Linked lists are one of the fundamental data structures used in computer programming. Unlike arrays, linked lists do not store data contiguously in memory. Instead, each element in a linked list contains a reference or pointer to the next element, allowing flexible organization of data.
Mastering different types of linked lists is key for any aspiring computer programmer. In this article, we will provide an overview of each type along with their advantages and drawbacks.
In this article
Part 1: What is a Singly Linked List Program in Data Structure?
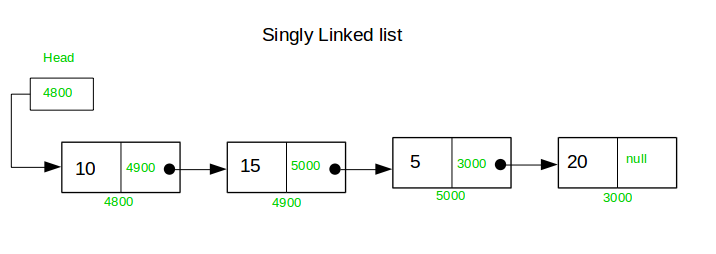
A singly linked list contains nodes where each node stores a data element and a reference or pointer to the next node. It can be traversed in only one direction from the first node called the head to the last node called the tail.
Part 2: Overview of a Doubly Linked List Program in Data Structure
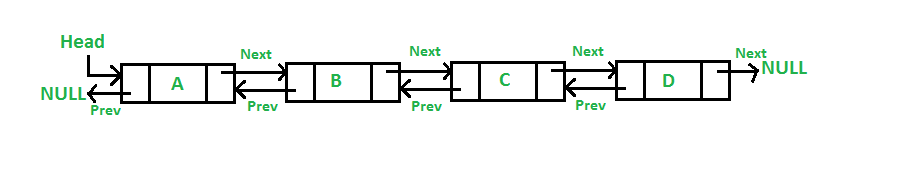
A doubly linked list contains nodes that contain data and pointers to both the previous and next nodes in the list. This forms a chain of nodes in two directions.
The key benefits provided by a doubly linked list are:
- Ability to traverse and access nodes both forward and backward efficiently. This makes several operations faster such as insertion and deletion.
- Provides stable and reliable access even if the first reference node gets deleted or disabled for some reason.
- Algorithms can easily access both ends of the linked list, the head and tail, from any node rather than traversing to them like in singly linked lists.
The downside with doubly linked lists is extra memory consumption as two reference pointers are needed per node rather than just one. Care also needs to be taken when manipulating the nodes to correctly update the forward and backward references. So there is some extra code complexity needed in managing both pointers.
Overall, doubly linked lists provide very flexible and fast access at the cost of extra memory usage and slightly more complex code for pointers.
Part 3: Understanding Circular Linked List Program in Data Structure
A circular linked list loops back to form a continuous circle of nodes. The tail node stores a pointer back to the first head node rather than terminating like a regular linked list.
Some advantages of circular linked lists are:
- Circular iteration – Algorithms can loop infinitely through the nodes which can be useful for creating repetitive tasks or circular buffers/queues.
- Can easily add nodes before the head or after the tail efficiently.
- Often used for advanced data structures like Fibonacci heaps.
Part 4: Pros and Cons of Using Linked Lists
Now that we have looked at different linked list types, let’s summarize some general pros and cons of using linked lists:
Pros:
- Dynamic data organization - Nodes can be easily inserted and removed without shifting the whole structure.
- Efficient memory usage – Only allocate what is needed with no wasted pre-allocated memory like arrays.
- Flexibility – Various types of linked lists provide specialized access like circular or double-ended.
Cons:
- No direct indexing – Must traverse sequentially node by node to access data, slower access.
- Extra overhead with each node to maintain the next pointer and sometimes the previous pointer.
- Risk of broken chain if pointer link between nodes breaks, harder to debug.
- No locality of reference is provided by contiguous memory-like arrays.
As with most data structures, there are always some tradeoffs. For most applications, linked lists provide very useful, flexible representation and access even with the extra memory and traversal overhead.
Part 5: Creating an Algorithm Flowchart Using EdrawMax
When creating complex linked list algorithms, using a tool like EdrawMax to map out a flowchart can be invaluable. A flowchart lets you visualize the logic flow before coding while also creating useful documentation.
Some benefits of using EdrawMax for programming flowcharts are:
- User-friendly interface with drag-and-drop symbols and drawing tools optimized for diagramming algorithms.
- Easy to add text descriptions, connectors, and different shapes to represent functions, loops, etc.
- Export flowcharts as image files or even as source code outlines.
- Creates professional-looking charts with styles and templates for compliance and presentation.
- Full compatibility with Microsoft Office and other document tools.
Here are the steps to create a simple programming flowchart using EdrawMax:
Step 1:
Launch the EdrawMax software on your computer. Click on "New" or "File" -> "New" to create a new document. Choose the "Flowchart" category from the template options.
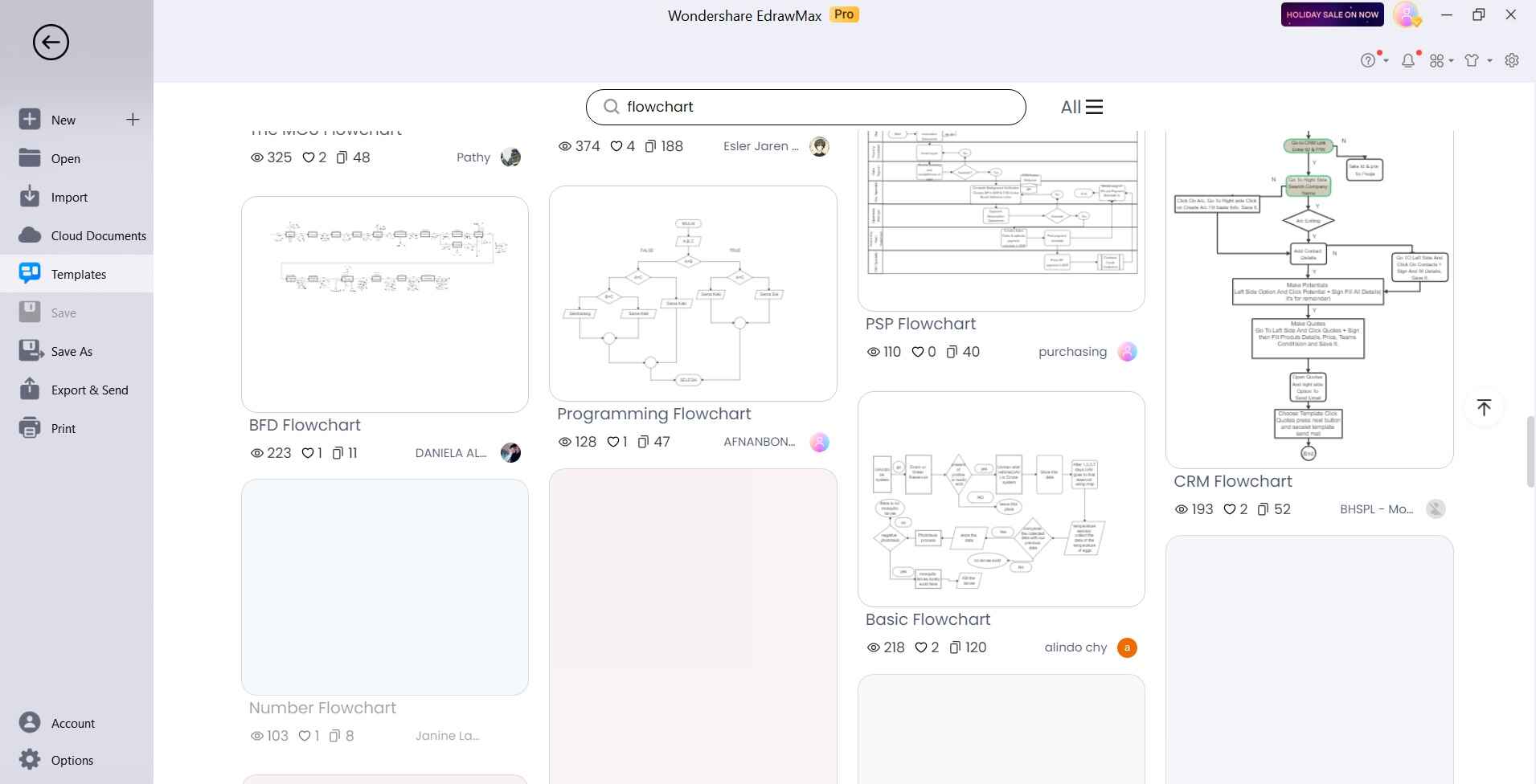
Step 2:
Explore the symbol library provided in EdrawMax. Drag and drop the necessary symbols onto the canvas.
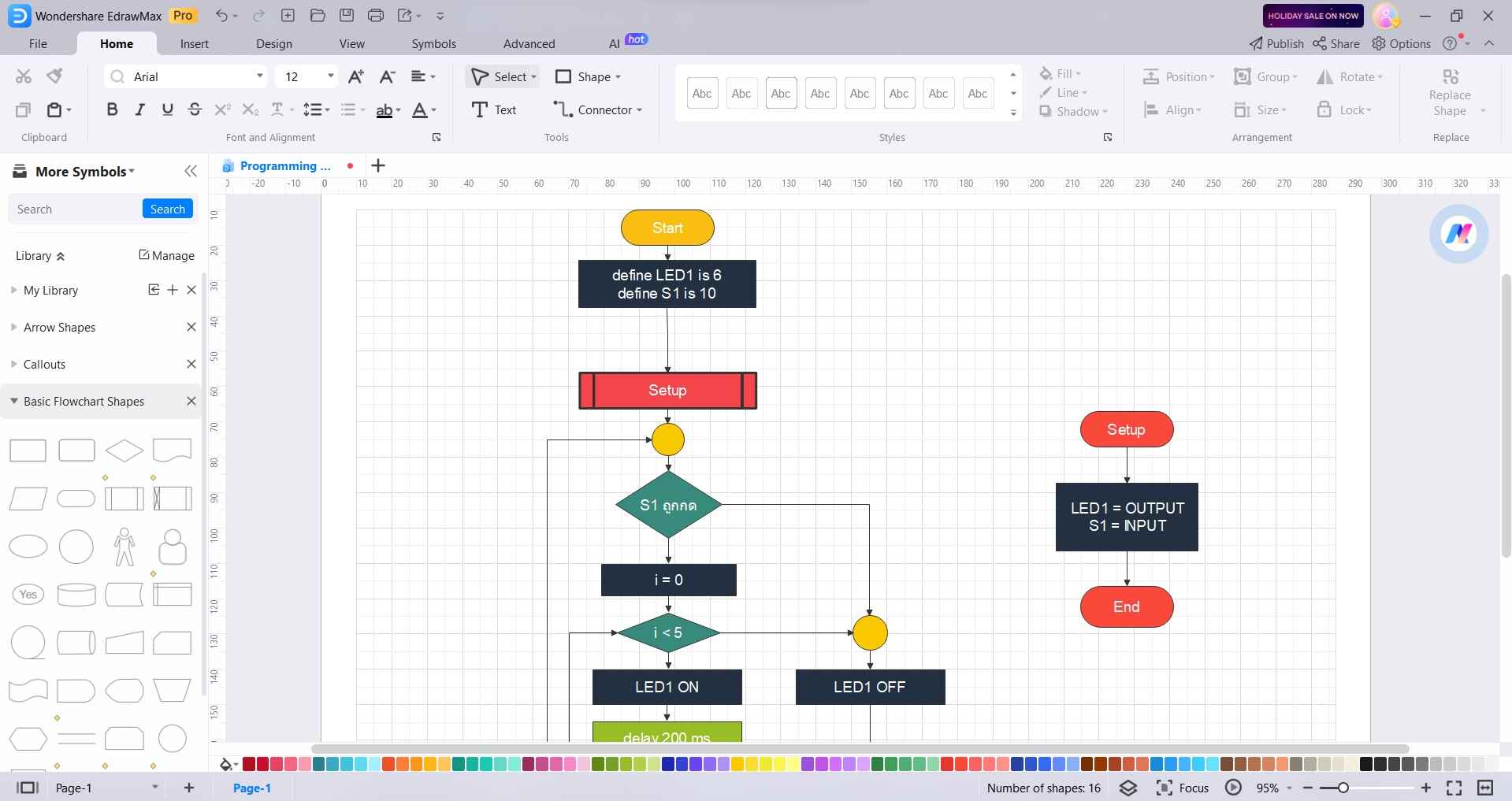
Step 3:
Use connectors to link the symbols together to form the logical flow.
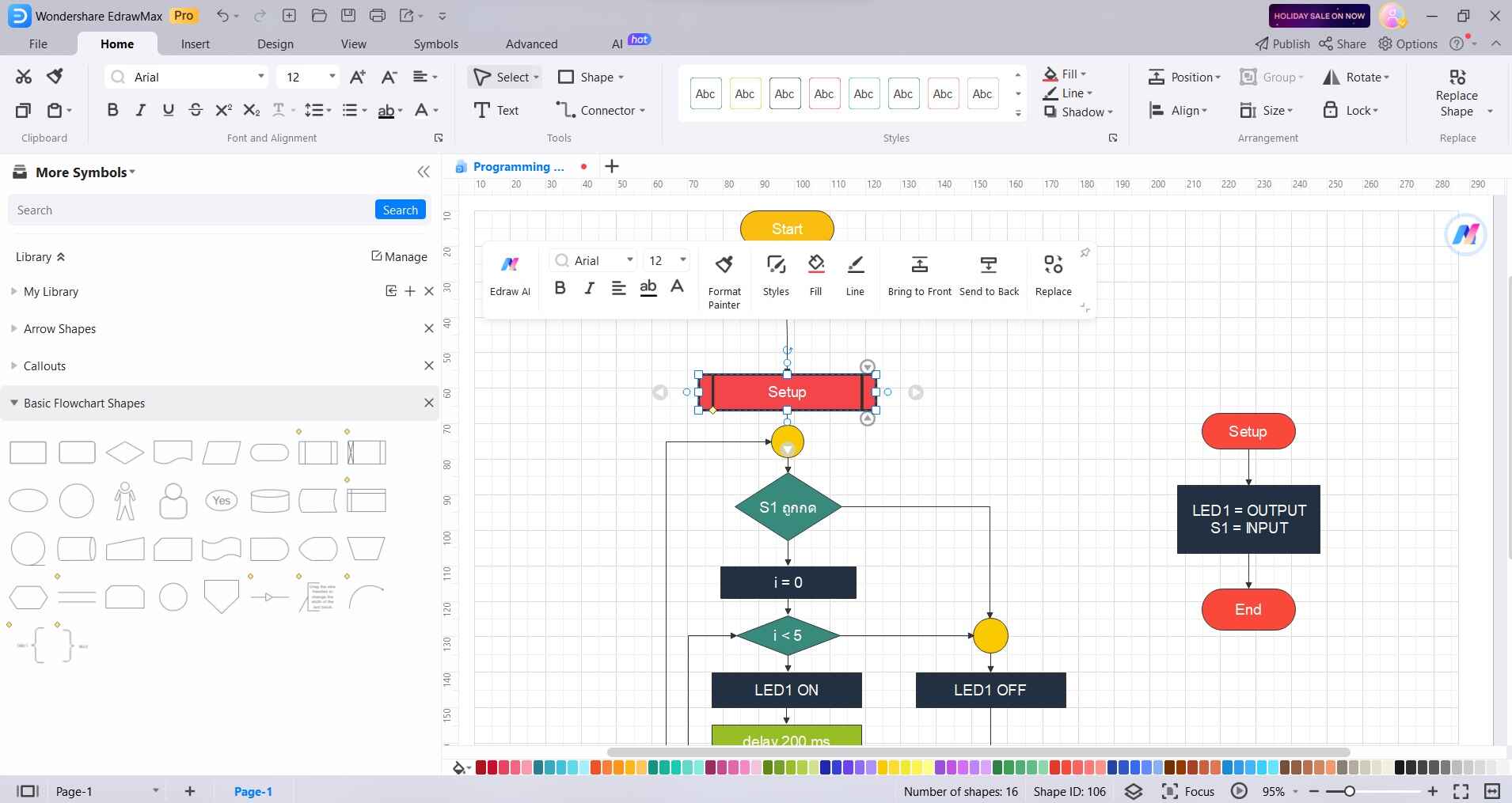
Step 4:
Customize the flowchart by changing colors, line styles, and fonts.
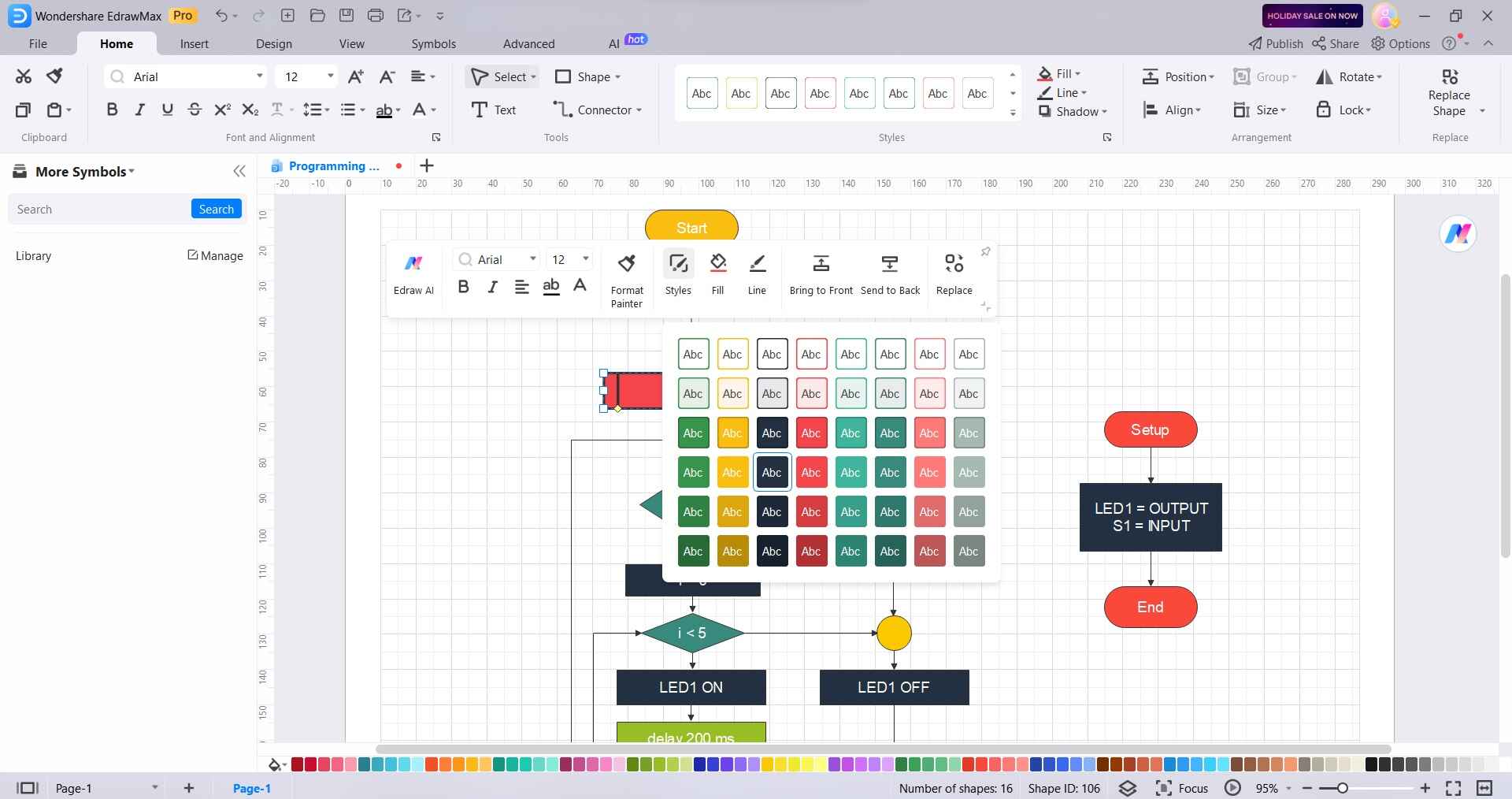
Step 5:
Save your work in EdrawMax's native format for future editing. You can also export the flowchart in various formats like JPG, PNG, PDF, etc.
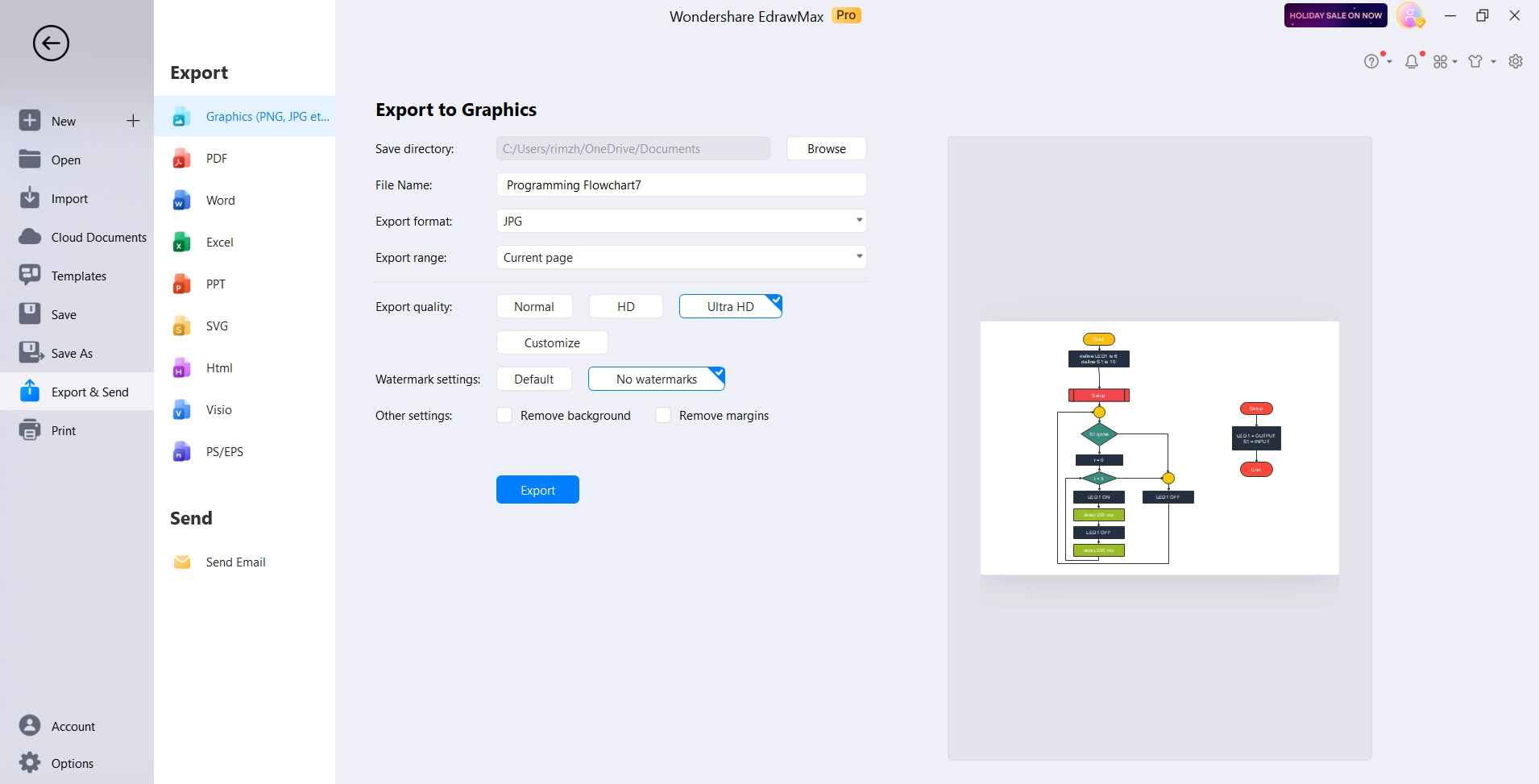
Follow these steps to create a simple programming flowchart using EdrawMax, allowing you to visualize and plan your program's logic systematically.
Conclusion
Mastering the practical implementation of essential data structures like linked lists marks an important programming milestone. Understanding the subtle differences between single, double, and circular linked lists in terms of efficiency tradeoffs, use cases, pros, and cons will pay dividends across a wide range of complex coding challenges.
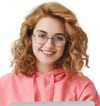