Linked lists are one of the fundamental data structures used in programming. They provide a flexible, dynamic way to store, organize and access data in a non-contiguous manner. Mastering linked lists in C++ is key for any aspiring programmer, as they offer efficiency and versatility across many use cases.
In this comprehensive guide, we will cover the ins and outs of implementing linked lists in C++, best practices for writing optimized code, and examples of common usage patterns.
In this article
Part 1: What is a Linked List in C++ Program?
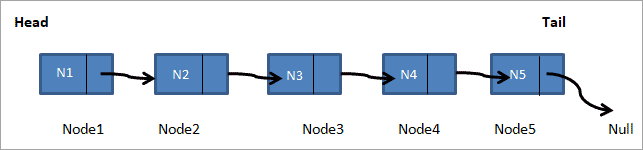
A linked list in C++ is a linear data structure that stores data elements called nodes. Each node contains two parts - the data itself and a pointer that refers to the memory address of the next node in the list.
Part 2: Types of Linked Lists in C++
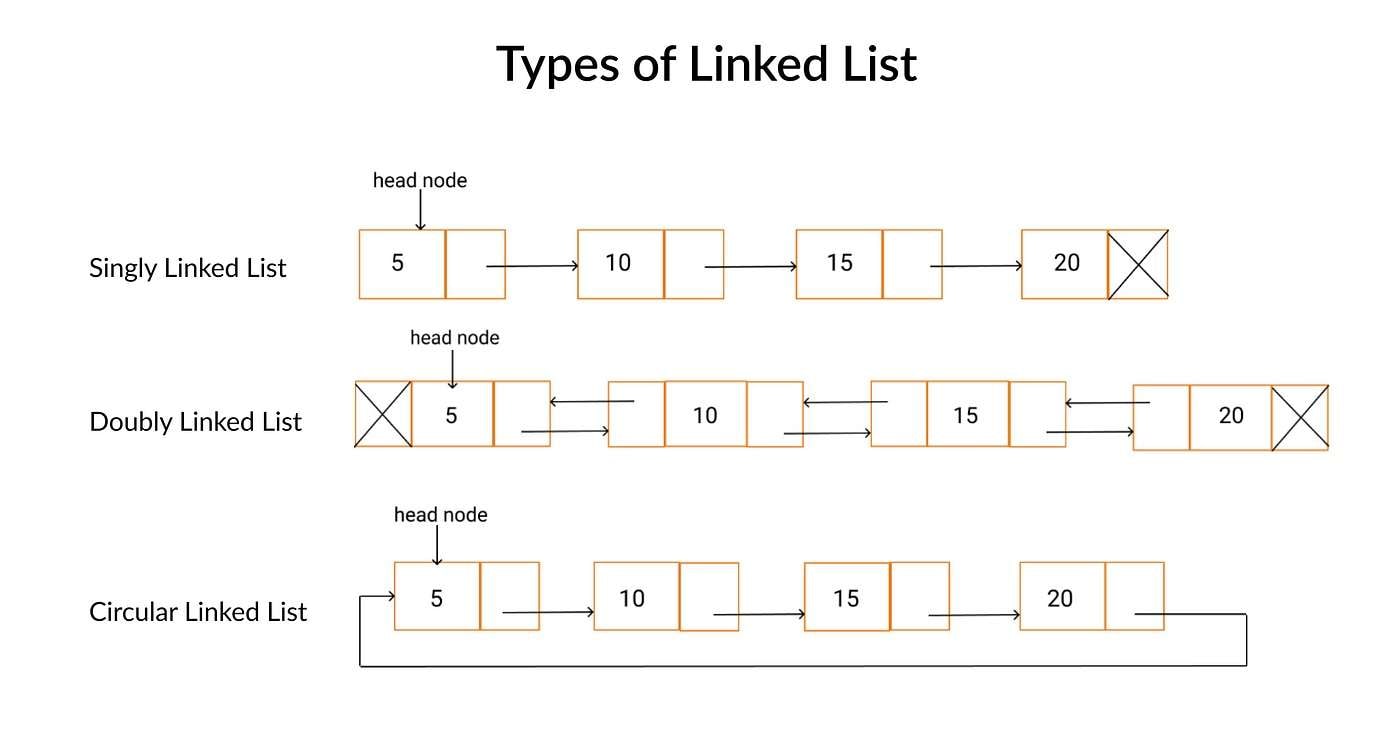
There are three common types of linked list implementations in C++ - singly linked lists, doubly linked lists, and circular linked lists.
A singly linked list contains nodes that store a data element and a pointer to the next node. This enables moving through the list in a singular direction. In a doubly linked list, each node also includes another pointer referring back to the previous node, enabling bidirectional traversal. Lastly, in a circular linked list, the “tail” refers back to the first node, forming a continuous loop.
The type of linked list to use depends on the specific requirements of the application. Singly-linked lists strike a balance between simplicity of implementation and functionality, while doubly-linked lists enable full backward and forward traversal. Circular linked lists are best suited for scenarios requiring traversal in a loop.
Part 3: Steps for Implementing a Linked List C++ Code
Follow these key steps when writing a linked list in C++ from scratch:
- Define a Node Structure with Data and Pointer Members: The Node class should contain the data to store and a pointer to refer to the next Node. For doubly linked lists, an additional pointer to the previous node is required.
- Create the Linked List Class Interface: The class encapsulating the linked list requires functions like addNode(), deleteNode(), printList(), etc. The class should hide internal complexity from outer code.
- Implement Member Functions: Functions to add and remove nodes should handle the allocation/deallocation of nodes dynamically using new and delete operators. The print function can traverse node pointers printing data.
- Instantiate List Objects: Create Linked List instances in the main() function or other parts of the code by declaring List objects and accessing member functions to manipulate the list.
Following these steps cleanly separates the interface from implementation and enables reusable, readable code.
Part 4: Things to Consider When Writing a Linked List C++ Program
Here are some best practices to optimize your linked list code in C++:
- Use Smart Pointers like unique_ptr over raw pointers for automatic memory management of nodes.
- Implement boundary checks and null checks to avoid crashes.
- Make functions modular by splitting complex tasks into helpers.
- Document functions clearly on expected inputs and return values.
- Use templates for implementing generic, reusable linked lists.
- Avoid memory leaks by diligently cleaning up old nodes.
- Run debugging checks like printing the list to validate correctness.
Carefully applying these tips will lead to robust, production-grade linked list implementations.
Part 5: Creating a Programming Algorithm Flowchart Using EdrawMax
EdrawMax stands as top-notch diagramming software, streamlining the creation of flowcharts, UML diagrams, mind maps, and beyond. With its intuitive drag-and-drop interface and extensive libraries of symbols, crafting programming algorithms becomes remarkably simple, empowering users at every skill level.
Here are the benefits of using EdrawMax for linked list algorithm flowcharts:
- Intuitive Drag-and-Drop Symbols - EdrawMax contains thousands of readymade vector symbols for every flowchart element - including processing steps, input/output, data, and connectors. These can be easily dragged onto the canvas.
- Powerful Customization Tools - Symbols, lines, and charts are completely customizable with styling tools for color, size, fonts, and more.
- Auto Connect Lines - Smart lines auto-connect symbols as they snap into place, auto-formatting for neat diagrams.
- Export as Image or PDF - Flowcharts are sharable by exporting to universal file formats like JPG, PNG, PDF, etc.
By boosting productivity and adding professional polish, EdrawMax becomes an invaluable aid when designing coding algorithms involving complex data structures like linked lists.
Here are the steps for creating a simple programming flowchart using EdrawMax:
Step 1: Launch the EdrawMax software on your device. Navigate to the "Flowchart" category or use the search bar to find flowchart templates. Select a suitable template to start with.
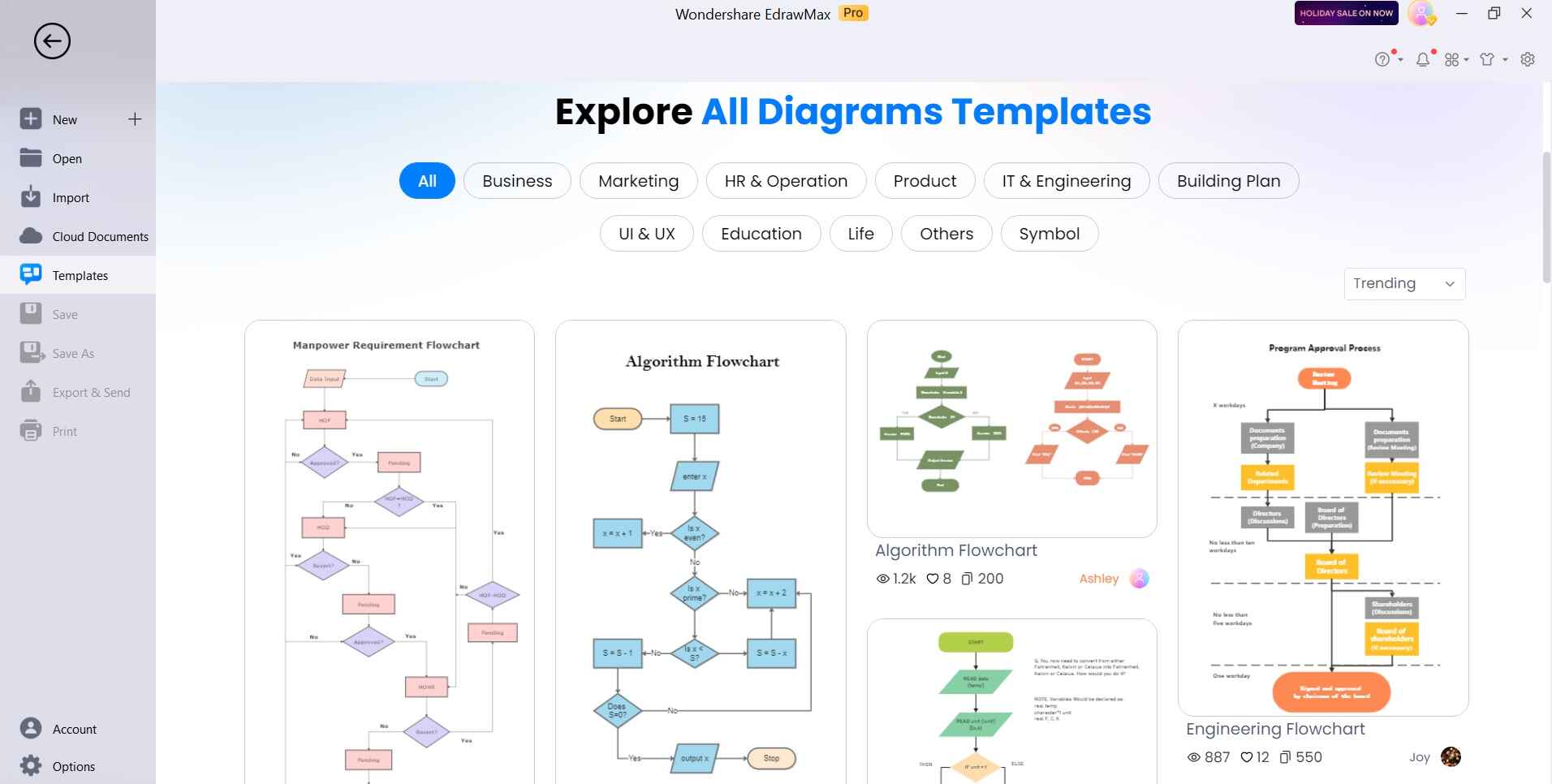
Step 2: Drag and drop the required symbols from the symbol library onto the canvas. Symbols represent actions, decisions, inputs, outputs, etc.
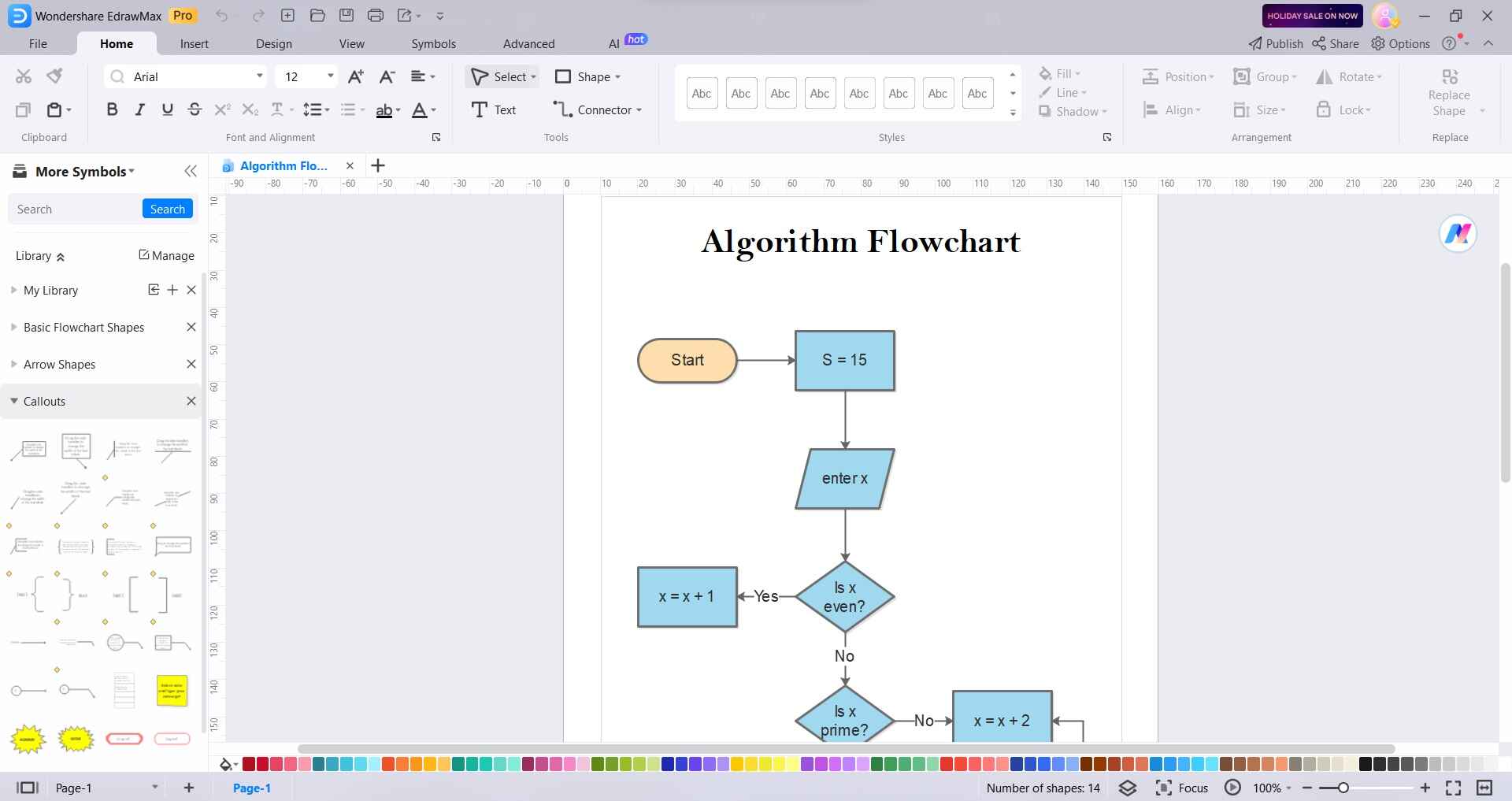
Step 3: Double-click on the symbols to add text or labels representing specific actions.
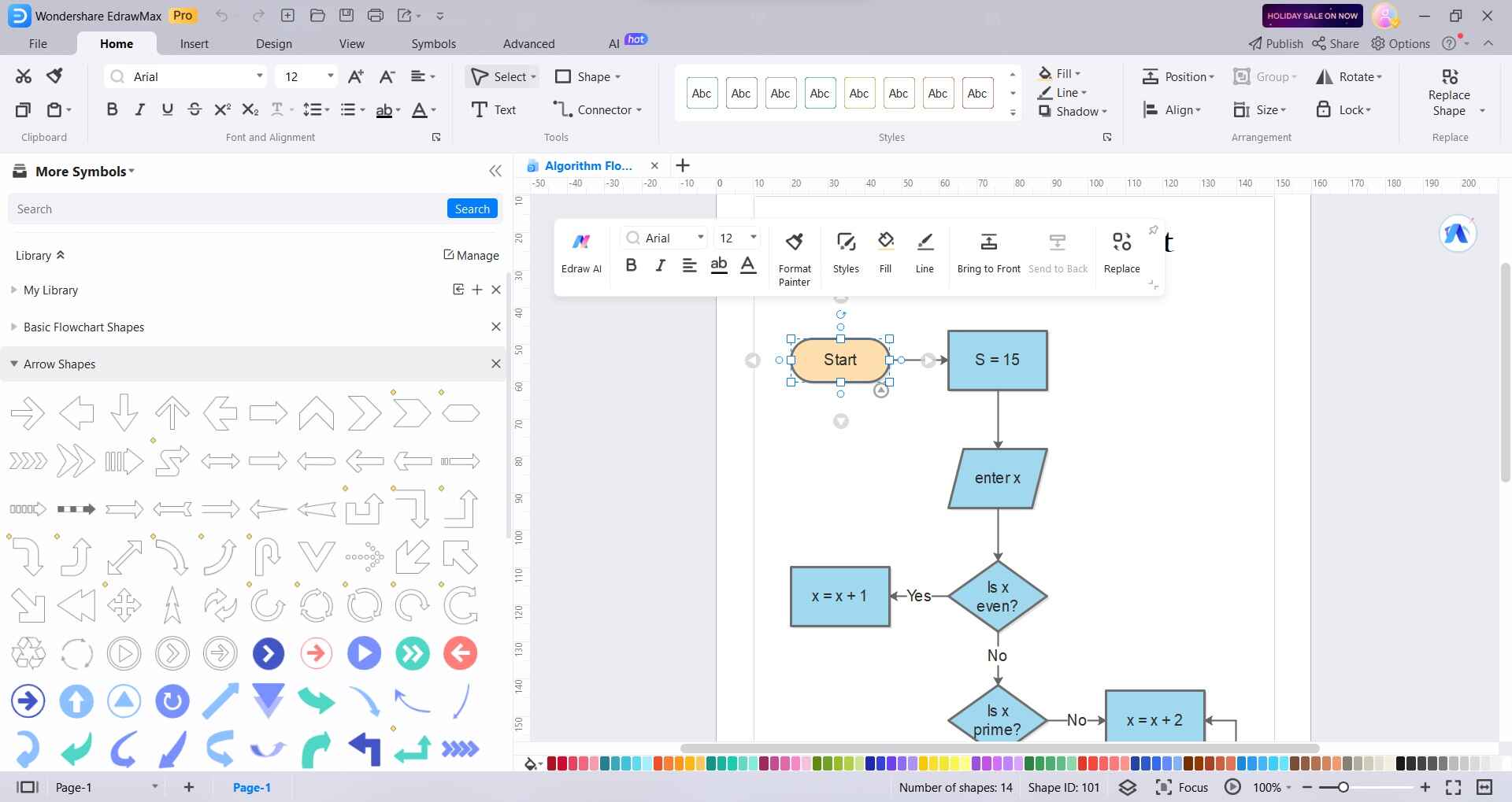
Step 4: Modify the appearance of symbols, lines, and text by changing colors, fonts, sizes, etc.
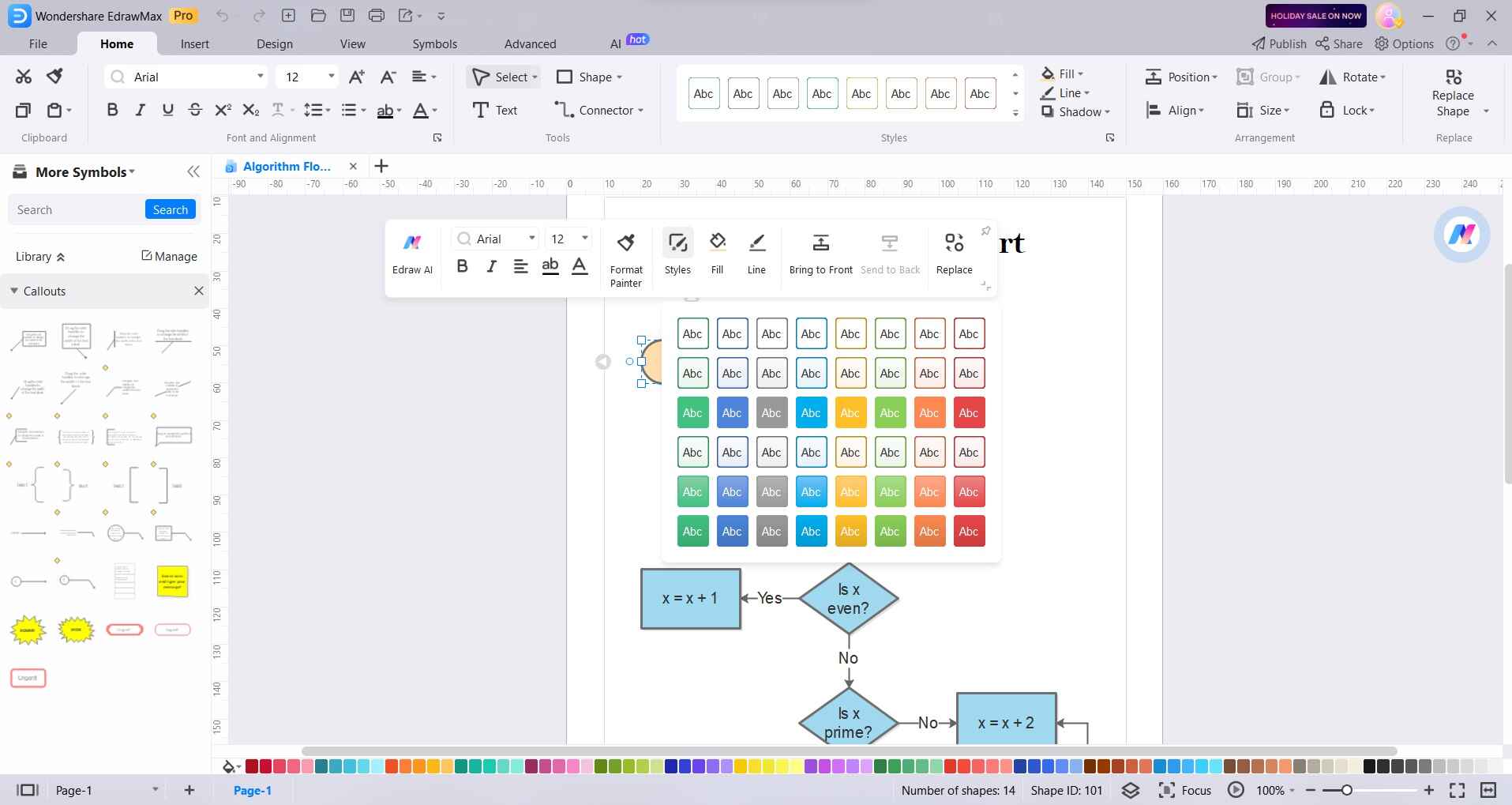
Step 5: Save your flowchart project in EdrawMax format or export it in a suitable format (PNG, JPG, PDF, etc.)
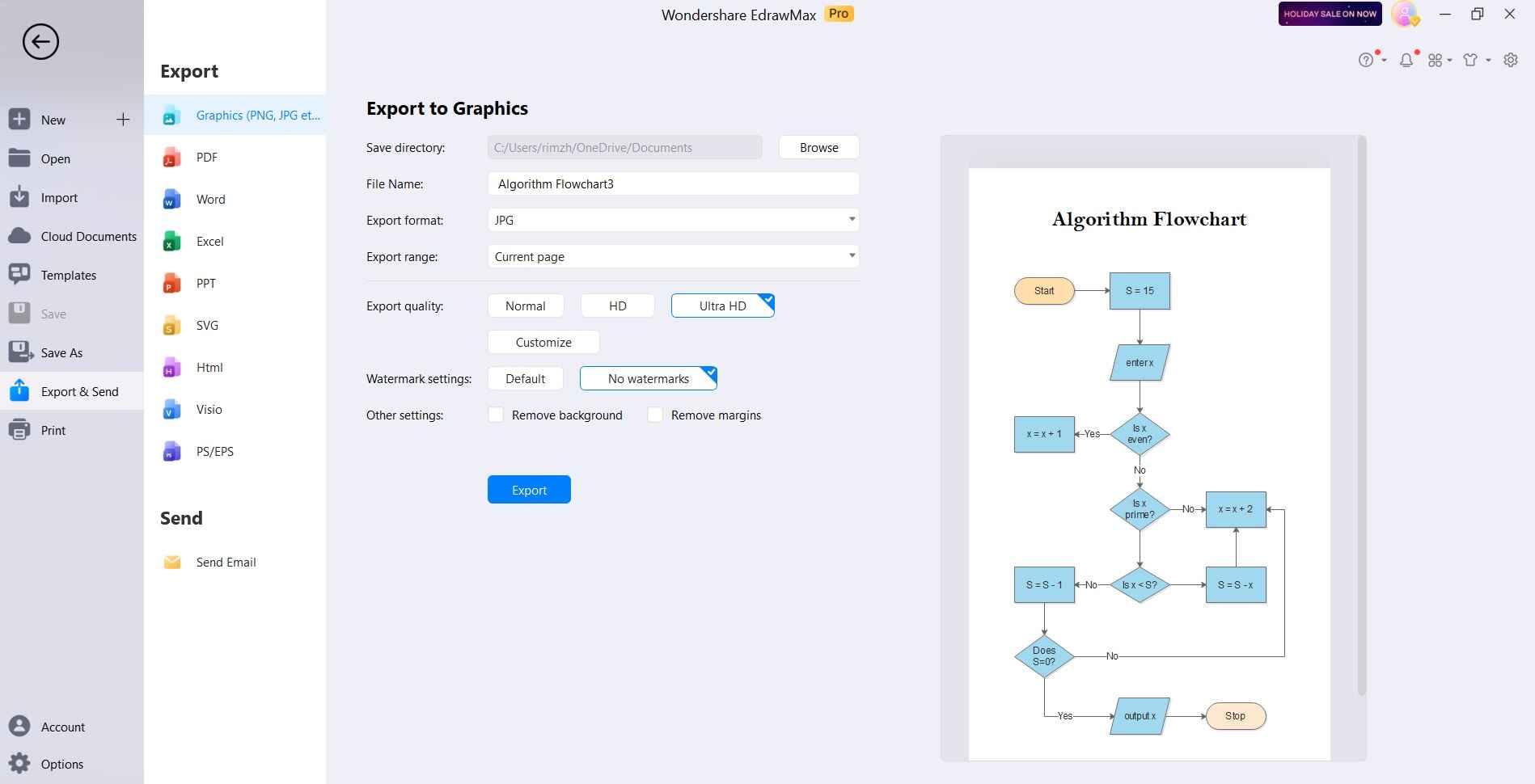
By following these steps, you can easily create a simple programming flowchart using EdrawMax, enabling you to visually map out your program's logic and structure.
Conclusion
Linked lists are a versatile data structure with nuanced implementation in C++. Learning to apply correct techniques for memory management, modularity, exception handling and testing is key to harnessing their power efficiently. Tools like EdrawMax also streamline diagramming complex algorithms during development.
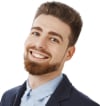