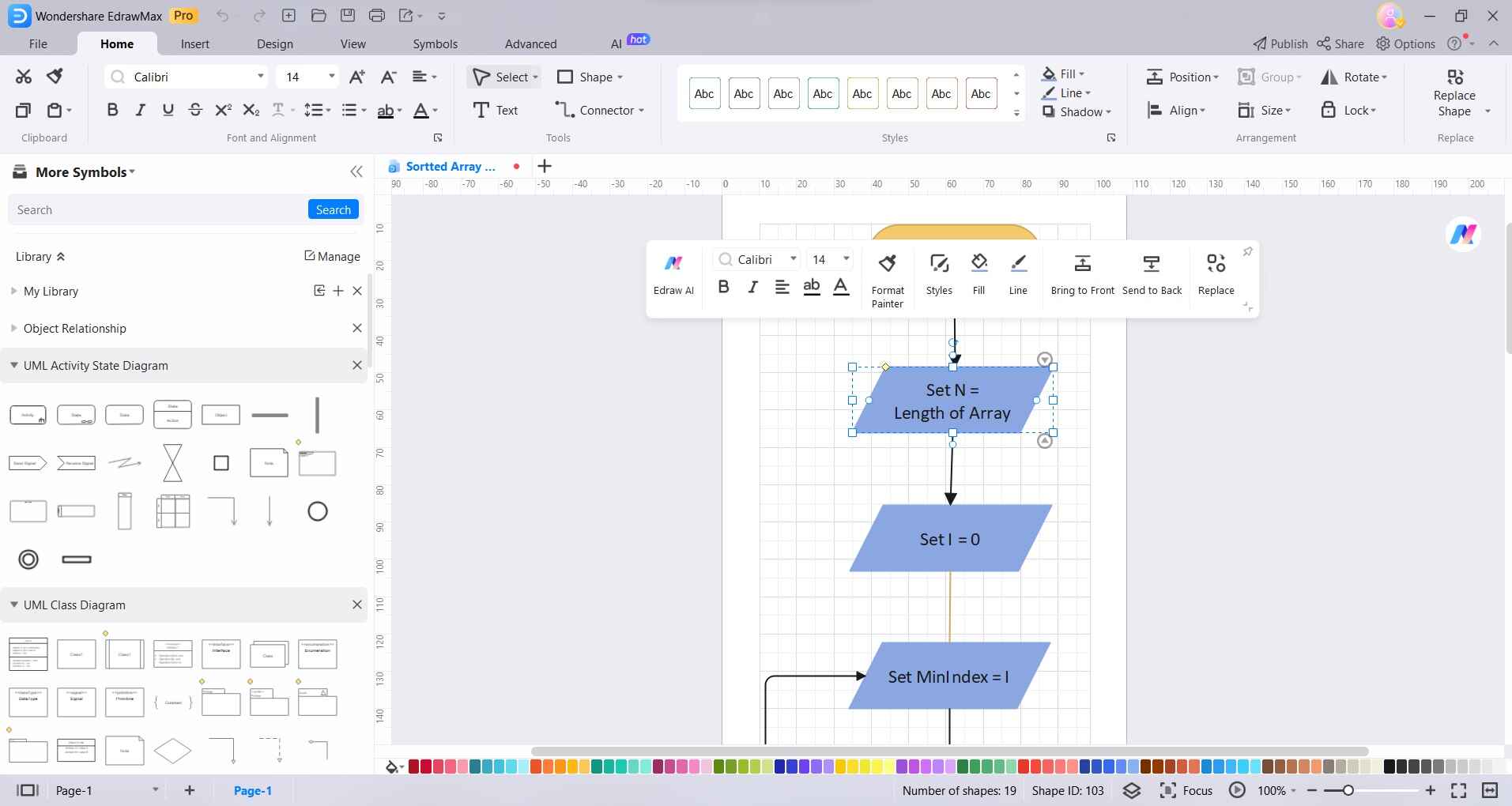
Step 4: Customize shapes, lines, and text by changing colors, line styles, fonts, and sizes. You can also align and distribute shapes for a neater appearance.
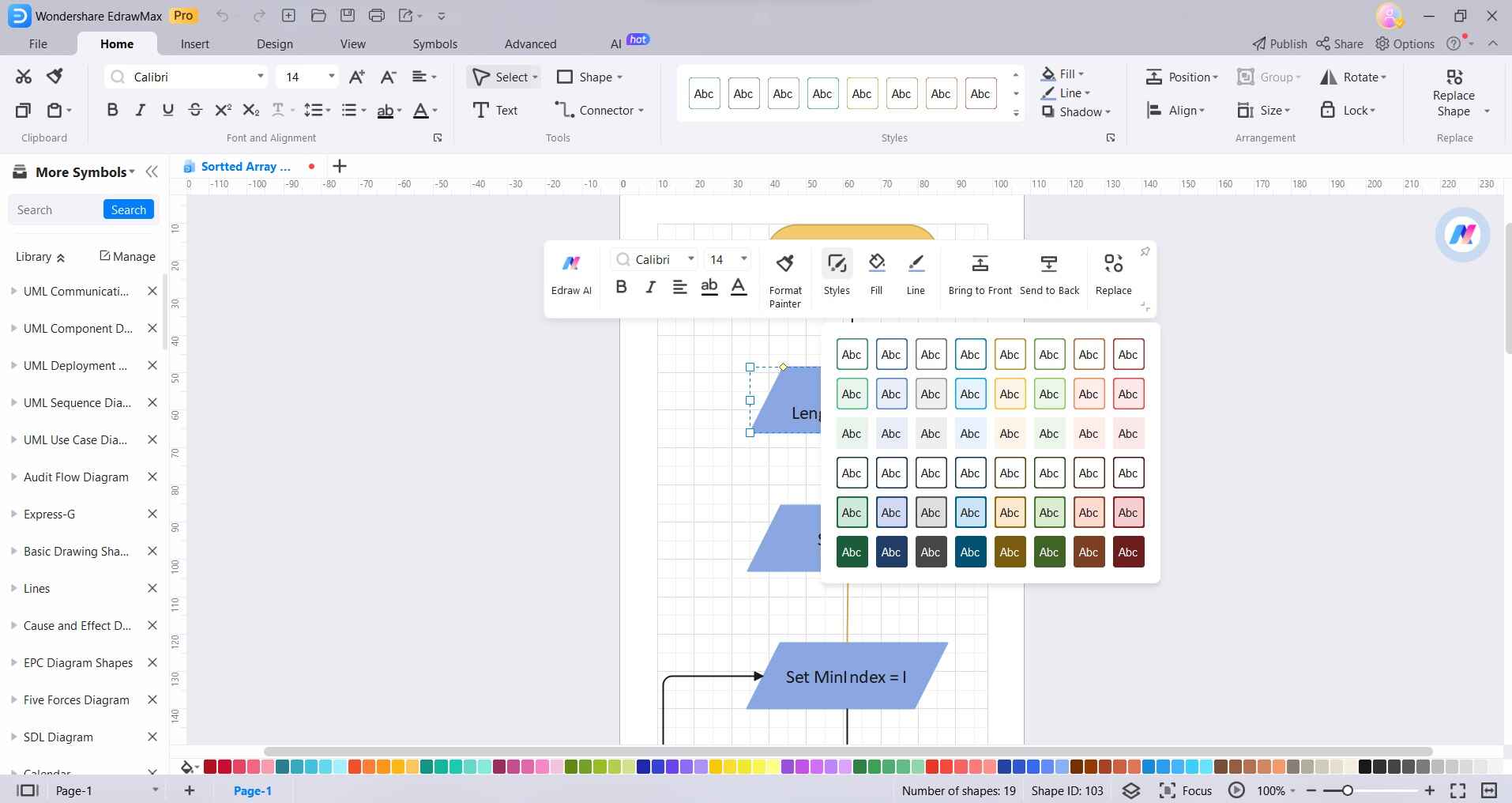
Step 5: Once completed, save your diagram by clicking on "File" and then "Save As." Choose your preferred file format and location.
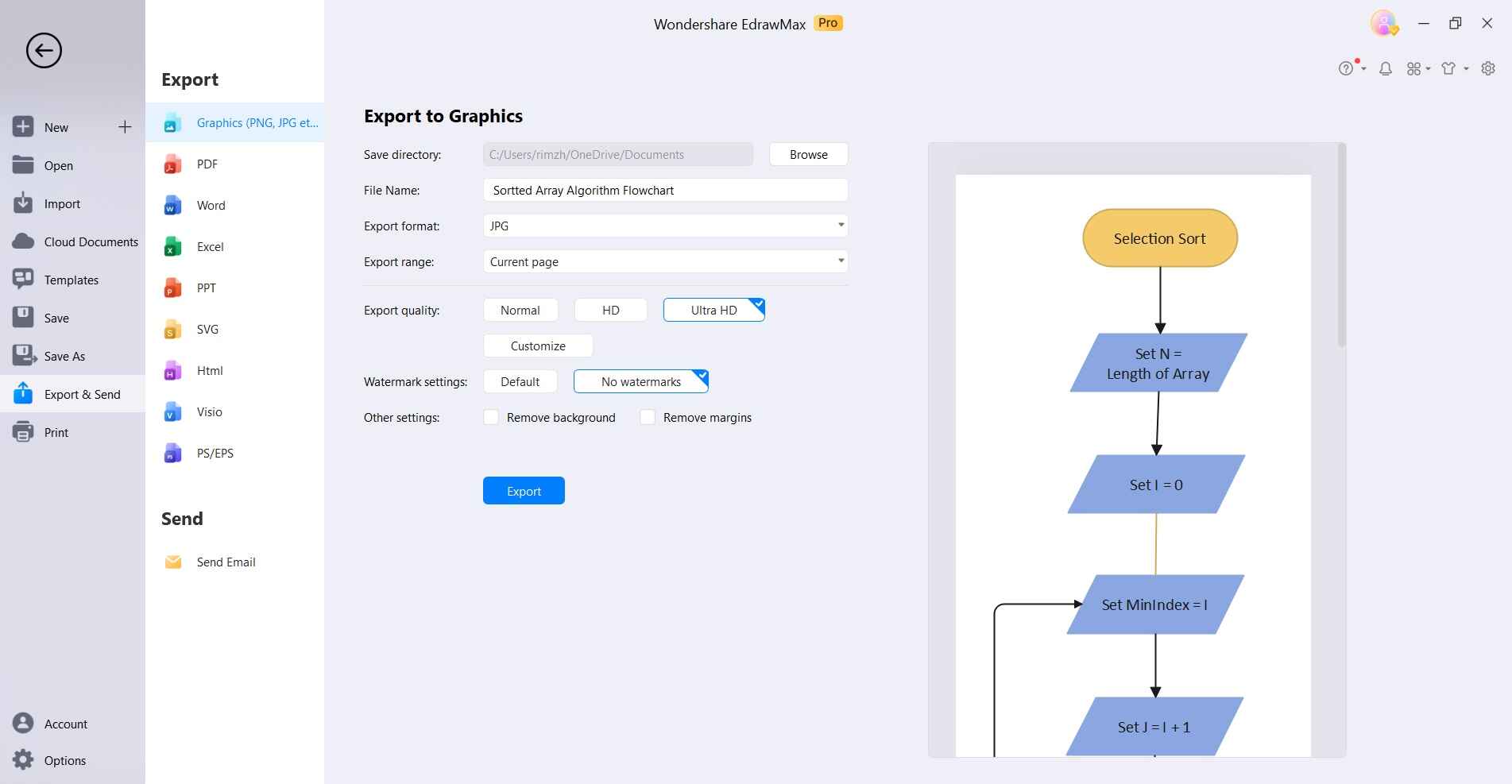
With the help of EdrawMax, you can get a good starting point for creating a simple programming diagram.
Conclusion
We have covered core concepts spanning what linked lists in data structure programs consist of, how they work internally, different types used in programming, contrast with other structures like queues and array sorts, design considerations when implementing linked lists, and using tools like EdrawMax to visualize them.
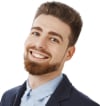