Data structures provide ways of organizing and storing data in computers so that they can be accessed and worked with efficiently. Stacks and queues are two commonly used linear data structures that enable data manipulation based on the last in, first out (LIFO) and first in, first out (FIFO) access mechanisms respectively.
This article provides an overview of stack programs in data structures, including types of data structure programs, and tips for using data structures effectively.
In this article
Part 1: What is a Stack Program in Data Structure?
Data structures provide ways of organizing and storing data in computers so that they can be accessed and worked with efficiently. Stacks and queues are two commonly used linear data structures that enable data manipulation based on the last in, first out (LIFO) and first in, first out (FIFO) access mechanisms respectively.
This article provides an overview of stack programs in data structures, including types of data structure programs, and tips for using data structures effectively.
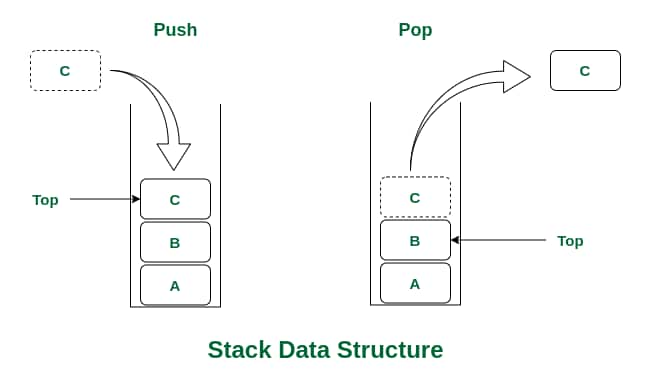
A stack, in abstract data types and computer science, refers to a linear data structure that follows the last in, first out (LIFO) principle for the addition and removal of elements. It means the last element inserted inside the stack is removed first.
Part 2: Common Types of Data Structure Programs
Some common data structure programs that are helpful for a programmer to know include:
#1 Binary Search Tree Program in Data Structure
A self-balancing binary search tree like AVL and red-black trees can be created to enable faster search, insert, and delete operations in O(log n) time. The value of each node in the binary search tree program must be greater than all the values in the left sub-tree and less than all the values in the right sub-tree.
#2 Bubble Sort Program in Data Structure
A bubble sort program can be written to sort elements of an array by making multiple passes through the array and comparing adjacent elements while swapping them if required. It is simpler to implement with two nested loops, but inefficient for sorting large data sets as its average and worst-case complexity is O(n^2).
#3 Queue Program in Data Structure
Queues function on a first in, first out (FIFO) principle, opposite to the stack's LIFO. Queue programs can use either arrays or linked lists to insert elements at the rear end, and remove elements from the front. It finds use cases like CPU task scheduling, printer spooling, etc.
#4 Quick Sort Program in Data Structure
Quick sort works by selecting a pivot element and partitioning the array into three regions - numbers less than pivot, numbers equal to pivot, and numbers greater than pivot - and recursively sorting them. Quicksort program is preferred for sorting as its average complexity is O(nlogn).
#5 Selection Sort Program in Data Structure
The selection sort program selects the smallest element of the unsorted list in each iteration and places it at the front. It requires scanning the complete array to find the minimum element in each pass. Simple, but inefficient due to O(n^2) complexity, selection sorts are rarely used for large data sets.
#6 Insertion Sort Program in Data Structure
Insertion sort programs start with an empty sorted list and insert each element from the main list into the appropriate position of the sorted list. Easy to code with O(n^2) complexity, insertion sorts work well for sorting fewer elements and allow adaptive behavior based on input data.
Part 3: Tips to Keep in Mind When Using Data Structures
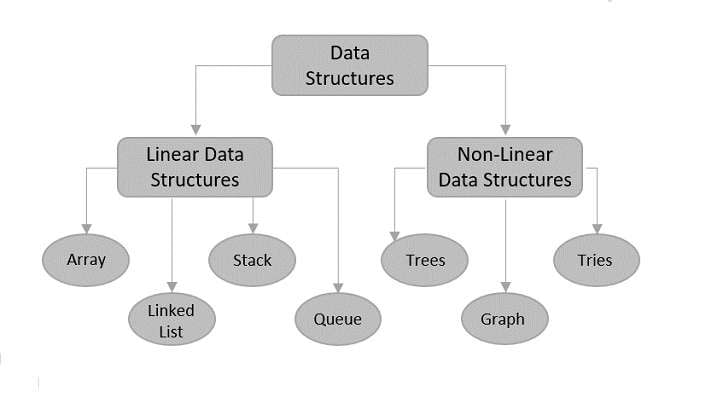
When implementing data structure programs, developers should keep these tips in mind:
- Analyze program requirements to identify suitable data structures based on access speed, storage efficiency, etc. Stack suits LIFO access, while queue matches FIFO access need.
- Use built-in data structures from standard libraries instead of creating your own implementations to reduce errors. For example, Stack class from Java Collections Framework.
- Add overflow checking and error handling code to data structure programs. Track empty and full conditions.
- Comment code appropriately to document data structure method behaviors and complex parts of the program.
- Test data structure program thoroughly with different use cases—normal, boundary, and erroneous input data.
Part 4: Creating a Programming Flowchart Using EdrawMax
A flowchart visually represents the logical flow and operations of a program. Using EdrawMax to create programming flowcharts brings important benefits that improve the software development process. Specifically, EdrawMax provides a visual and structured way to map out program logic that promotes better understanding compared to just textual representations.
Here are the steps to create a simple programming flowchart using EdrawMax:
Step 1:
Launch the EdrawMax software on your computer. In EdrawMax, select the "Flowchart" category from the template gallery. Choose a blank flowchart template or select a pre-designed template that suits your needs.
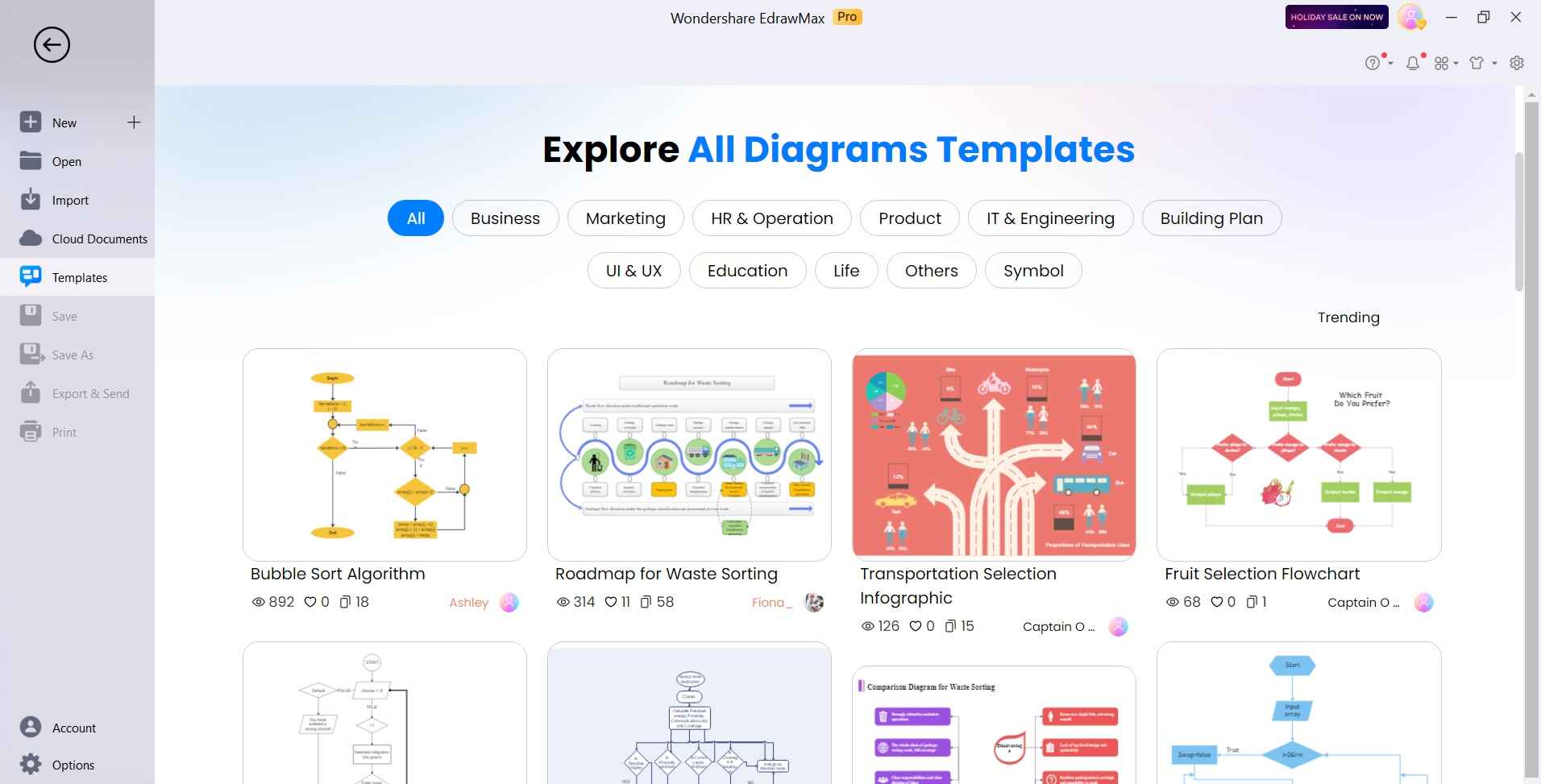
Step 2:
Use the symbol library in EdrawMax to add shapes and symbols to represent different elements of your program.
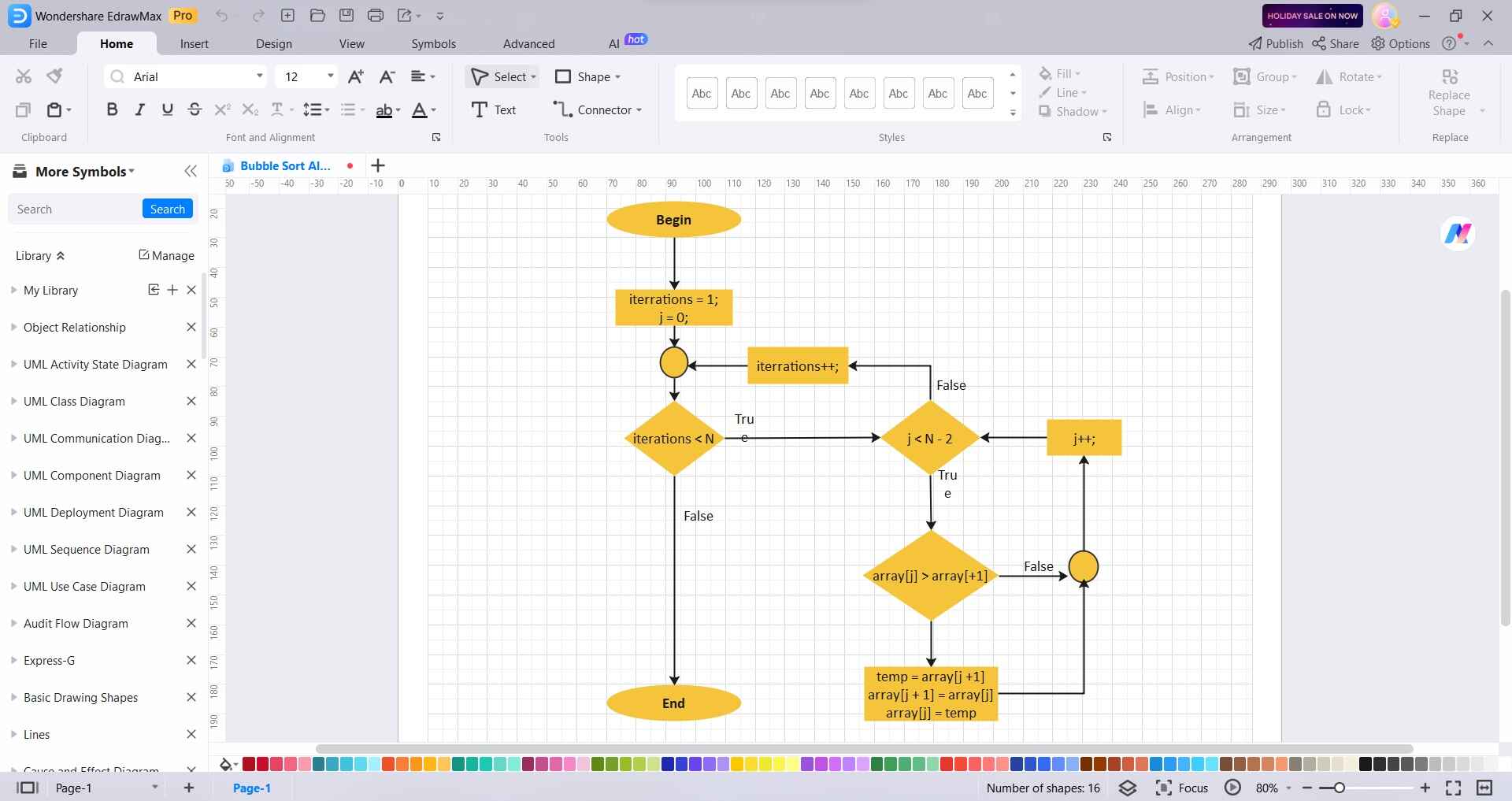
Step 3:
Add text to each symbol to describe the actions or processes it represents.
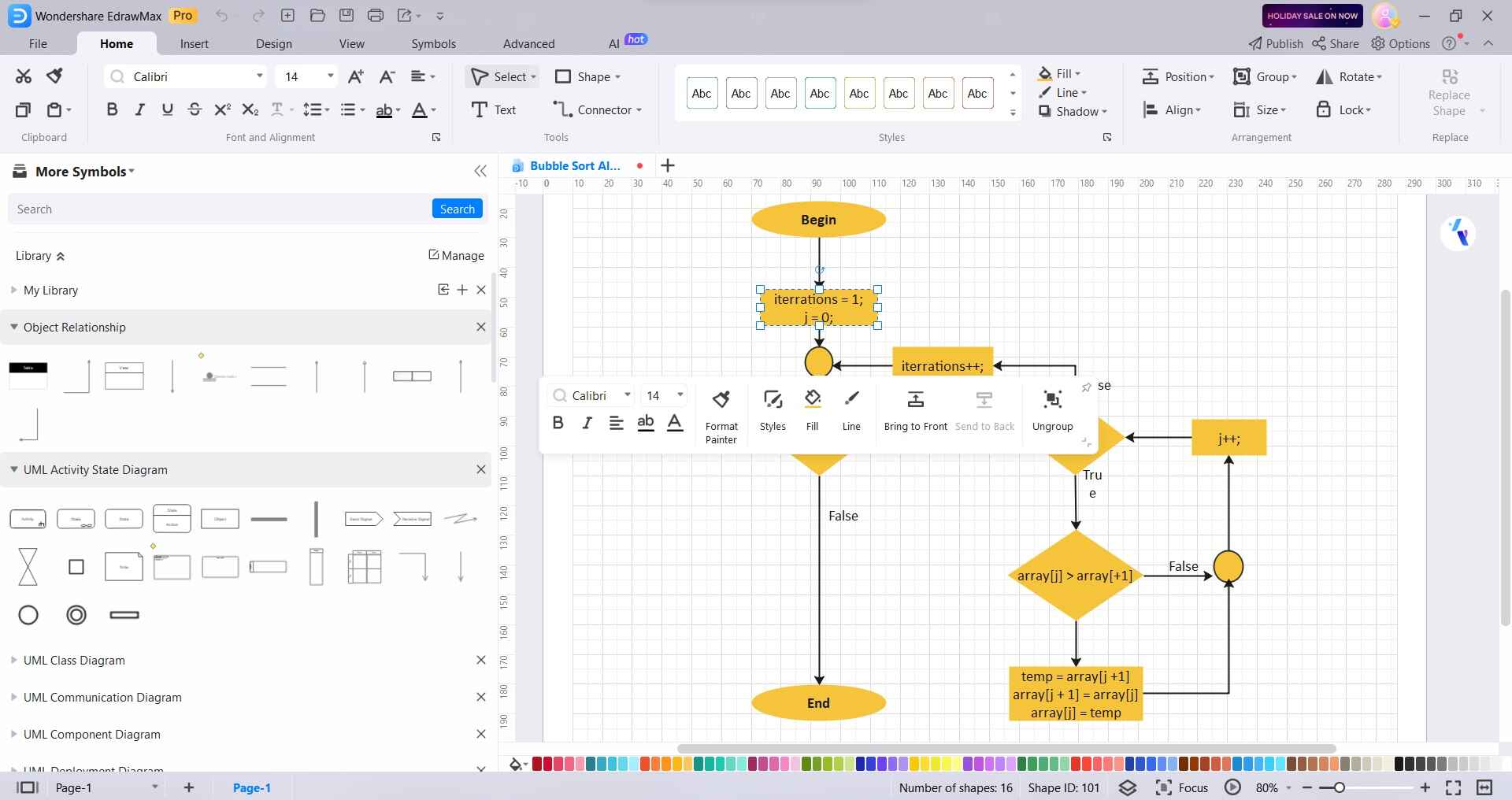
Step 4:
Customize the flowchart by changing colors, fonts, and sizes to make it more visually appealing and easier to understand.
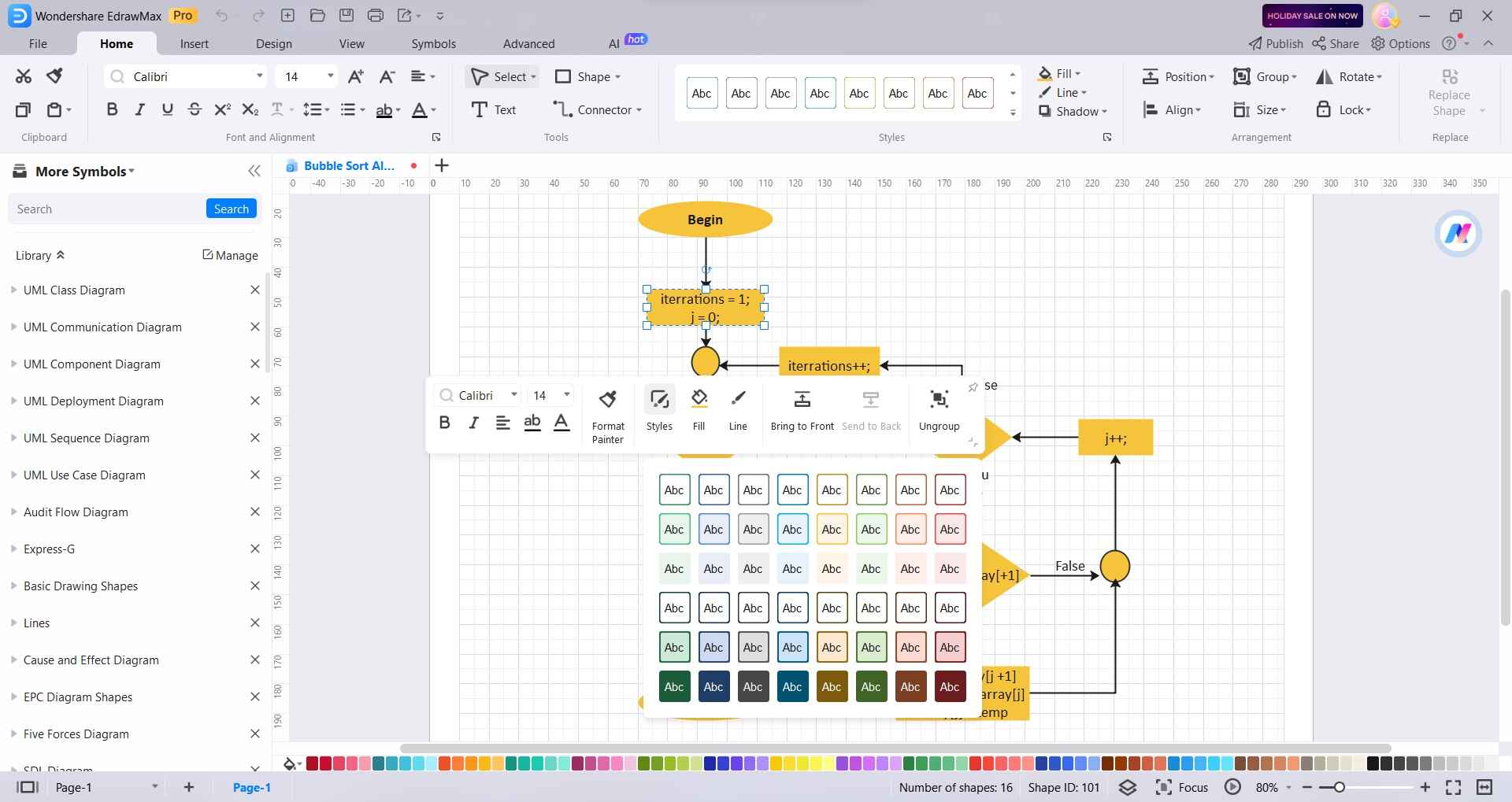
Step 5:
Once you're satisfied with the flowchart, save your work in EdrawMax format.
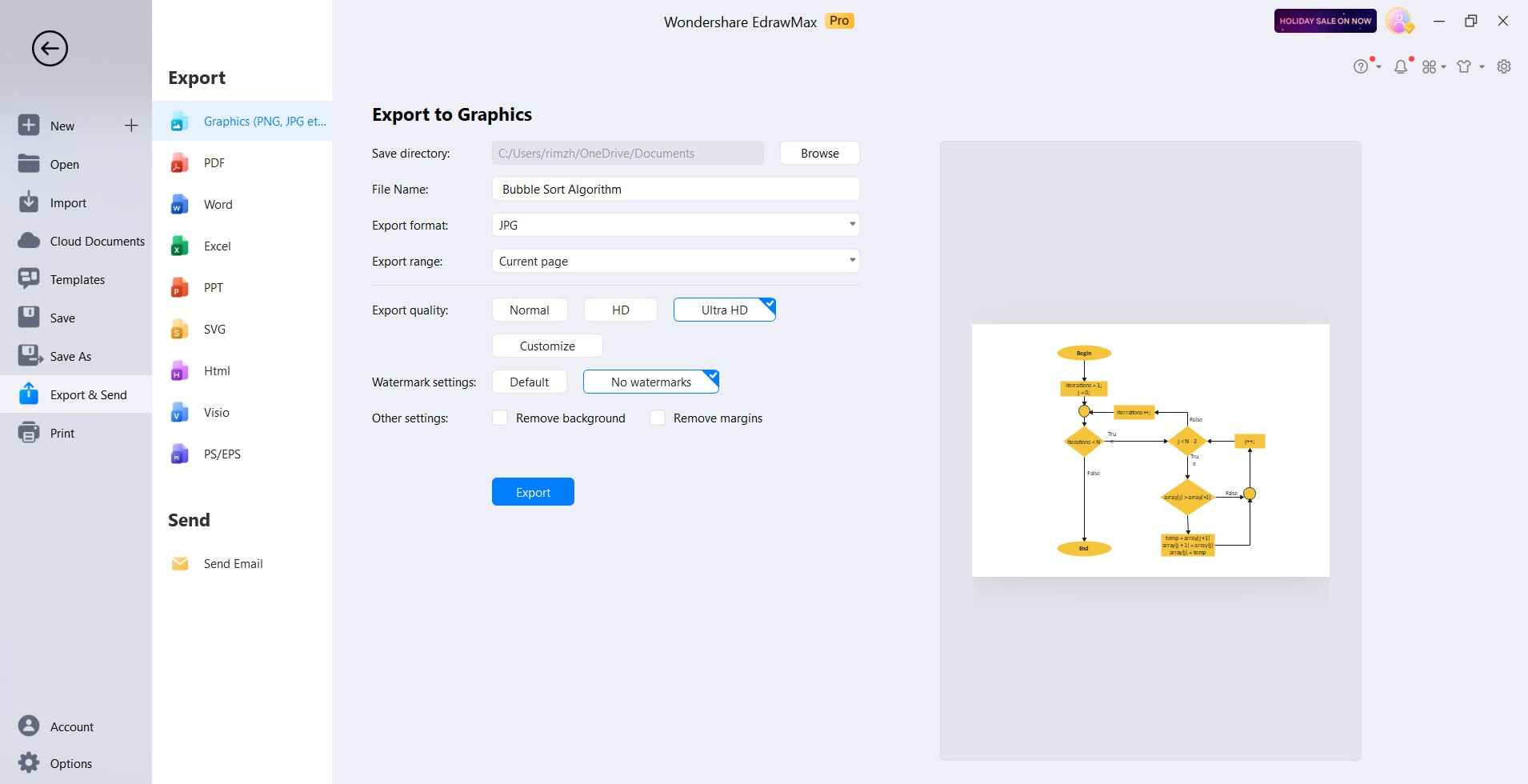
EdrawMax enables quick creation using ready symbols and offers built-in smart drawing tools to align elements and other useful features.
Conclusion
The stack data structure provides a LIFO access mechanism well-suited for reversing data, parsing expressions, etc. Stack programs require tracking overflow and empty conditions. Knowledge of basic data structures, using standard libraries, adding validation checks, testing thoroughly, and creating program flowcharts are key ways to efficiently implement robust programs around linear data structures like stacks, queues, and more.