Data structures are foundational components in computer science and programming. They allow organized and efficient storage and retrieval of information.
One important data structure is the min heap, which arranges data in a complete binary tree that satisfies the heap property - each node's value is less than or equal to that of its children. Implementing a min heap in C++ allows for fast access to the minimum value, making it useful for priority queues, graph algorithms, and sorting algorithms.
In this article
Part 1: What is a Min Heap C++?
Data structures are foundational components in computer science and programming. They allow organized and efficient storage and retrieval of information.
One important data structure is the min-heap, which arranges data in a complete binary tree that satisfies the heap property - each node's value is less than or equal to that of its children. Implementing a min heap in C++ allows for fast access to the minimum value, making it useful for priority queues, graph algorithms, and sorting algorithms.
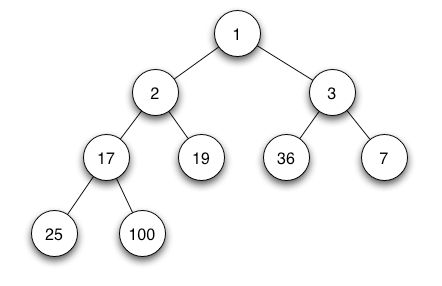
A min heap in C++ is a complete binary tree data structure that implements a priority queue to access the smallest key value quickly. It utilizes an array to represent the binary tree structure, allowing fast allocation and access.
Part 2: Steps to Build Min Heap in C++
Here are the basic steps to build a min heap data structure in C++:
- Include required header files like
and . - Create a MinHeap class with a vector to store heap elements and an integer to track size.
- Write helper functions like getParentIndex(), getLeftChildIndex(), getRightChildIndex() to navigate nodes.
- Implement the minHeapify() function to maintain the heap property by recursively swapping a node with its smallest child if necessary.
- Create the insertKey() public method to insert a new element in the next available spot and call minHeapify() on it.
- Implement the extractMin() method to remove the root element, swap it with the last element, decrement size, and call minHeapify() on the new root.
- Include other public methods like decreaseKey() as needed for priority queue functionality.
- Create driver code to test the implementation by inserting elements and extracting the minimum key.
Following these steps will result in an efficient min heap implementation in C++ using vector and helper methods to enforce the critical heap properties.
Part 3: Overview of a JavaScript Min Heap
While C++ offers excellent performance for data structures, JavaScript also supports the implementation of the min heap for web development purposes.
Key aspects of a min heap in JavaScript include:
- Stored in an Array object to represent the tree.
- Parent/child access via Math.floor((index-1)/2) and 2*index + 1.
- minHeapify() method to maintain heap structure.
- insert() handles appending items and bubbling them up.
- extractMin() returns the smallest by replacing it, bubbling the last item down.
This allows min heaps to deliver fast priority queue performance in web apps.
Part 4: Pros and Cons of Using Min Heap
There are several benefits as well as some disadvantages or challenges to using a min heap data structure:
Pros:
- Fast constant time extraction of minimum value.
- An automatically sorted structure makes retrievals easy.
- Useful for algorithms like Dijkstra's shortest path.
- Flexible priority queue implementation.
- Efficiently utilize available storage space.
Cons:
- The slower linear time complexity for inserts compared to a hash table.
- Implementing insertions/deletions with swapping can be complex.
- No quick access to arbitrary elements like an array.
- Heap sort has slower O(nlogn) complexity than quick sort's average case.
Overall, min heaps provide excellent efficiency guarantees for access to the smallest element. This makes them ideal for priority queues and graph algorithms. But lookup and search are slower than other structures.
Part 5: Creating a Programming Flowchart Using EdrawMax
An important part of designing and visualizing an efficient min heap C++ structure is planning the programming flow with flowcharts. EdrawMax is an invaluable tool for quickly creating programming flowcharts.
With an intuitive drag-and-drop interface and abundant built-in symbols for things like loops, functions, decisions, inputs/outputs, and more, EdrawMax simplifies flowchart creation.
This clear visualization of the programming logic makes implementations much easier. EdrawMax integrates seamlessly with Microsoft Word as well for reports.
Some key benefits of EdrawMax for programming include:
- Quick editing and rearrangement of flowchart components.
- Powerful styling like color coding execution paths.
- Optimization of program structure.
- Easy sharing of charts with team members.
By facilitating the planning of code logic, EdrawMax noticeably improves programming efficiency.
Here are the steps for creating a simple programming flowchart using EdrawMax:
Step 1:
Launch the EdrawMax software on your computer. Look for the "Flowchart" category or search for "Flowchart" in the template gallery. Select a template that fits your needs or start with a blank canvas.
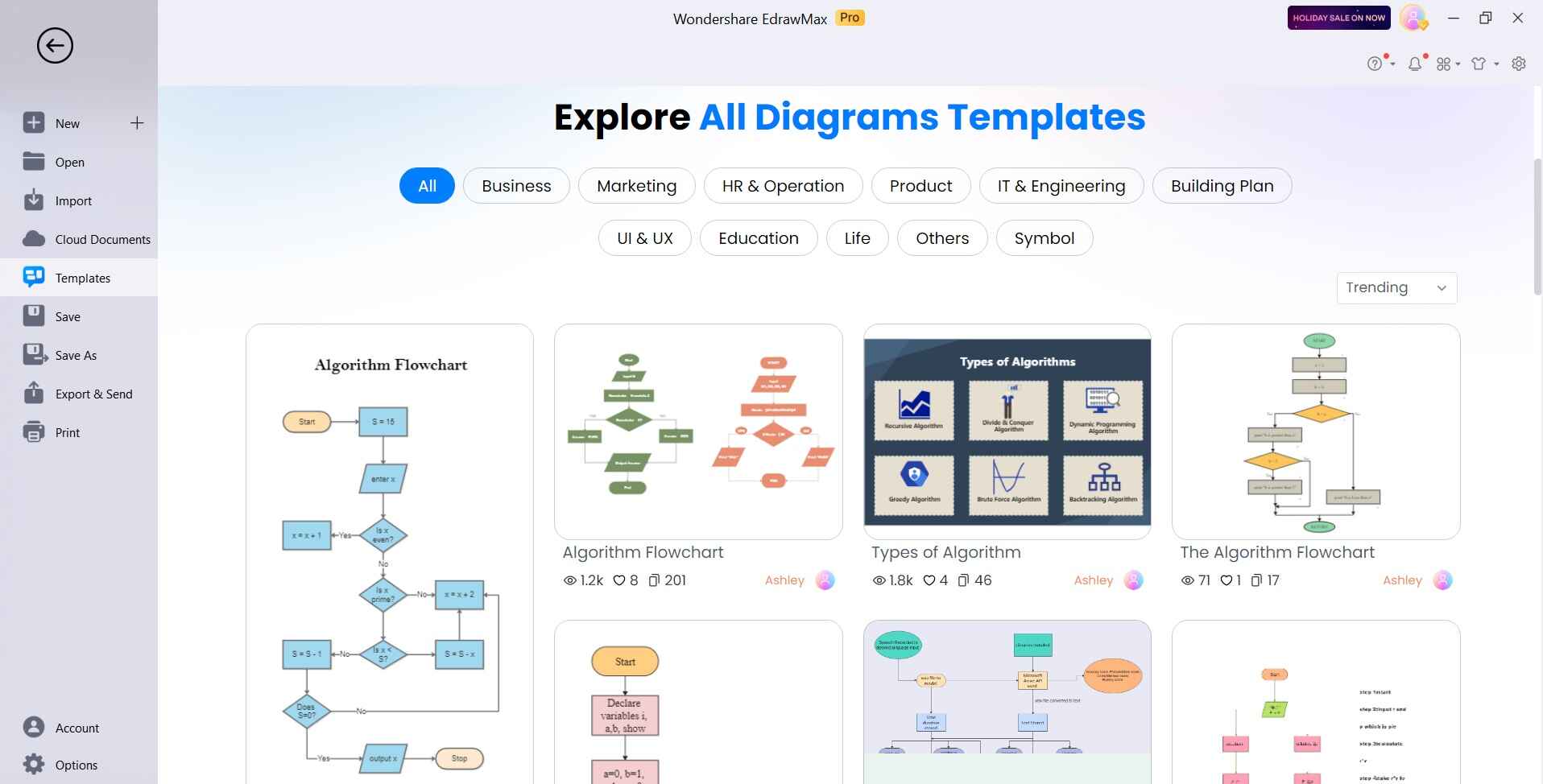
Step 2:
Drag and drop shapes from the library onto the canvas.
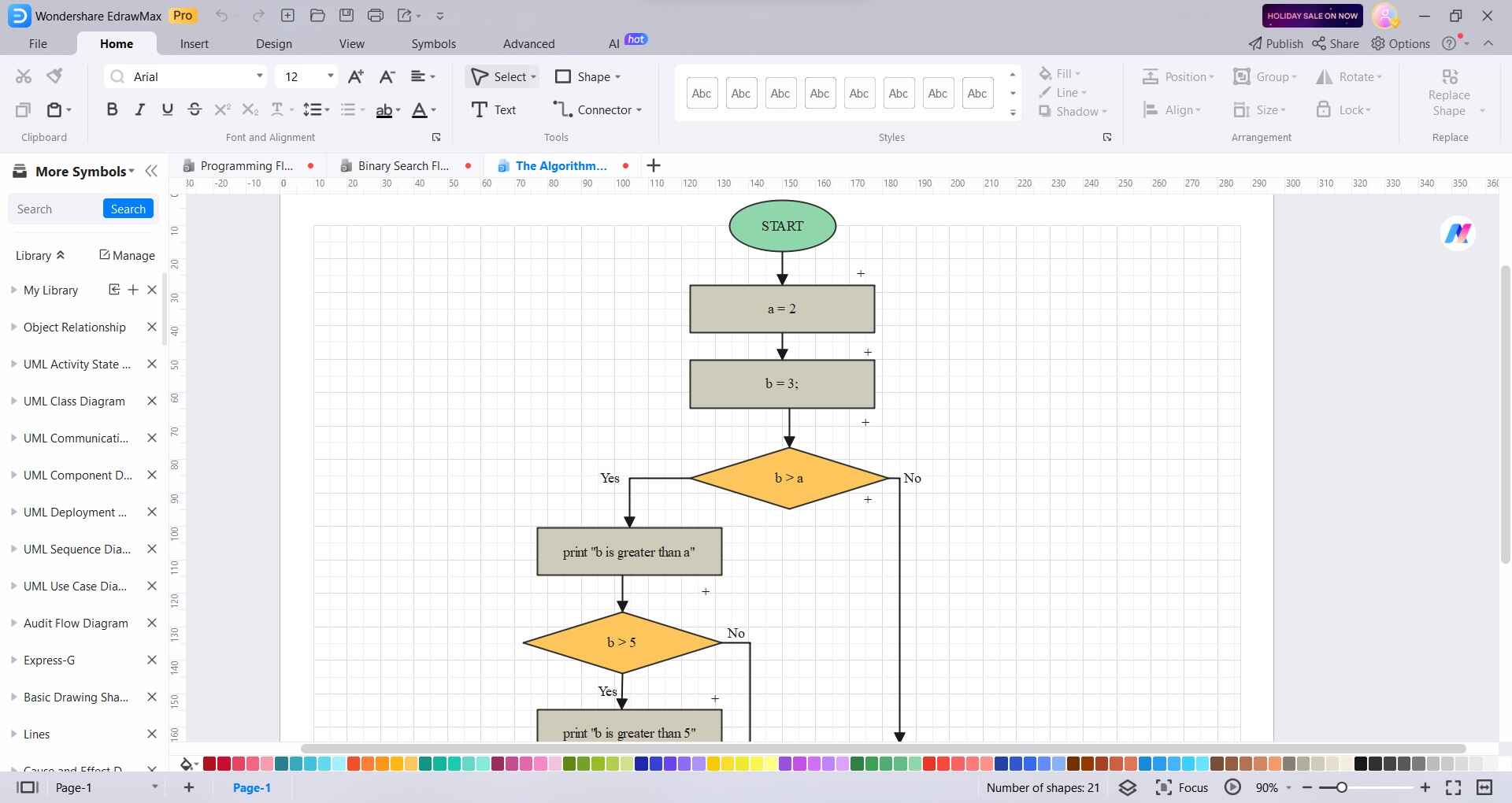
Step 3:
Use connectors or arrows to link the shapes in the sequence you want for your program's flow.
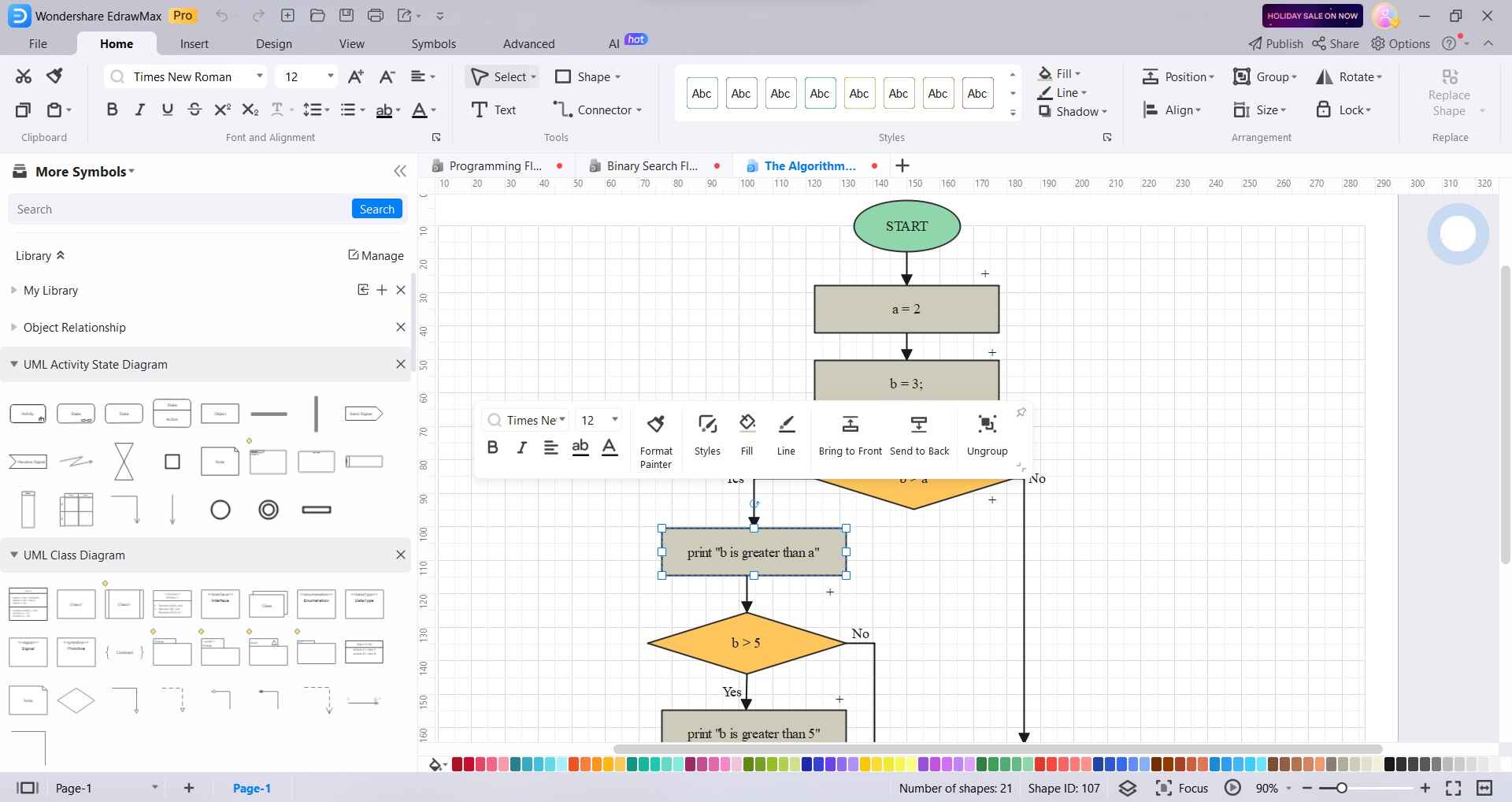
Step 4:
You can also change the colors, fonts, sizes, and other attributes to make your flowchart more visually appealing and understandable.
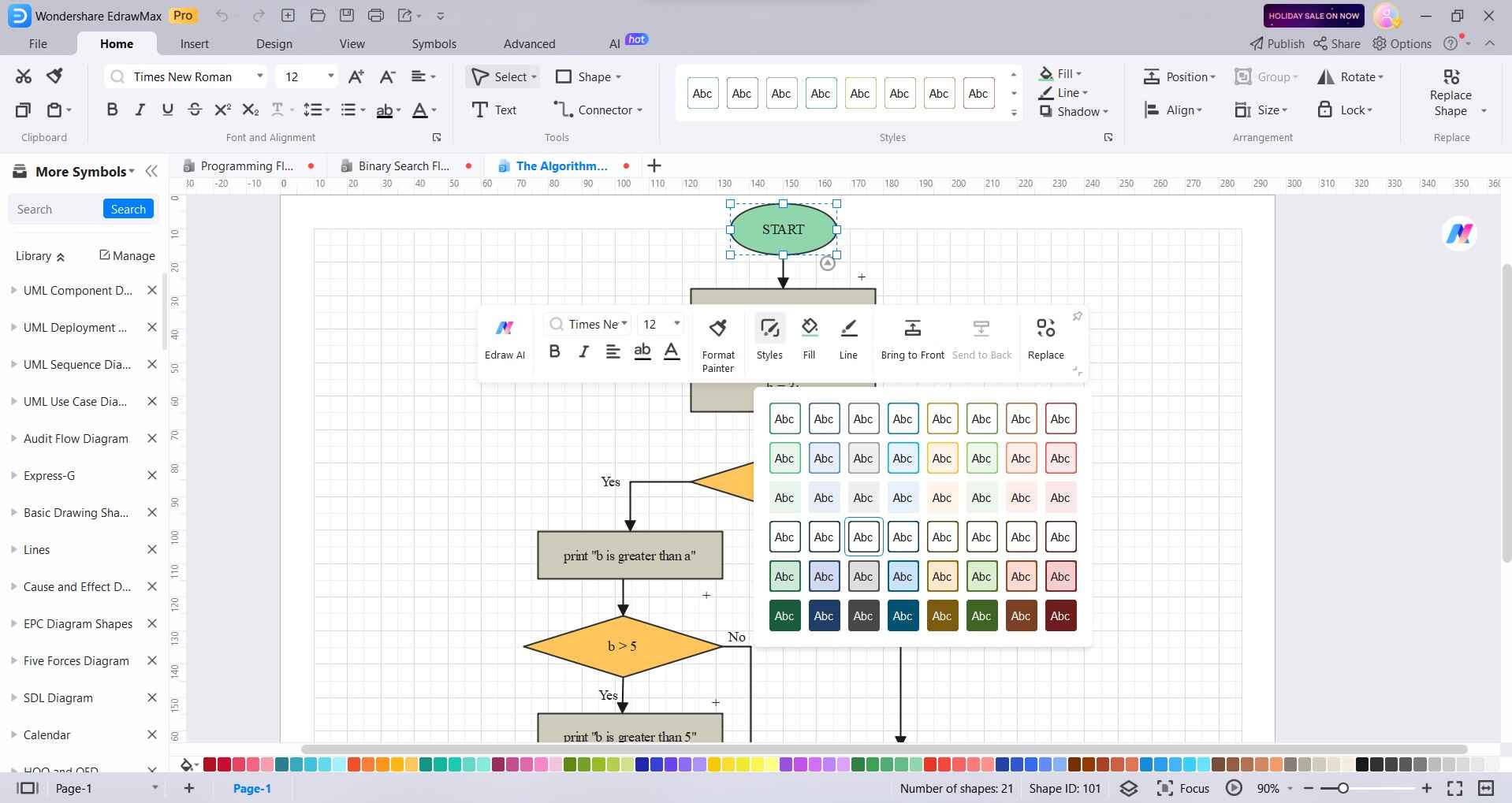
Step 5:
Once completed, save your flowchart in your preferred format (e.g., .eddx, .png, .jpg, .pdf) to your computer for later editing or sharing purposes.
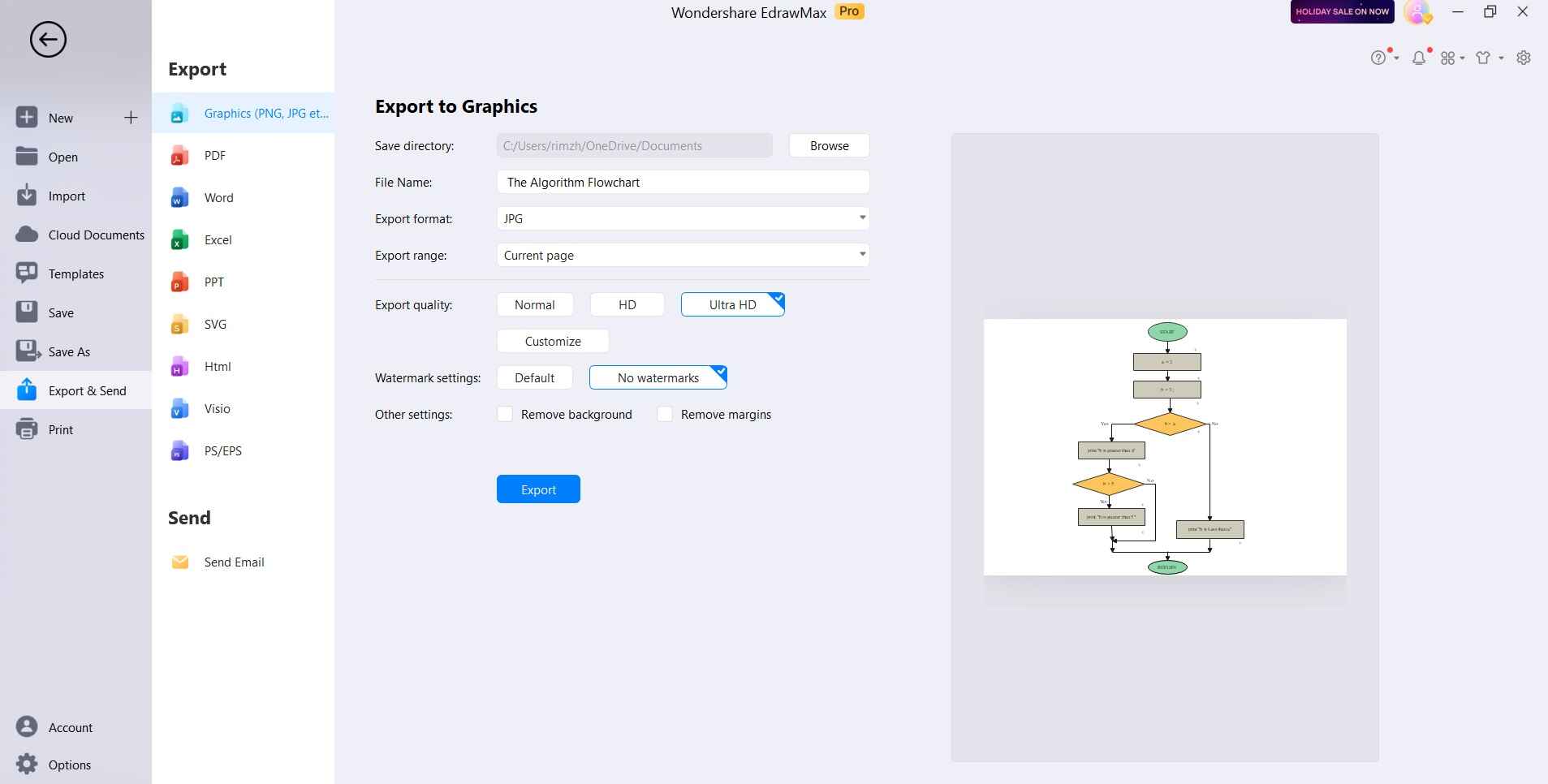
EdrawMax offers a user-friendly interface with drag-and-drop functionality, making it relatively easy to create flowcharts.
Conclusion
The min heap is a versatile and effective data structure for fast access to minimum values and efficient priority queue functionality. By organizing data in a complete binary tree adhering to the heap property, min values can be retrieved in constant time.
Overall, min heaps are indispensable when optimizing for fast minimal value retrieval or priority queues for algorithms and data-driven applications.