Trees are one of the most versatile and widely used data structures in computer science and programming. In Java, trees provide an effective way to handle hierarchical and recursive data with efficient algorithms for insertion, deletion, and traversal. Mastering trees is key for intermediate and advanced Java developers.
This article will provide an overview of working with trees in Java, including key algorithms and implementation details.
In this article
Part 1: What is a Data Structure in Java?
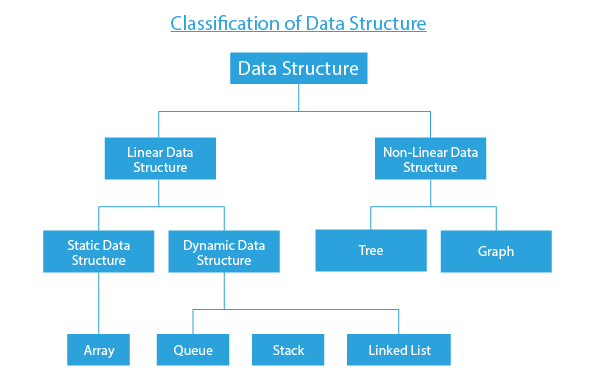
A data structure is a systematic way to organize data in a computer program so that it can be used efficiently. Some common data structures in Java include arrays, linked lists, stacks, queues, trees, graphs, and hash tables. Choosing the right data structure ensures operations like search, insert, and delete can be done quickly.
Part 2: Overview of Trees in Java
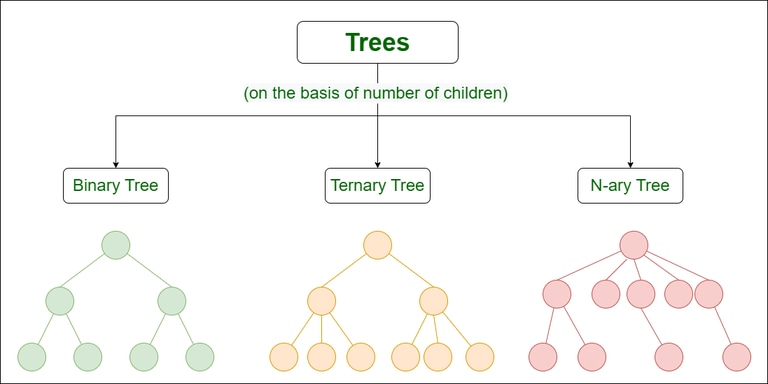
Trees organize data in a hierarchical structure, where each node can have zero or more child nodes. In Java, each tree node is an object containing its data as well as references to its child nodes. Common types of trees in Java include binary trees, in which nodes have up to two children, and n-ary trees, where nodes can have more than two children. Key operations on trees include traversal, insertion, deletion, and balancing algorithms.
Some benefits of using trees in Java include:
- Efficient searches - Trees can be searched recursively, allowing quick access to any data node.
- Flexible size - Trees can grow very deep and large as needed for data storage.
- Hierarchical ordering - Data can be sorted and maintained in the parent-child hierarchy.
- Sorting and ordering - Tree nodes can easily keep data in sorted order.
Part 3: Stack and Queue in Java: What’s the Difference?
In Java, stacks and queues provide two fundamental linear data structures. Yet, their operational methods showcase notable differences.
A stack operates under the Last In, First Out (LIFO) principle. Picture a stack of dishes: the most recently added dish is the first to be taken out. Stacks exclusively grant access to the topmost item. The primary operations for a stack involve adding data with push() and removing data with pop().
In contrast, a queue works as FIFO - First In, First Out. Imagine a line of people waiting. The first person to join will also be the first to leave. The key operations on a queue are enqueue() to insert an item and dequeue() to remove an item.
The main differences in practice are:
- Stacks allow access only to the last inserted data, while queues allow access to the first inserted data.
- Stacks are conceptually simple with only push/pop access, while queues allow insert and remove operations independent of each other.
- Stacks are useful for nested function calls or undo features; queues model real-world waiting.
Part 4: What is a Java Source File Structure?
Java utilizes a structured system to store source code in .java files with specific requirements:
- Package Statement - Contains the package name declaring what group this class belongs to. Helps avoid naming conflicts.
- Imports - Allows access to external libraries. import statements should be directly after the package statement.
- Class Declaration - Contains class name, extends if subclass implements if interfaces used.
- Class Body - Enclosed in { } brackets, contains all fields and methods.
- Comments - Can use line comments with // or block comments between /* and */
- Proper indentation and spacing - Makes code visually clear for easy reading.
Part 5: Creating a Programming Algorithm Flowchart Using EdrawMax
Flowcharts are diagrams that visualize a program’s algorithms and logic flows using symbols connected by arrows showing the order of steps. Flowcharts help programmers carefully plan program structure before coding and make algorithms easier to explain to others.
EdrawMax is a user-friendly software for creating all kinds of diagrams and charts. Creating algorithms flowcharts in EdrawMax can provide significant benefits to programmers. A key advantage is that it allows programmers to easily visualize the logical flow of their code before implementation.
Mapping out algorithms via flowcharts can often reveal potential issues with the order of steps, process bottlenecks, or branching complexity that may not be readily apparent by simply reading through the written algorithm.
Here are the steps for creating a programming algorithm flowchart using EdrawMax:
Step 1:
Launch the EdrawMax application on your computer. Click on "File" or "New" to create a new document. Alternatively, you can also switch to the “Template” category and search for “Programming Flowcharts”.
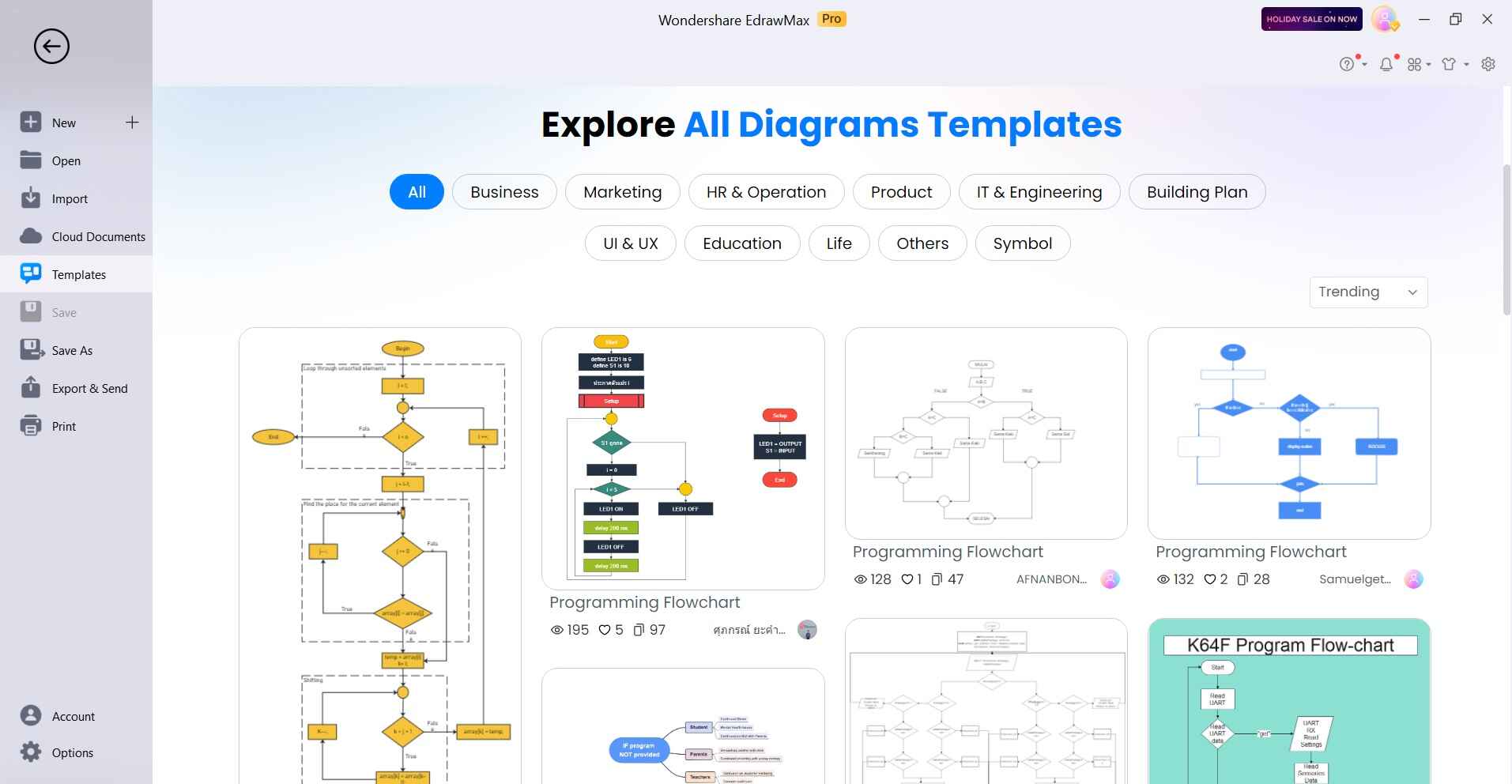
Step 2:
Drag and drop symbols from the left-hand side symbol library onto the canvas.
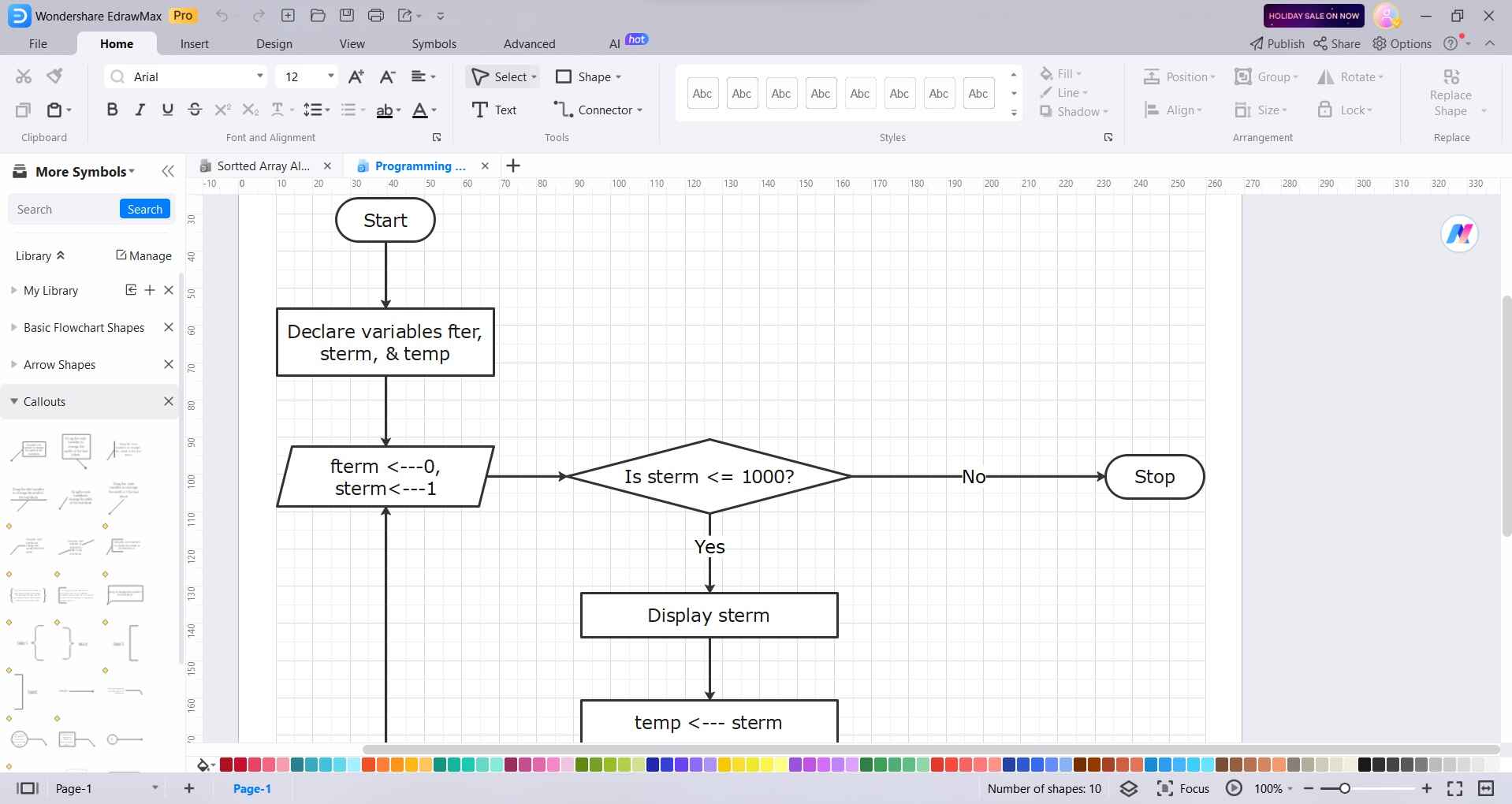
Step 3:
Double-click on the symbols to add text and labels inside them.
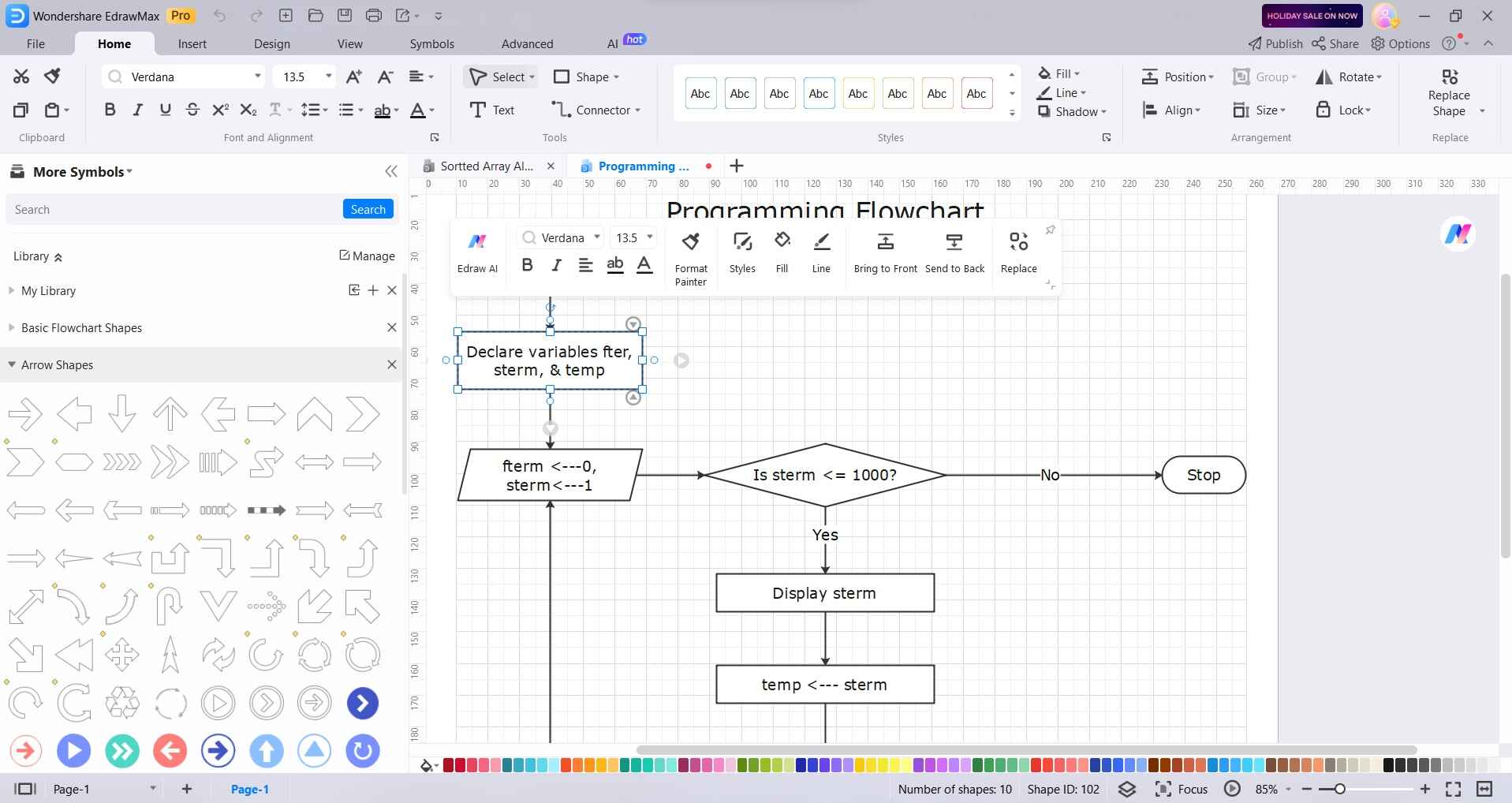
Step 4:
Use formatting options to change the color, size, and style of the symbols and connectors to enhance readability.
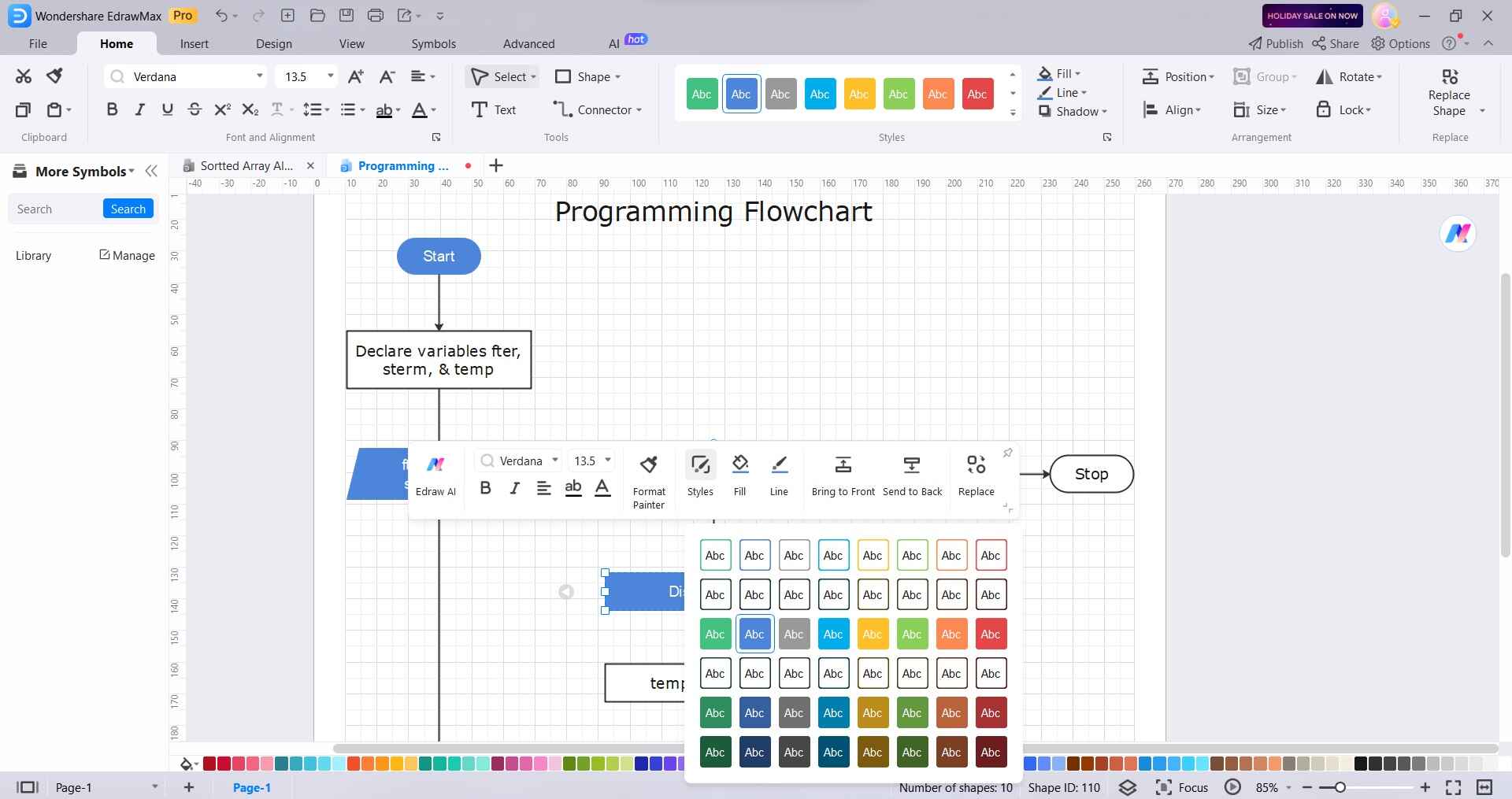
Step 5:
Once you've completed your flowchart, save your work by clicking on "File" > "Save As." Choose your preferred file format (such as .eddx, .png, .jpg, etc.) for saving and sharing the flowchart.
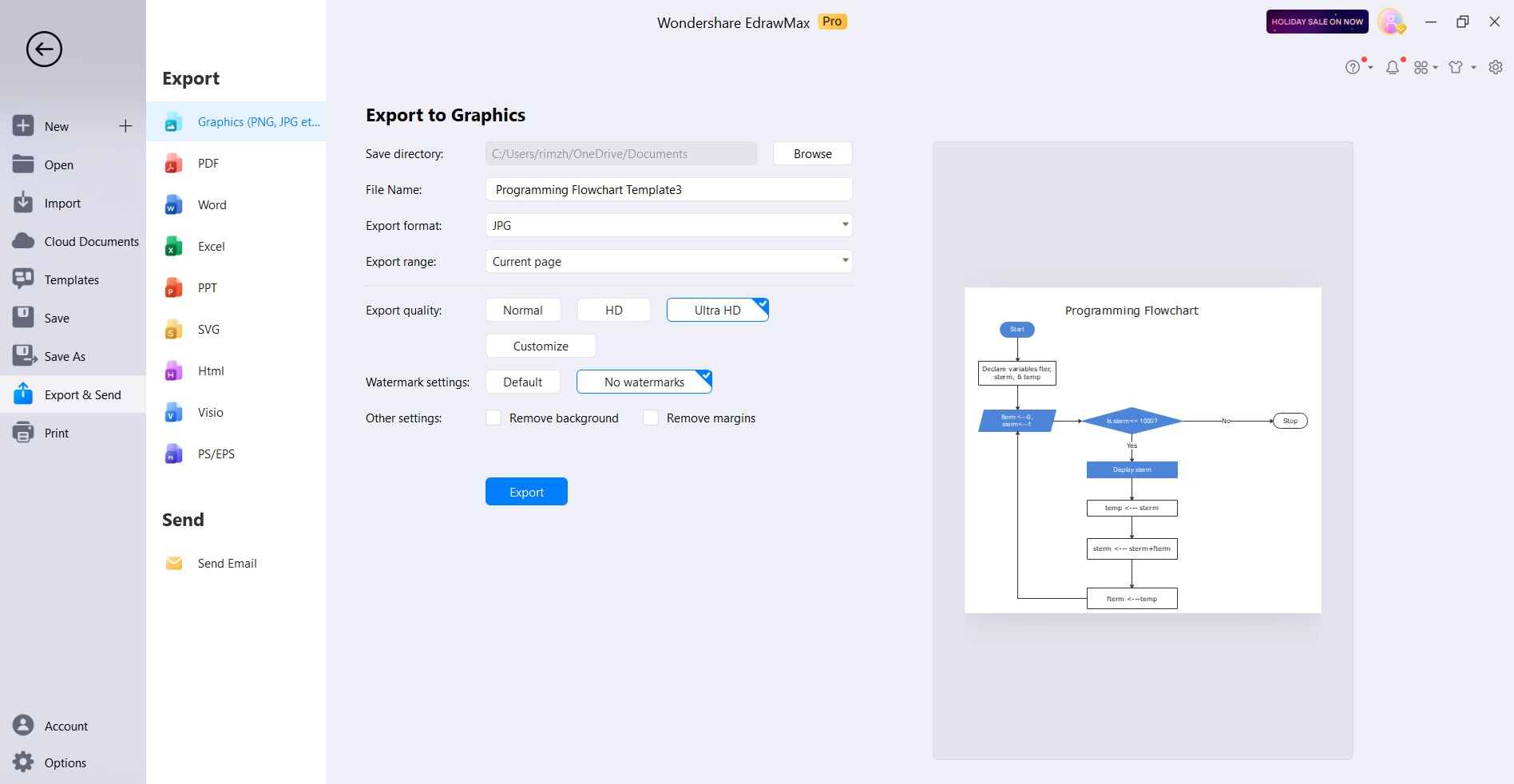
Feel free to explore additional tools and options offered by the software to create a comprehensive and visually appealing flowchart for your programming algorithm.
Conclusion
Trees in Java provide powerful hierarchical data storage and access for organizing data in Java programs. Core knowledge areas for working with trees include understanding key data structures, differences between stacks and queues, the structure of Java source file structure for implementations, and using tools like EdrawMax to map programming algorithms.
Learning efficient tree traversal algorithms, insert and delete operations, and balancing techniques allows intermediate Java developers to leverage trees for projects from web apps to complex analysis.
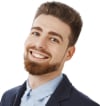