Queues are a fundamental data structure used in computer science and programming to store and retrieve data items in a first-in-first-out (FIFO) order. Queues have a wide range of applications such as operating system task scheduling, breadth-first search algorithms, and more. In Python, queues can be implemented easily using built-in data structures like lists or collections dequeue.
This comprehensive guide will provide an overview of queues and their implementation in Python.
In this article
Part 1: What is a Queue in Python?
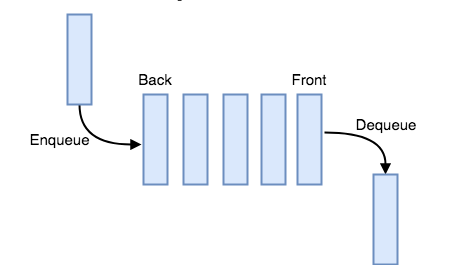
A queue is an ordered collection of items where additions happen at one end (the rear) and removals happen at the other end (the front). The order maintained in a queue is First In First Out (FIFO) — the item that goes in first comes out first.
Part 2: Overview of Stacks and Queues in Python
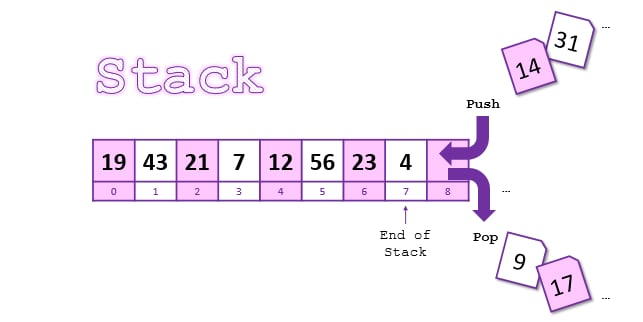
While queues follow FIFO ordering, stacks are a closely related data structure that follows LIFO (Last In First Out) ordering. The key difference is the end where elements are added/removed.
In stacks, both insertion and removal happen only from one end called the “top”.
In Python, stacks can be implemented using lists just like queues:
- append() to push/add to the top of the stack.
- pop() to pop/remove from the top of the stack.
The main operations of stacks and queues are:
Stacks:
- Push - Add the item to the top of the stack.
- Pop - Remove items from the top of the stack.
Queues:
- Enqueue - Adding an element at the rear of the queue.
- Dequeue - Removing an element from the front of the queue.
Queues and stacks allow ordered access to data in Python. Queues tend to be more useful for most real-world applications.
Part 3: Steps to Implement Queue in Python
Here are the main steps to implement a queue in Python:
- Import the queue module: To work with queue data structure, import the queue module in Python:
import queue
- Create a Queue Object: Create an object of the Queue class to instantiate a queue:
q = queue.Queue()
For multithreaded queues, use
Queue.Queue()
instead. - Add Items to the Queue (Enqueue): Use the
put()
method to add an item to the rear end of the queue:q.put(item)
- Remove Items from the Queue (Dequeue): Use the
get()
method to remove an item from the front end of the queue:q.get()
This removes and returns the item that was first enqueued.
- Check Queue Size: Get the size of the queue using
q.qsize()
method. - Check if Queue is Empty: Check if the queue is empty using
q.empty()
method. Returns True or False.
By following these steps, you can start implementing queues easily in your Python code for any use case.
Part 4: Tips for Implementing Queue in Python
Here are some tips for working with queues effectively in Python:
- Import
deque
for faster performance compared to lists when implementing queues. - Specify a max size for the queue while instantiating if needed to bound memory usage.
- Catch the
Empty
exception when removing from an empty queue to avoid errors. - For multithreaded queues, use
Queue
class instead ofqueue
to enable thread-safe locking mechanisms.
Following these tips will help you implement high-performance queues, avoid common errors, and enable thread-safe queue usage in your Python code.
Part 5: Pros and Cons of Using Queues in Python
Here are some key pros and cons of using queues in Python:
Pros:
- Maintains sequential FIFO order
- Efficient O(1) operations
- Useful for scheduling tasks, breadth-first search, etc.
- Thread-safe implementations possible
- Prevents race conditions and inconsistent output
Cons:
- Basic queues are unbounded so can grow large
- Not ideal for random access to elements
- Dequeuing from an empty queue raises an exception
- Multithreaded queues have a processing overhead
Part 6: Creating a Programming Flowchart Using EdrawMax
Creating visual flowcharts makes programs easier to understand, maintain, and debug. EdrawMax provides pre-made symbols and templates to create professional flowcharts quickly even with no prior experience. The program flow can be customized as needed by adding more steps or logic branches.
Here are the steps to create a simple programming flowchart using EdrawMax:
Step 1:
Launch the EdrawMax application on your device. Look for the "Flowchart" category or use the search bar to find flowchart templates. Choose a template that suits your needs or start from scratch with a blank canvas.
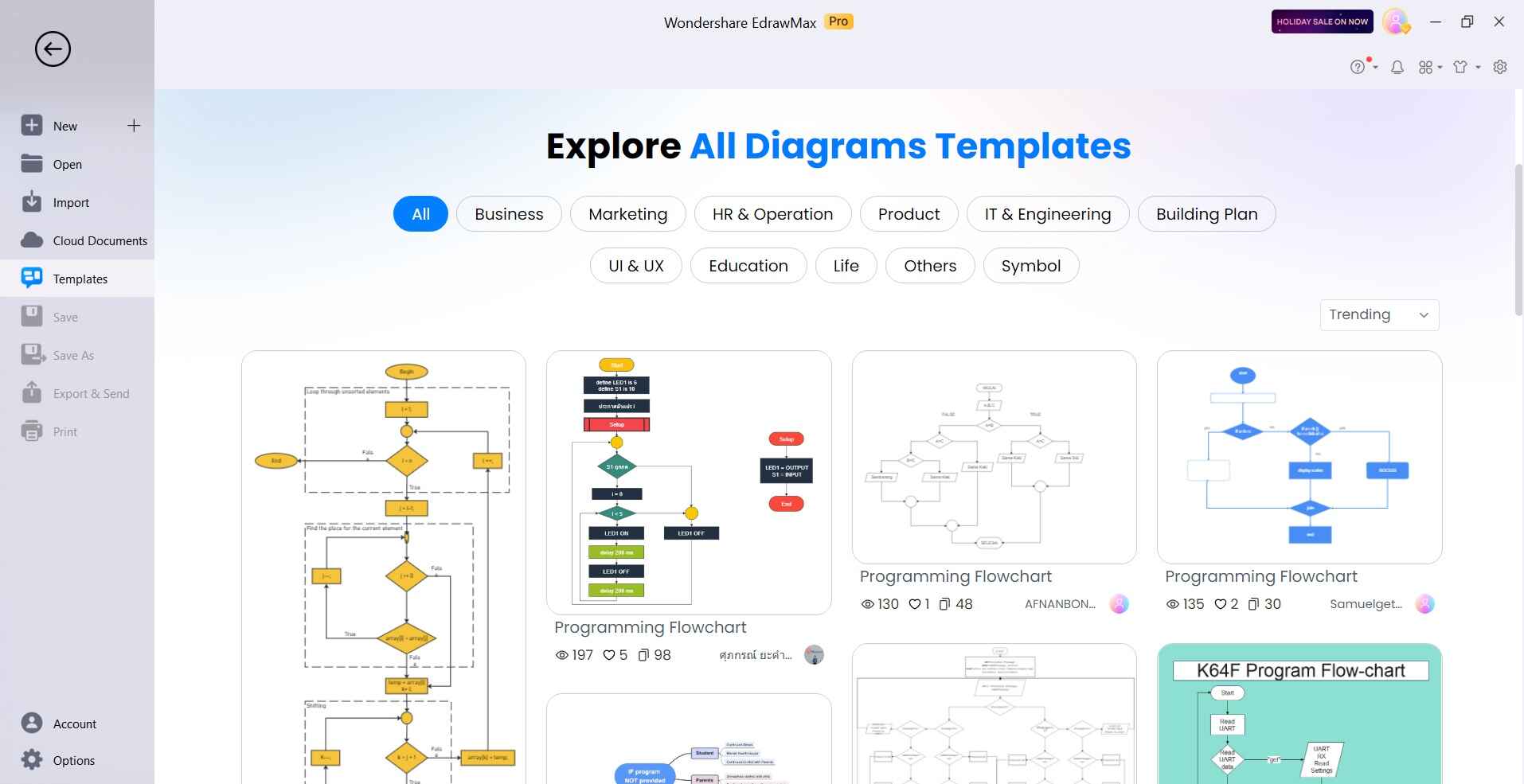
Step 2:
Drag and drop shapes and symbols from the toolbar onto the canvas.
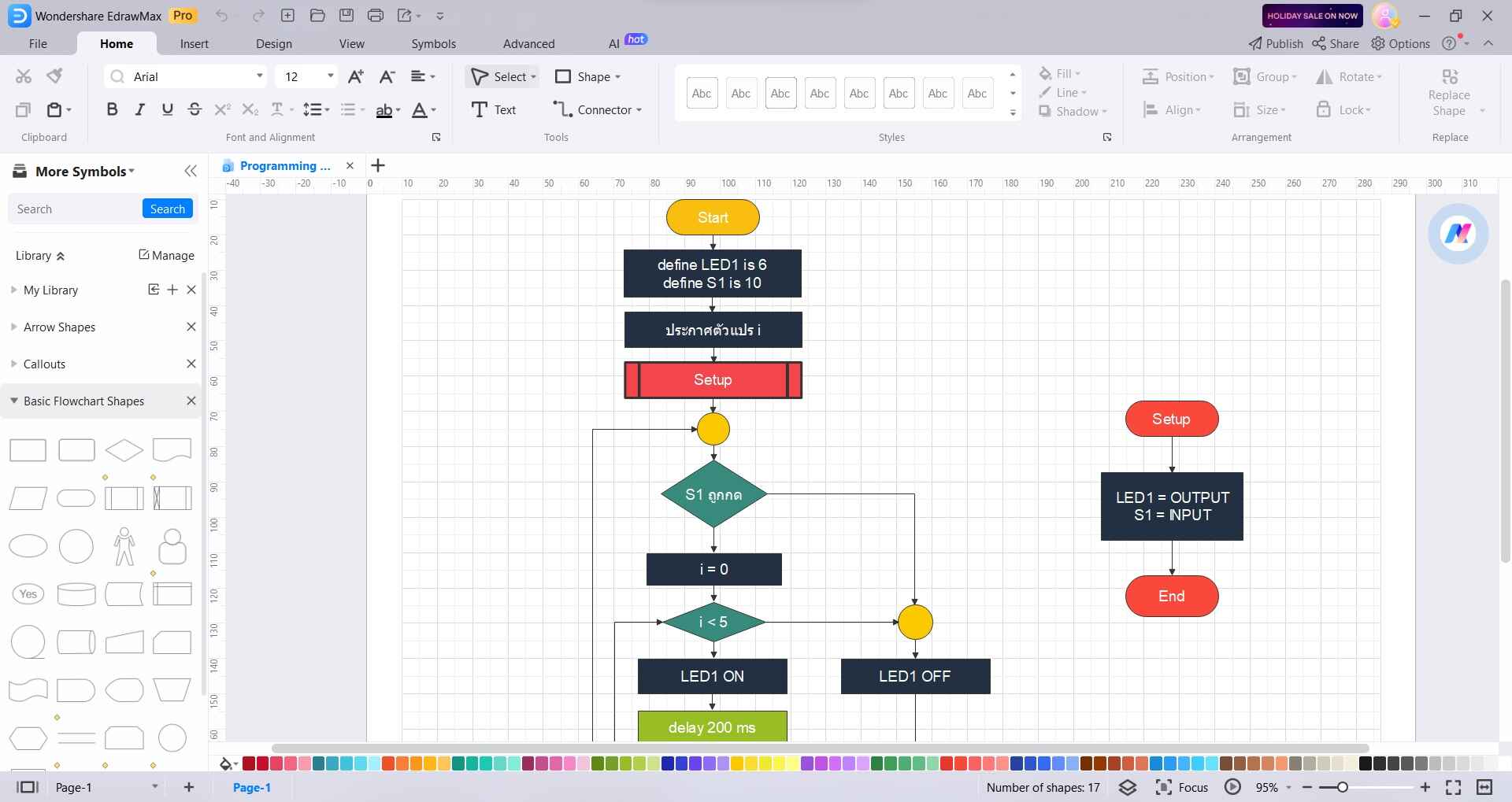
Step 3:
Use connectors or arrows to link the shapes together, indicating the flow of the program.
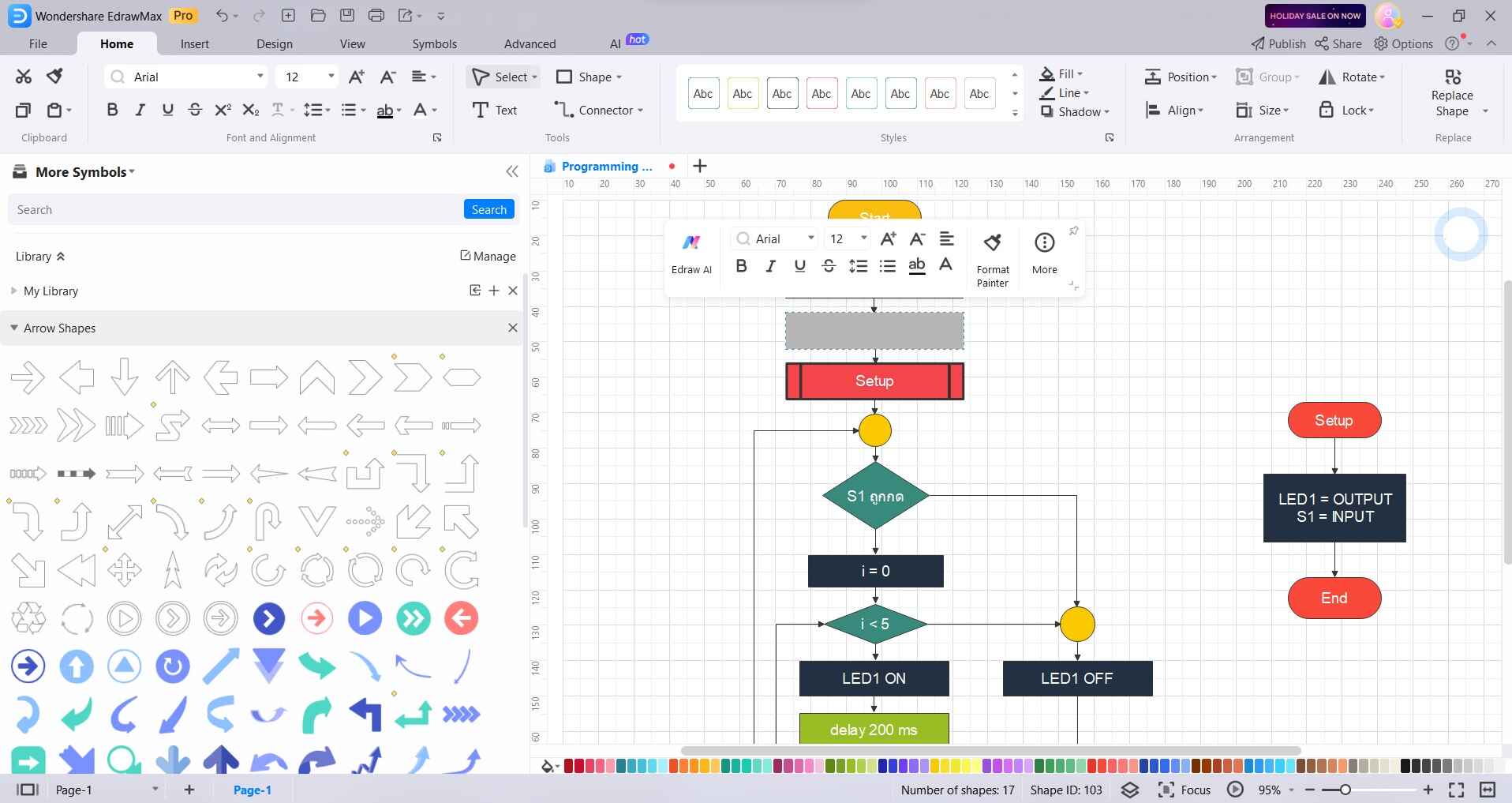
Step 4:
Customize the text size, font, and colors to enhance readability.
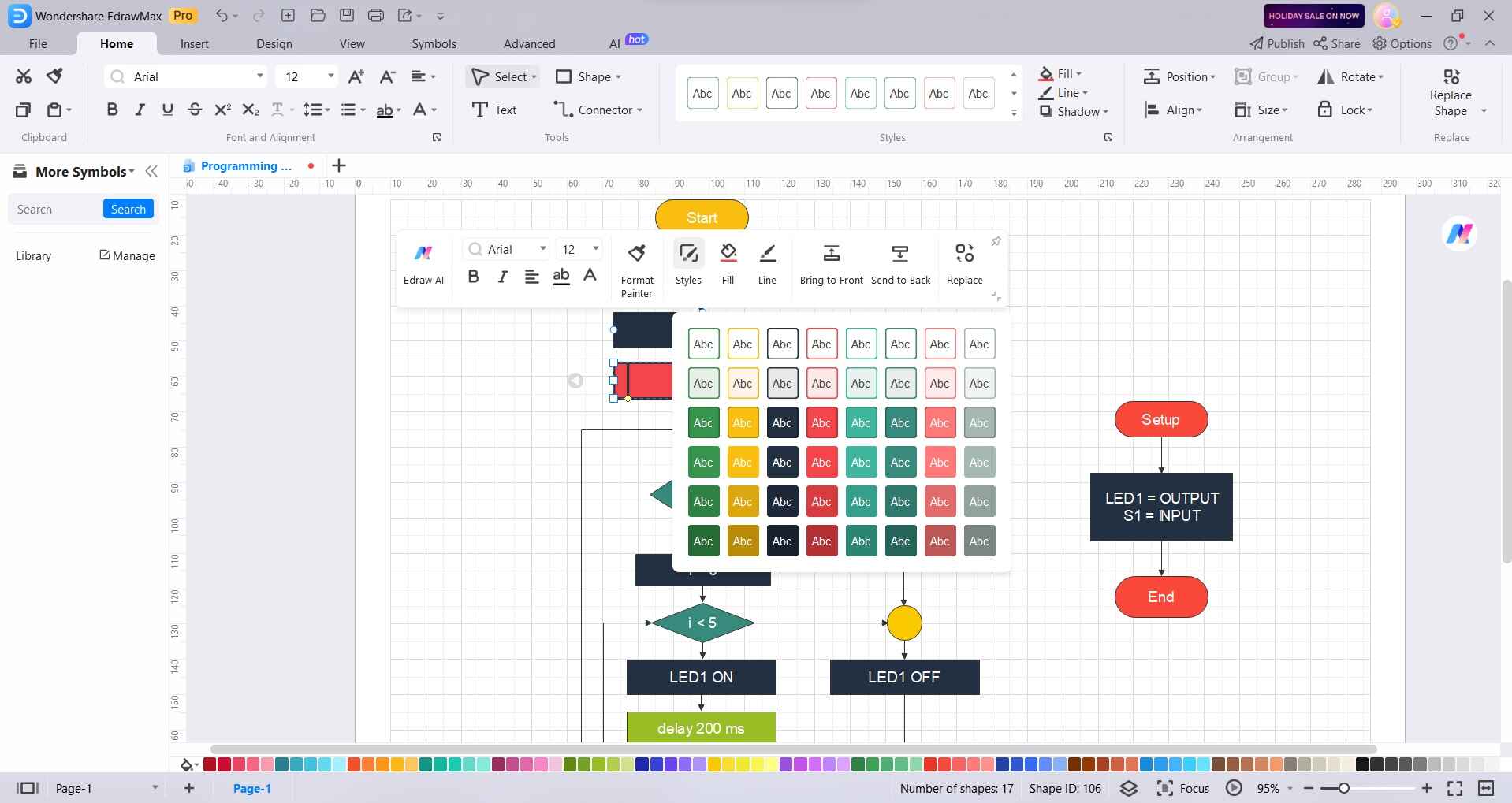
Step 5:
Once satisfied, save your flowchart within EdrawMax. You can export it in various formats (PNG, JPEG, PDF, etc.) to share or integrate it into your documentation or presentations.
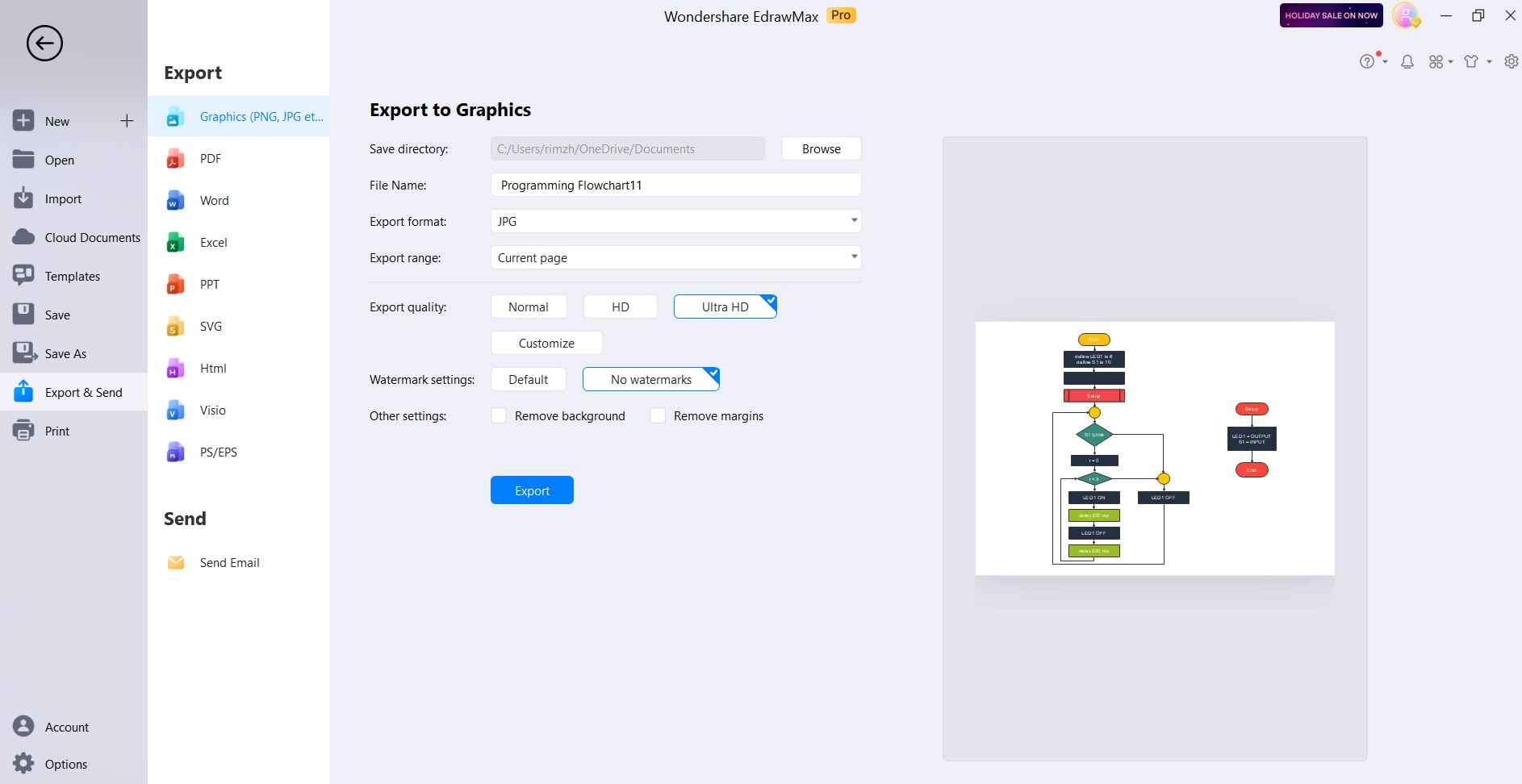
Flowcharts are an integral part of documentation in software development. EdrawMax enables the creating of detailed flowcharts to visualize queue implementations and other programs in Python. The diagrams can be integrated into technical documentation for enhanced understanding.
Conclusion
Queues are a key data structure in Python used widely for the orderly processing and scheduling of items. This guide covered core concepts like differences from stacks, implementation steps, tips for optimization, pros and cons, and creating flowcharts using EdrawMax.
Key takeaways include using deque for performance, setting bounds to limit memory usage, handling empty queues, and enabling thread safety. Visualizing program logic using EdrawMax improves documentation and maintenance.
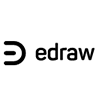