Priority queues are useful data structures that provide constant time access to the minimum or maximum element. In Python, priority queues can be efficiently implemented using the heapq module.
This article will walk through how to implement priority queues in Python, including operations like push, pop, and peek. We'll also discuss the pros and cons of using priority queues and look at creating a programming flowchart for visualization.
In this article
Part 1: What is a Priority Queue in Python?
Priority queues are useful data structures that provide constant time access to the minimum or maximum element. In Python, priority queues can be efficiently implemented using the heapq
module.
This article will walk through how to implement priority queues in Python, including operations like push, pop, and peek. We'll also discuss the pros and cons of using priority queues and look at creating a programming flowchart for visualization.
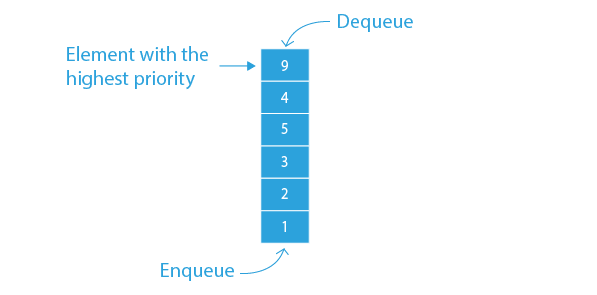
A priority queue is an abstract data type similar to a regular queue, except each element has a certain priority associated with it. The priority of the elements determines the order in which elements are removed from the priority queue. In a priority queue, an item possessing a higher priority receives service ahead of an item with a lower priority.
Part 2: Overview of Golang Priority Queue
Golang provides a container/heap
package that can be used to implement priority queues.
Some key characteristics of Golang's priority queue:
- Implements a min-heap data structure for efficient ordering and retrieval.
- Provides
Push()
andPop()
methods to insert/remove elements. Peek()
method to access minimum elements without removal.- Implements heapify, allowing the creation of a heap from the slice.
- Uses comparators to customize priority and ordering.
Overall, Golang provides an efficient priority queue implementation out of the box via the heap package.
Part 3: Steps to Implement Priority Queue Using Heap
Here are the steps to implement a priority queue using a heap without code:
Step 1: Define the Priority Queue - Start by defining a data structure to represent the priority queue.
Step 2: Choose a Heap Implementation - Decide on a heap data structure implementation, like a binary heap or Fibonacci heap. Heaps are suitable for priority queues due to their efficient insertion and extraction of elements based on priority.
Step 3: Represent Priority and Elements - Each element in the priority queue should have both a priority value and the actual data or item.
Step 4: Implement Insertion - Define the insertion process for adding elements into the priority queue. When inserting an element, place it in the appropriate position in the heap according to its priority.
Step 5: Implement Extraction - Implement the process to extract the highest priority element from the priority queue. This involves removing the element with the highest priority from the heap.
Step 6: Maintain Heap Property - Ensure that the heap property is maintained after every insertion or extraction operation. In a max-heap, for instance, the highest priority element should always be at the root.
Step 7: Define Additional Operations - Consider implementing other operations like checking if the priority queue is empty, determining its size, or peeking at the highest priority element without removing it.
Step 8: Test the Implementation - Create test cases to validate the functionality of the priority queue. Test different scenarios involving insertion, extraction, and edge cases to ensure correct behavior.
By following these steps, you can create a priority queue using a heap data structure, allowing for efficient handling of elements based on their priorities.
Part 4: Pros and Cons of Using Priority Queue in Python
Pros:
- Efficient ordering and retrieval in O(log n) time.
- Simple to use with the
heapq
module. - Supports multiple item priority levels.
- Thread-safe when using Python's multiprocessing module.
- Flexible - can write custom heuristics for comparing elements.
Cons:
- Contained within memory, could overflow for large queues.
- Element priority is set upon insertion, with no updating after.
- No searching or random access like arrays/lists.
- Not efficient when priority is the same for most elements.
Overall, priority queues shine for order-sensitive queuing tasks. The speed and simplicity make them a useful data structure.
Part 5: Creating a Programming Flowchart Using EdrawMax
EdrawMax is professional diagramming software that can help create comprehensive flowcharts and visualizations of code logic and program workflow.
Here are some key reasons why EdrawMax is useful for programming flowcharts:
- Includes a variety of flowcharts, UML diagrams, and visualization templates specifically for programming. This accelerates the diagram design.
- Drag-and-drop interface makes it simple to construct programming flowcharts intuitively. No special technical skills are required.
- Smart auto-placement of shapes and connectors streamlines diagram building. Just add elements and EdrawMax neatly aligns and arranges them.
- Supports export to multiple file types including image files, PDF, Word, Excel, and PowerPoint. Great for documentation.
- Teams can collaborate on diagrams together in real time with smooth cloud integration.
Here are the steps to create a simple programming algorithm flowchart using EdrawMax:
Step 1:
Launch the EdrawMax application on your PC. Select the "Flowchart" category from the template options or use the search bar to find flowchart templates. Choose a blank flowchart template to start from scratch.
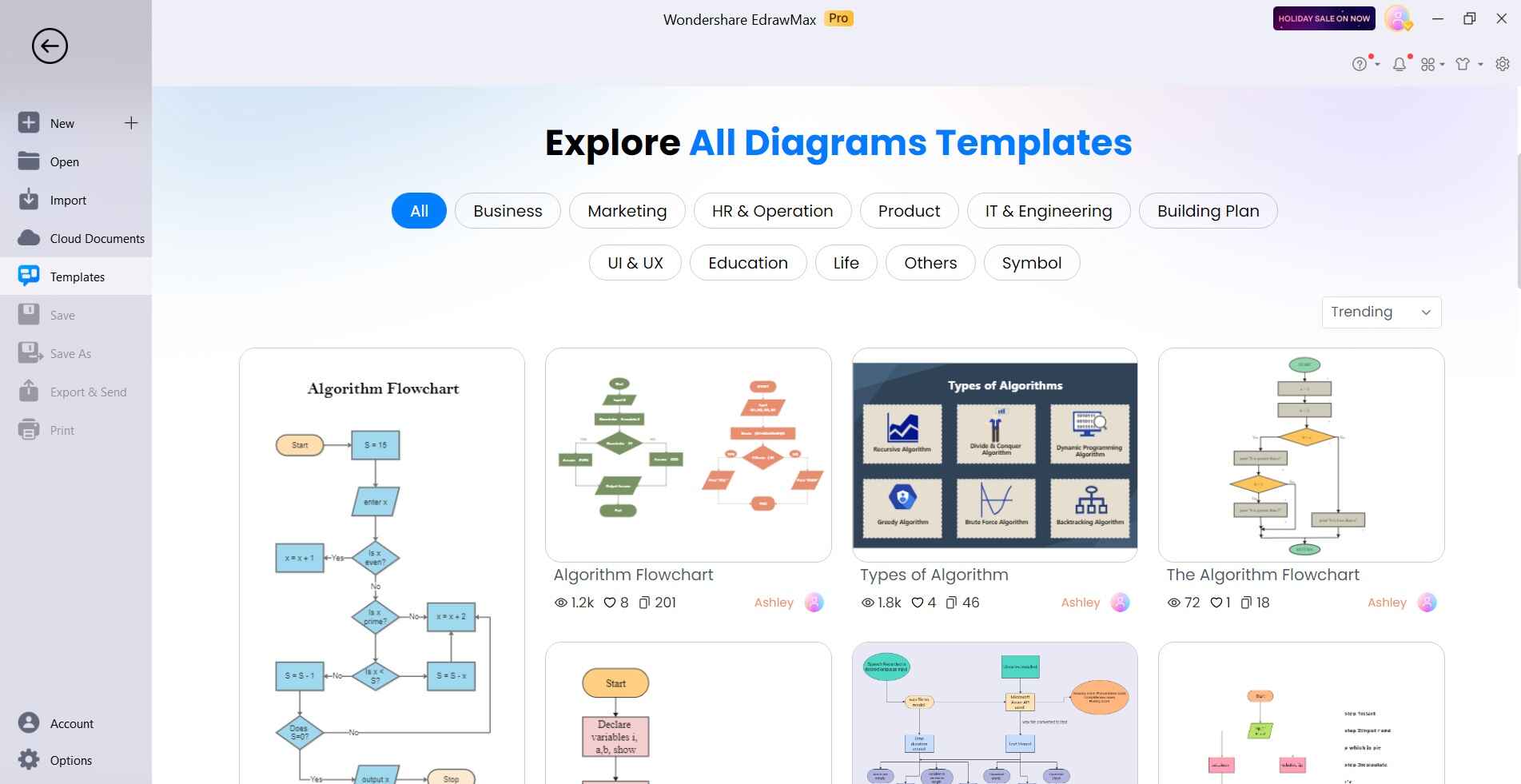
Step 2:
Drag and drop the "Start/End" symbol from the symbol library onto the canvas.
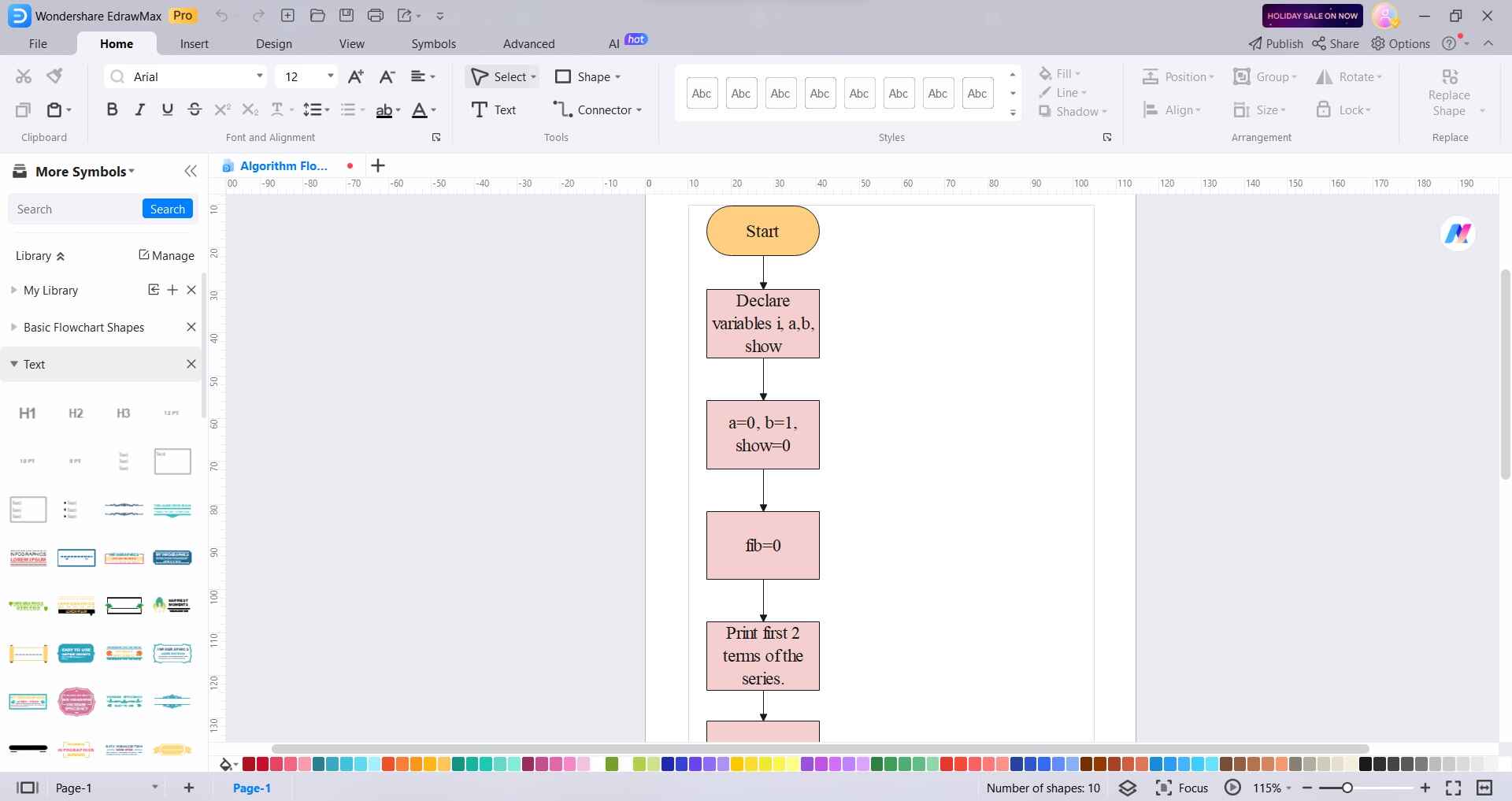
Step 3:
Incorporate decision symbols (diamond shapes) to represent conditional statements or branches in your algorithm.
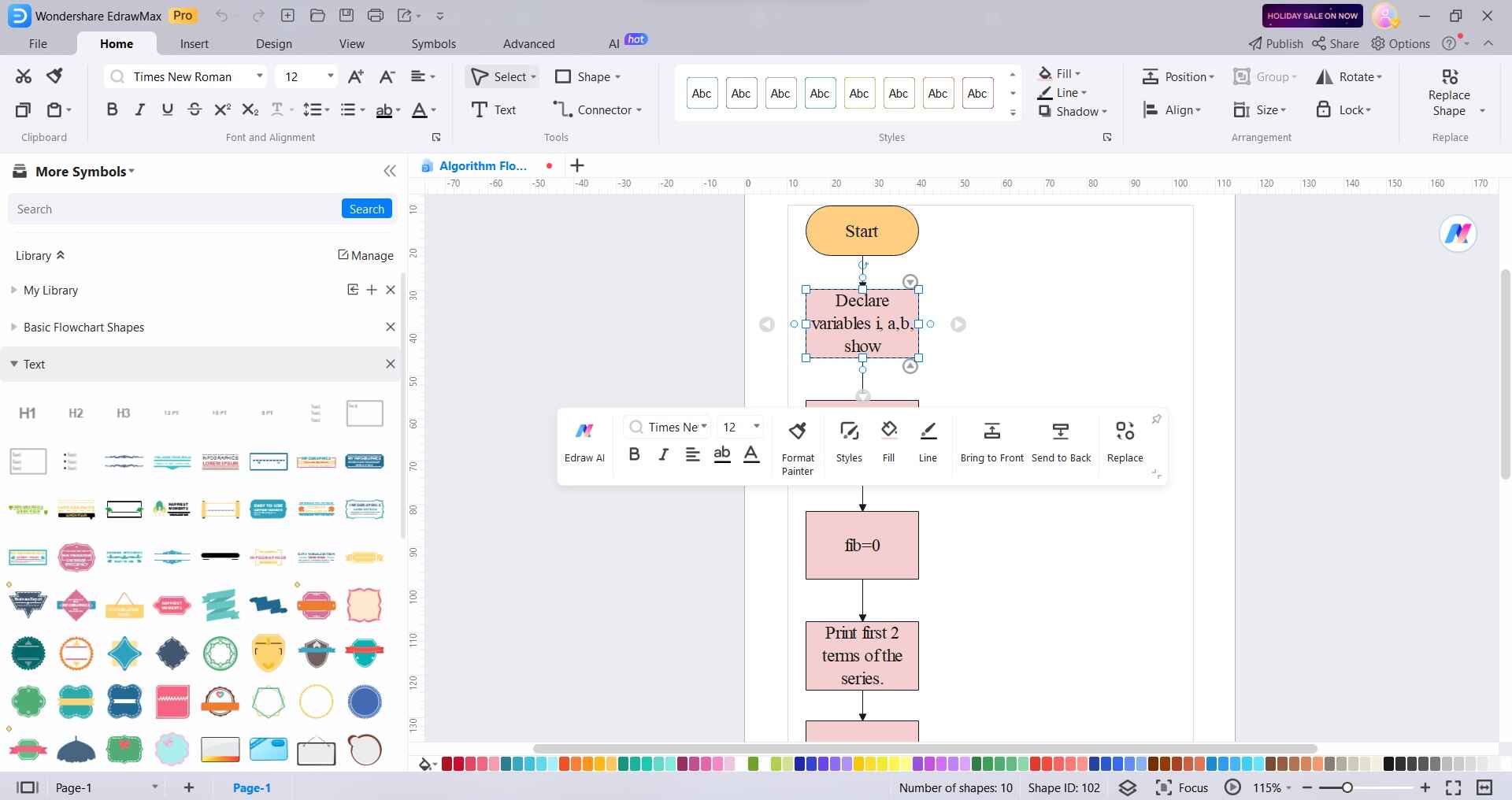
Step 4:
Adjust the layout, colors, spacing, and alignment of symbols and text to enhance readability.
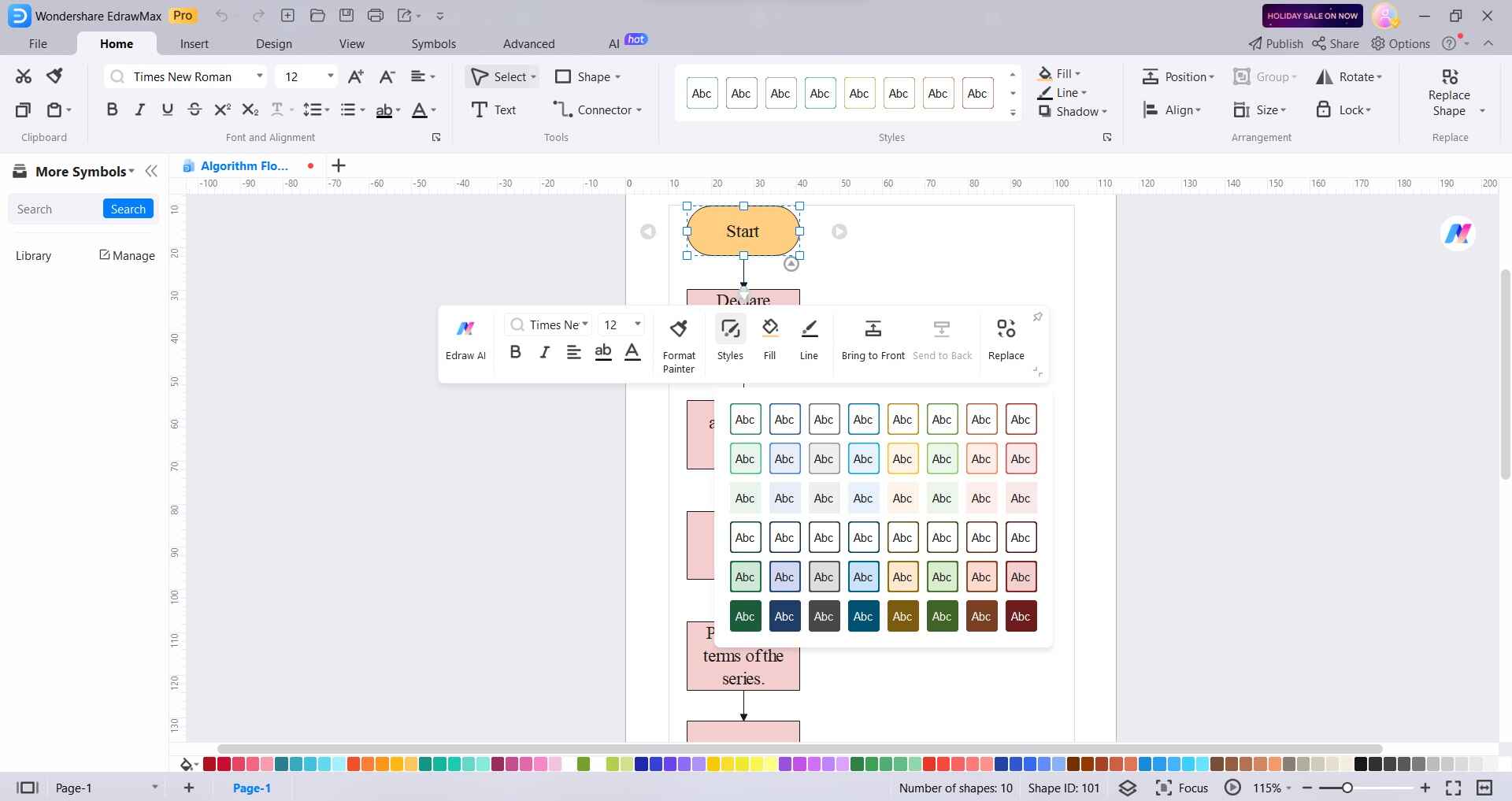
Step 5:
Once you're satisfied with the flowchart, save your work. EdrawMax allows you to save your file in various formats (e.g., .eddx, .pdf, .png). Choose the appropriate format for your needs.
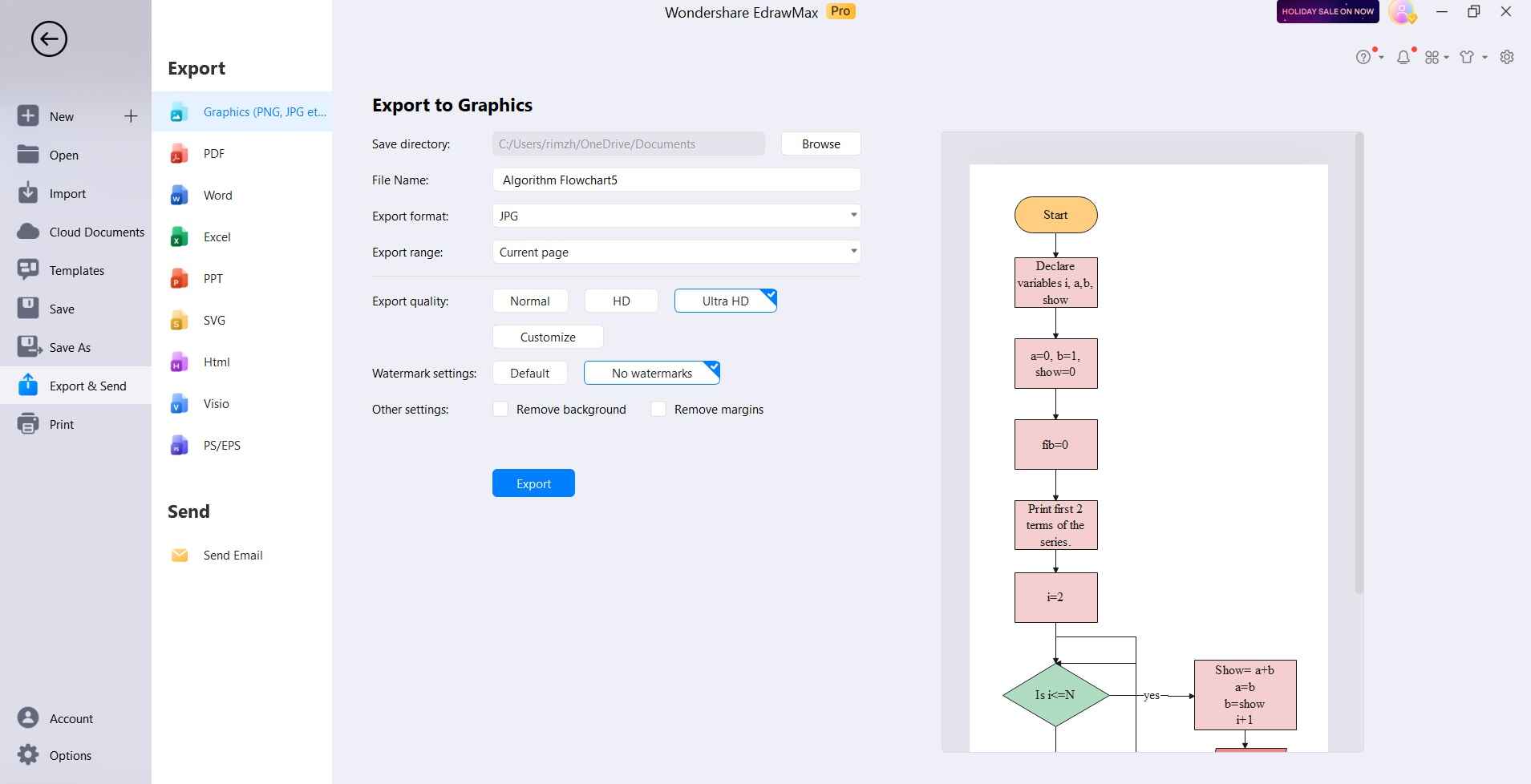
EdrawMax offers a user-friendly interface with drag-and-drop functionality, making it relatively easy to create flowcharts.
Conclusion
Priority queues are ordered data structures that efficiently retrieve elements by priority level. The simple approach highlighted, along with pros like speed and concurrency safety, make priority queues useful for scheduling applications and order-sensitive tasks. Diagramming software like EdrawMax can further improve code development by facilitating visualization of program logic and workflow.
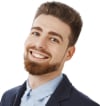