Linked lists are one of the fundamental data structures used in programming. They provide a way to store linear collections of data and allow efficient insertions and deletions. Unlike arrays, linked lists are dynamic in size and don't require contiguous memory allocation. In C programming, linked lists can be implemented using pointers and dynamic memory allocation.
In this article, we will focus on implementing a singly linked list in C. A singly linked list contains nodes that store data and a pointer to the next node in the list.
In this article
Part 1: What is a Singly Linked List Program?
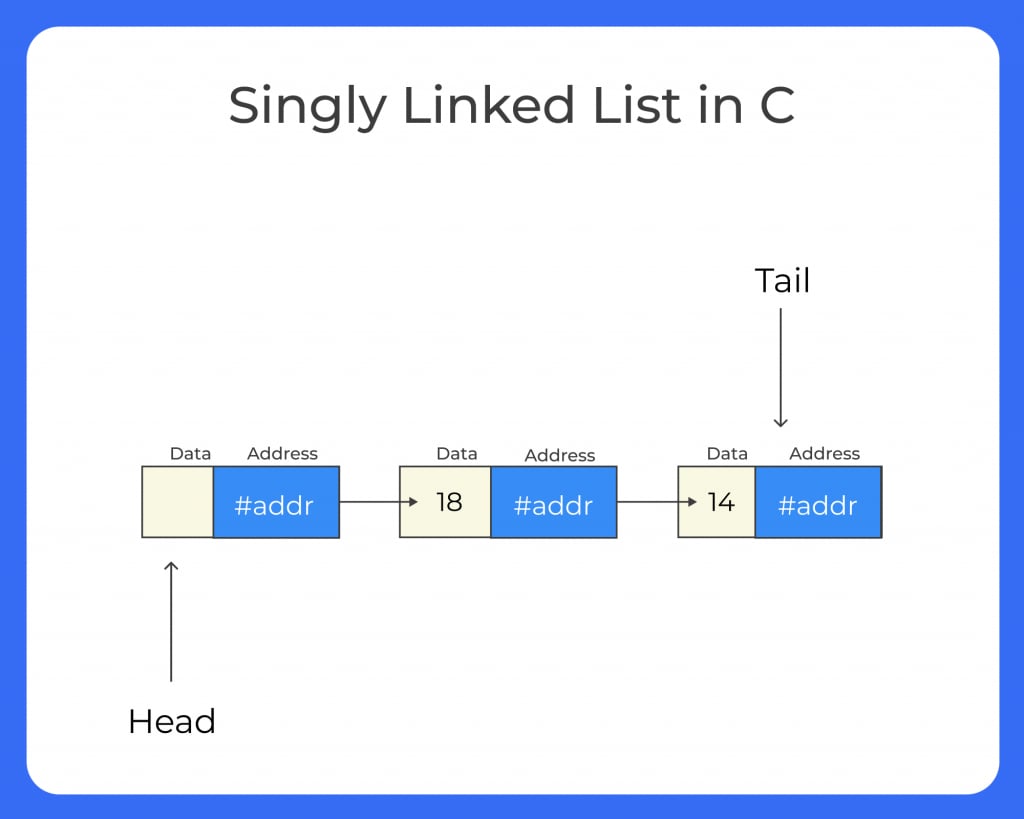
A singly linked list is a linear data structure that consists of nodes where each node contains data and a pointer to the next node in the list. It can be visualized as a chain of nodes where each node has the address of the next node.
Some key properties of a singly linked list program are:
- It consists of nodes where each node stores data and a pointer to the next node.
- The first node is called the head. The last node points to NULL indicating the end of the list.
- Insertion and deletion of nodes can be done efficiently without shifting elements as in arrays.
- It does not require contiguous memory locations, unlike arrays. Memory is allocated dynamically to nodes.
- Nodes can be inserted and removed from any position in the list by just modifying the address pointer.
By implementing a linked list program in C, we can understand core concepts like dynamic memory allocation, pointers, and pointer manipulation which are key to many data structures and algorithms.
Part 2: Overview of Singly Linked List Program in C
A Singly Linked List program in C is like a chain of connected blocks, where each block holds some information and points to the next block. It's handy for managing data because you can easily add, remove, or find information in this chain.
This program helps you learn how to organize and work with data efficiently, kind of like arranging things in a line to access them quickly when needed.
Part 3: Steps for Implementing Singly List Program in C with All Operations
Here are the steps for implementing a singly linked list program in C with all operations in simple terms:
- Node Definition: Create a structure defining a node with data and a pointer to the next node.
- Initialization: Set up functions to initialize an empty list and handle the head pointer.
- Insertion: Implement functions to add nodes at the beginning, end, or a specific position in the list.
- Deletion: Develop methods to remove nodes from the beginning, end, or at a specific position in the list.
- Traversal: Create a function to traverse the list, displaying or performing operations on each node.
- Search: Design a function to find a specific element in the list based on its value.
- Update: Implement a method to modify the value of a node at a given position.
- Counting Nodes: Develop a function to count the total number of nodes in the list.
- Cleanup: Ensure to have a function that deallocates memory, freeing all nodes in the list.
- Testing: Finally, thoroughly test each operation to ensure the correctness and proper functionality of the singly linked list program.
Part 4: Key Takeaways of a Singly List Program in C With Output
Let us summarize some key takeaways from implementing a singly linked list program in C:
- Linked lists provide efficient insertion and deletion compared to arrays. Memory is allocated dynamically.
- Each node stores data and the address of the next node. This linking is done using self-referential struct pointers.
- All operations work by modifying the next pointers - insertions, deletions, traversals.
- Head and tail pointers are used to keep track of the start and end of the list.
- Dynamic memory allocation (
malloc
,free
) is used to create and destroy nodes.
Part 5: Creating a Programming Flowchart Using EdrawMax
An important part of building programs is planning the programming logic visually before coding. The best way to do this is to create flowcharts showing the key steps.
EdrawMax is a useful diagramming and flowchart software that can help in planning complex programs through flowcharts.
Here is a sample flowchart created in EdrawMax for a linked list program:
Step 1:
Launch the EdrawMax application on your computer. Choose "Flowchart" or a specific flowchart template from the available options in the template gallery.
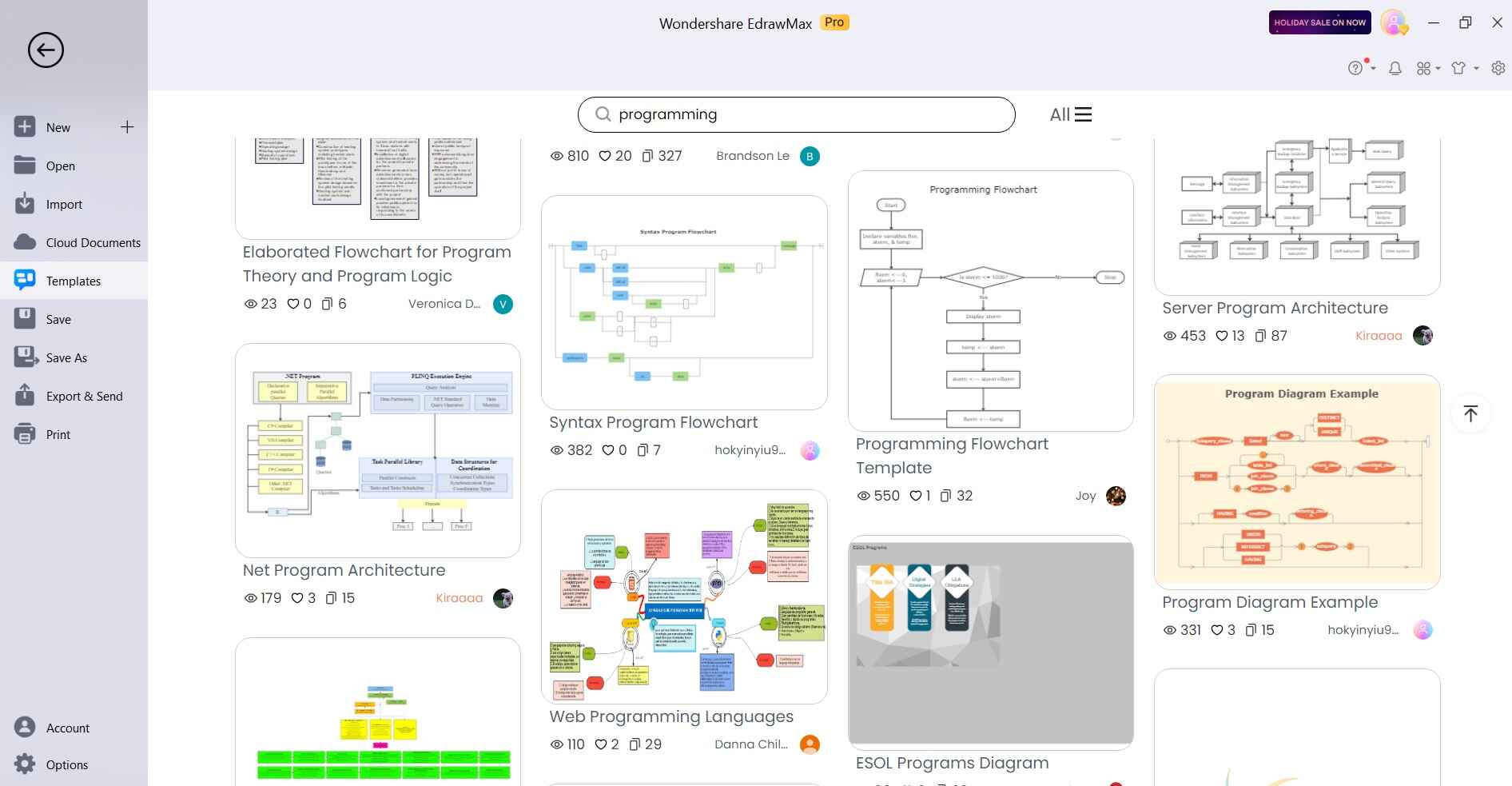
Step 2:
Drag and drop the required symbols from the symbol library onto the canvas.
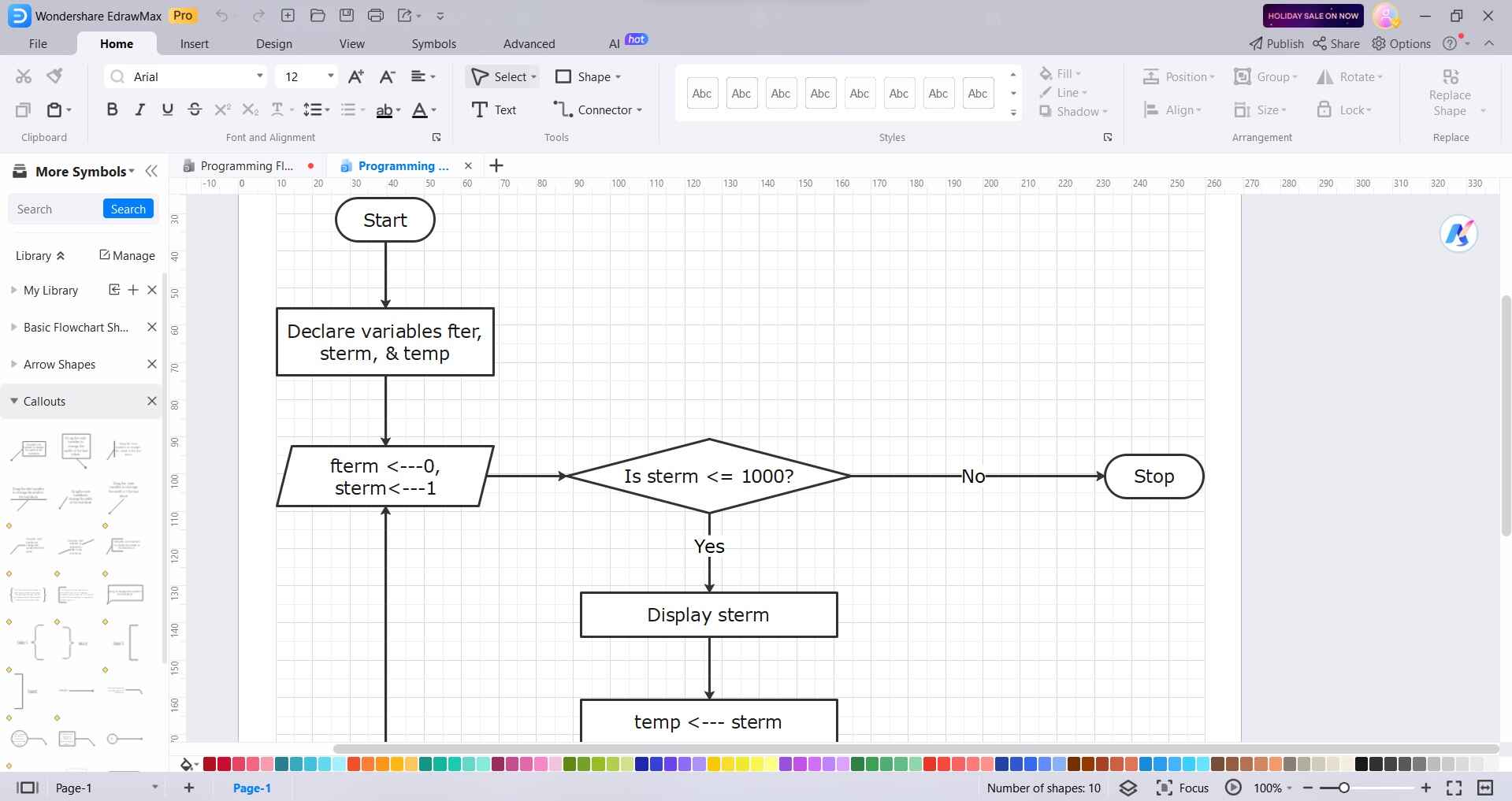
Step 3:
Use arrows or connectors to link the symbols and create a flow.
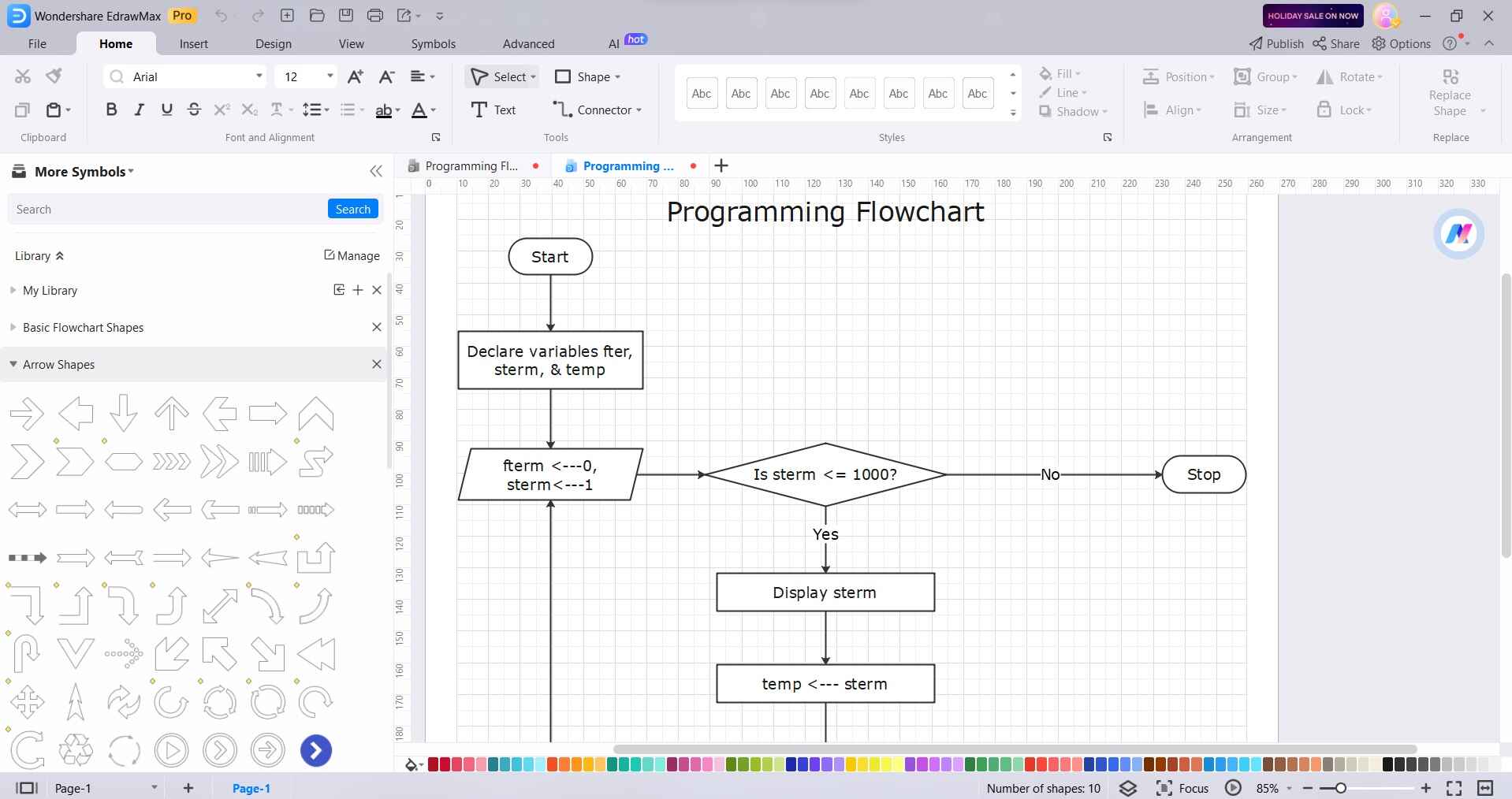
Step 4:
Customize the flowchart by adjusting the size, colors, and styles of the symbols, text, and connectors to make it clear and visually appealing.
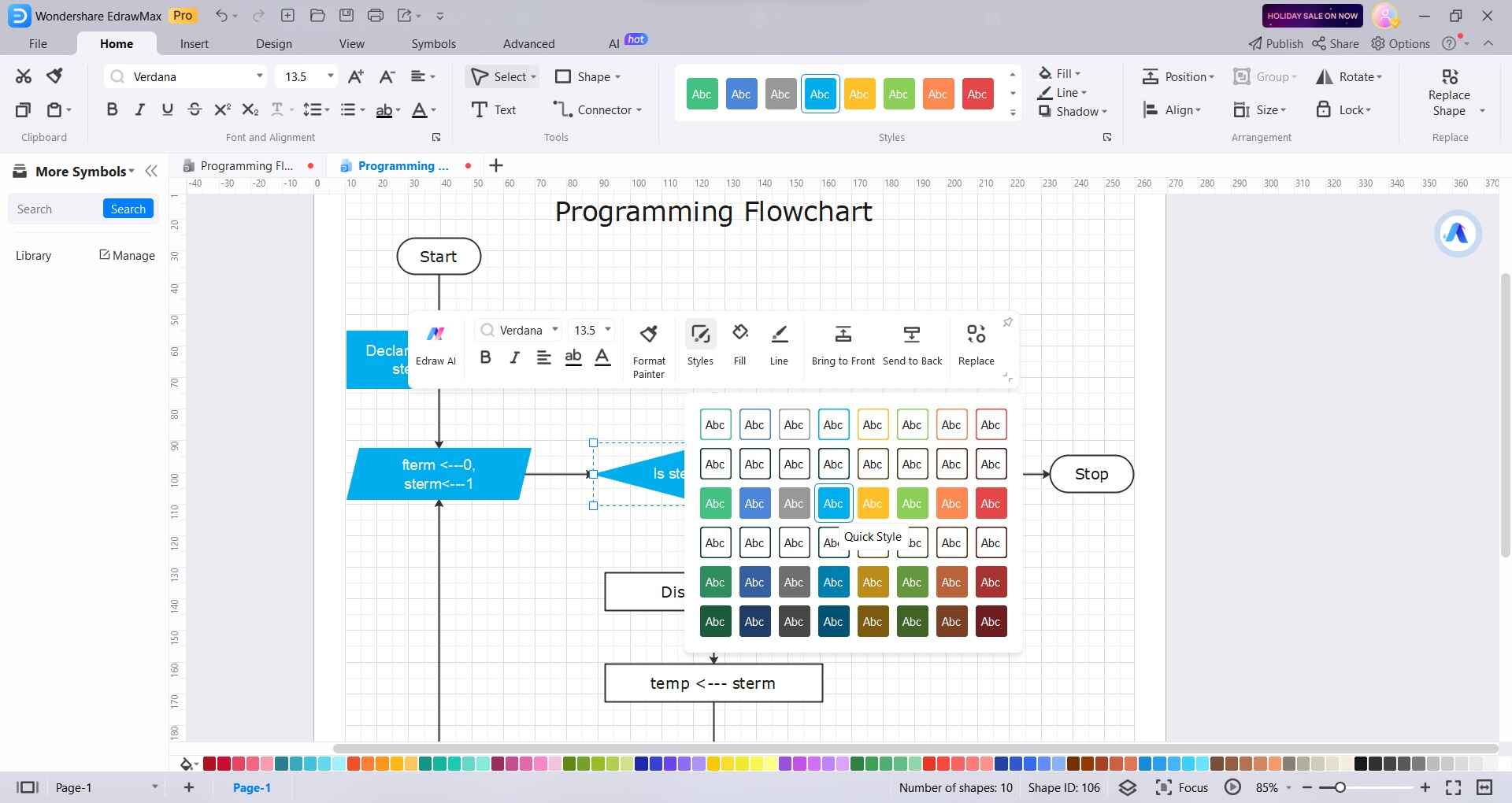
Step 5:
Once completed, save your flowchart in the desired format (e.g., PNG, JPEG, PDF) or directly share it from EdrawMax.
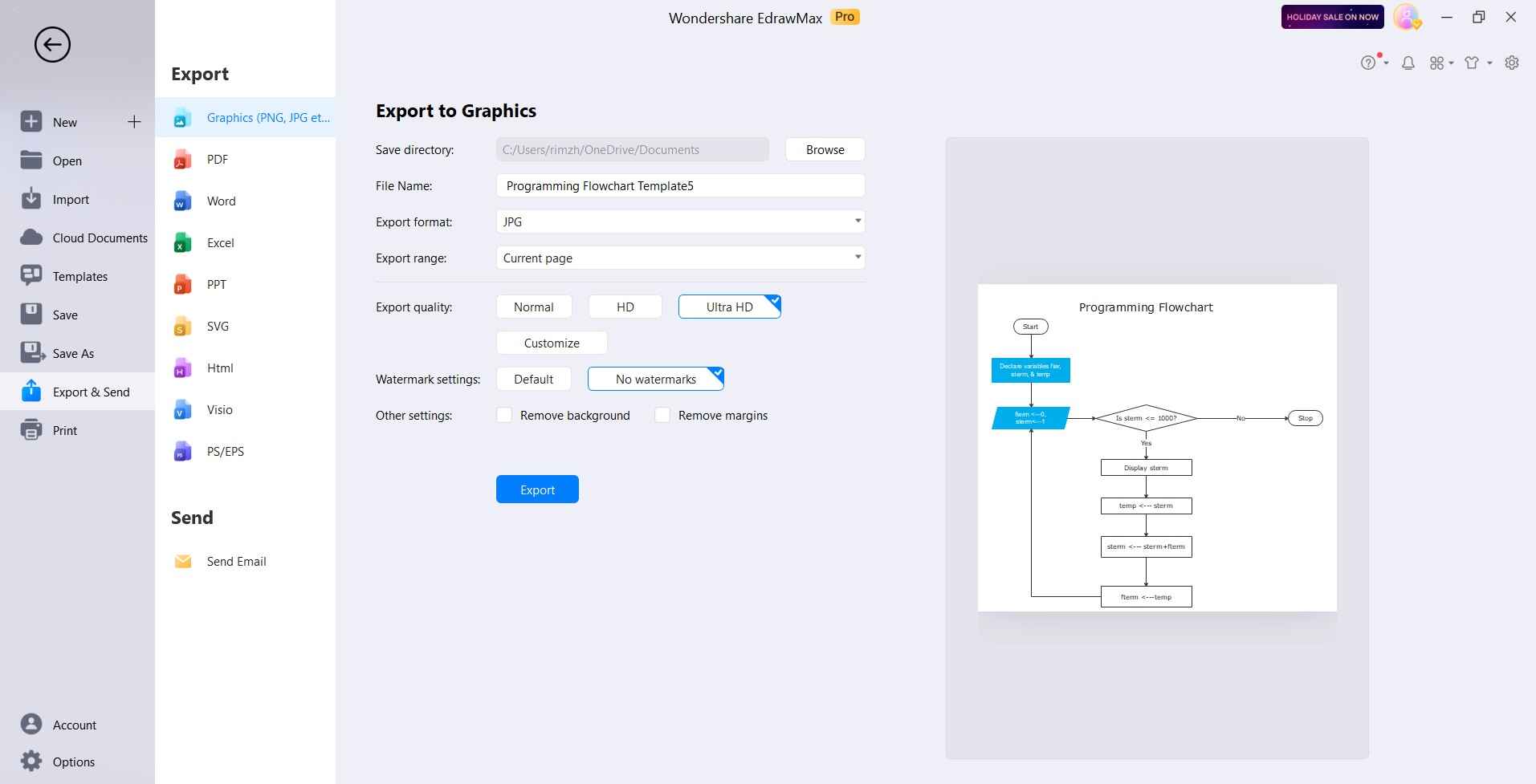
Using a tool like EdrawMax improves planning and documentation for complex programs. The visual representation through flowcharts enhances understanding of the logic and reduces errors.
Conclusion
Linked lists are a key data structure that every C programmer should know. In this article, we went over how to build a complete singly linked list program in C implementing basic operations like insertion, deletion, and traversal.