Queues are one of the most fundamental data structures used in programming. They allow for efficient first-in-first-out (FIFO) operations and have numerous applications in systems programming. In C, queues can be implemented in different ways, such as using arrays or linked lists.
In this article, we will learn about circular queue programs in C using structures. We will cover the basics of queues and the workings of a circular queue program in C.
In this article
Part 1: What is a Queue Program in C?
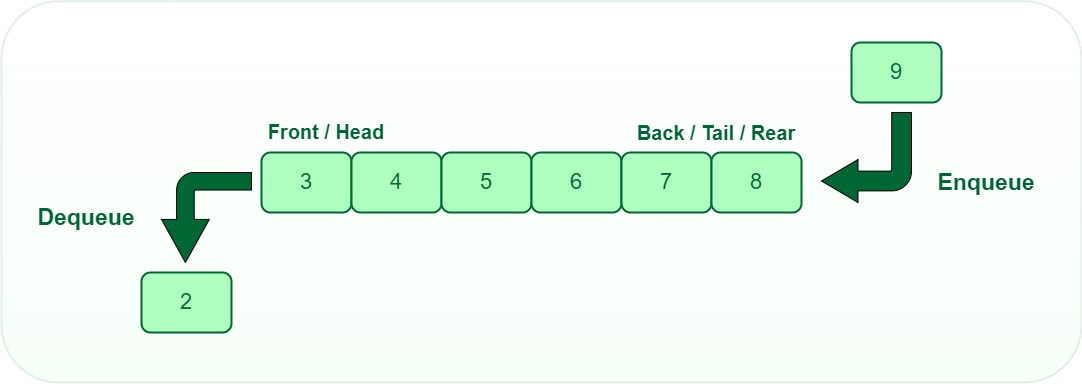
A queue is an abstract data type that serves as a collection of elements, with two main operations:
- Enqueue: Adds an element to the back of the queue.
- Dequeue: Removes an element from the front of the queue.
This makes queues FIFO data structures - first-in, first-out. The element added to the queue first will be processed first.
In C, queues can be implemented using arrays, linked lists, or other data structures. The array-based implementation is one of the simplest, keeping track of the head and tail positions to handle enqueue and dequeue operations.
Queues have many uses in systems programming:
- Task scheduling: Tasks can be queued up to execute in order.
- Buffering: Queues can act as buffers for resources like printers, network connections, etc.
- BFS graph algorithms: Queues facilitate breadth-first search traversal.
- Simulations: Queues model real-world queues and help simulate processes.
Queues in C provide an efficient, linear data structure that is indispensable when programming systems and simulations.
Part 2: Overview of Circular Queue Program in C
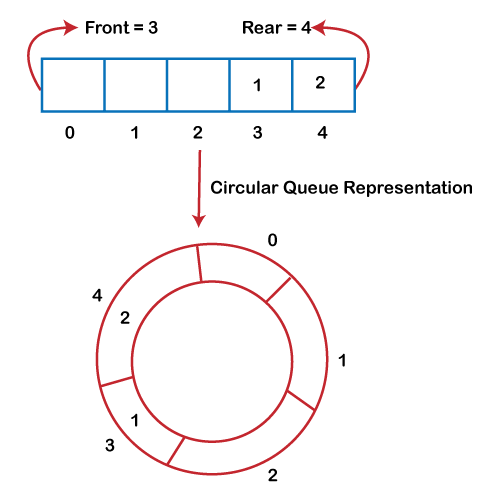
A circular queue is an optimized queue implementation that makes efficient use of allocated memory. In a normal queue, when elements are removed from the front, the space remains unused. In a circular queue, the available space cycles around continuously as elements are added and removed.
Circular queues have a fixed size or capacity and make use of modulo arithmetic to circle the queue space. The key differences from a normal queue are:
- Space is reused - when an element is dequeued, the freed space can be overwritten by new elements.
- The head and tail positions cycle around, while never actually reaching the end.
- Logic handles the wrap-around of the tail to the beginning of an array.
This results in optimal usage of allocated queue space, at the cost of additional enqueue/dequeue logic.
Circular queues have the same FIFO property as normal queues. The oldest element added to the queue is always at the head and will be dequeued first.
Part 3: Queue Program in Java and Queue Program in Python: A Comparison
Before we dive into the C implementation, let's briefly compare queues in C to queues in Python and Java.
In Java, queues can be implemented using the Queue interface, LinkedList class, ArrayDeque, or PriorityQueue. These provide O(1) enqueue and dequeue, along with additional capabilities like priority-based ordering with PriorityQueue.
In Python, queues are implemented using the queue module. You can choose between FIFO, LIFO, and priority queues. The queue includes locking for thread safety, which isn't handled by the C implementation.
Compared to Java and Python, queues in C:
- Require manual memory management when using arrays.
- Don't include advanced implementations - only FIFO behavior.
- Aren't thread safe - you'll have to handle concurrency control.
- Provide lower-level control and less abstraction.
So while C's queue doesn't have as many bells and whistles, its simplicity can be an advantage for systems programming. You still get efficient FIFO behavior with very low overhead.
Part 4: Creating a Programming Flowchart Using EdrawMax
An important part of implementing data structures like queues is designing and visualizing the programming flow. Flowcharts help outline the key steps and logic for code organization and readability.
EdrawMax is an invaluable tool for quickly creating programming flowcharts. It provides pre-made flowchart templates and symbols for all common elements like processes, decisions, inputs/outputs, loops, etc.
The drag-and-drop interface makes it easy to arrange flowchart elements, unlike drawing them manually. Automatic formatting maintains proper alignments and spacing. Flowcharts can be exported as image files or included directly in documentation.
Here are the steps for creating a programming flowchart using EdrawMax:
Step 1:
Launch the EdrawMax software on your computer. Click on "New" or "File" > "New" to start a new document. Choose the "Flowchart" category or use the search bar to find flowchart templates.
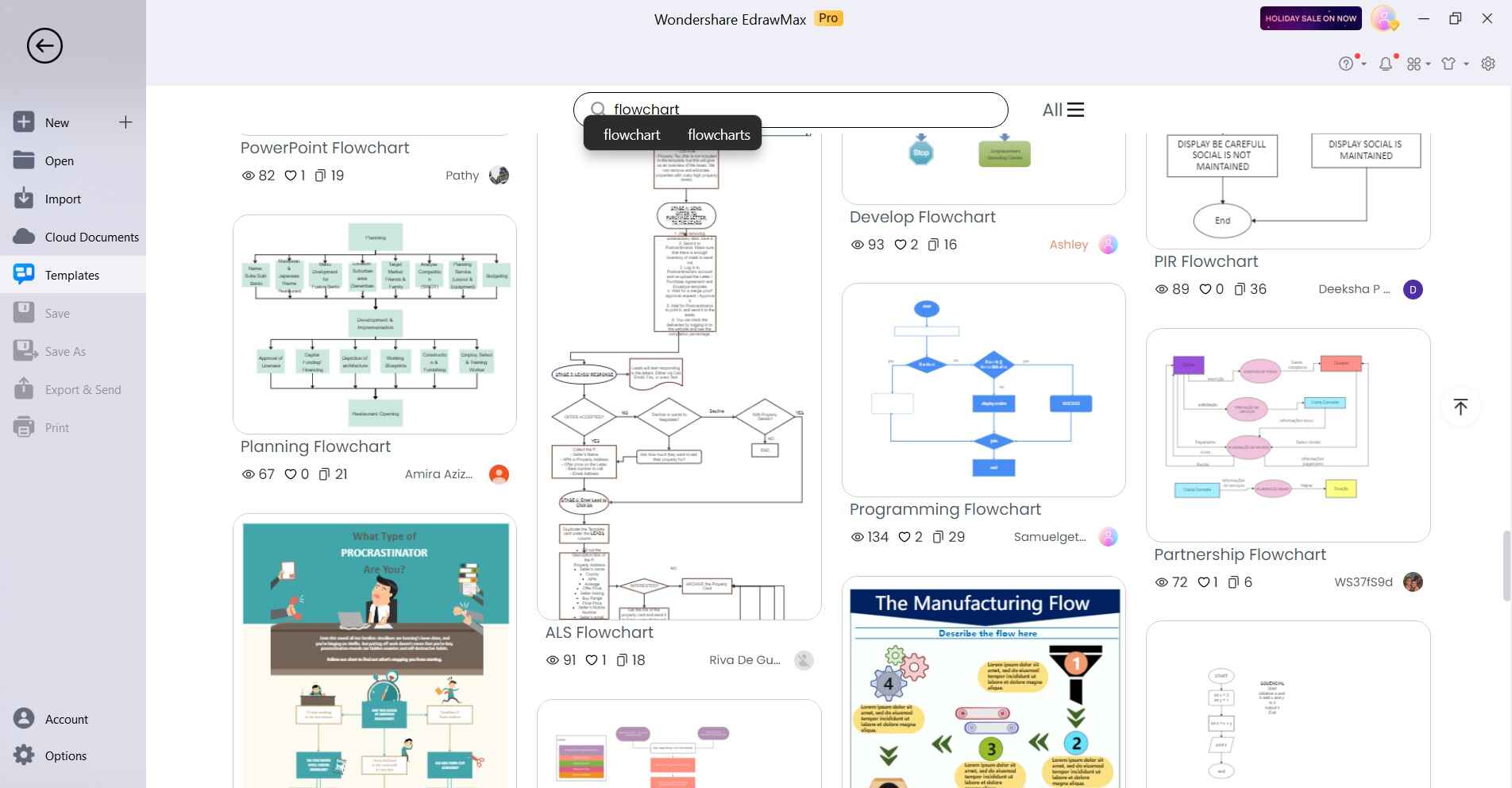
Step 2:
Drag and drop shapes from the left-hand side panel onto the canvas.
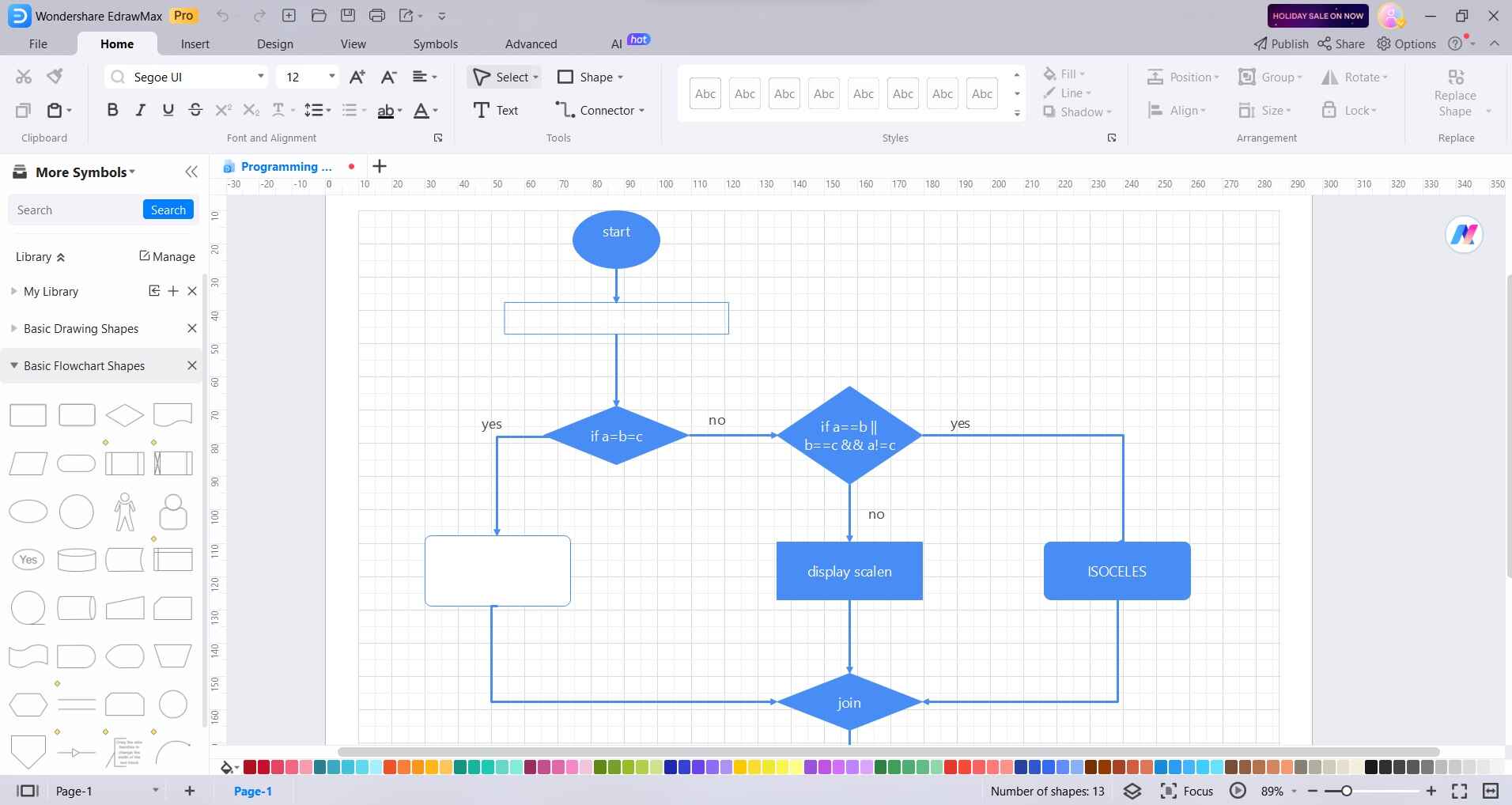
Step 3:
Click on the connector symbol on the toolbar, then click and drag from one shape to another to create connections.
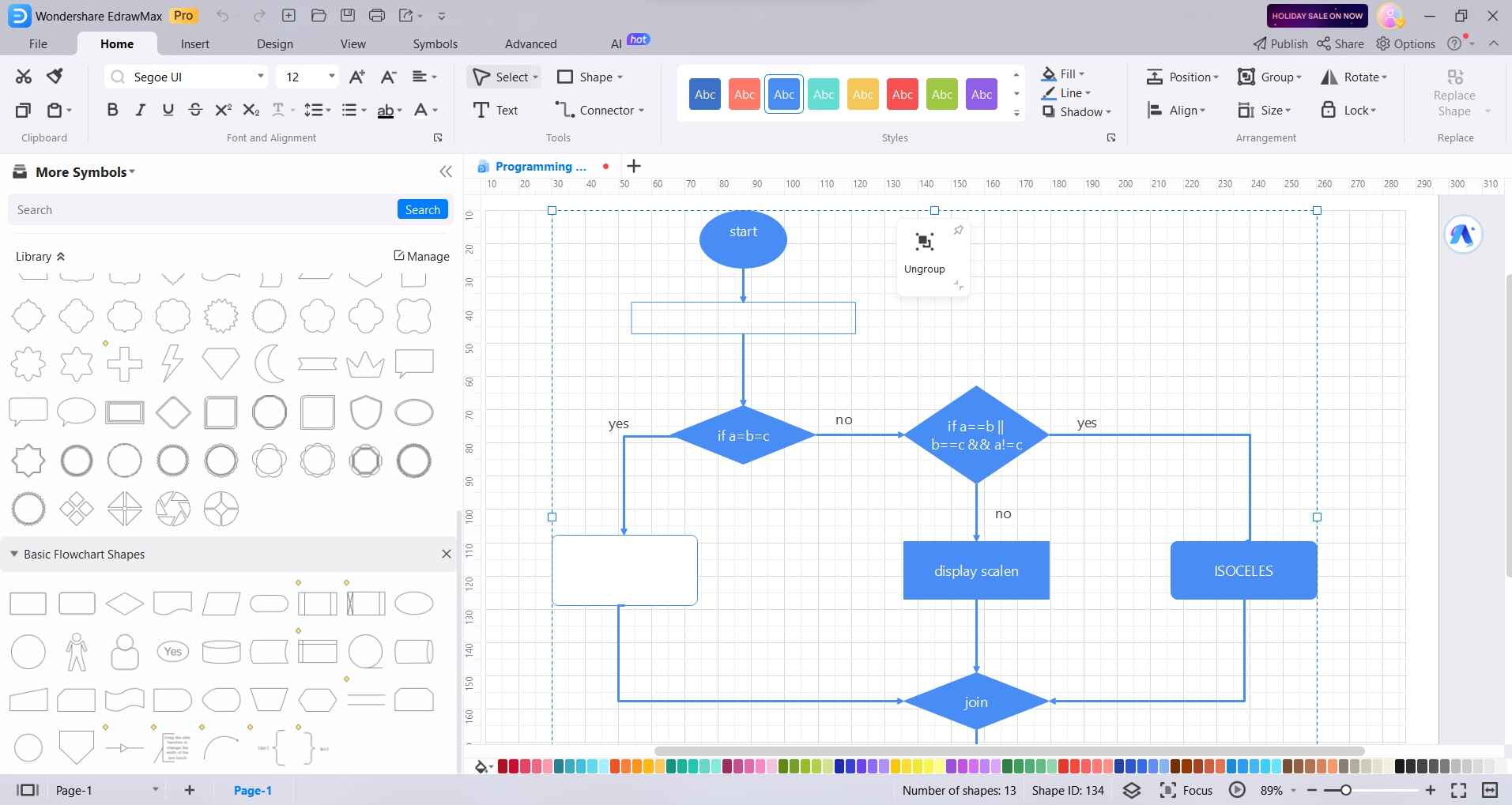
Step 4:
Change colors, font sizes, and styles to make the flowchart visually appealing and easy to follow.
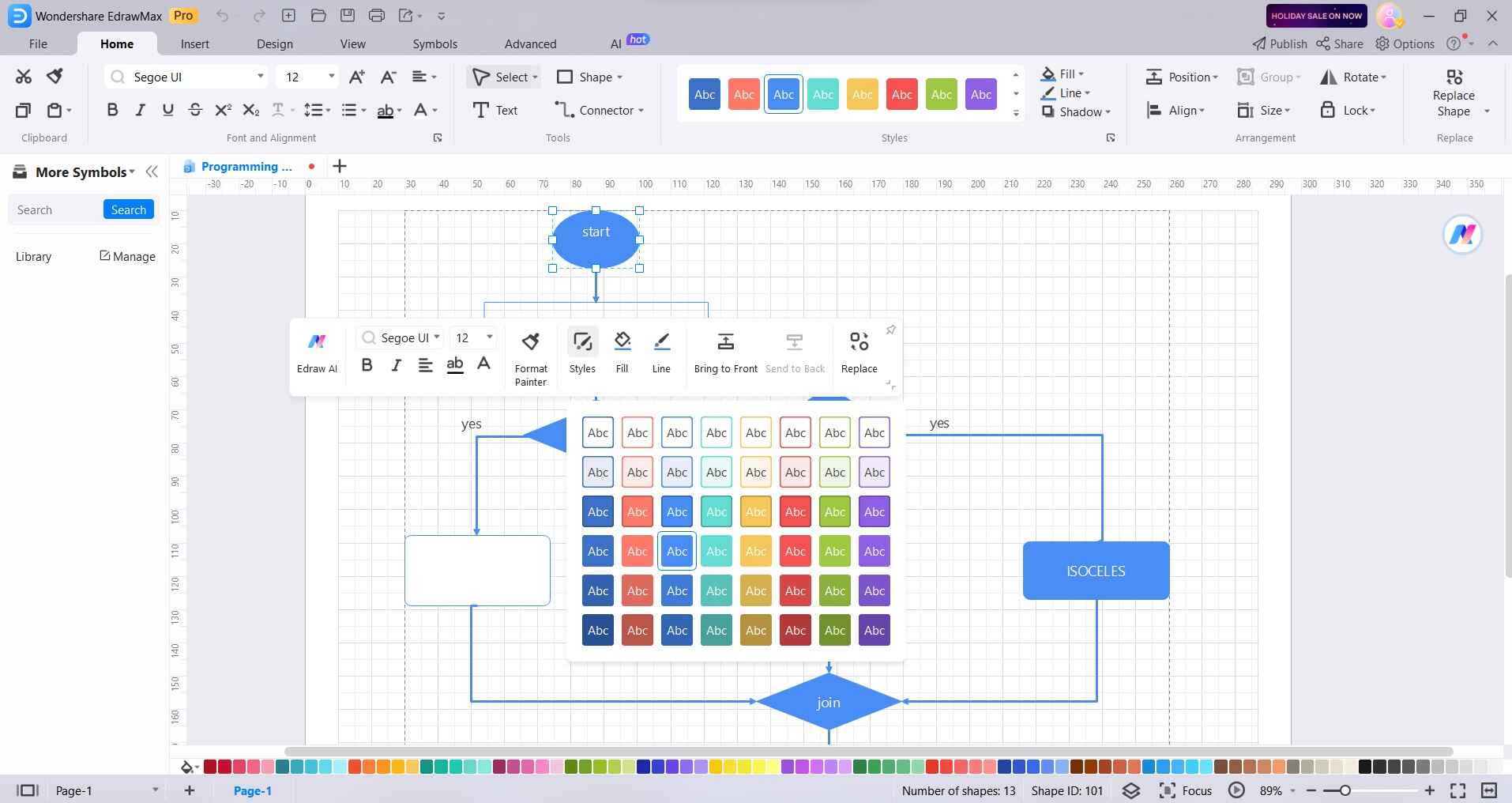
Step 5:
Once satisfied, save your flowchart in the desired format (such as PNG, JPEG, PDF) by selecting "File" > "Save As" or "Export" options.
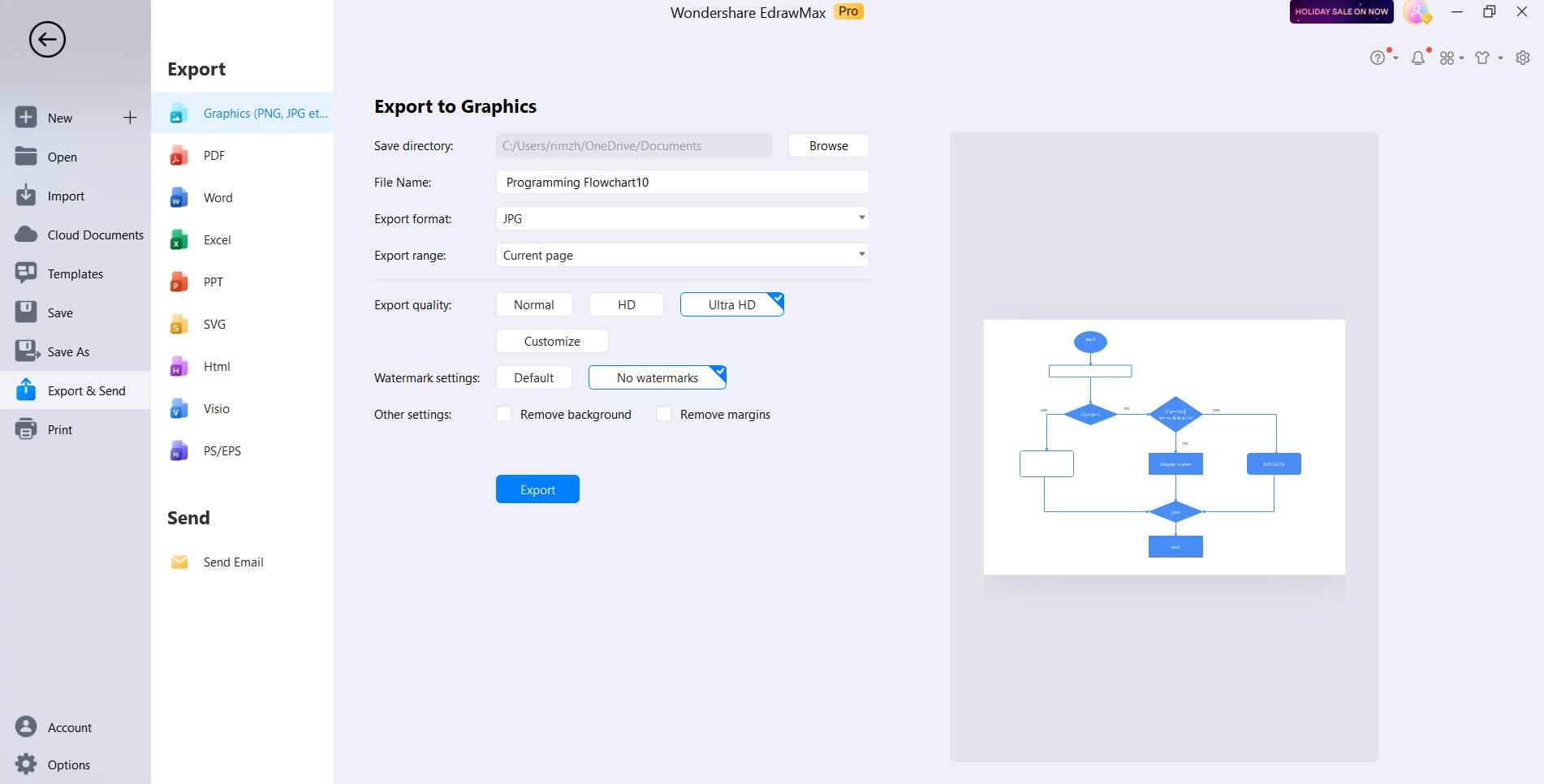
In summary, EdrawMax enables the rapid creation of professional flowcharts to design and document code. It improves development speed, quality, and organization for data structures like queues.
Conclusion
Queues are a fundamental programming concept that enables efficient FIFO data access. In C, circular queues provide a reusable fixed-size implementation by cycling through a fixed buffer.
We walked through core techniques like tracking head and tail indexes modulo capacity, handling wrap-around, and implementing overflow protection. This demonstrates a practical structured circular queue in C.
Queues in C are versatile for systems programming and are lacking in higher-level languages like Java or Python. EdrawMax accelerates development by facilitating flowchart-based design before coding data structures.
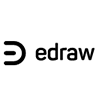