Heap sort is an efficient sorting algorithm that works by organizing data into a specialized tree-like structure known as a heap. The heap itself has some useful properties that allow the algorithm to access the data in an ordered fashion, even though it is stored in an unordered binary tree. While it has similar time complexities to popular algorithms like quick sort, heap sort has one distinct advantage - it performs sorting in place with no need for additional storage space.
In this article, we will take an in-depth look at heap sort with detailed C code examples and walkthroughs.
In this article
Part 1: What is a Heap Sort Program?
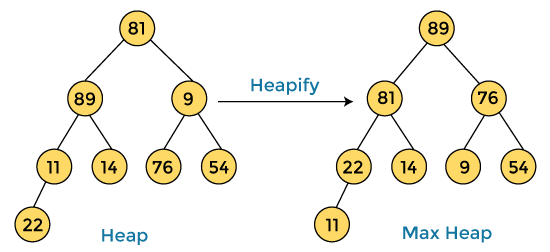
In essence, a heap sort organizes data based on binary heaps - tree data structures where each parent node is related to child nodes. Specifically, it utilizes max and min heaps where parent nodes are greater than (max) or less than (min) child node values to enable quick access to maximum and minimum values in the dataset respectively.
The key steps involved in a typical heap sort are:
- Convert the input unsorted array into a specialized max/min heap. This heap will allow easy access to the current extremum (maximum or minimum).
- Swap the first element (greatest/smallest) in the heap with the last element in the input array. Reduce array size by 1.
- Convert the smaller array back into a heap structure, putting the new maximum/minimum value in the first position again.
- Repeat steps 2-3 reducing array size by 1 each time until the whole array is sorted.
As we’ll see through the C program example, maintaining the heap property while sorting is crucial for ensuring worst-case O(nlogn) time complexity.
Part 2: Steps for Implementing a Heap Sort Program in C
Here are the steps to implement a generic heap sort algorithm in C without providing the actual code.
- Heapify Function: Write a function heapify to maintain the heap structure: compare a node with its children and swap if needed to maintain the heap property.
- Build Max Heap: Create a max heap from the array using the heapify function.
- Heap Sort: Continuously extract the maximum element (root) and place it at the end of the array.
- Implementation: Develop a heapSort function to manage building the heap and sorting.
- Testing: Validate the implementation with different input arrays to ensure correctness.
- Optimization: Optimize and debug as needed by tracing through the code for proper heap structure maintenance and sorting.
- Finalization: Once validated, encapsulate the algorithm within a main function for practical usage.
Part 3: Things to Consider When Using a Heap Sort
Heap sort is a versatile sorting algorithm and can be remarkably efficient with an optimal O(nlogn) performance. However, there are some considerations when deciding if heap sort fits your use case:
- The algorithm performs sorting in place so is very memory efficient. But it is not a stable sort i.e. elements with the same value may get swapped, changing order.
- There are faster algorithms for smaller data sizes. For arrays under ~100 elements, simple sorts like insertion sort will be faster.
- The worst case is poor at O(nlogn). Algorithms like quick sort have better consistency with an average case = best case.
- Not easy to parallelize efficiently due to sequential element access and swap requirements. Parallel versions can be complex to design.
Overall heap sort works well for medium-sized arrays where stability is not required. It is popular in languages emphasizing memory efficiency like Java.
Part 4: Creating an Algorithm Flowchart using EdrawMax
EdrawMax is an invaluable tool for programmers to visualize algorithms and program logic flows. Creating detailed flowchart diagrams in EdrawMax provides significant benefits for understanding and implementing complex code like heap sort.
Firstly, the intuitive drag-and-drop interface makes it easy to translate programming concepts. Secondly, the styling customizability enables programmers to craft clean, well-formatted charts.
In summary, EdrawMax charts lend visibility into coded algorithms, simplify complex program analysis, and enable collaborative programming - reasons why it should be a vital part of every programmer's toolkit alongside traditional code editors.
Here are the steps to create a simple sorted array algorithm flowchart using EdrawMax:
Step 1:
Launch the EdrawMax application on your computer. Choose the "Flowchart" category from the template gallery.
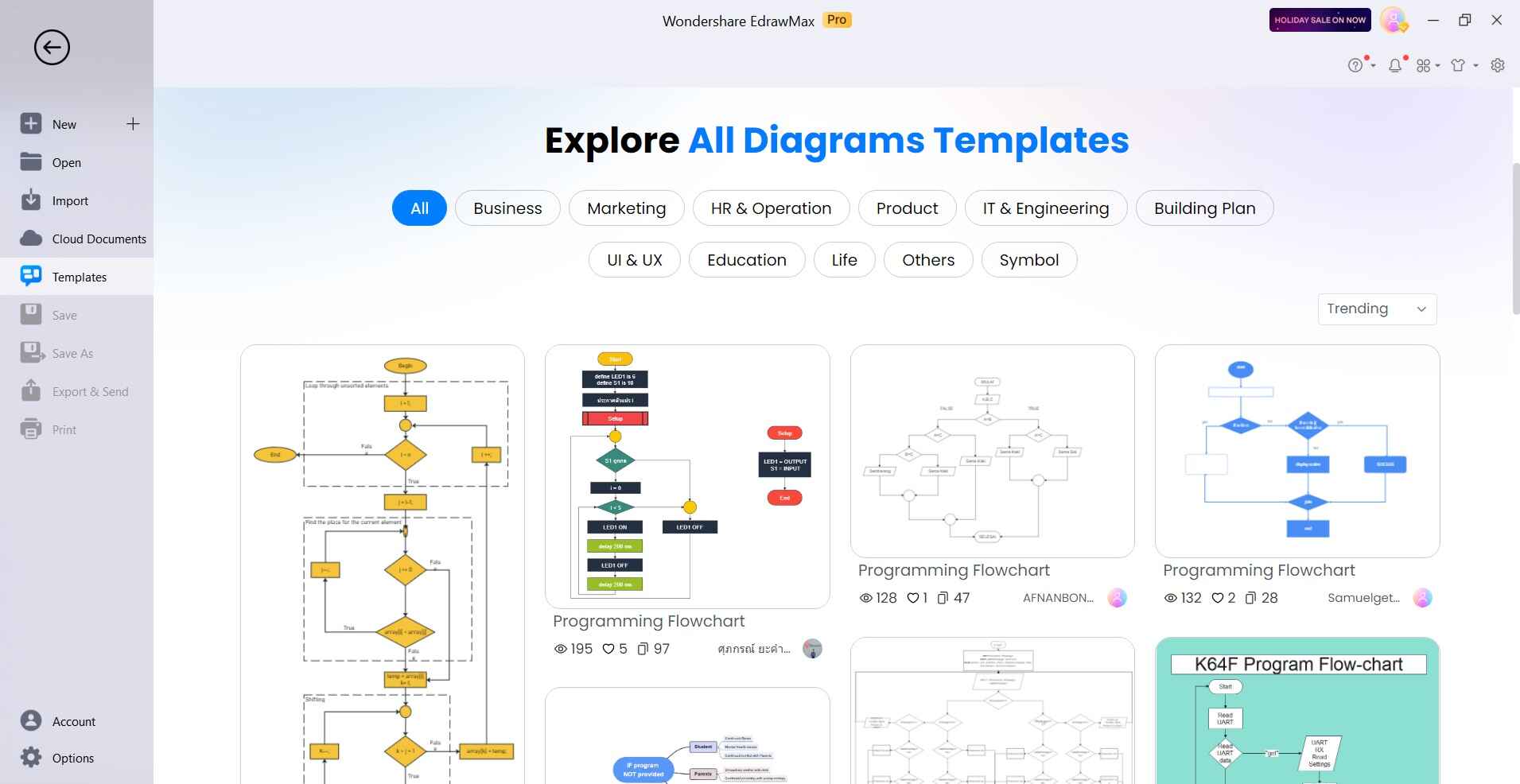
Step 2:
Use appropriate symbols (start/end, process, decision, input/output) from the symbol library provided in EdrawMax. Drag and drop these symbols onto the canvas to represent the steps of your algorithm.
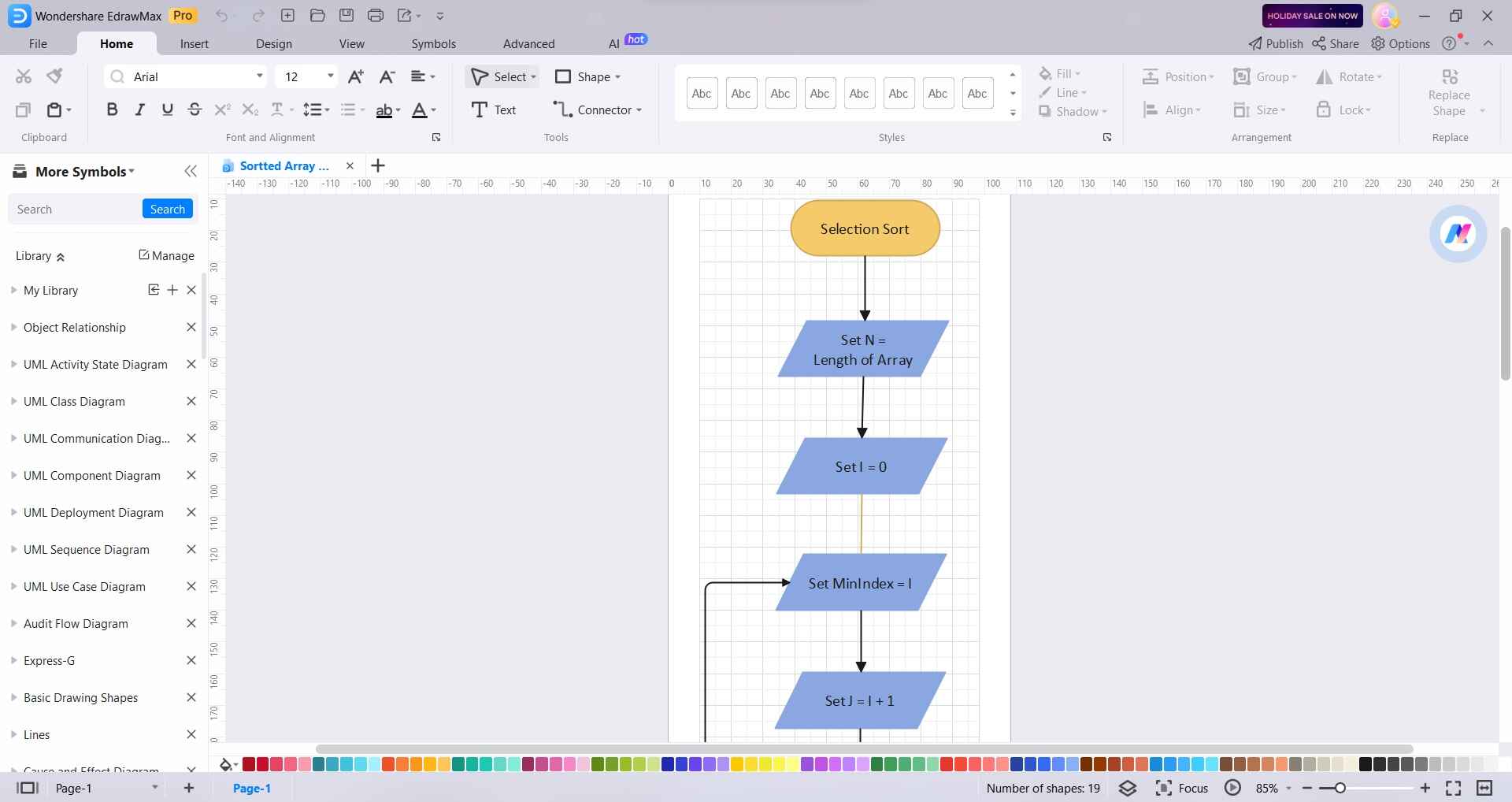
Step 3:
Label each symbol with descriptive text to explain the action or decision.
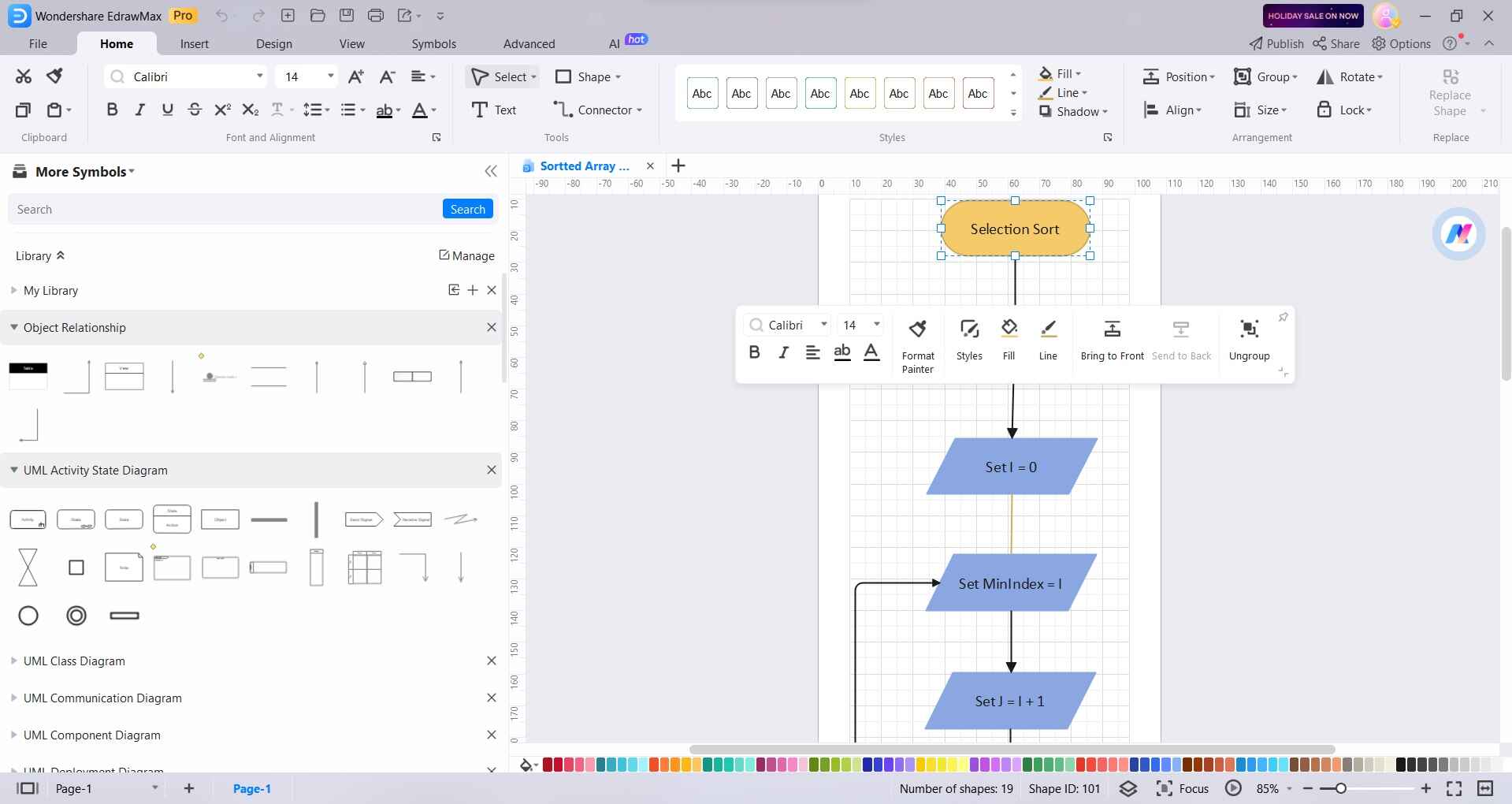
Step 4:
Customize the flowchart by changing colors, line styles, or shapes. Use formatting tools provided in EdrawMax to enhance the visual appeal and readability.
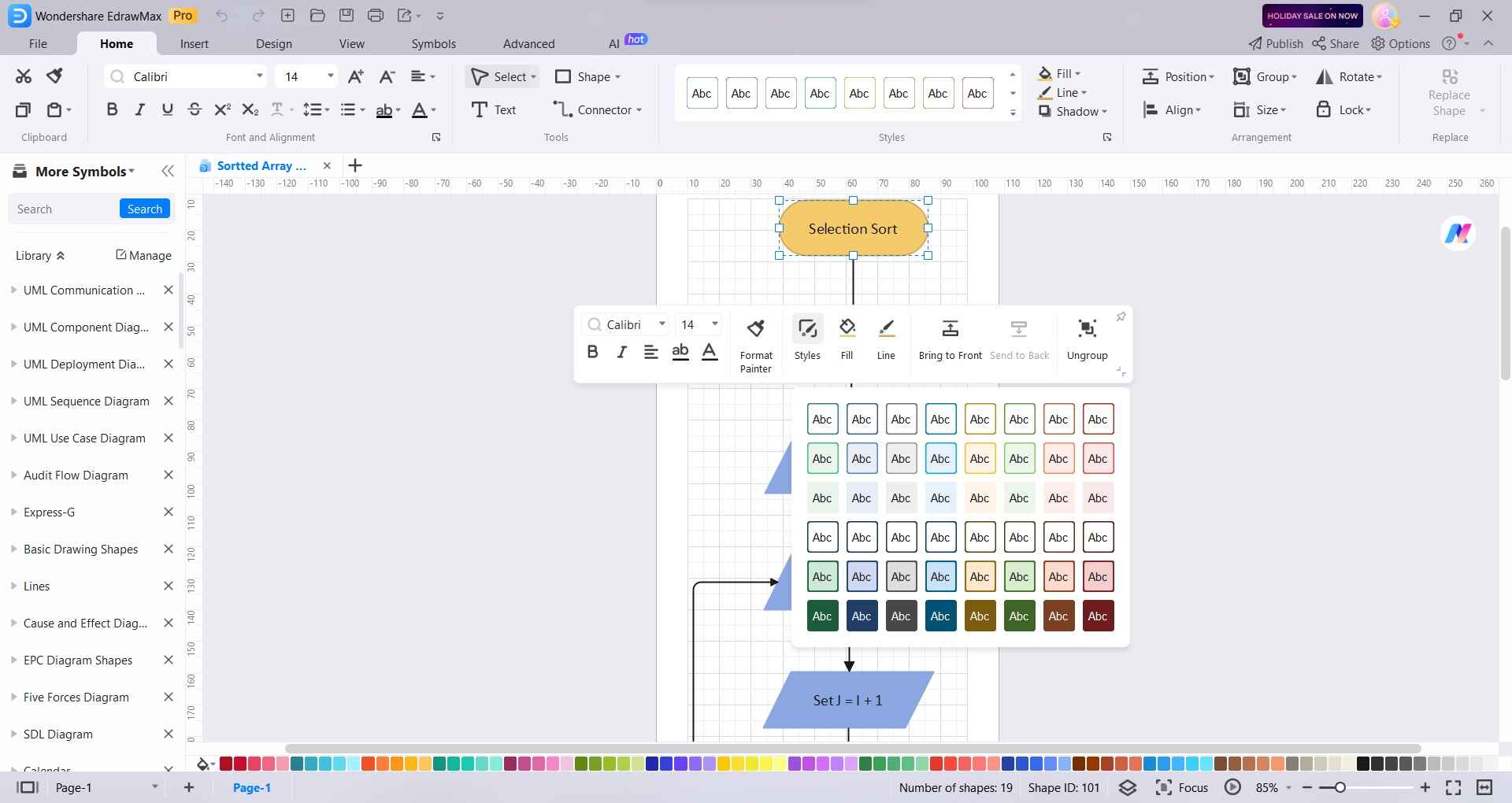
Step 5:
Once completed, save your flowchart in a preferred format (e.g., .eddx, .jpg, .png). You can also export it in various file formats or share it directly from EdrawMax.
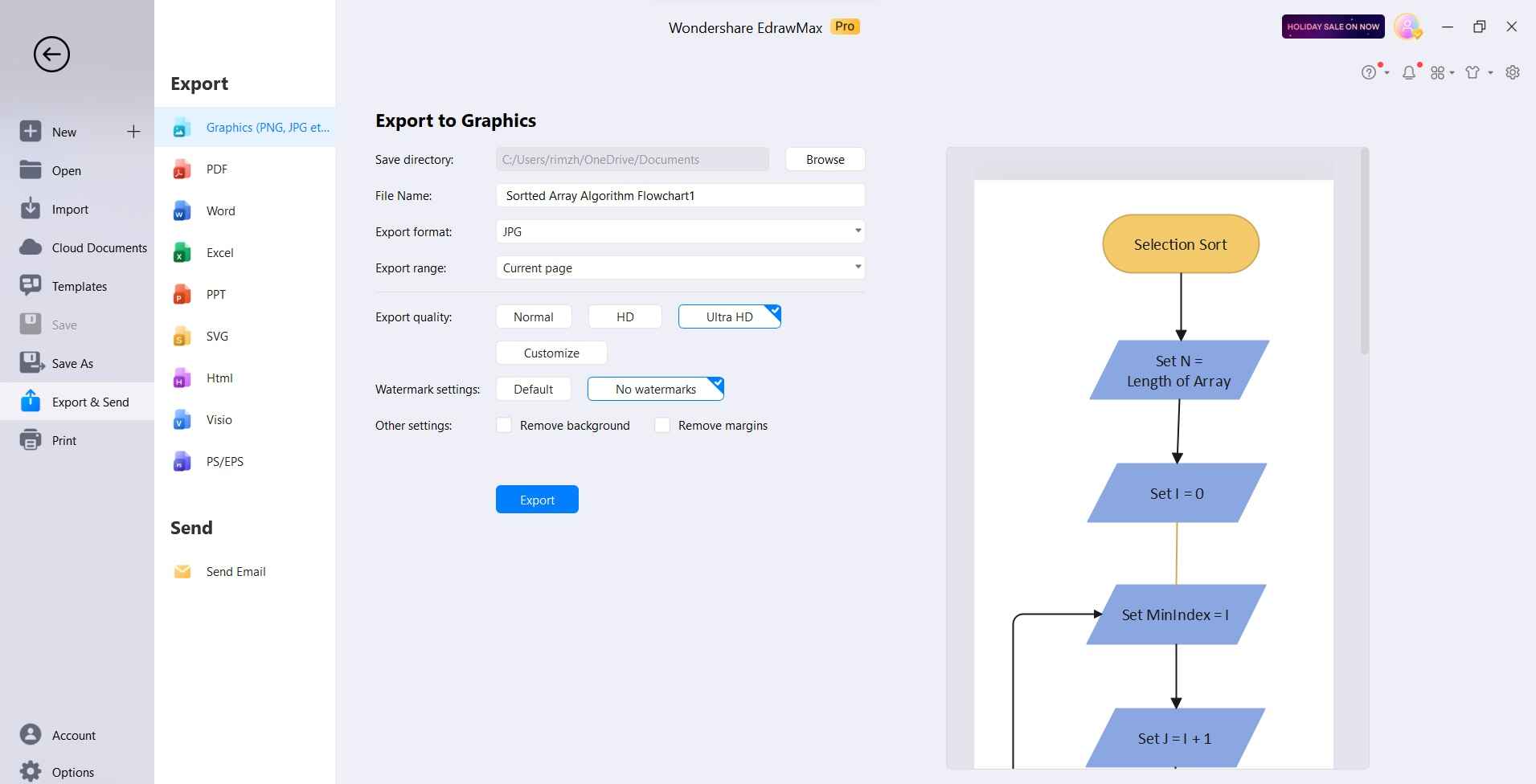
Following these steps will help you create a clear and comprehensible programming algorithm flowchart using EdrawMax.
Conclusion
We have explored heap sort in-depth - understanding the essential heap concepts, walking through a full C program, contrasting Python and Java, considering real-world usage, and visualizing the algorithm.
Heap sort is an extremely useful addition to any programmer's toolbox allowing efficient in-place sorting with minimal memory overhead.
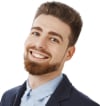