Implementing efficient data structures is crucial for writing optimized programs in any programming language. Stacks and queues are fundamental linear data structures used extensively across various applications.
This article provides a comprehensive guide to efficiently implementing a stack code structure in C programming language.
In this article
Part 1: What is a Stack C Code Structure?
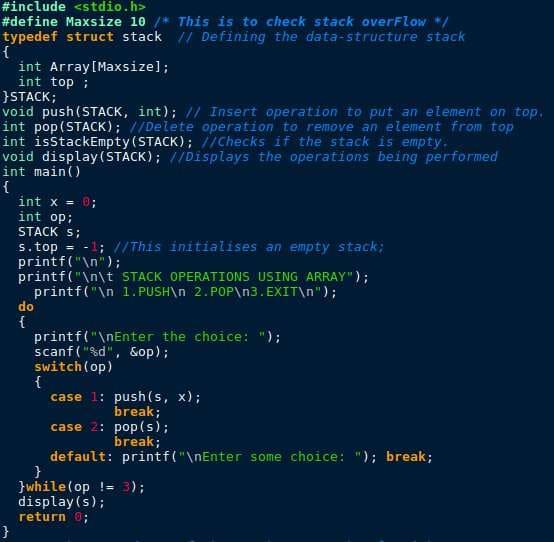
A stack refers to an abstract data type that serves as a collection of elements, with two main operations - push and pop. The push operation adds an element to the top of the stack while the pop operation removes the most recently added element.
Stacks follow the Last In First Out (LIFO) order, meaning the most recently added element is removed first. In C, stacks can be implemented using arrays or linked lists. Using arrays is easier and more efficient to code.
Part 2: Steps in Implementing a C Code Structure
- Include header files: Standard C header files like
#include
,#include
, and#include
should be included which contain definitions for input/output functions, dynamic memory allocation, and console input/output. - Define stack properties: The maximum size of the stack must be defined along with a structure representing stack elements. These include an integer variable 'top' to track the topmost element and an array to store elements.
- Implement push operation: This adds a new element to the top of the stack. First, check if the stack is already full then display an error. If not, increase the value of 'top', add a new element at index 'top' in an array, and display a confirmatory message.
- Implement pop operation: This removes the topmost element from the stack. Check if the stack is empty, then show an error. If not, decrease the 'top' variable, remove the element at that index from the array, and display the removed element's value.
- Additional functions like displaying stack elements and checking empty/full stack can also be added.
Part 3: Overview of a Python Code Structure
Unlike C, Python supports both stack and queue natively so no fixed size or data structure needs to be defined explicitly. The list data type can implement basic stack operations conveniently using append()
and pop()
methods. append()
adds an element to the end of the list (top of stack) while pop()
removes the element from the end of the list (top of stack). So just declaring a list implements a dynamic stack.
Part 4: Major Difference Between C Code Structure and Python Code Structure
The major differences include:
- C requires explicitly defining the stack properties like maximum size and data structure representations which Python handles dynamically.
- Memory management is manual in C while Python handles it automatically.
- Stack operations like push/pop need to be coded in C but readily available in Python lists via append/pop methods.
- C code is prone to risks like buffer overflow or segmentation faults due to memory access errors while Python checks boundaries automatically.
- C provides more control, predictability, and efficiency over Python which is more programmer-friendly.
Part 5: What is a Binary Search Tree?
A binary search tree (BST) is a rooted binary tree data structure that maintains the property where the value in each node must be greater than or equal to any value stored in the left sub-tree, and be less than or equal to values in the right sub-tree.
Part 6: Creating a Programming Flowchart Using EdrawMax
EdrawMax is a cross-platform diagramming and charting software providing ready-made templates for a wide selection of diagrams. Flowcharts make complex program logic highly readable and easy to comprehend. To efficiently implement any data structure in code, first planning the high-level steps in a flowchart helps.
EdrawMax allows quickly dragging and dropping flowchart symbols and modifying connections easily to modify flowcharts on the fly. The intuitive UI coupled with thousands of templates and symbols across categories makes EdrawMax the go-to tool for programming flowcharts.
Some key benefits are:
- Intuitive drag-drop interface and editors to simplify modifications.
- Supports Flowcharts, UML diagrams, Network Diagrams, and more.
- Provides advanced customization through size, color, images, and more.
- Integrates with Microsoft Word, Excel, and PowerPoint.
- Shareable online via customizable share links.
Here are the steps for creating a simple programming flowchart using EdrawMax:
Step 1:
Launch the EdrawMax application on your computer. Look for the flowchart templates or choose "File" > "New" > "Flowchart" to start a new document specifically for a flowchart.
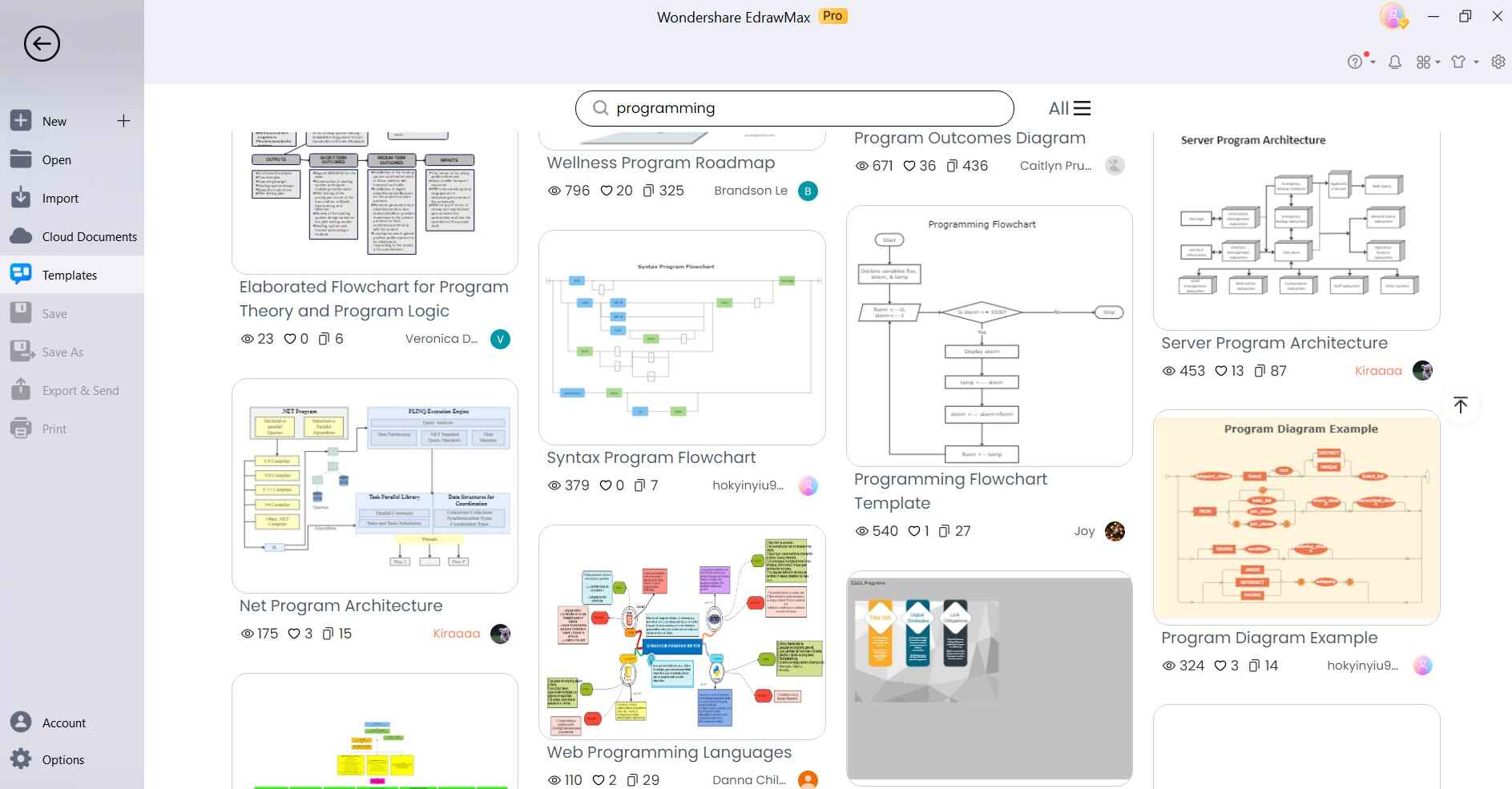
Step 2:
Use the toolbar or the shape library to add shapes for different elements of your flowchart. Common shapes include Start/End, Process, Decision, Input/Output, etc. Drag and drop these shapes onto your canvas.
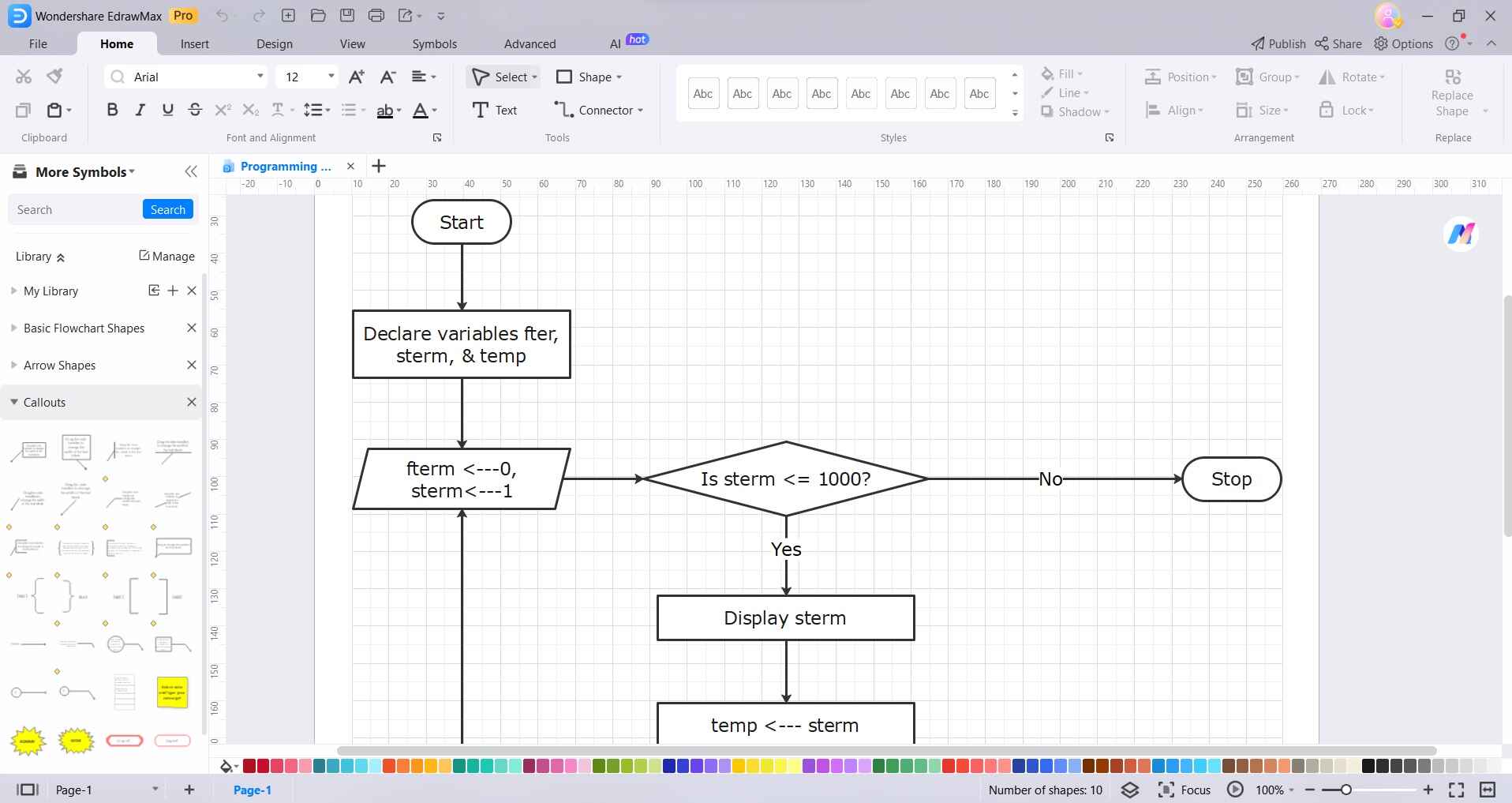
Step 3:
Double-click on the shapes to add text and labels. Describe the actions or processes associated with each shape in your flowchart.
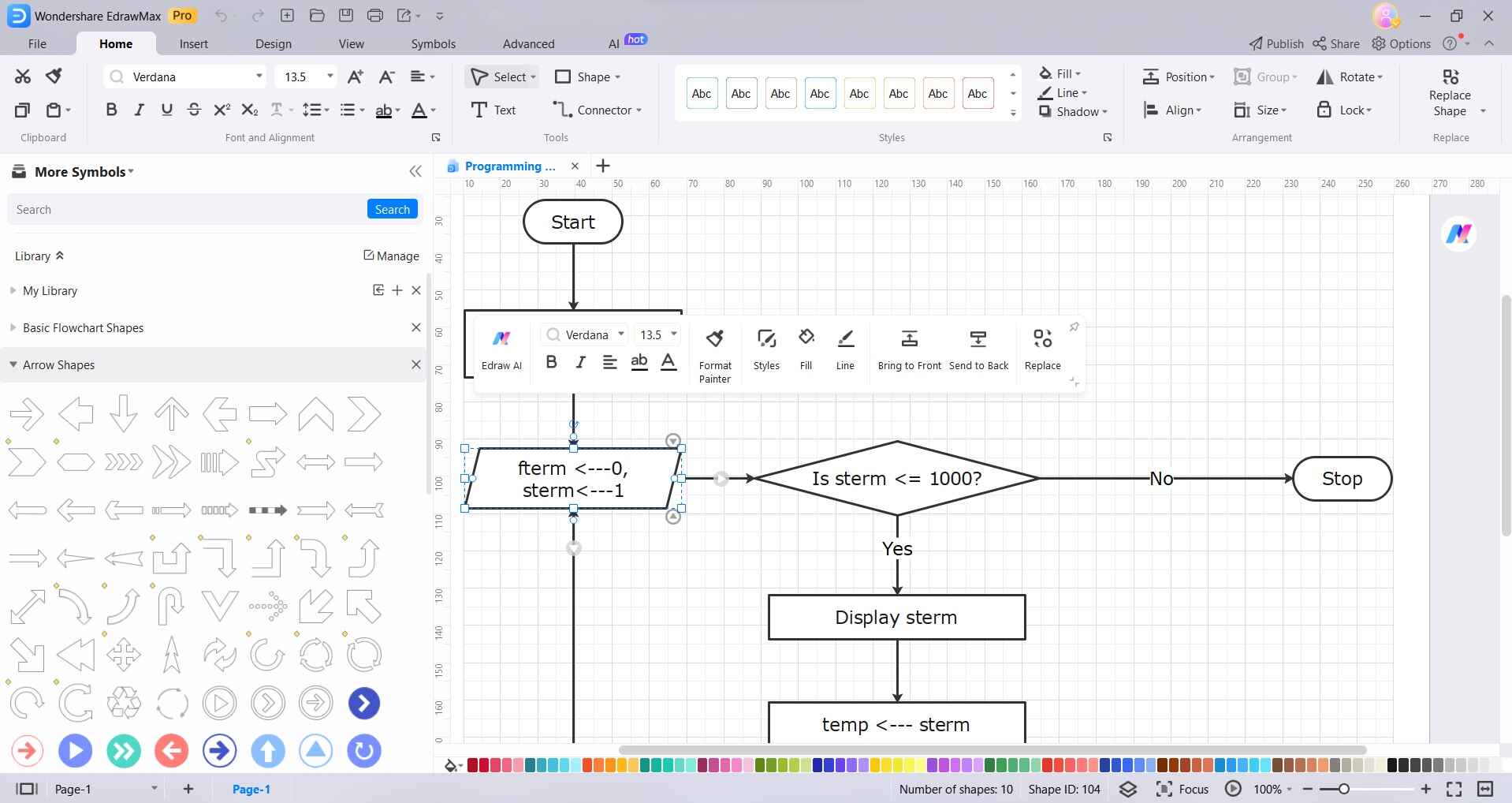
Step 4:
Change colors, sizes, fonts, etc., to make your flowchart more visually appealing and understandable.
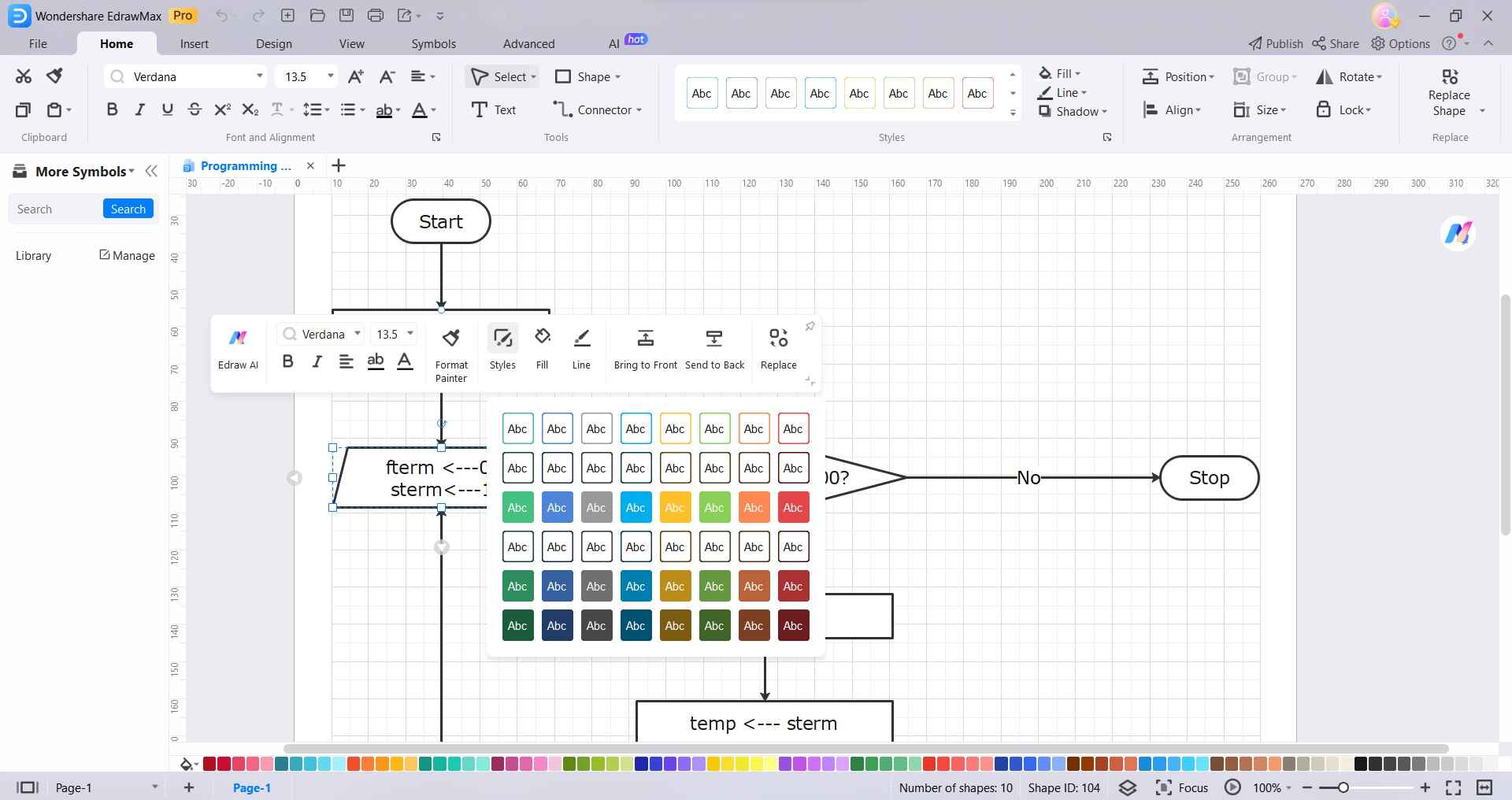
Step 5:
Once you're satisfied, save your flowchart in your desired format (EdrawMax file, PDF, image, etc.). EdrawMax allows you to export in various file types for easy sharing and use.
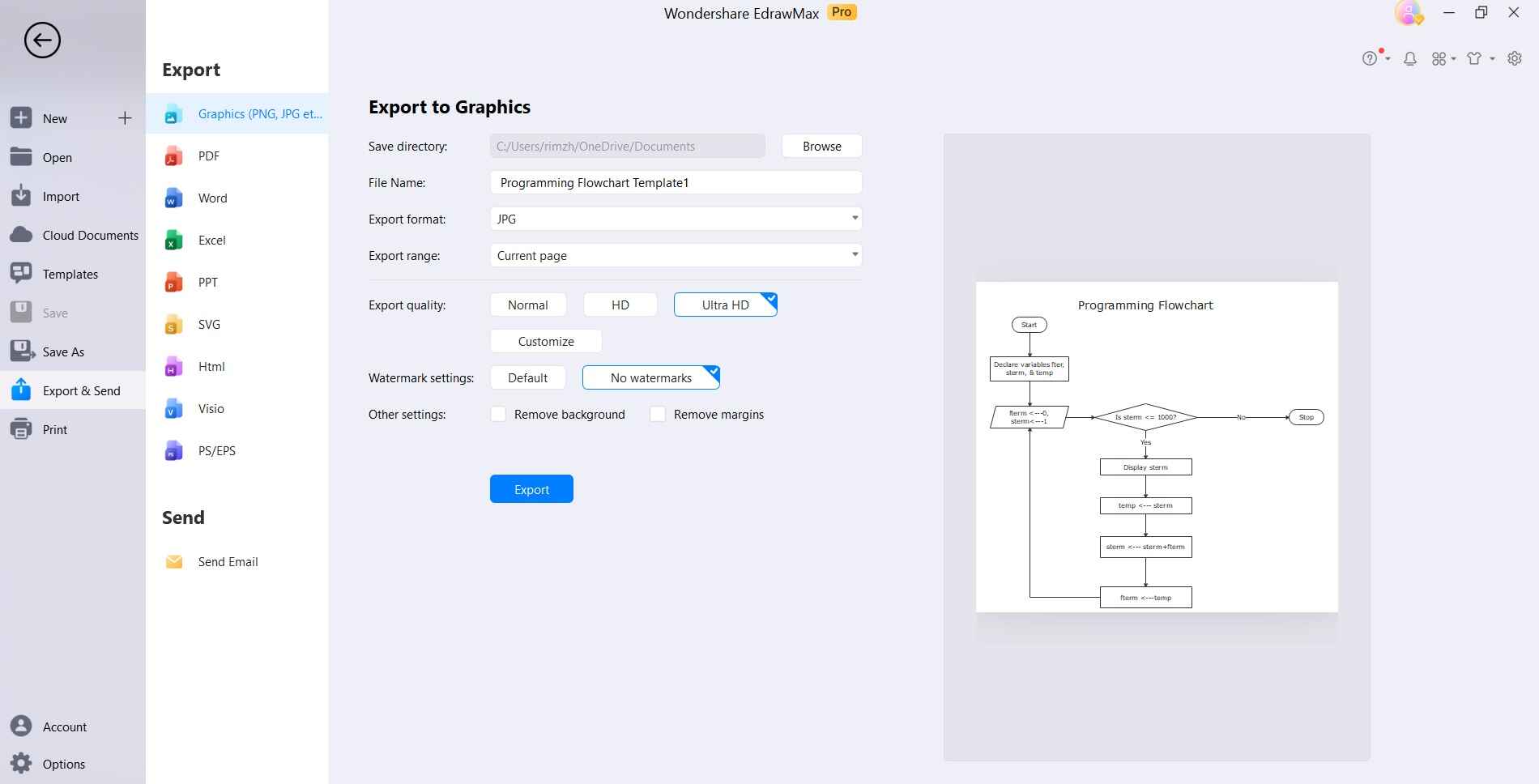
And that’s it! EdrawMax offers many tools and options to create intricate and detailed flowcharts for various programming processes.
Conclusion
Efficient data structure implementation is key for optimizing software application performance and stability. Through this guide, we covered the process of implementing stacks in C, differences from Python lists, an overview of binary search trees, and using EdrawMax to plan program flow.
Proper analysis of use cases, planning flowcharts, and structured implementation following these best practices will ensure the building of efficient and bug-free programs.
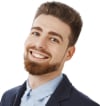