A binary search tree is a very useful data structure in computer science that organizes data in a way that allows for quick search, insertion, and deletion operations. The key characteristic of a binary search tree is that the data elements stored in the left subtree of any node are less than that node's data, and the data elements stored in the right subtree are greater than that node's data.
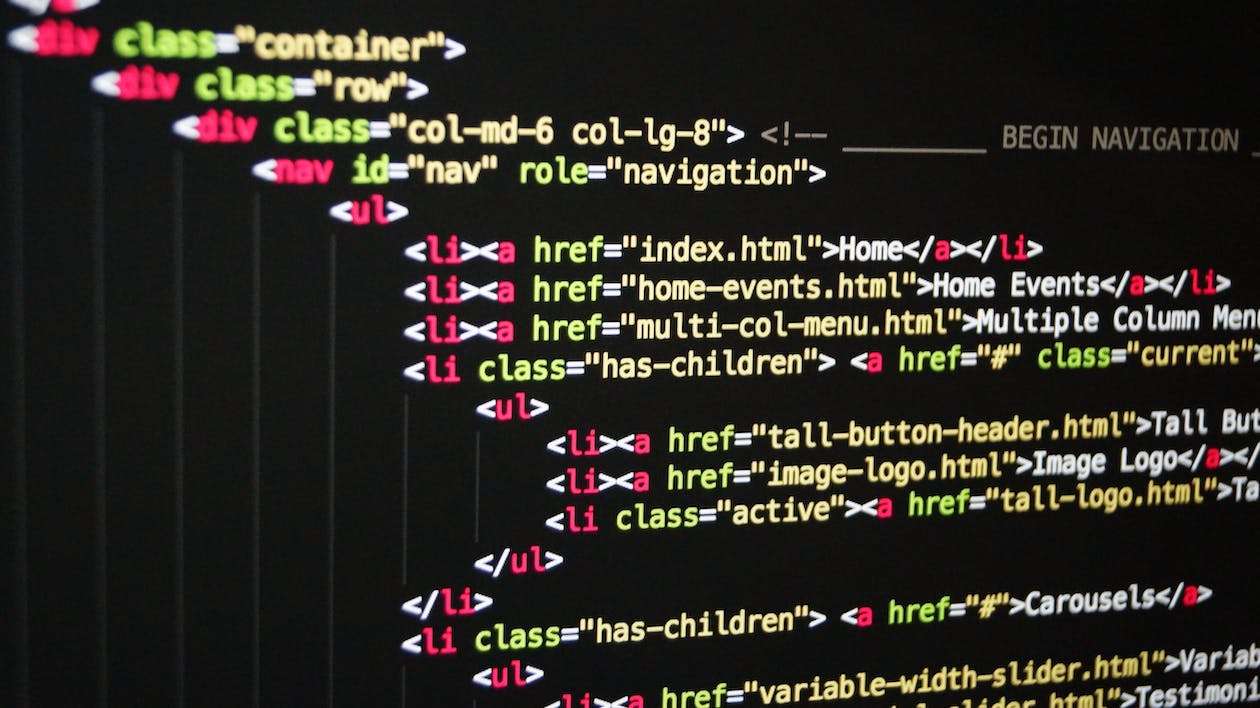
In this article
- What is a Binary Search Tree Program in C++
- Key Components of a Binary Search Tree Program
- Benefits of Using a Binary Search Tree Program in C++
- Role of Binary Search Tree Program in Data Structure
- Binary Search Tree Program in Python
- Create a Binary Search Flowchart Using EdrawMax Easily
- Conclusion
Part 1: What is a Binary Search Tree Program in C++
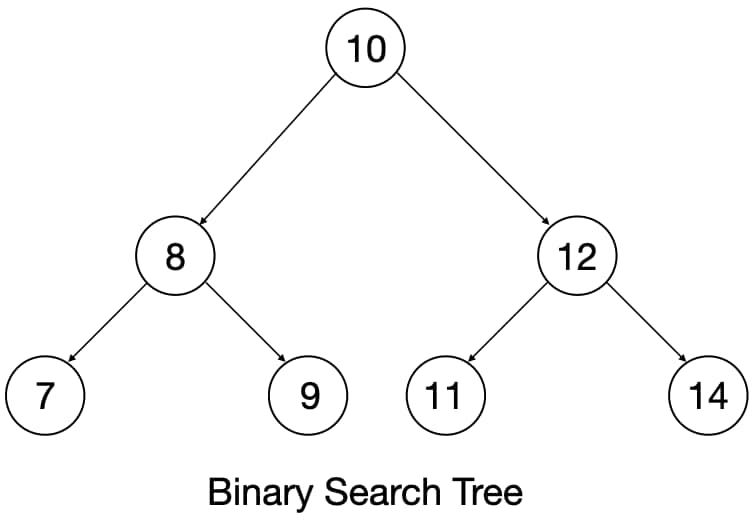
A binary search tree program in C++ allows the creation and manipulation of a binary search tree data structure. It enables organizing data in a hierarchical manner where each node has at most two children, represented as left and right subtree pointers.
The C++ binary search tree has nodes that store data elements like integers, floats, or custom objects. It maintains the property that elements in the left subtree are less than or equal to the current node, which is less than or equal to elements in the right subtree. Key operations like search, insert, delete, and traverse can be implemented through recursive or iterative logic written in C++.
Part 2: Key Components of a Binary Search Tree Program
The key components that make up a binary search tree program in C++ include:
- Node structure - Houses data elements and left/right pointers to children.
- Root node - The top node of the tree from which everything descends.
- Insert operation - Adds new elements in the appropriate subtree.
- Delete operation - Removes existing elements properly.
- Search operation - Finds specific elements in the tree.
- Traversal logic - Visits all nodes systematically.
- Balance handling - Keeps tree shallow for quick ops.
Each node contains the data and links to children. The insert and delete ops maintain the ordering by respective comparisons. The search navigates accordingly. Inorder traversal visits the nodes in order. Balance maintenance is done through rotations.
Part 3: Benefits of Using a Binary Search Tree Program in C++
Implementing a binary search tree data structure through a C++ program has several notable benefits, including:
- Rapid search, insert, and delete operations: Due to the ordering of data and hierarchical nature, common ops are very fast, enabling efficient access.
- Flexibility of data: C++ BSTs can store any data type, like primitives or custom objects, through templates.
- Reusability: BST code can be reused across projects as a standalone class or part of a larger program.
- Educational value: Implementing a BST helps better understand data structure concepts that translate to real-world software engineering problems.
With the combination of features like speed, flexibility, reusability, and learning, a C++ BST provides high pragmatic value.
Part 4: Role of Binary Search Tree Program in Data Structure
As a fundamental data structure in computer science, binary search trees serve an important role through abilities like:
- Organizing data for quick retrieval.
- Supporting rapid operations through ordering.
- Complementing algorithms that use ordering and hierarchy.
- Boosting program efficiency for a variety of problems.
- Serving as a building block for more complex data structures.
Overall, mastering the foundations with a C++ BST program enables leveraging data structures for problem-solving.
Part 5: Binary Search Tree Program in Python
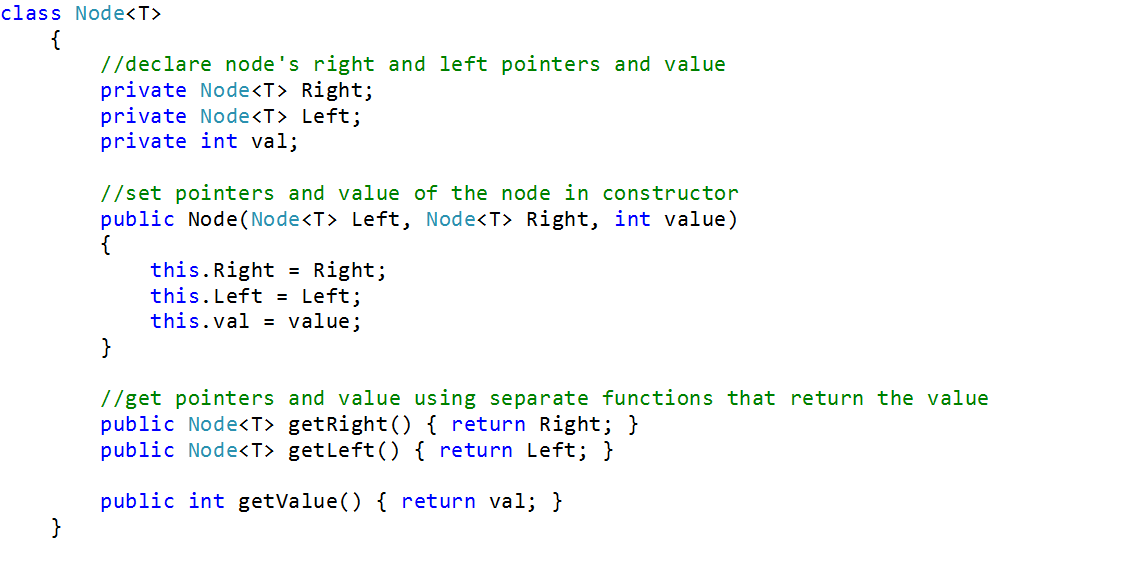
A BST can also be created in Python for similar purposes. As a high-level language, Python focuses more on code readability while C++ delivers optimal performance. A Binary search tree program in Python would have similar insert, search, and delete logic, but utilize built-in references instead of pointers. It would use fewer lines of more expressive code at the cost of speed.
Part 6: Create a Binary Search Flowchart Using EdrawMax Easily
Before coding a Binary Search Tree program in C++, it helps to map out the logic visually through a flowchart diagram. This allows designing the essential operations in a structured manner before implementation.
One such helpful tool for this purpose is EdrawMax. As a cross-platform visualization software, EdrawMax streamlines flowchart design with templates, symbols, and intuitive editing. It offers deep integration with popular programming languages and paradigms. With EdrawMax's rich features, the entire flow from planning to development is accelerated for an efficient coding experience.
Here are the steps to create a binary search flowchart using EdrawMax:
Step 1:
Launch the EdrawMax application on your computer. Choose a flowchart template or start with a blank canvas.
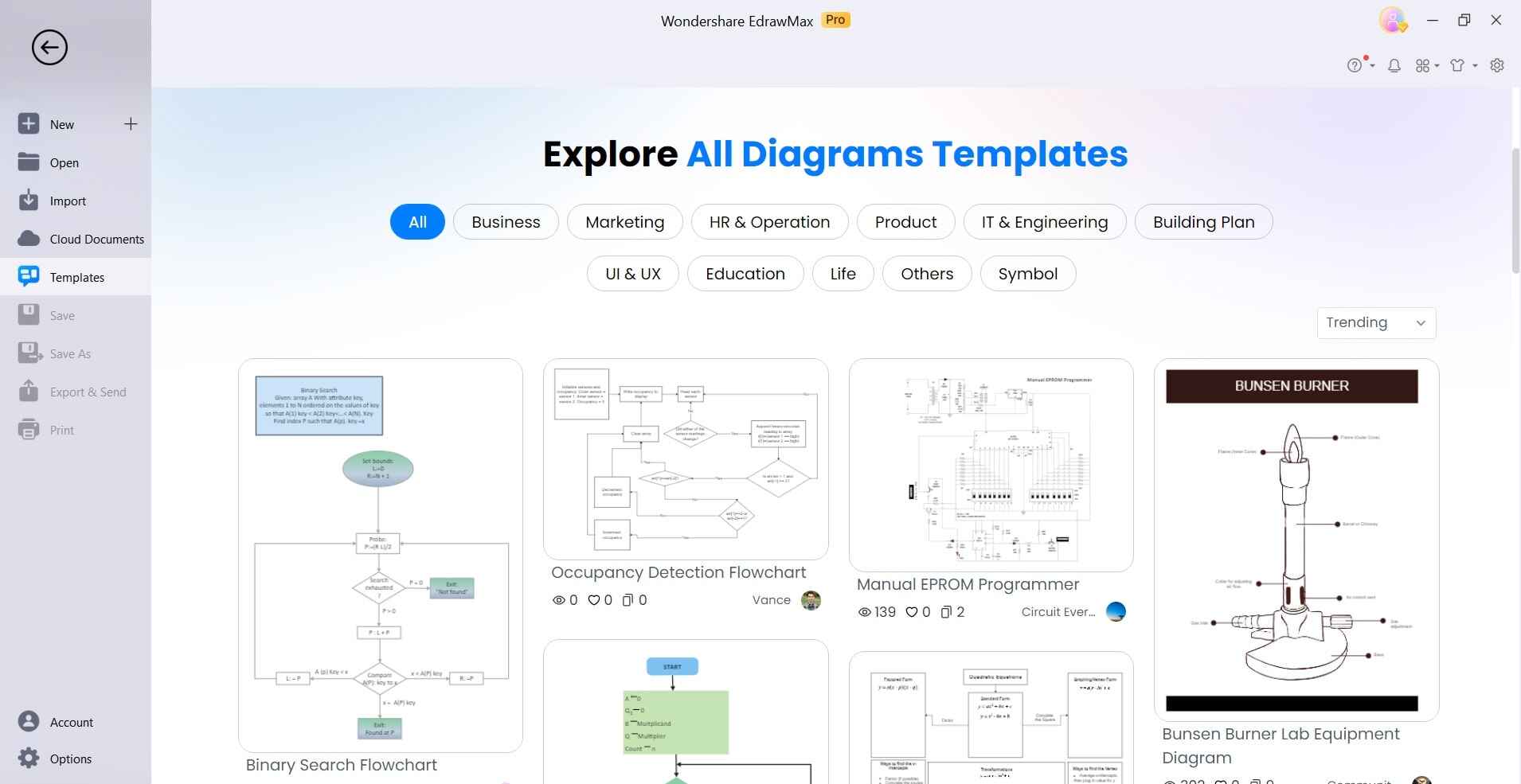
Step 2:
Drag and drop the start symbol onto the canvas, representing the start of the flowchart.
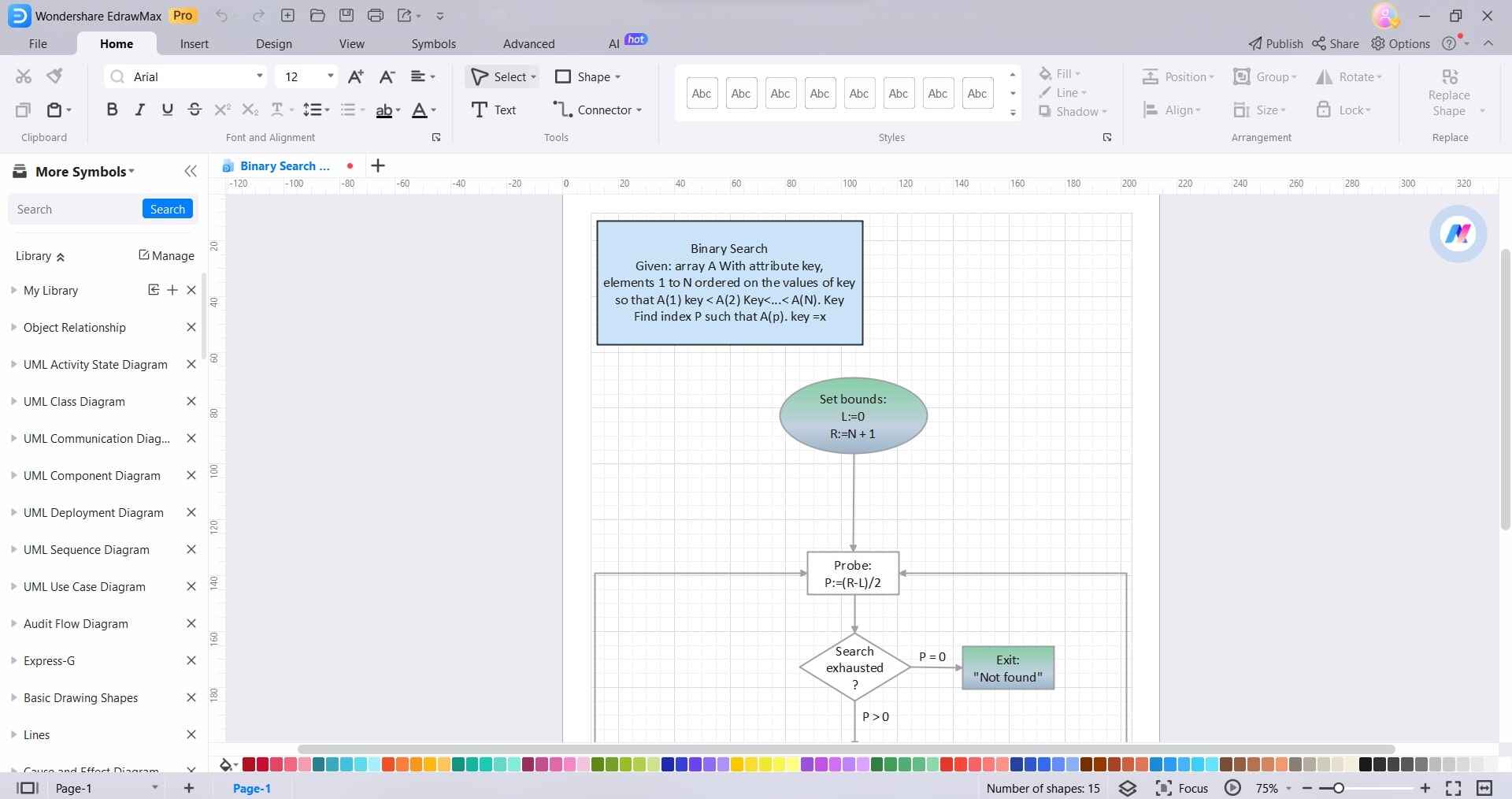
Step 3:
Use decision symbols to indicate binary decisions in the algorithm.
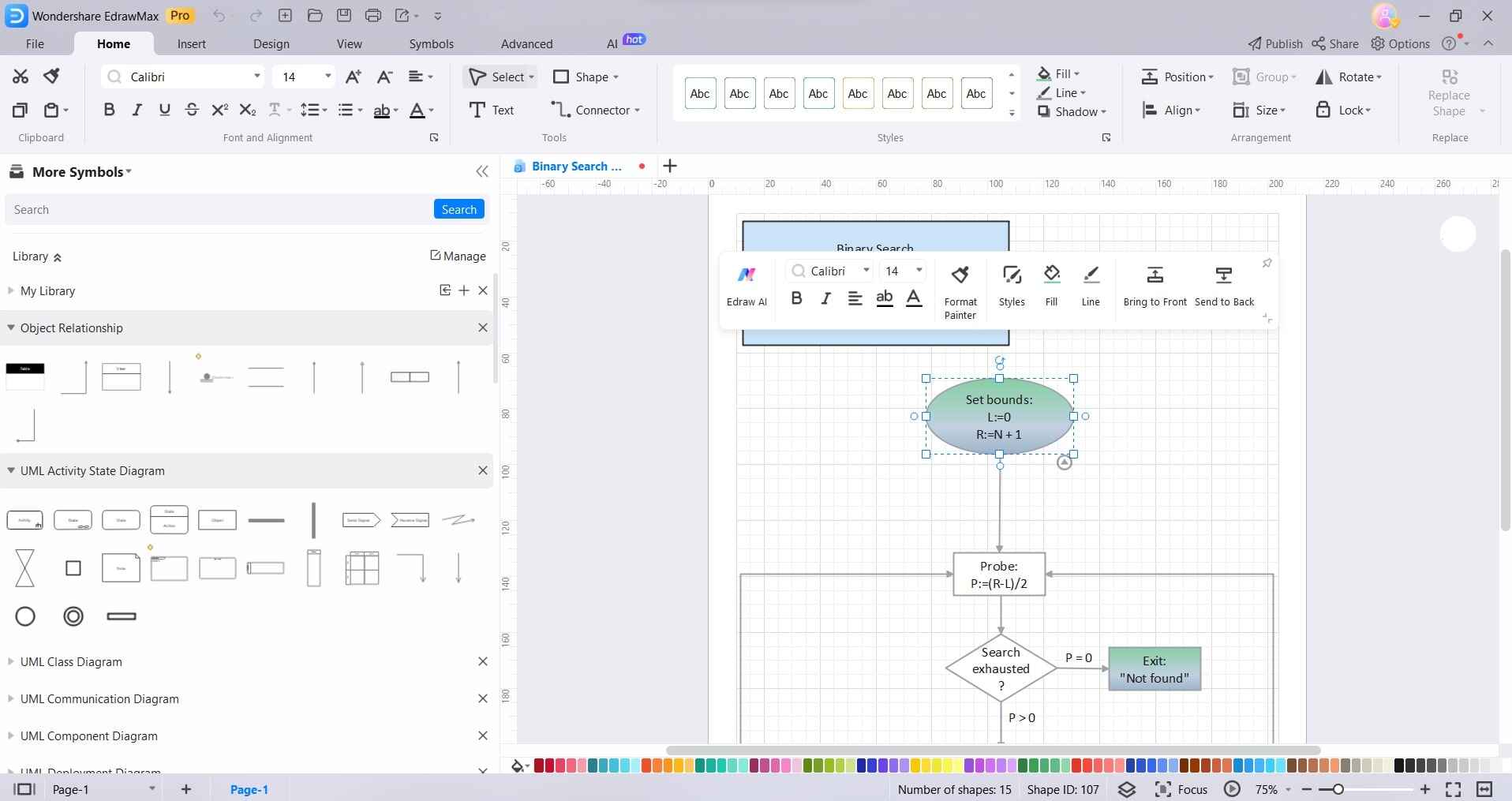
Step 4:
Select individual symbols or sections of your flowchart and use the color palette provided in EdrawMax to modify the fill or outline colors.
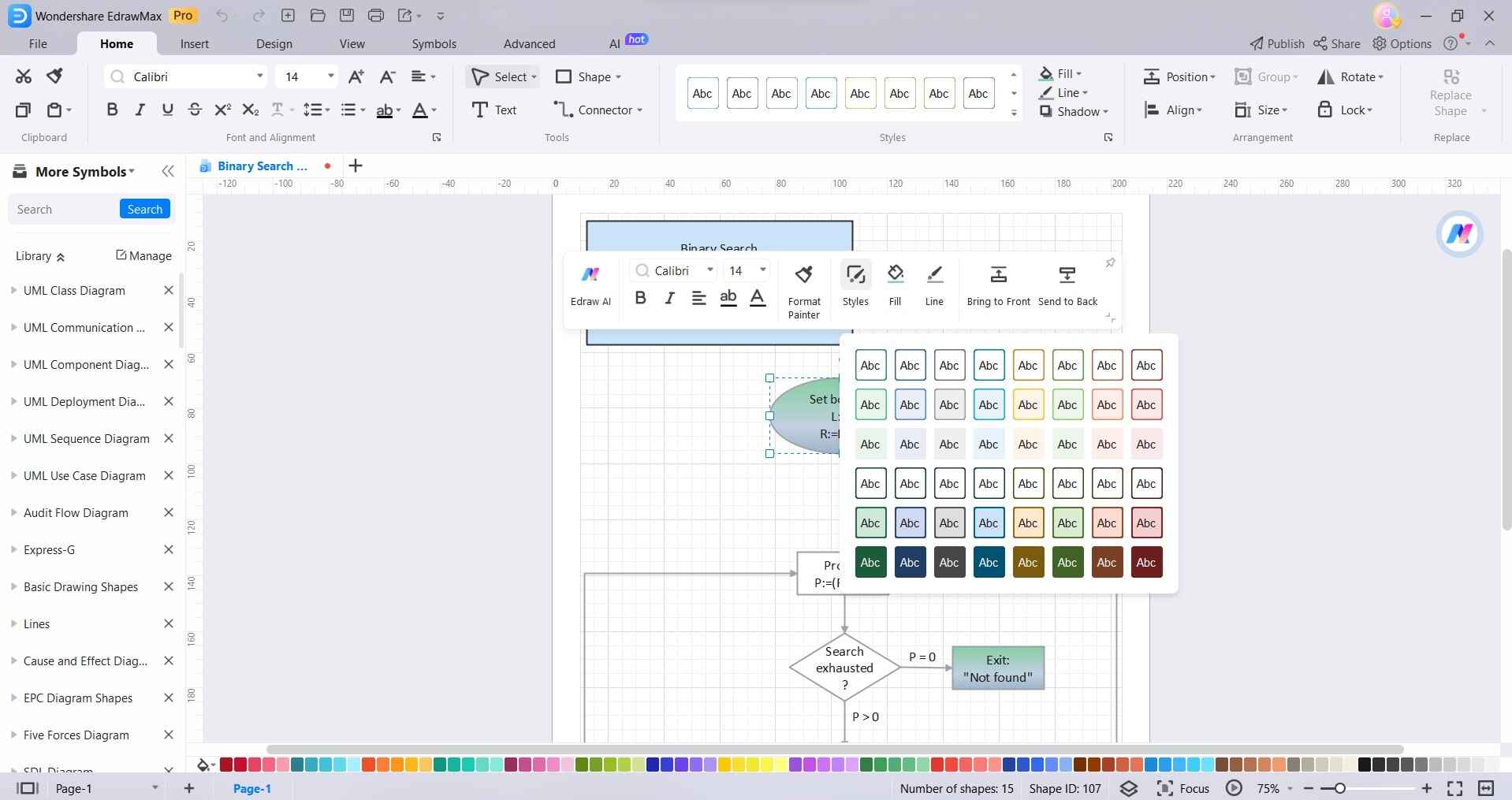
Step 5:
Once satisfied with the flowchart, save your work in EdrawMax. You can export it in various formats like PNG, JPEG, PDF, etc., for sharing or integration into documents or presentations.
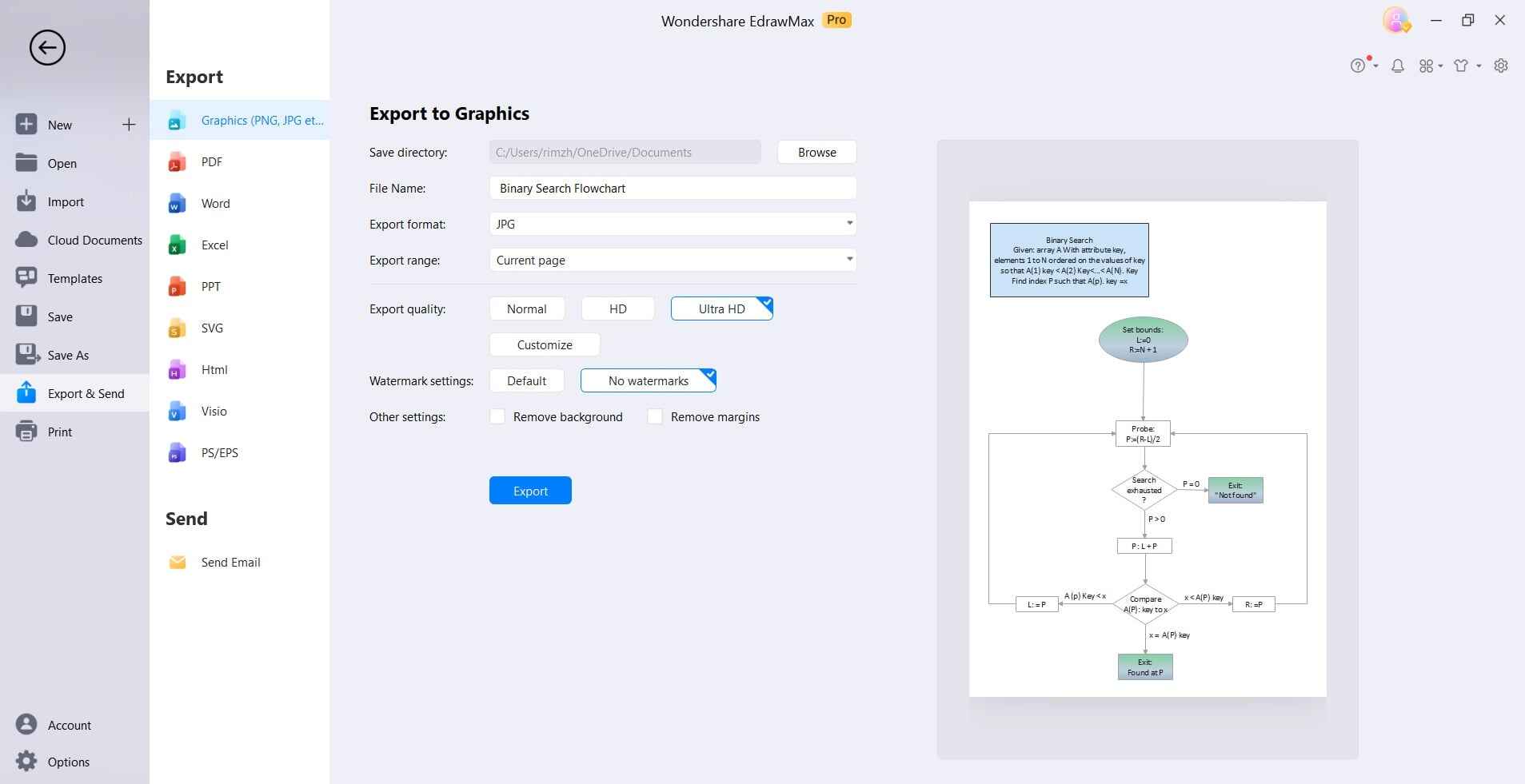
EdrawMax offers various symbols and shapes suitable for creating flowcharts, allowing you to visually represent the binary search algorithm step by step.
Conclusion
Mastering data structures like the Binary Search Tree program in C and C++ paves the way for solving complex coding challenges. A BST strikes the right balance of simplicity for learning while providing high performance to leverage across applications.
Whether for education or professional projects, walking through the hands-on process from flowcharting to code delivers valuable insights into algorithmic thinking aligned with software engineering best practices.
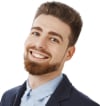