A max heap is an important data structure used to implement priority queues and facilitate fast access to the maximum element. It is implemented using a complete binary tree that satisfies the max heap property - the value of each node is greater than or equal to the value of its children. Mastering max heaps is key for anyone looking to improve their data structures and algorithms skills.
In this article
Part 1: What is a Max Heap Python?
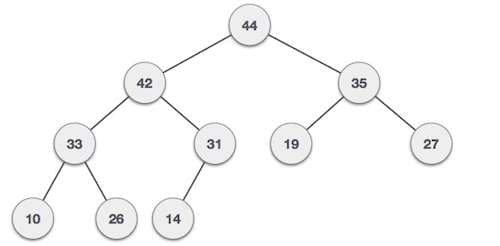
A max heap Python refers to the max heap data structure implemented in Python. It is based on the heapq module which provides the heappushpop(), heappush(), and heappop() functions to insert and remove elements while maintaining the max heap structure.
Part 2: Difference Between Max Heap Python and Max Heap C++
While conceptually max heaps in Python and C++ are identical, there are some key differences when it comes to actual implementation:
- Python's heapq module provides ready-made functions to create and manage a max heap whereas C++ uses classes to implement a max heap.
- Python handles most of the underlying heap operations implicitly while they have to be explicitly coded out in C++.
- Insertion and deletion of elements have a complexity of O(Log n) in both cases but the constants differ based on indexing calculations, swapping elements, etc.
- Python's max heap cannot be accessed directly whereas C++ implementation allows direct access to any element through the use of pointers or iterators.
So in essence, Python provides easier management but less flexibility compared to C++ implementation.
Part 3: Overview of Min Heap and Max Heap
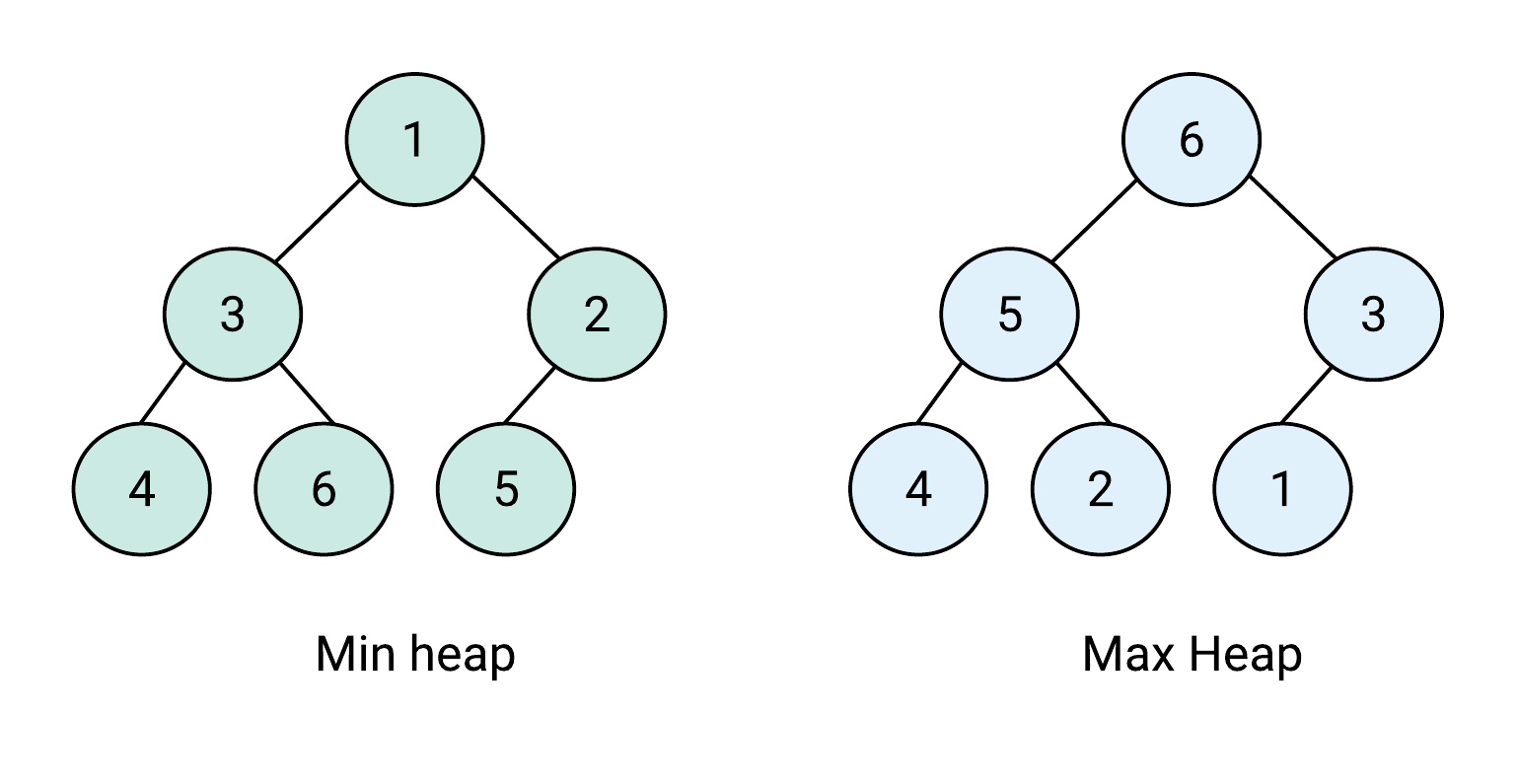
Before we dive into building a max heap, it's useful to distinguish between a min heap and a max heap:
Min Heap:
- The root node has the minimum value.
- Parent nodes have values less than or equal to child nodes.
- Used to implement priority queues requiring minimum value extraction.
Max Heap:
- The root node has the maximum value.
- Parent nodes have values greater than or equal to child nodes.
- Used to implement priority queues requiring maximum value extraction.
Choosing one over the other depends on the target application.
Part 4: Steps to Build Max Heap Effortlessly
Here is a step-by-step process to build a max heap:
- Define Node Structure with Key Value: Create a node structure with a key or value attribute and pointers to track left and right child nodes.
- Initialize Empty Heap: Start with an empty heap. Initialize an array, list, or vector to store heap elements.
- Insert New Elements: New elements can be inserted at the end of the existing heap. Then "heapify up" to maintain max heap property.
- Implement Heapify Up: To maintain the max heap order property, swap the newly inserted element with its parent if it is greater than its parent. Keep comparing with parents until the max heap property holds.
- Implement Extract Max Element: The root contains the greatest element in a max heap. Save and remove it to extract the max element.
- Heapify Down: With the root removed, the new root element may violate the max heap property.
Following the above steps and using the Python heapq module enables building a max heap with ease!
Part 5: Creating a Programming Flowchart Using EdrawMax
EdrawMax is a cross-platform diagramming and visualization software that can greatly help in designing, documenting, and visually depicting workflows for data structures like heaps.
Here are some key benefits of using EdrawMax for creating max heap programming flowcharts:
- Intuitive Drag and Drop Interface: No coding required! Easy to learn and master tool with drag and drop symbols and template examples across different flowchart types.
- Powerful Customization Options: Customize flowchart appearance through flexible styling options for shapes, alignments, fonts colors, etc. based on preferences.
- Automatic Chart Layout Features: Smartly align, distribute, and orient flowchart shapes and connectors with minimal effort through auto layout features.
- Interactive Navigation and Highlighting: Interactive click-and-select features make it easier to visually distinguish different processes across complex flowcharts.
- Effective Diagramming for Code Logic: Helps turn complex code logic into easy-to-understand visual diagrams through various flowchart types - program flowcharts, UML diagrams, etc.
By following max heap implementation steps, we've covered the key essentials to mastering max heaps through code.
Step 1:
Launch the EdrawMax application on your computer. Once in the software, navigate to the "Flowchart" category or search for "Flowchart" in the template search bar. Choose a suitable template to start with or begin with a blank canvas.
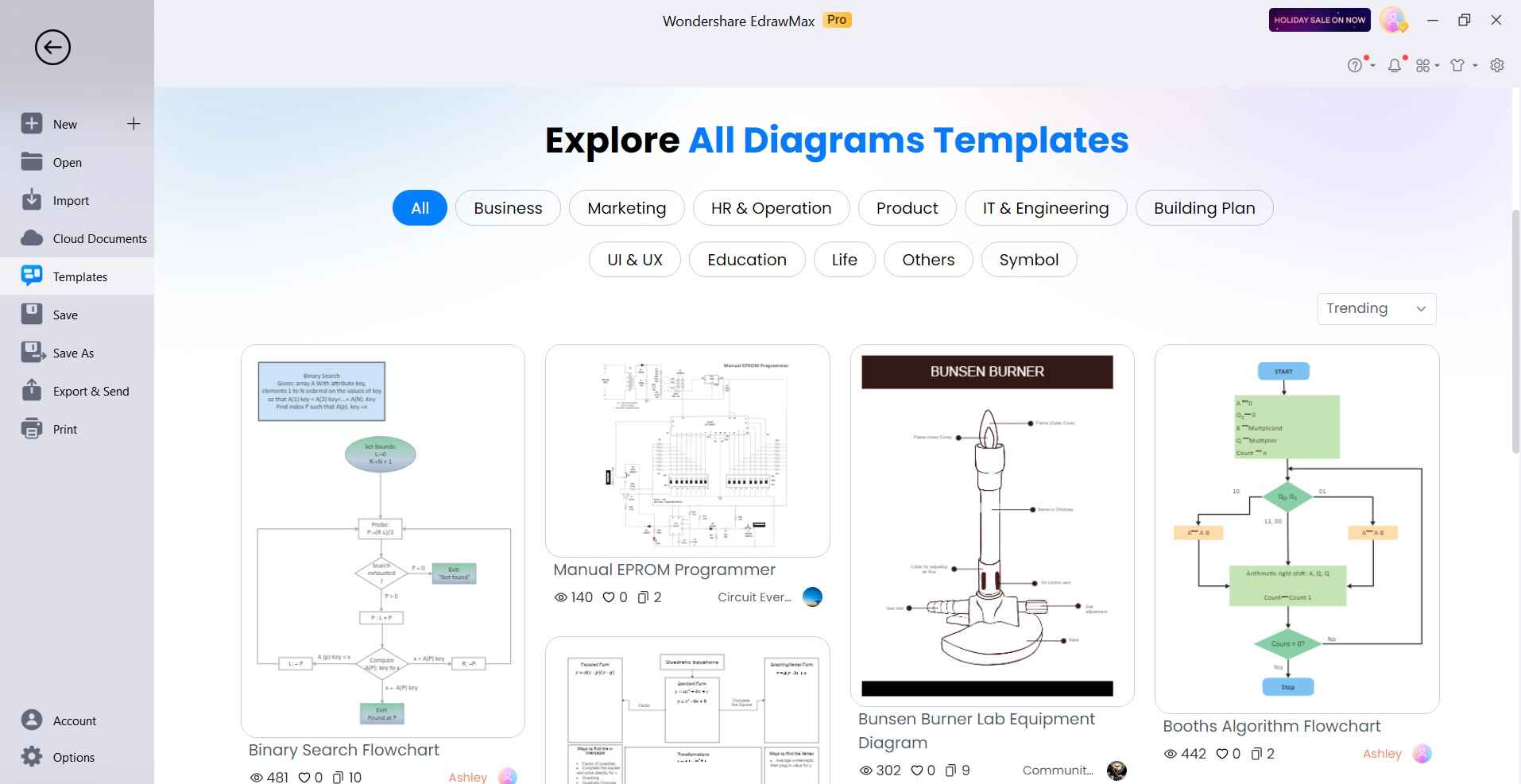
Step 2:
Drag and drop shapes from the left-hand side panel onto the canvas.
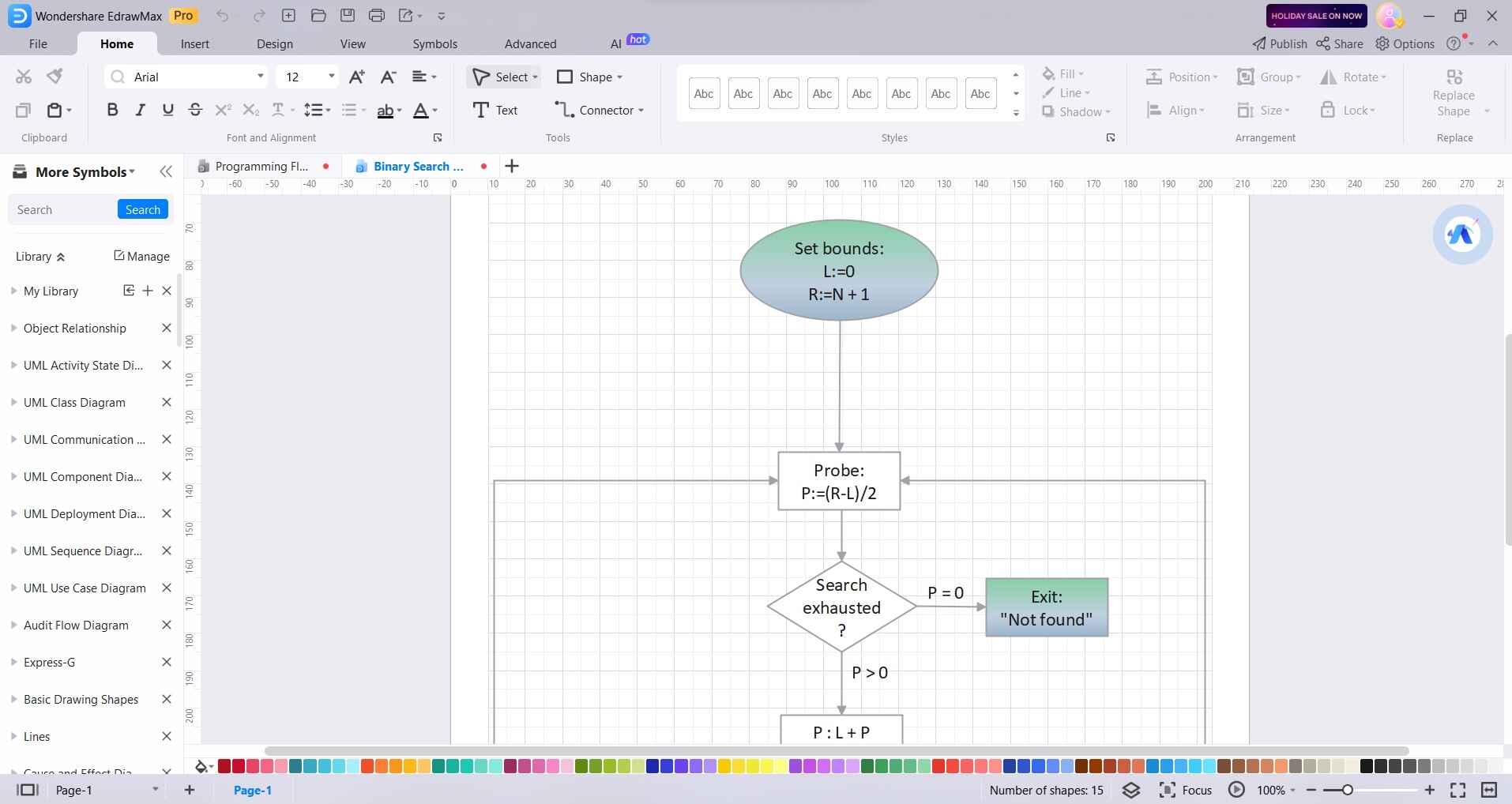
Step 3:
Double-click on each shape to add text or labels representing actions, decisions, or process steps.
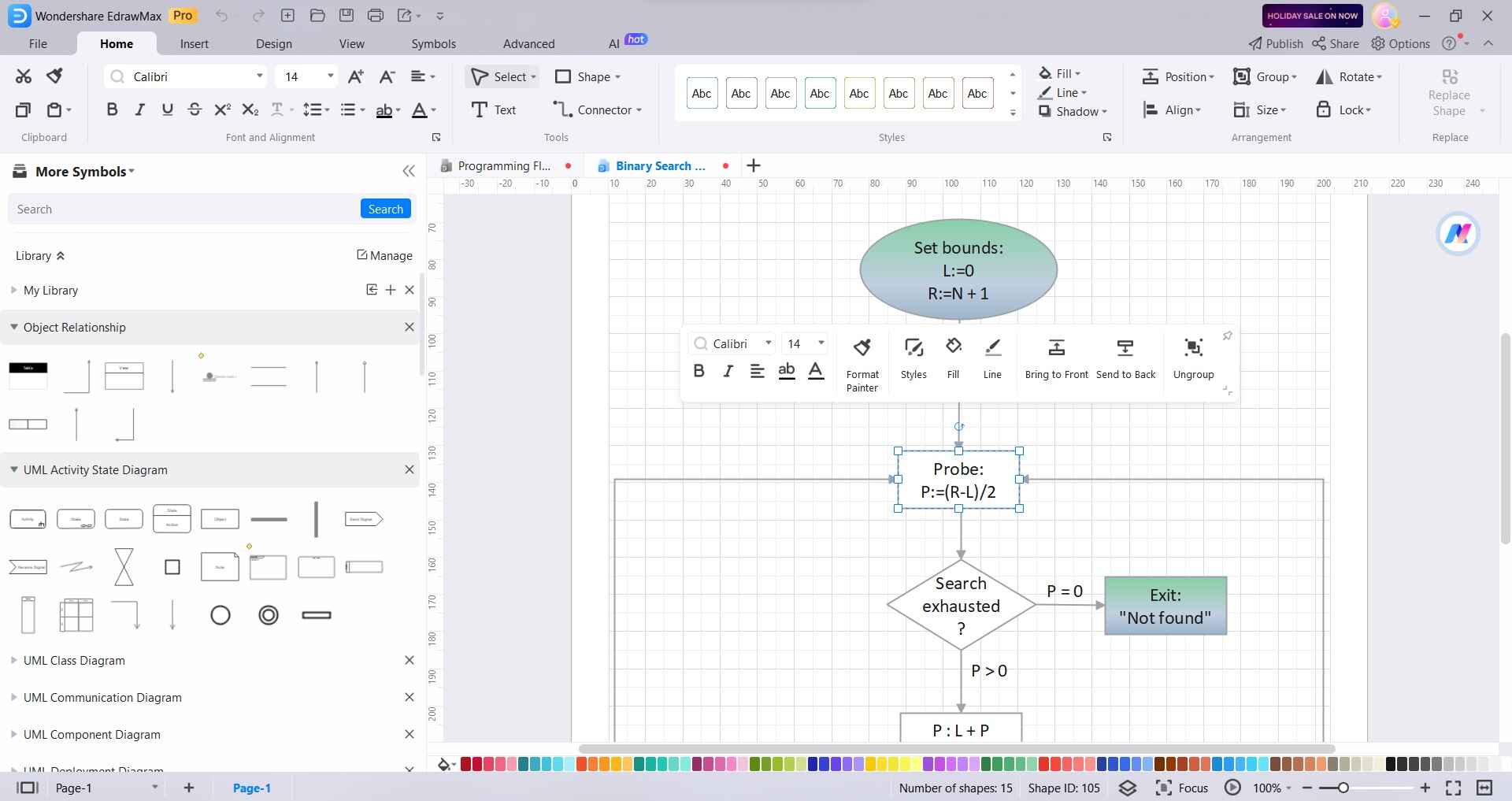
Step 4:
Customize the appearance of shapes, lines, and text using the formatting options.
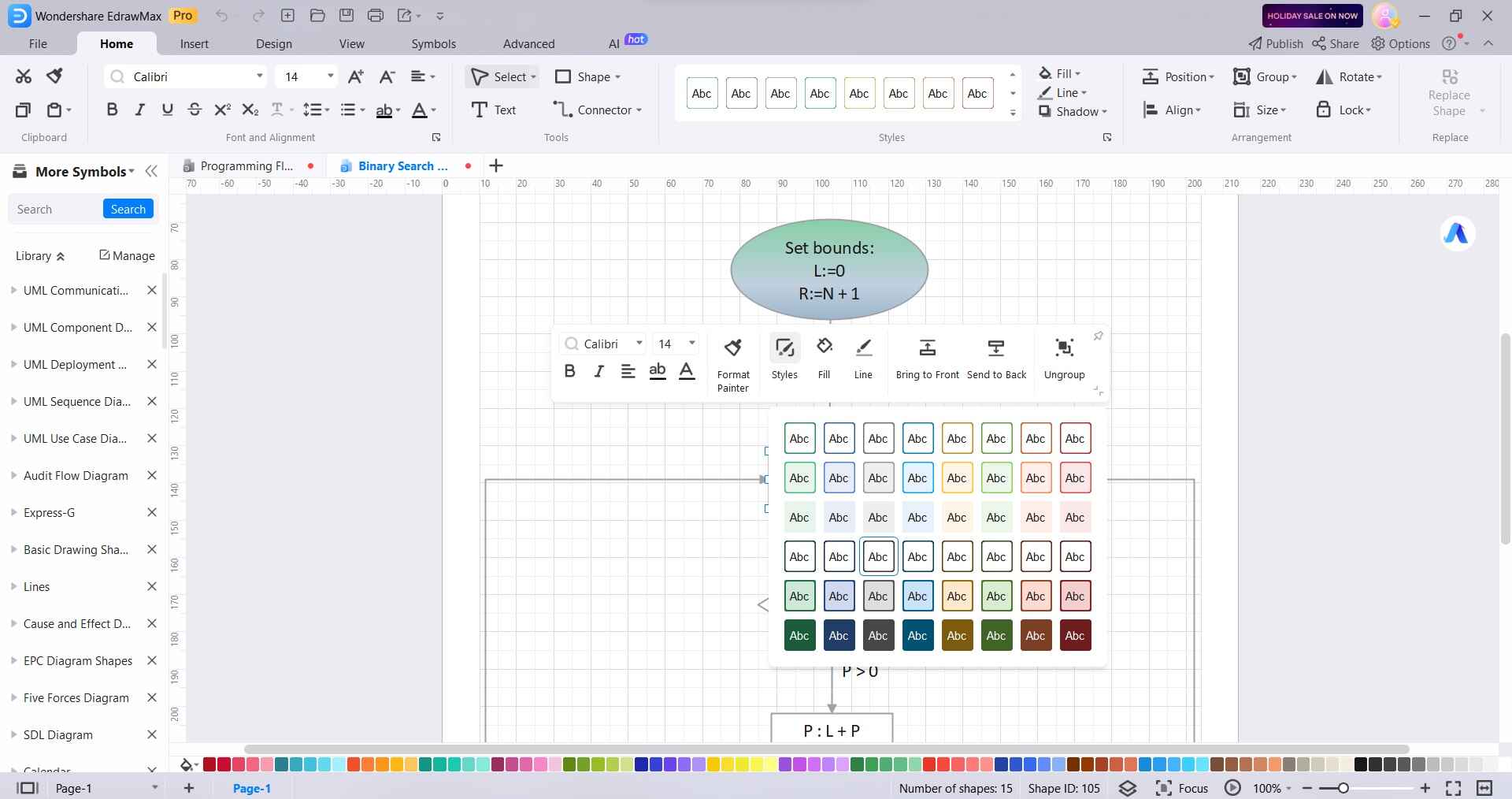
Step 5:
Once satisfied, save your flowchart in your desired format (EdrawMax native file, PDF, PNG, etc.) and location on your computer.
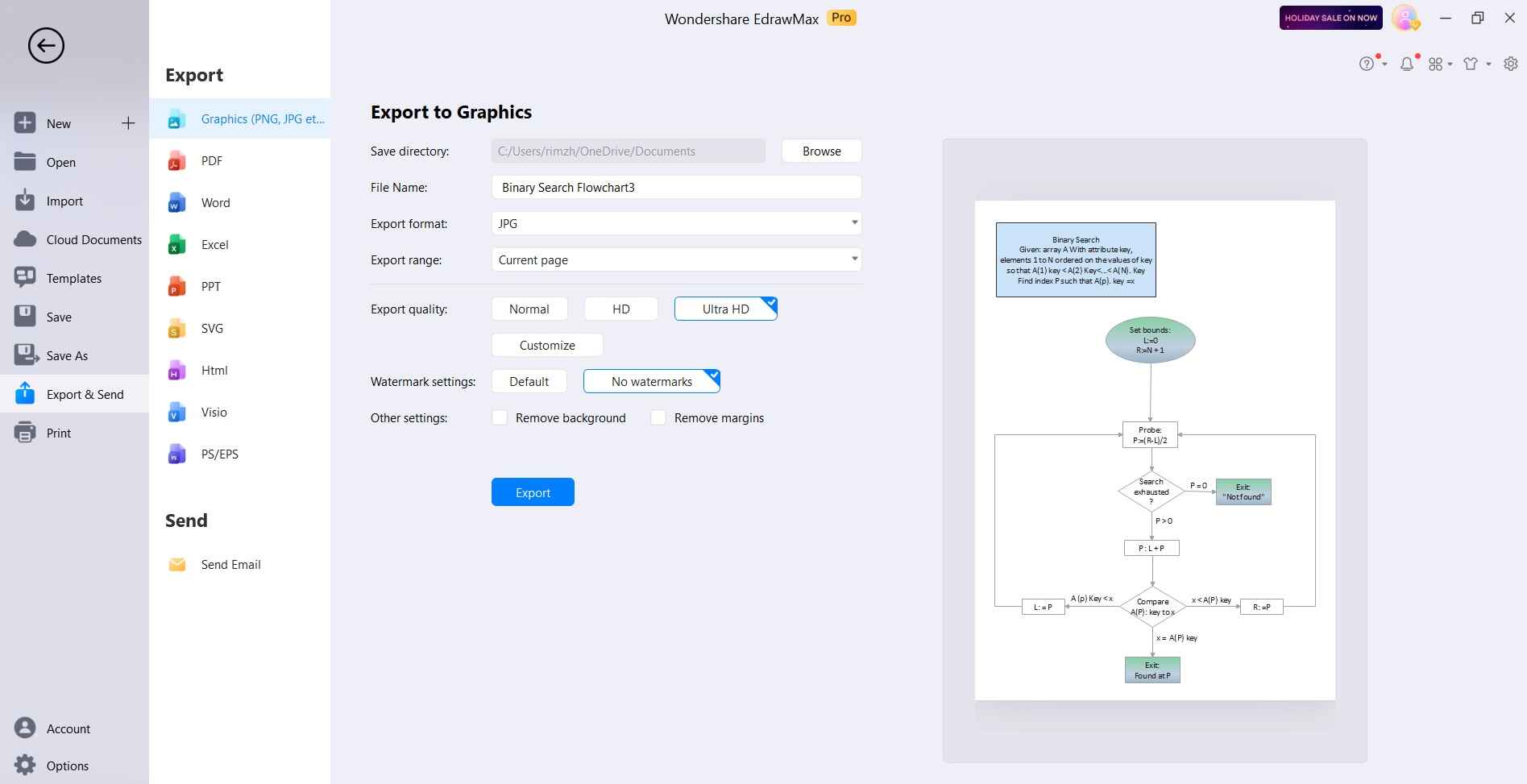
EdrawMax takes it further through its powerful flowchart and visualization capabilities for showcasing programming logic!
Conclusion
There you have it - a definitive guide to understanding and efficiently implementing max heaps in Python. We looked at properties and inner workings of a max heap Python, differences when using C++, an overview of max vs min heaps, a step-by-step process to build a max heap, and finally how EdrawMax simplifies turning code logic through impactful visualized flowcharts.
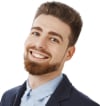