Data structures provide organized and efficient ways of storing, accessing, and manipulating data in computer programs. Choosing the right data structure for a particular task can greatly improve code efficiency and performance.
Python offers built-in data structure types like stacks, queues, and trees. This article will provide a comprehensive guide to the most common data structures used in Python.
In this article
Part 1: What is a Data Structure in Python?
A data structure in Python refers to a particular way of organizing data in the computer's memory so that it can be accessed and worked with efficiently. Some key characteristics of data structures in Python include:
- They are containers that hold data of a specific type. For example, lists hold an ordered collection of objects, while dictionaries store key-value pairs.
- They use certain rules and organizing principles to determine how data is stored, related, and operated upon. These rules allow for quicker access and analysis compared to unstructured data.
Well-designed data structures optimized for data access, modification, and size efficiency. Picking suitable data structures is key for writing optimized Python code.
Part 2: Types of Data Structures in Python
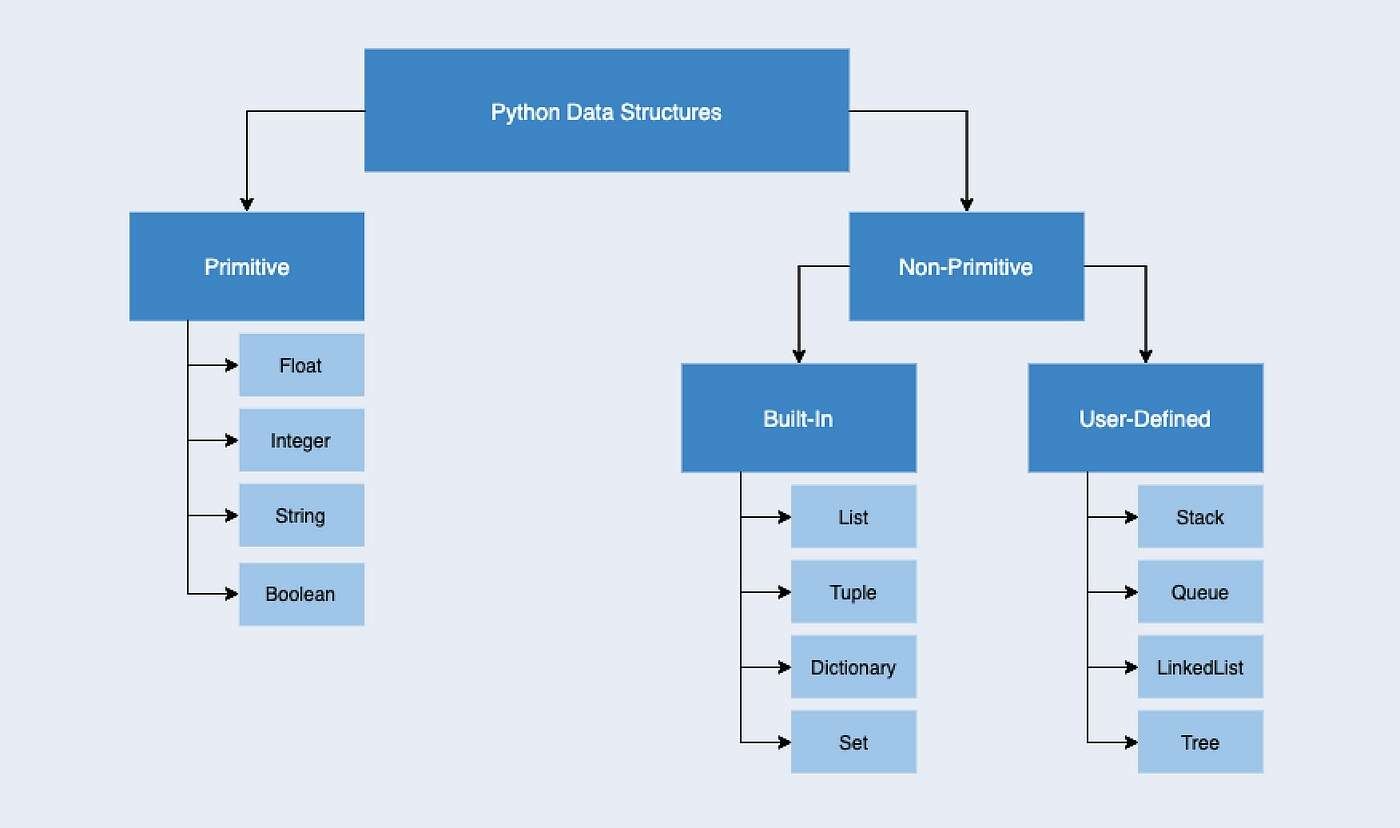
Some important data structures in Python include:
#1 Tree
A tree data structure in Python is used to store data in a parent-child relationship. The top node of the tree is called the root, while the leaf nodes are at the bottom with no children. Trees allow efficient insertion, searching, and removal of elements.
#2 Heap
A heap data structure in Python is a specialized tree data structure that satisfies the “heap property” - child nodes are always smaller than parent nodes. Elements can be efficiently added or removed from heaps in O(log N) time.
#3 List
List data structures in Python are mutable sequences capable of holding any data type, offering a host of methods and easy iteration for loops. Common operations include appending/removing elements, sorting, slicing, searching, and reversing lists in O(N) time.
#4 Stack
The Stack data structure in Python is linear Last-in-first-out (LIFO) which allows adding and removing elements in sequential order. Push and pop are the key operations offered by stacks. This makes them useful for various computing tasks like managing function calls, syntax parsing, and more.
#5 Queue
In contrast to stacks, queue data structure in Python are First In First Out (FIFO) structures suitable for buffered waiting line scenarios. Items are added to the queue's tail and taken out from its head.
Part 3: Difference Between Queue and Stack Data Structures in Python
While queue and stack data structures in Python may seem similar since they manage ordered collections of elements, there are some key differences:
- Ordering: Queues follow FIFO while stacks follow LIFO.
- Ends: Insertion and deletion happen at opposite ends for queues and stacks.
- Use cases: Stacks handle function calls, and queues handle processing workflows.
- Implementation: Stacks use linked lists or arrays, and queues often use doubly linked lists.
- Speed: Stacks have faster operations compared to queues.
Recognizing these distinctions allows selecting the right data structure for algorithm and program needs.
Part 4: Things to Consider When Implementing a Data Structure in Python
Here are some key considerations when implementing a data structure in Python:
- Mutable or immutable: Will the objects need to be modified after creation? Immutable structures like tuples can offer protection when data should not change.
- Supported operations: Define the key use cases upfront - access, search, insertions, etc. This impacts the choice of data structure.
- Efficiency: Structure the data to optimize for common operations based on computational complexity.
- Data relationships: Data structures like trees and graphs manage hierarchical, interconnected data very differently from linear structures.
Considering these design aspects allows for crafting optimized data structures for program needs.
Part 5: Creating a Programming Flowchart Using EdrawMax
EdrawMax is professional diagramming software that allows the easy creation of various diagrams and charts. When planning a programming project, EdrawMax helps map out the logical flow using flowcharts. Some key benefits include:
- Intuitive drag-and-drop: Easily construct flowcharts by adding common shapes and connecting them.
- Templates and symbols: Numerous templates like programming flowcharts with ready shapes reduce effort.
- Flexible editing: Dynamic editor makes updating charts simple.
- Export options: Charts can be exported as images, PDFs, word files, and more for sharing.
- Visualization: Charts allow clearer visualization of programming logic flows.
Here are the steps to create a simple programming flowchart using EdrawMax:
Step 1:
Launch the EdrawMax software on your computer. Click on "New" or "File" to start a new document. Choose the "Flowchart" category or search for "Flowchart" in the search bar to find suitable templates.
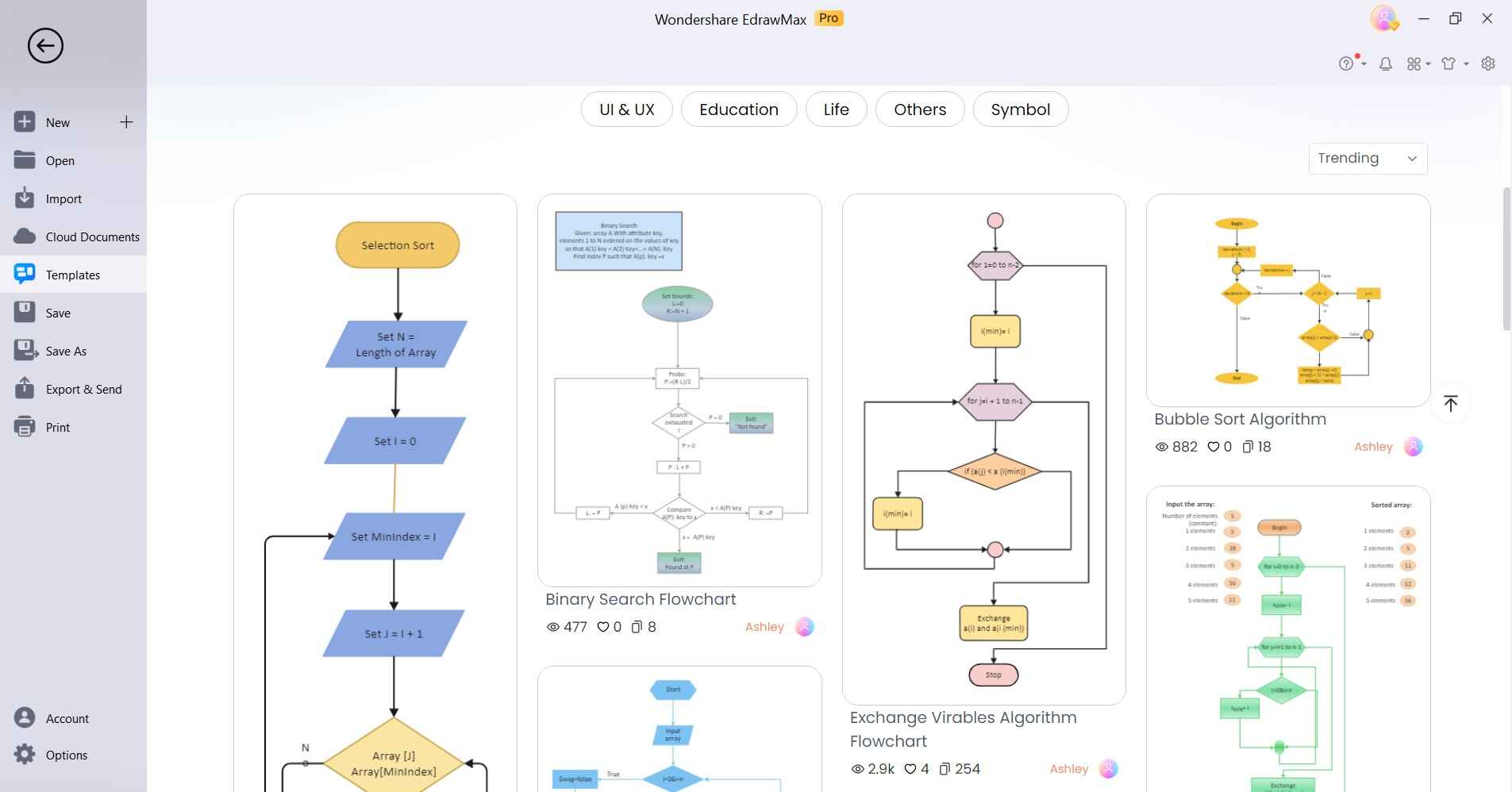
Step 2:
Drag and drop symbols onto the drawing page.
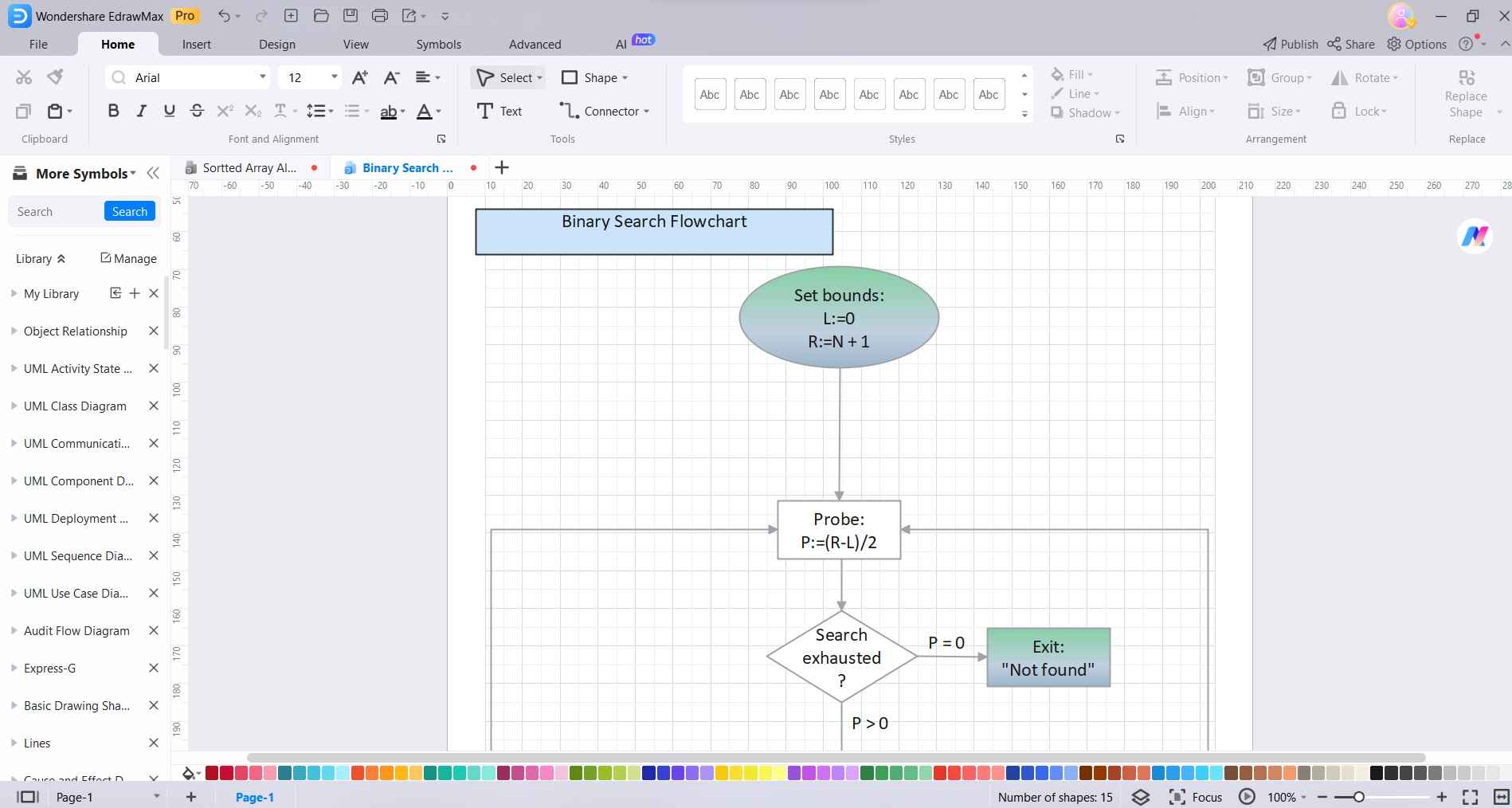
Step 3:
Use connector lines (arrows or lines) to link the symbols.
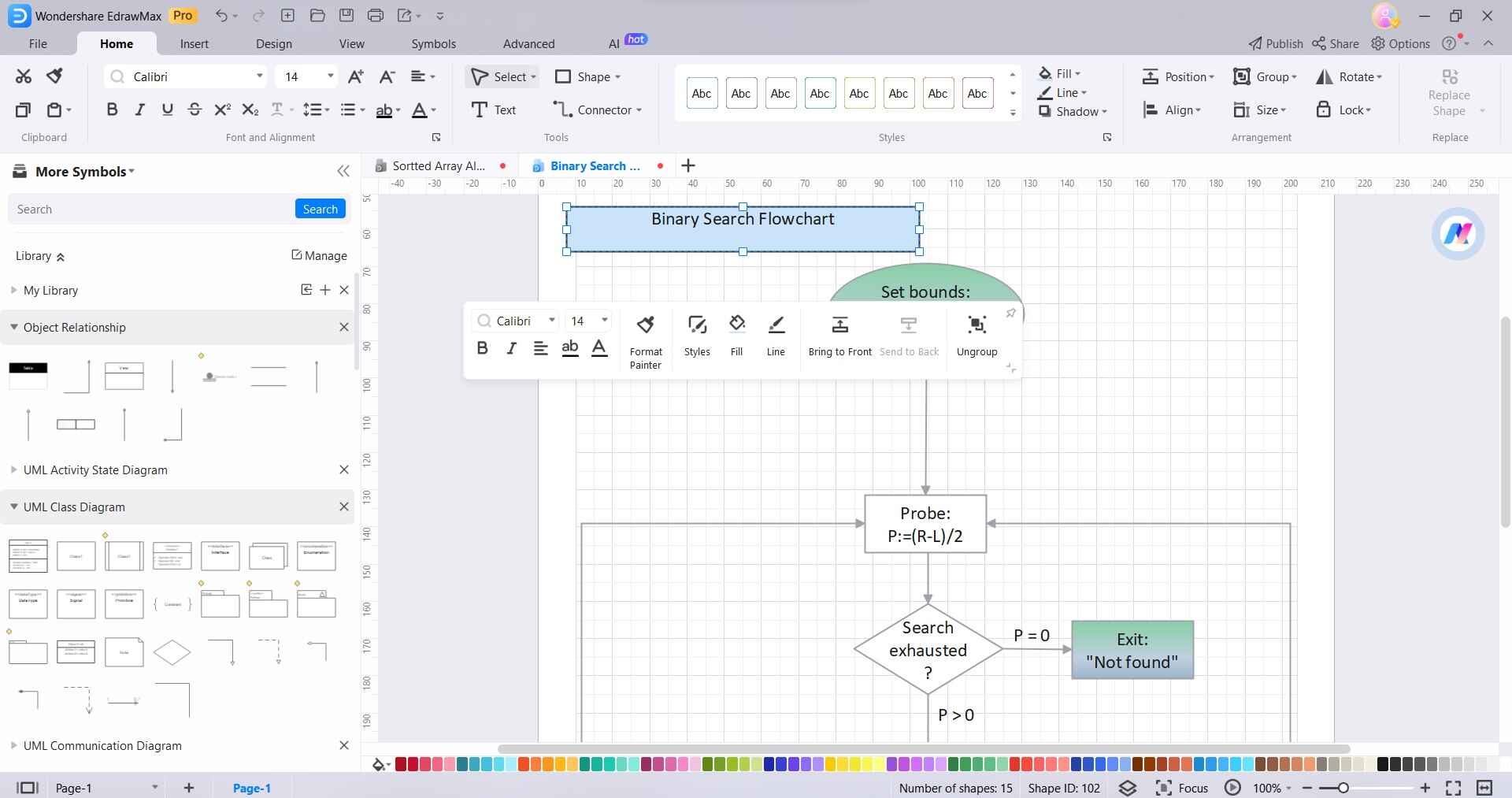
Step 4:
You can customize the flowchart by changing colors, font sizes, and styles to make it more visually appealing and easy to understand.
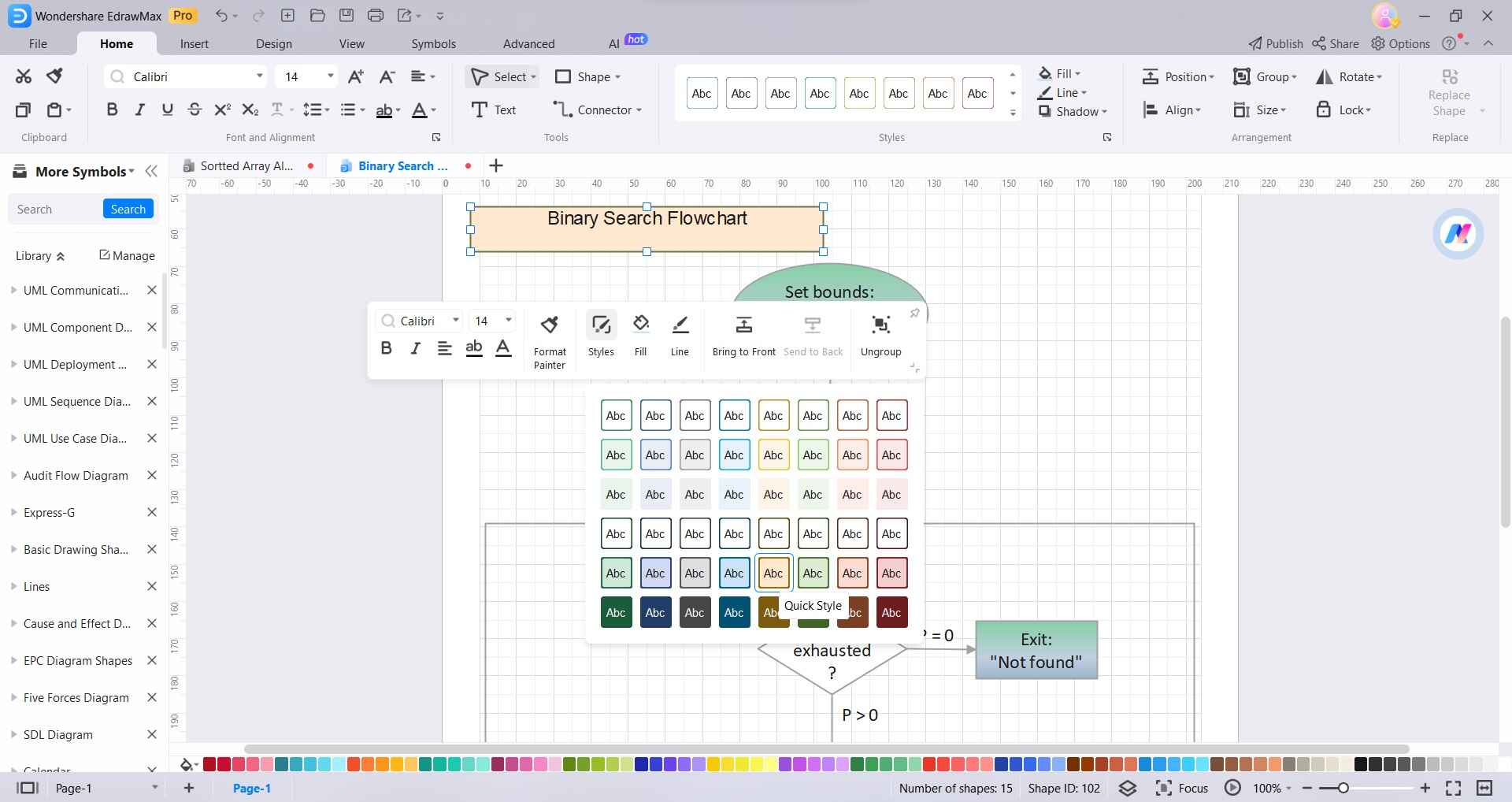
Step 5:
Save your flowchart in EdrawMax's native format (.eddx) or export it in a preferred format such as PNG, JPEG, PDF, etc.
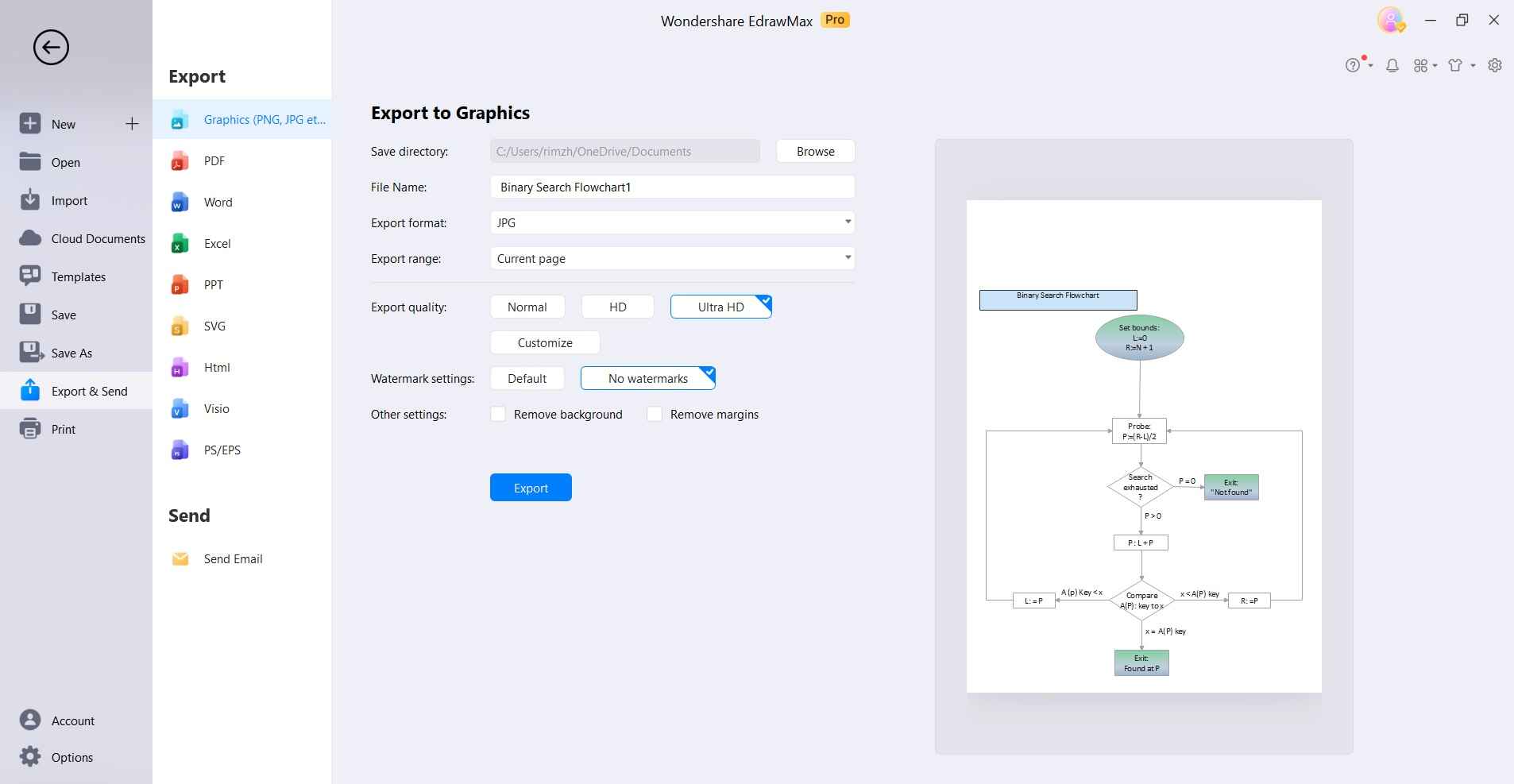
EdrawMax offers various symbols and features to create complex or detailed flowcharts. Explore its functionalities to enhance your flowchart as needed.
Conclusion
Python offers a variety of essential data structure implementations such as arrays, lists, stacks, queues, trees, graphs, and hash tables. Understanding the core capabilities of these data structures as outlined allows properly applying the right structure for a given programming task for efficiency.
Extending Python’s built-in structures with custom data structures when needed and leveraging helpful design tools like EdrawMax further help manage program complexity.