Linked lists are one of the fundamental data structures used in programming. They provide a linear collection of data elements where each element points to the next element in the list. Linked lists are dynamic, flexible, and efficient for insertion and deletion operations.
In this article, we will learn how to create a simple linked list program in C from scratch by following a step-by-step implementation.
In this article
Part 1: What is a Linked List Program in C?
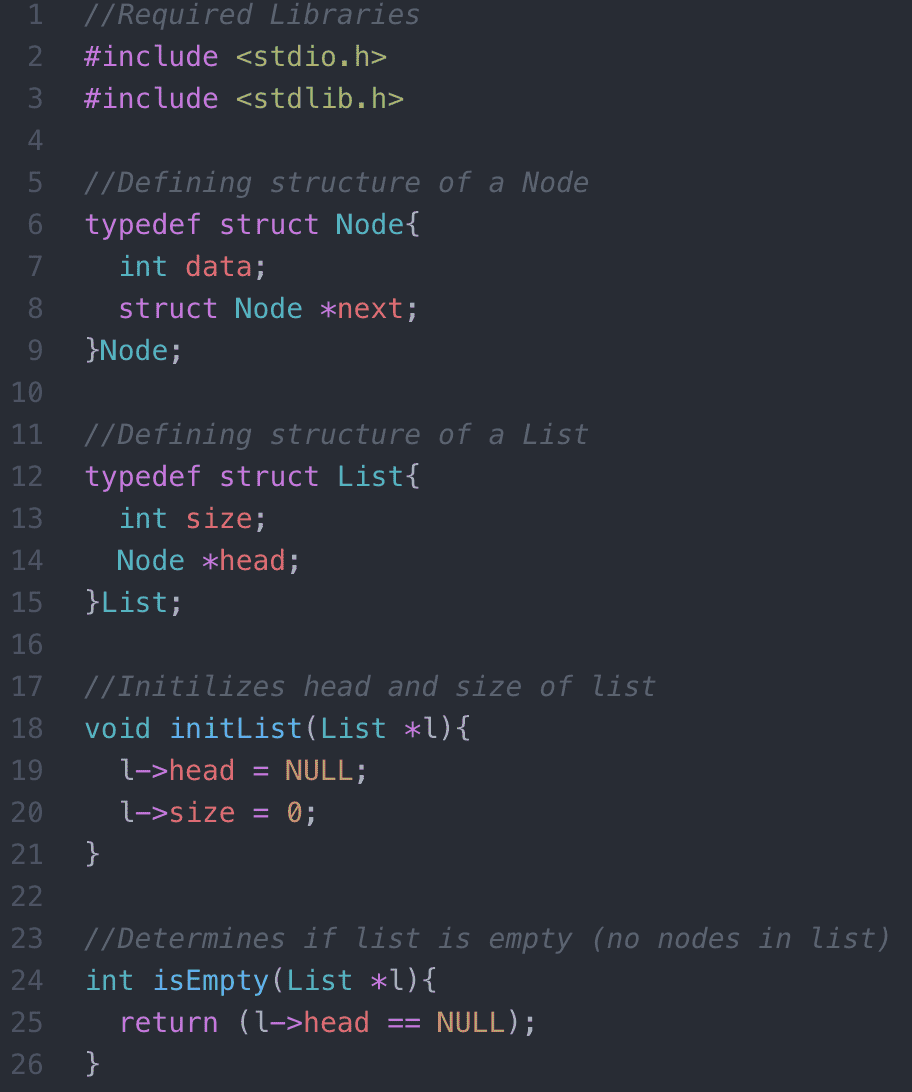
A linked list in C programming is a linear data structure that consists of a group of nodes in a sequence. Each node contains two parts:
- Data
- Address to the next node (pointer to next node)
In a Linked List in C, each node points to the next node in a sequence, forming a chain-like connection. The final node in this structure points to NULL, marking the end of the list.
Some key properties of linked lists are:
- Items can easily be added or removed without reallocation of memory
- Memory is dynamic
- No wastage of memory
- Sizes can increase or decrease as required
Part 2: Difference Between Singly and Doubly Linked List Program in C
Primarily, two kinds of linked lists exist: singly linked lists and doubly linked lists. The key distinctions between them are:
- Singly Linked List: Singly-linked lists have one pointer to the next node. Each node stores a single pointer (address of the next node).
- Doubly Linked List: In a doubly linked list, each node consists of three parts: node data, a pointer to the next node (next address), and a pointer to the previous node (previous address). So each node maintains two links.
The advantage of a doubly linked list over a singly linked list is that we can traverse the DLL (Doubly Linked List) in both forward and backward directions. This enables more flexibility in operations.
Part 3: Steps for Implementing a Simple Linked List Program in C
Here are the key steps to create a simple linked list program in C from scratch:
1. Include header files: stdio.h, stdlib.h, malloc.h
2. Create a Node structure with two members:
- int data (to store node value)
- struct Node* next (Contains the address of the next node)
3. Create linked list functions:
- createNode() – to allocate memory for each node
- insertAtFront() – insert at beginning
- printList() – traverse and print
4. Define main():
- Create a start node and initialize the head
- Insert nodes using insertAtFront()
- Print nodes using printList()
- Free memory allocated to a linked list
Part 4: Overview of Linked List Program in Python
The implementation of linked lists in Python is simpler compared to C since Python has built-in dynamic memory allocation. We do not need to allocate/free memory explicitly.
The Node class contains the node value and reference to the next node. The Linked List class contains a Node object that refers to the head node and methods to add, and remove nodes, compute length, and print contents.
Some key points:
- Each Node refers to the next node creating the linkage.
- The LinkedList class holds a reference to only the head node.
- Appending involves traversing towards the tail of the list.
- node.next refers to the next node object in the linked list.
So Python handles most complexities allowing developers to focus on core logic.
Part 5: Creating an Algorithm Flowchart Using EdrawMax
When implementing complex programs like linked lists, using a flowchart to visualize logic flow is highly beneficial. You can use EdrawMax to create flowcharts that provide an overview of the key steps.
Some benefits of using EdrawMax for programming flowcharts:
- Ready-made flowchart templates for multiple scenarios.
- Drag-and-drop interface to easily build flowcharts.
- Professional flowchart symbols to depict various functions.
- Ability to add connections to show complex flows.
- Flowchart examples available for reference.
- Shareable across teams for better understanding.
By creating flowcharts for data structure implementation, we can easily visualize the logical flow, detect flaws in the design, improve the readability of complex sections, and streamline development workflow.
Here are the steps to create a simple algorithm flowchart using EdrawMax:
Step 1:
Launch the EdrawMax software on your computer. Click on "File" and select "New" to start a new document. Choose the "Algorithm Flowchart" category from the template gallery.
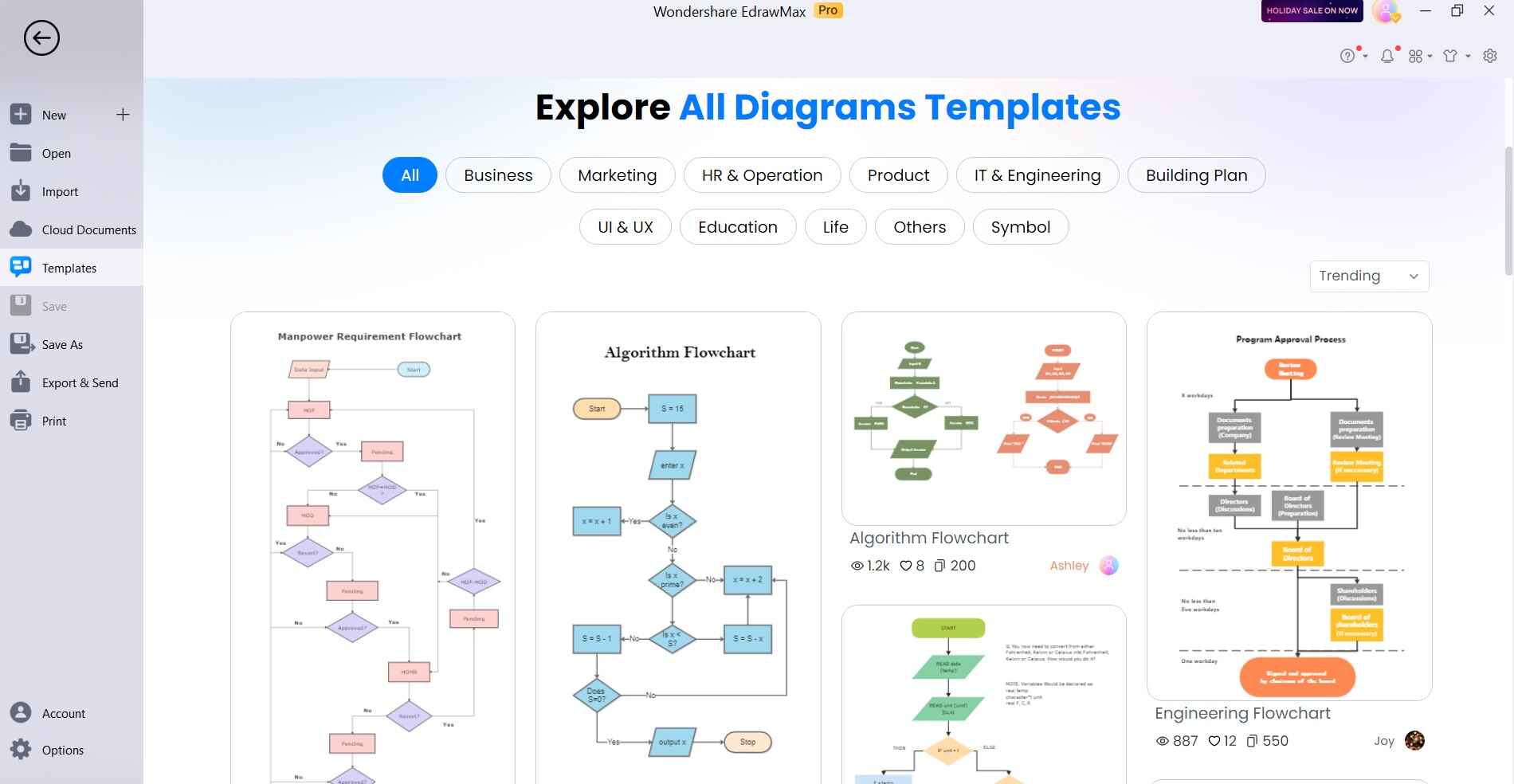
Step 2:
Drag and drop the necessary symbols and shapes from the left-hand sidebar onto the canvas.
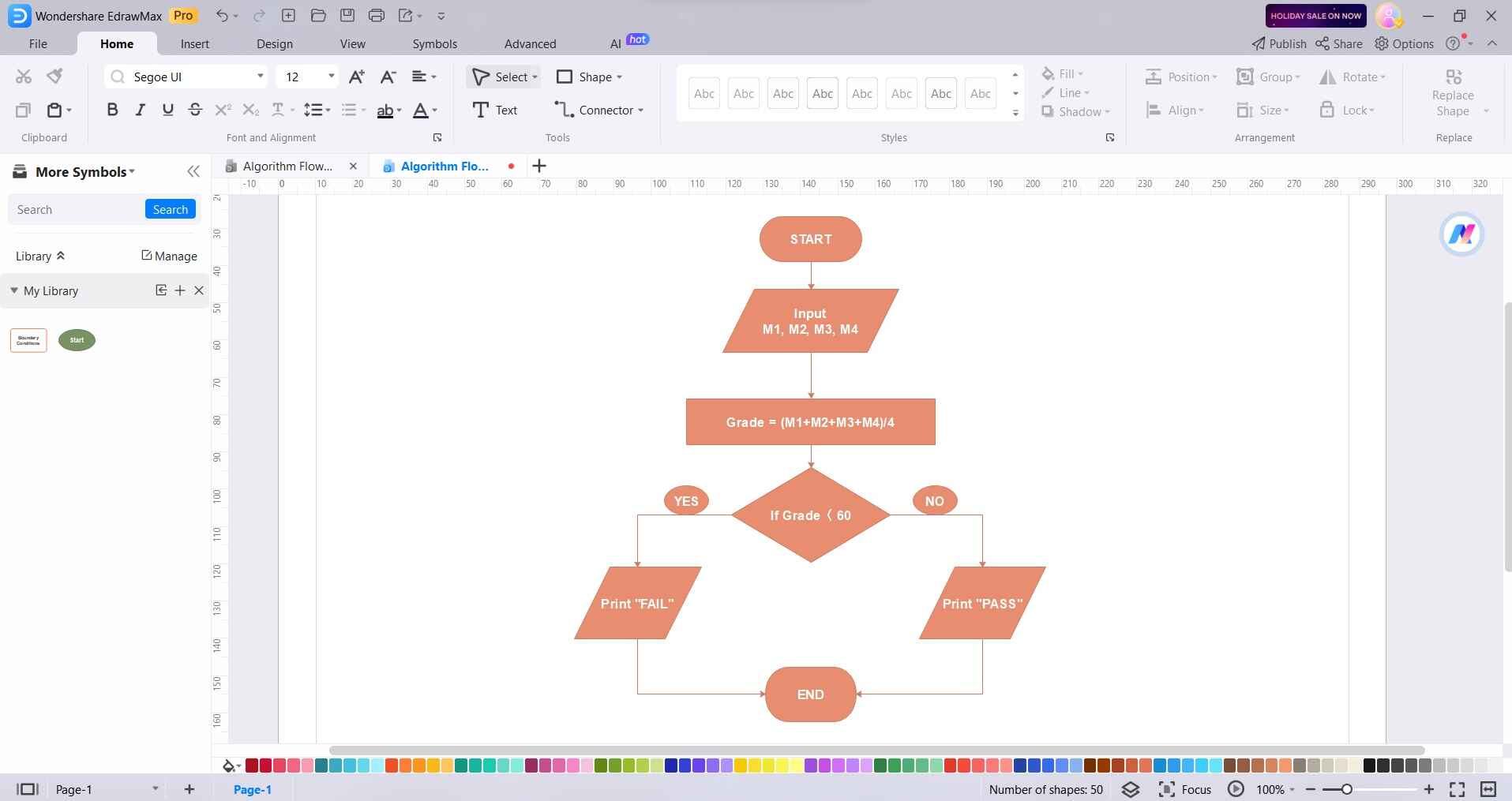
Step 3:
Double-click on shapes to add text and input your algorithmic steps or descriptions.
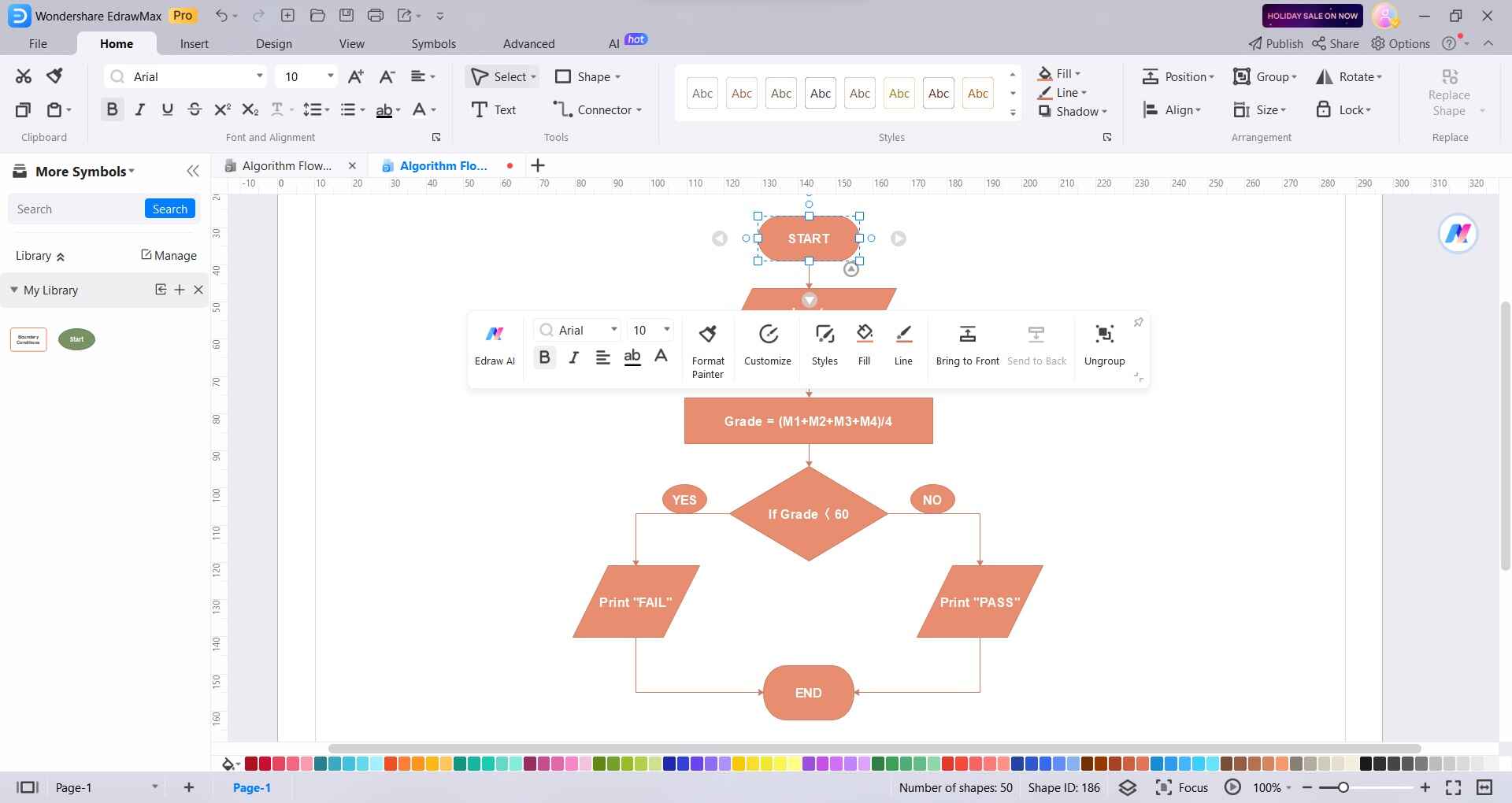
Step 4:
Customize the appearance by changing colors, fonts, sizes, and styles to make the flowchart more visually appealing and understandable.
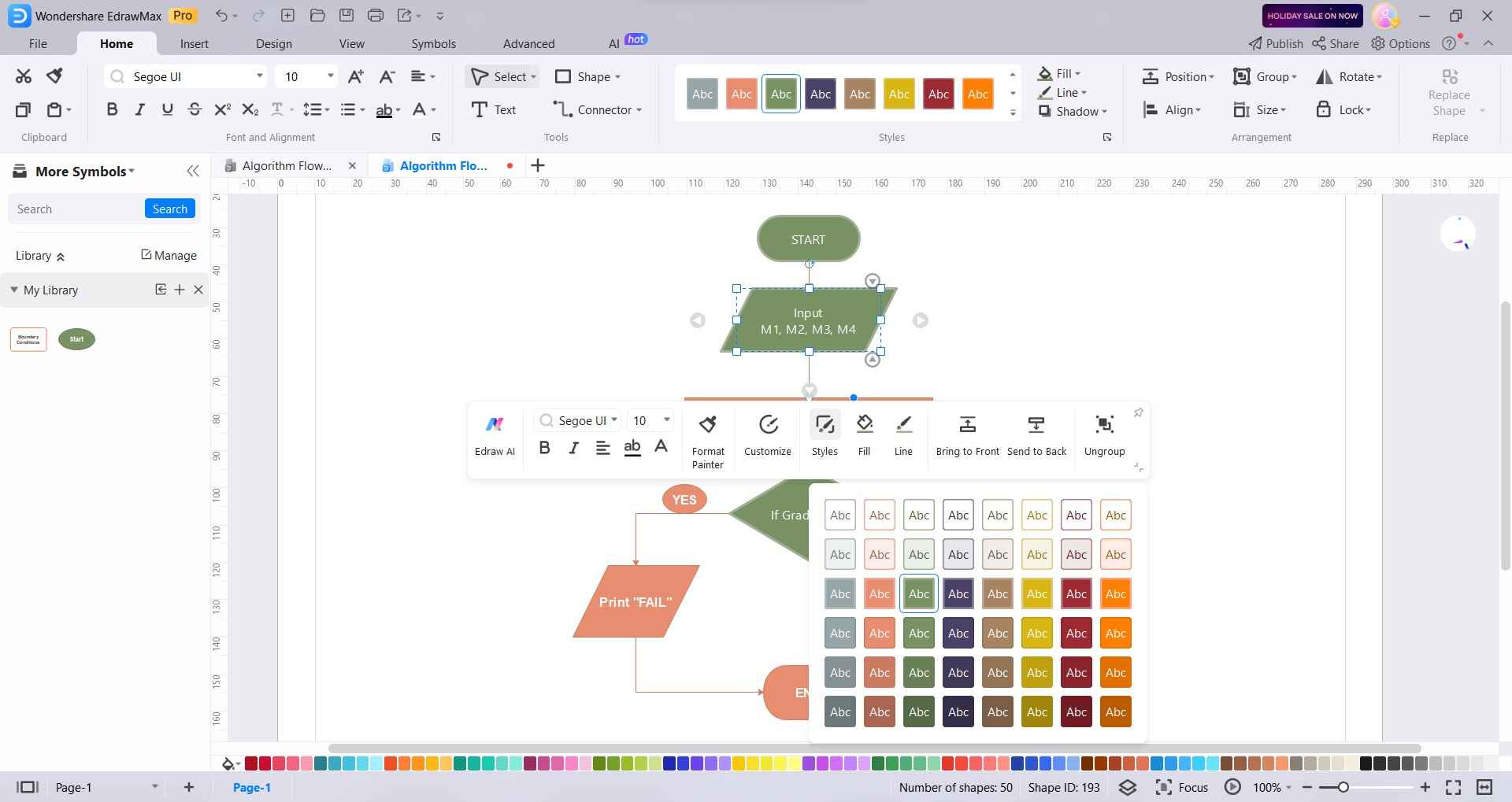
Step 5:
Once completed, save your flowchart by clicking on "File" and selecting "Save As." You can also export your flowchart to various formats like PNG, JPEG, PDF, etc., by clicking on "File" and choosing "Export."
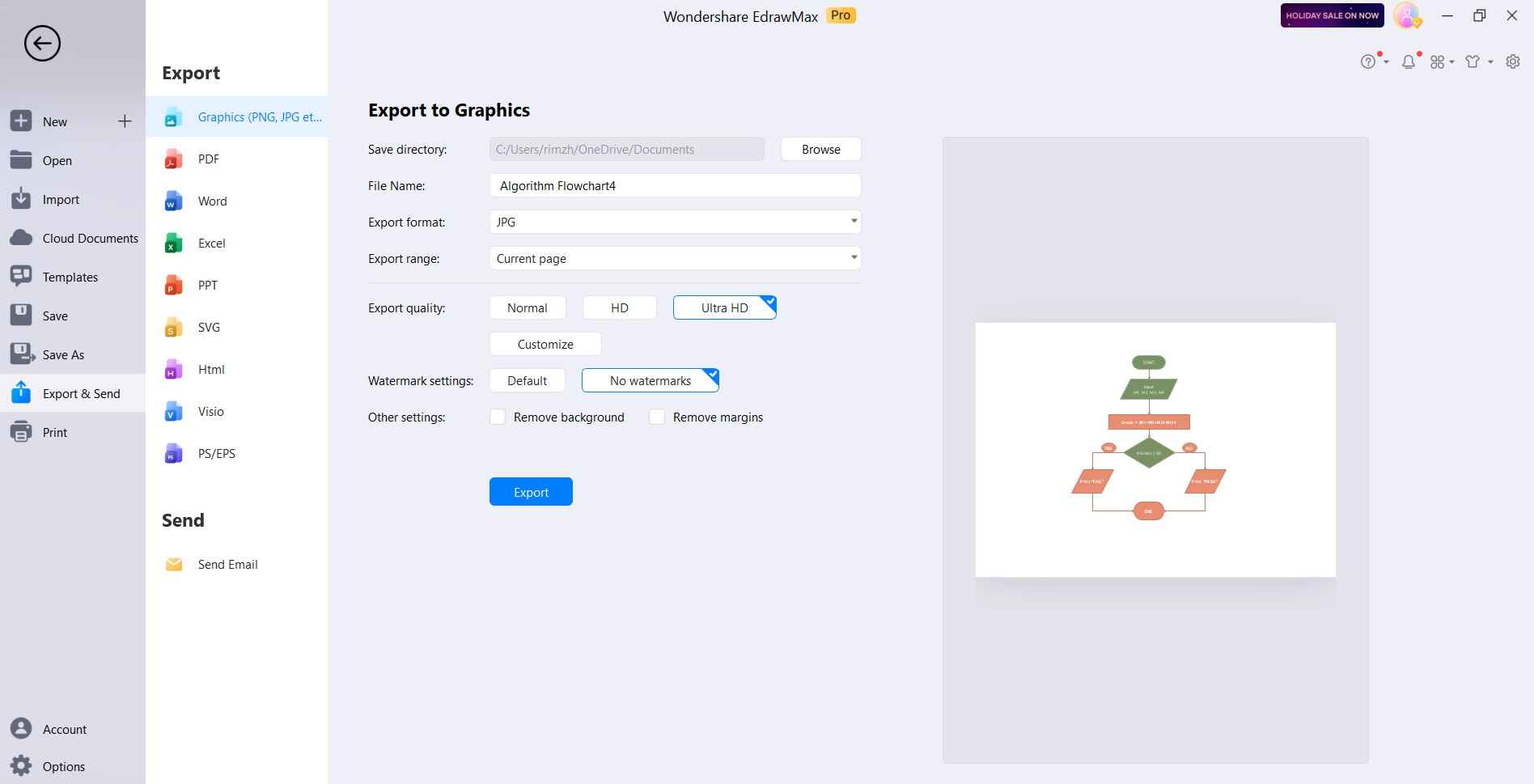
These steps should help you create a simple algorithm flowchart using EdrawMax. Feel free to explore additional features and tools within EdrawMax to enhance your flowchart as needed!
Conclusion
In this article, we discussed the step-by-step process of building a linked list program in C – understanding concepts, differences from doubly linked lists, structuring nodes, writing helper functions, and implementing core logic.
We also saw an overview of Python’s simpler linked list constructs. Finally, we highlighted the importance of using tools like EdrawMax to create algorithm flowcharts to improve the development of complex programs like linked lists.
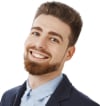