Linked lists are among the fundamental data structures used in computer programming. They provide an efficient way to store dynamic collections of data and allow for constant-time insertions and deletions.
In this comprehensive guide, we will cover everything you need to know about implementing linked lists in Java, one of the most utilized object-oriented languages.
In this article
Part 1: What is a Linked List?
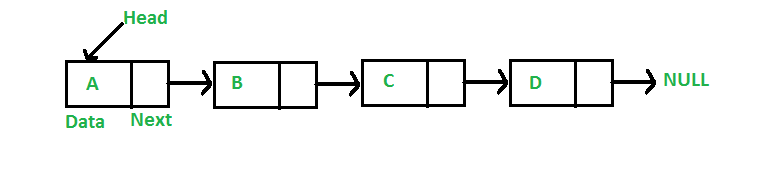
A linked list is a linear data structure consisting of a sequence of data elements called nodes. Each node contains data and a pointer that refers to the next node in the sequence.
The principal benefit of a linked list is efficient memory usage and flexibility - linked list nodes use only the memory required for their data. Plus, linked lists can expand without having to preallocate large blocks of memory.
Nodes in a linked list do not have to occupy consecutive memory locations like arrays do. The pointers in each node identify where the next node resides in memory.
Part 2: Steps for Implementing Linked List in Java
Follow these key steps to implement a basic linked list in Java:
- Create a Node class with attributes for data storage and a next node reference.
- Create a Linked List class with a reference to the head node. Include methods to add, remove, and find nodes.
- Instantiate an empty linked list by creating a head node and setting its reference to null.
- Write functions to insert nodes at head, tail, or arbitrary positions by updating node references.
- Write functions to delete nodes by updating the previous node's next pointer.
- Print contents of the linked list by iterating through nodes via the next pointers.
Part 3: Types of Linked Lists
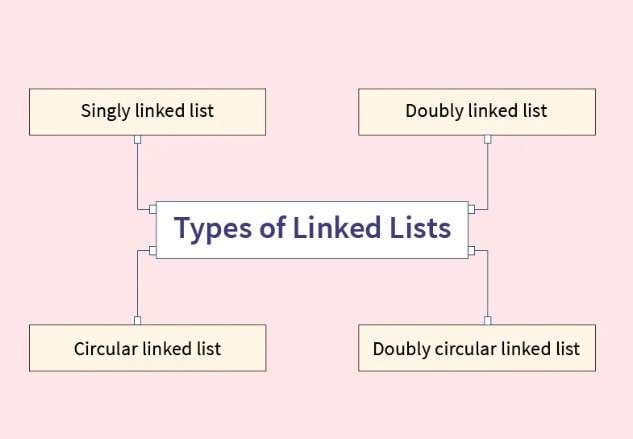
There are several specific kinds of linked list implementations:
- Singly Linked Lists: Most basic. Each node points to the next node. Finding previous nodes requires iterating from the head.
- Doubly Linked Lists: Nodes have added backward nextNode pointers allowing bidirectional traversal. Extra memory but faster lookups.
- Circular Linked Lists: The next pointer on the last node refers back to the first node forming a non-ending loop. Useful in applications that repeat, like playlists.
Part 4: Overview of Golang Linked List
Golang has built-in linked list support through a container/list package. Some key characteristics:
- Implements a doubly-linked list. Nodes can be traversed forward and backward.
- Thread-safe for concurrent mutation from multiple goroutines.
- Methods like PushFront, InsertAfter, and Remove are provided for mutating the list.
- Can create elem nodes that store any type of data value.
- Forwards and backward iterations are supported via Next() and Prev().
Part 5: Example of Stack Program in C Using Linked List
Here is an outline for a stack data structure implementation in C using a linked list:
- Define Node structure with int data and next node pointer.
- Implement a TOP pointer to track the top of the stack.
- push(): create a new node, set data value, set next to the current top, reset top pointer.
- pop(): save the top node, set the top to the next node, and return the saved node data.
- isEmpty(): check if the top pointer is null.
- printStack(): iterate from the top and print each node's data.
With these functions, you can use a linked list as a LIFO abstract data type to provide standard stack operations.
Part 6: Creating a Programming Algorithm Flowchart Using EdrawMax
EdrawMax is professional diagramming software for all kinds of diagrams from flowcharts to entity models and programming algorithms.
Some key reasons to use EdrawMax for linked list algorithm flowcharts:
- Provides standard flowchart symbols and templates for faster editing.
- Drag-and-drop symbols and auto-connecting lines.
- Automatic stylistic formatting tools.
- Easy to reorganize steps by moving symbols around.
- Shareable as image files or editable diagrams.
Using EdrawMax greatly speeds up the process of creating accurate flowcharts to document complex programming algorithms. After structuring the algorithm steps in EdrawMax, you can easily export them for documentation or discussion.
Here are the steps to create a simple programming binary search flowchart using EdrawMax:
Step 1:
Open EdrawMax and select the Flowchart category from the diagrams menu. Choose the Blank Template.
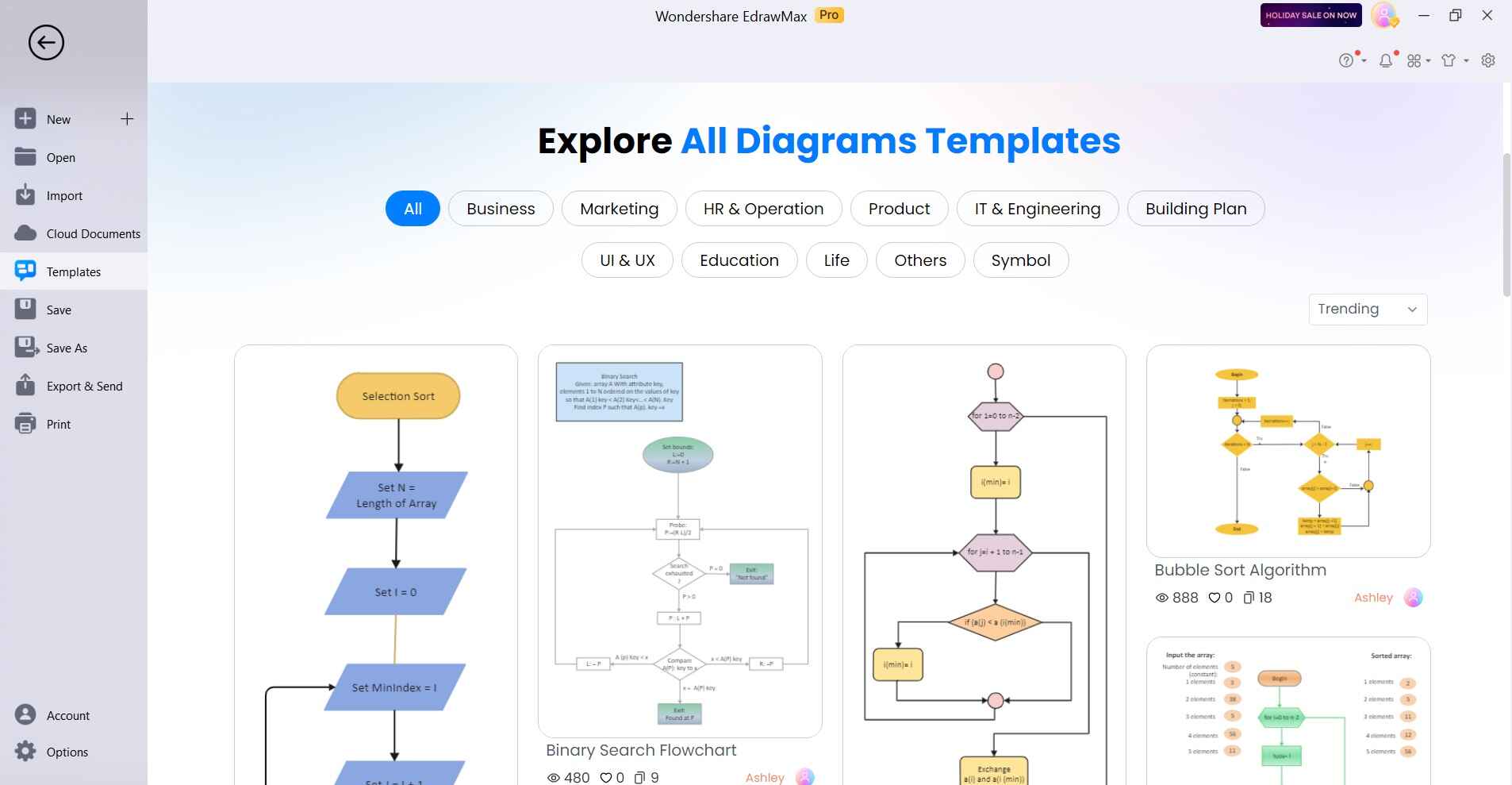
Step 2:
Drag and drop programming flowchart symbols like Terminator, Process, Input/Output, and Decision from the left Libraries panel onto the canvas.
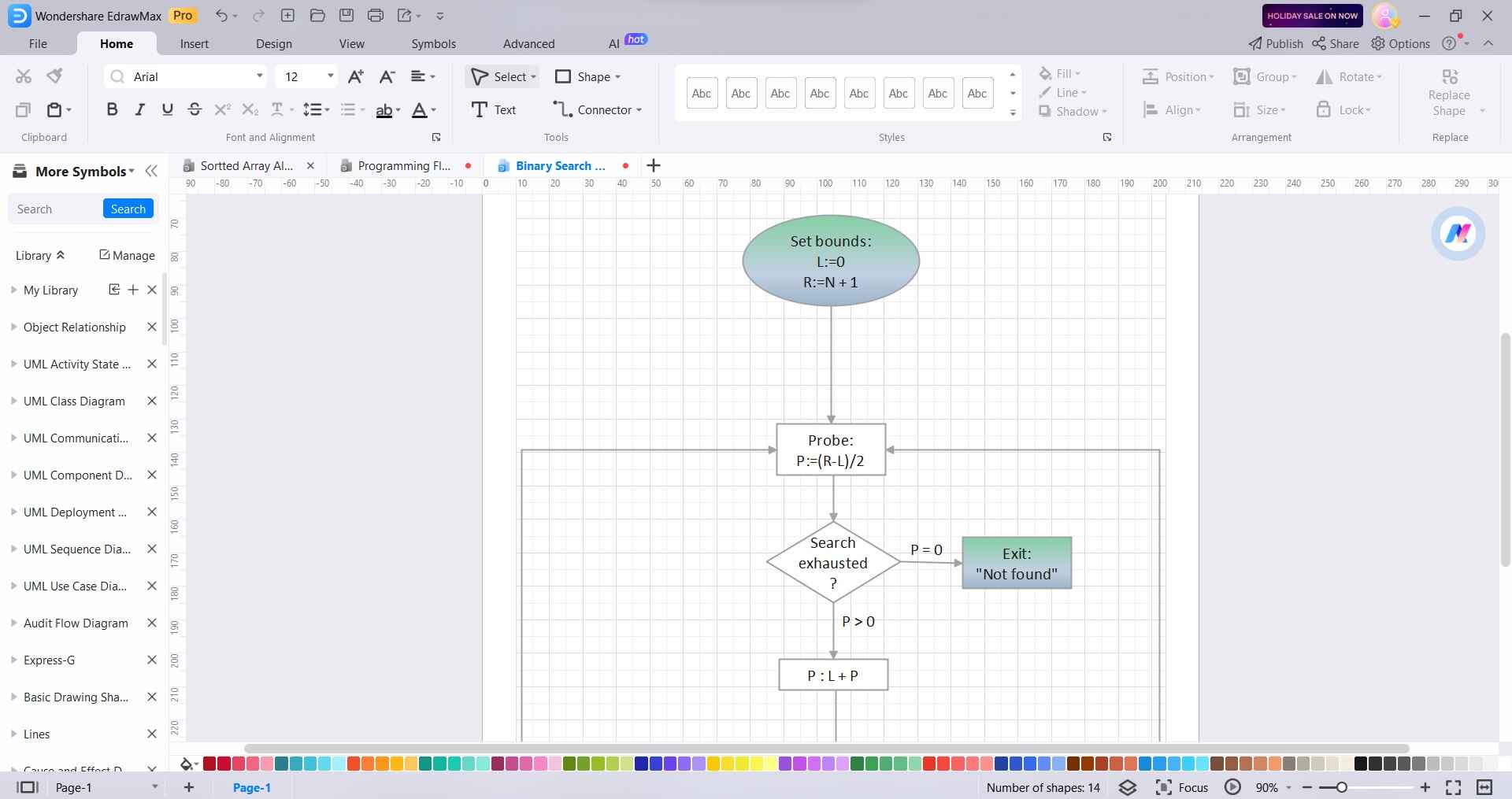
Step 3:
Double-click on each symbol to enter a description of that programming step.
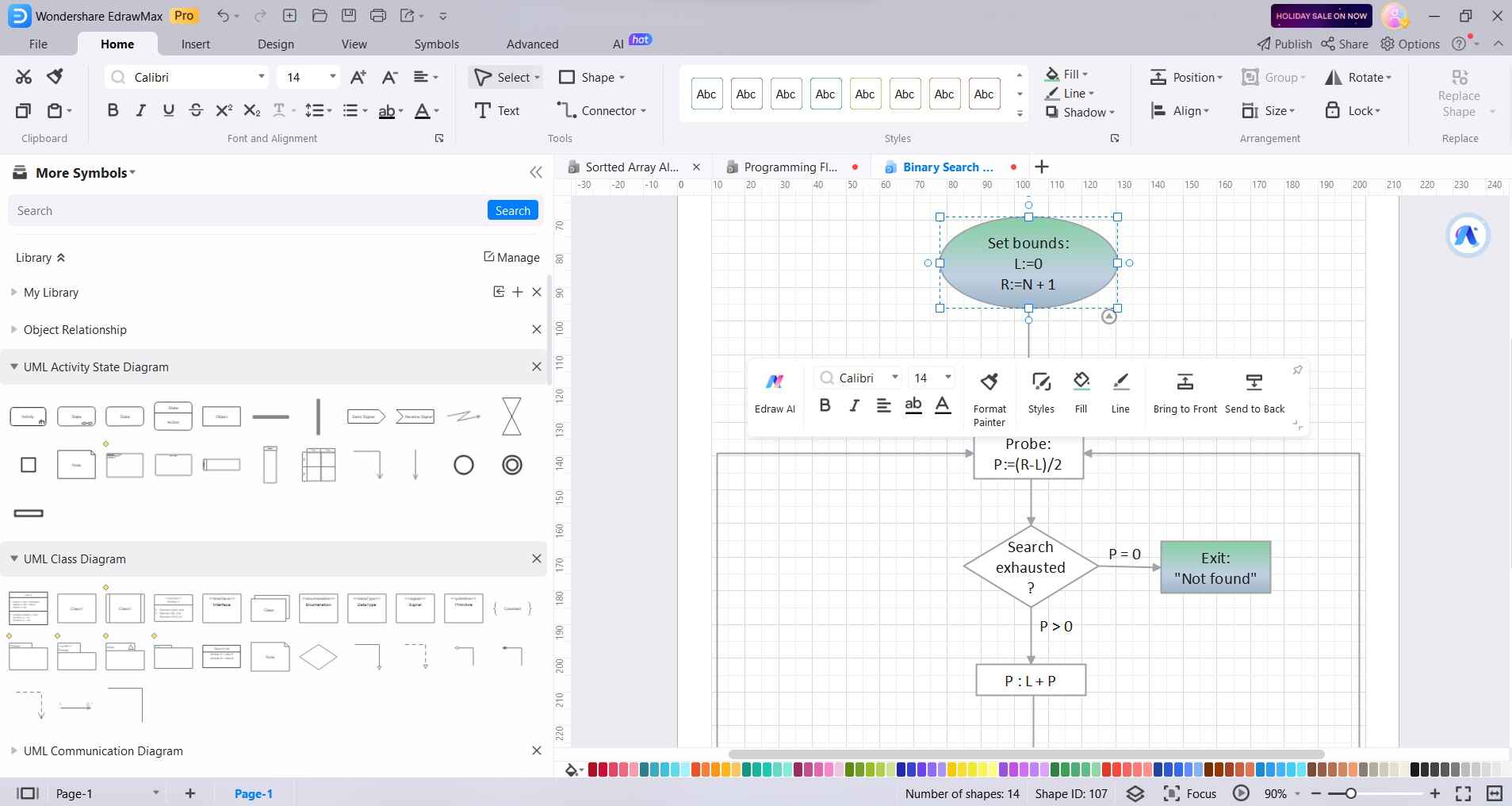
Step 4:
Organize the flowchart elements in a logical sequence. Adjust the layout, size, and formatting of symbols and connectors for clarity and readability.
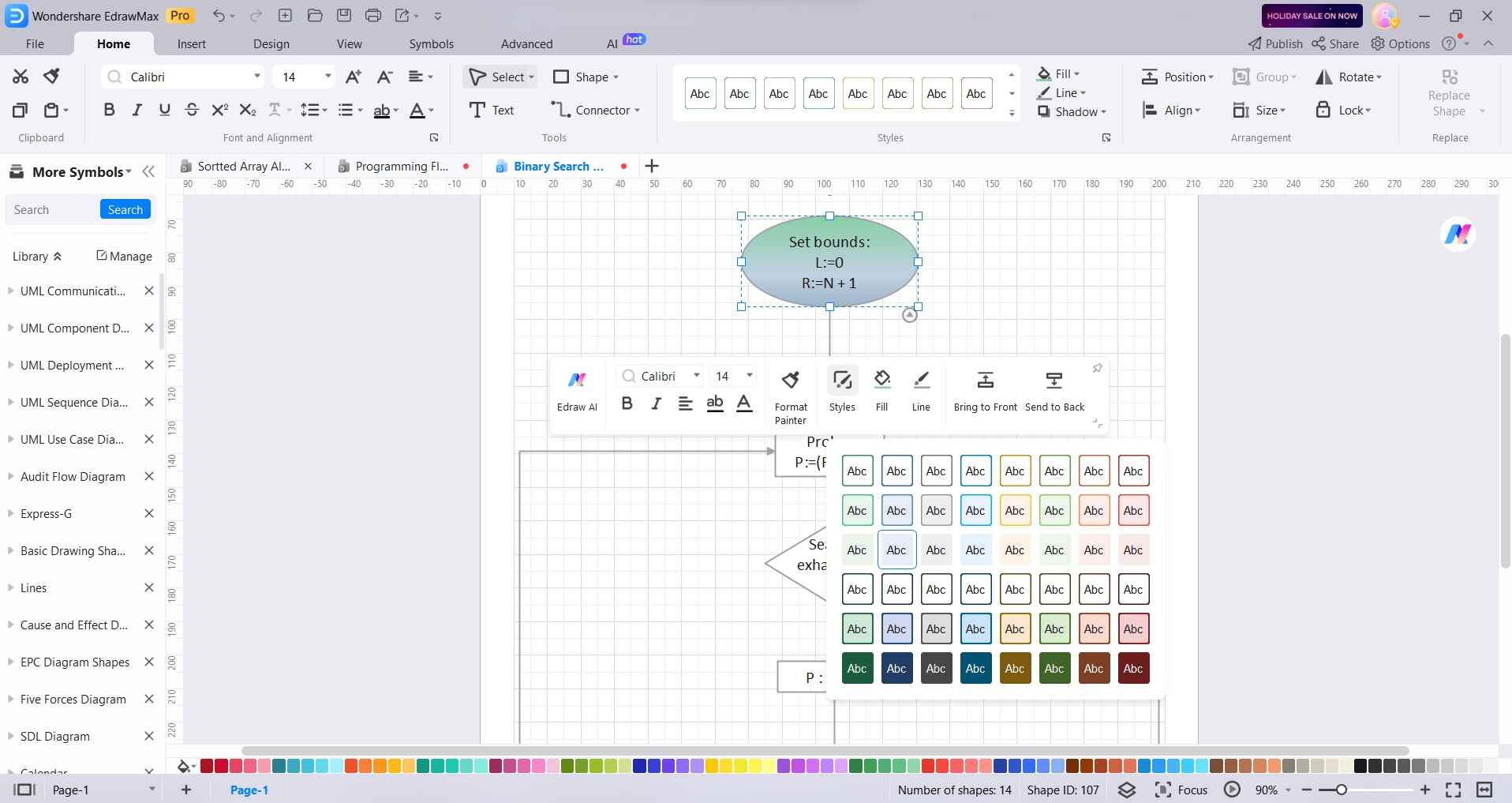
Step 5:
Save your flowchart by clicking on "File" and then "Save As." Choose the desired format (EdrawMax's native format or others like PNG, JPG, or PDF) for exporting the flowchart.
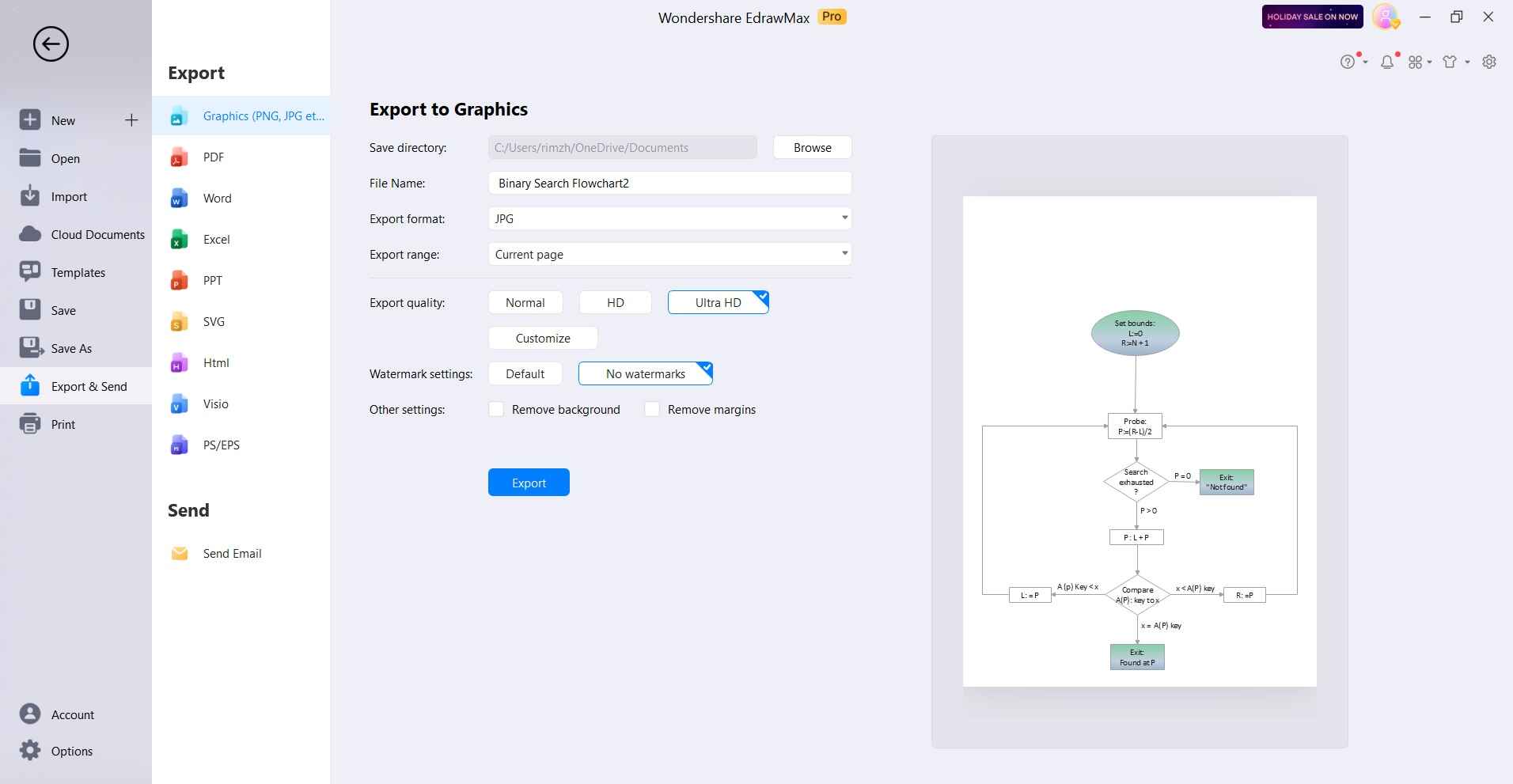
Following these steps will help you create a simple programming algorithm flowchart using EdrawMax, enabling you to visualize and communicate your algorithm effectively.
Conclusion
Linked lists provide memory and performance advantages over arrays and are ubiquitous in programming. As seen in this guide, implementing a basic linked list in Java just requires defining Nodes, implementing a Linked List class with pointer operations, and writing functions for mutating the list by adding/removing nodes.
With this foundation on the key concepts and implementation of linked lists plus a method for illustrating algorithms via EdrawMax, Java developers will be well prepared to effectively leverage linked lists.