Python Binary trees are fundamental data structures in computer science, where each node can have up to two child nodes. They enable efficient implementation of algorithms through their recursive nature.
In this article, we will explore binary trees by looking at their structure, walking through how to create one in C, examining complex Python Binary Trees, and using diagrams to understand them better.
In this article
Part 1: What is a Binary Tree Program in C
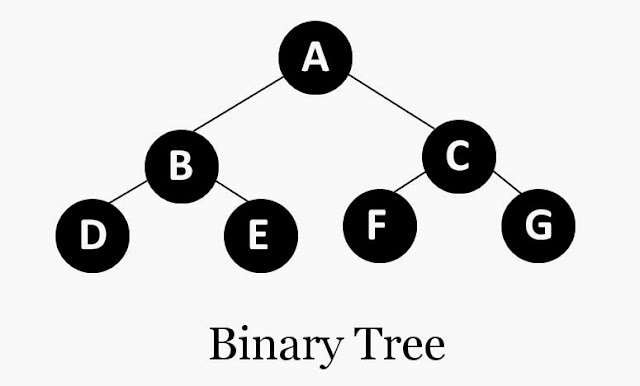
At its core, a binary tree is a hierarchical tree data structure where every node has a maximum of two children, referred to as the left and right child. Unlike a linear structure like a linked list, the recursive tree structure allows efficiently representing and implementing recursive algorithms.
In the C programming language, each binary tree node needs to store a data element, as well as pointers to potential left and right child nodes. The data elements could be integers, strings, or custom structs. The pointers allow linking together the nodes recursively to enable traversing the tree.
Some key characteristics of binary trees are:
- Recursive Structure: Nodes can point to child nodes to form recursive hierarchies
- Linked with Pointers: Nodes store pointers to left and right children
- Restricted Degree: Nodes have a maximum of two child nodes
- Unordered: Does not require sorting or ordering of node values
- Useful for Inserts/Deletes: Quick to insert and delete nodes
When making a binary tree in C, key tasks include defining the node structure to store data and links and tree operations to insert, find, or print nodes by recursively traversing the structure.
Part 2: Steps to Create Binary Tree Program
Here are the key steps to create a binary tree data structure in C:
- Define Node Structure: Represents an individual node with data and links.
- Initialize Tree Pointer: Create root node pointer, initially set to null.
- Insert Node Function: Recursively inserts a new node into the tree.
- Search Node Function: Recursively searches for a node by value.
- Print/Traverse Function: Recursively prints or processes tree nodes.
- Delete Node Function: Deletes nodes recursively as needed.
- Main Driver Code: Test core tree functionality.
First, we define the Node structure to store integer data and the left and right child pointers.
Next, initialize an empty root node pointer. This will become the entry point to our tree.
We can then implement key operations like inserting new nodes into the tree structure recursively and writing recursive search and print functions to match. Delete functionality can also be added to remove nodes from the tree.
Finally, a main driver function ties things together by initializing a new tree, testing insertion, printing out elements, searching, and more.
With these foundations, complex binary trees can be implemented in C for diverse projects.
Part 3: Overview of a Balanced Binary Tree Python
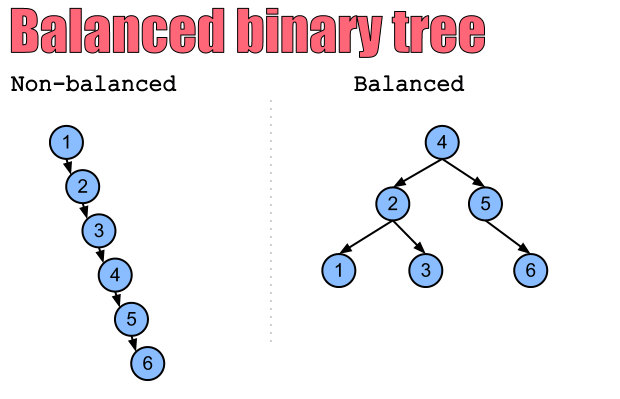
While basic binary trees are useful, balanced binary tree variants exist to improve performance through enforced structure constraints.
One example is an AVL or height-balanced binary tree. This augments the binary tree by enforcing that the heights of left and right subtrees of every node differ by at most 1. By balancing heights, lookup and insert efficiency is improved.
Python provides a good high-level language to implement these more complex balanced binary tree algorithms clearly and concisely, hiding lower-level pointer mechanics.
By augmenting binary trees with self-balancing capabilities and additional optimizations in this way, efficiency and usage possibilities are expanded significantly. Python enables directly expressing these improved algorithms at a higher level.
Part 4: Create a Binary Search Flowchart Using EdrawMax
Along with textually coding binary trees, creating accompanying visual diagrams is invaluable for thoroughly understanding, debugging, and presenting the structures and processes involved.
EdrawMax is cross-platform diagramming software that makes visually mapping out binary trees simple and intuitive through features like:
- Drag and drop interface to easily add and connect nodes.
- Templates for flowcharts, trees, algorithms, and more.
- Automatic layout alignment and restructuring abilities.
- Professional-looking output with just a few clicks.
This enables the drafting of elegantly laid out binary tree diagrams that greatly complement your code.
Here are the steps to create a simple binary tree diagram using EdrawMax:
Step 1:
Launch EdrawMax on your device. Look for the "Flowchart" category or search for "binary search flowchart" in the template search bar. Choose a blank flowchart template or one that closely resembles what you want.
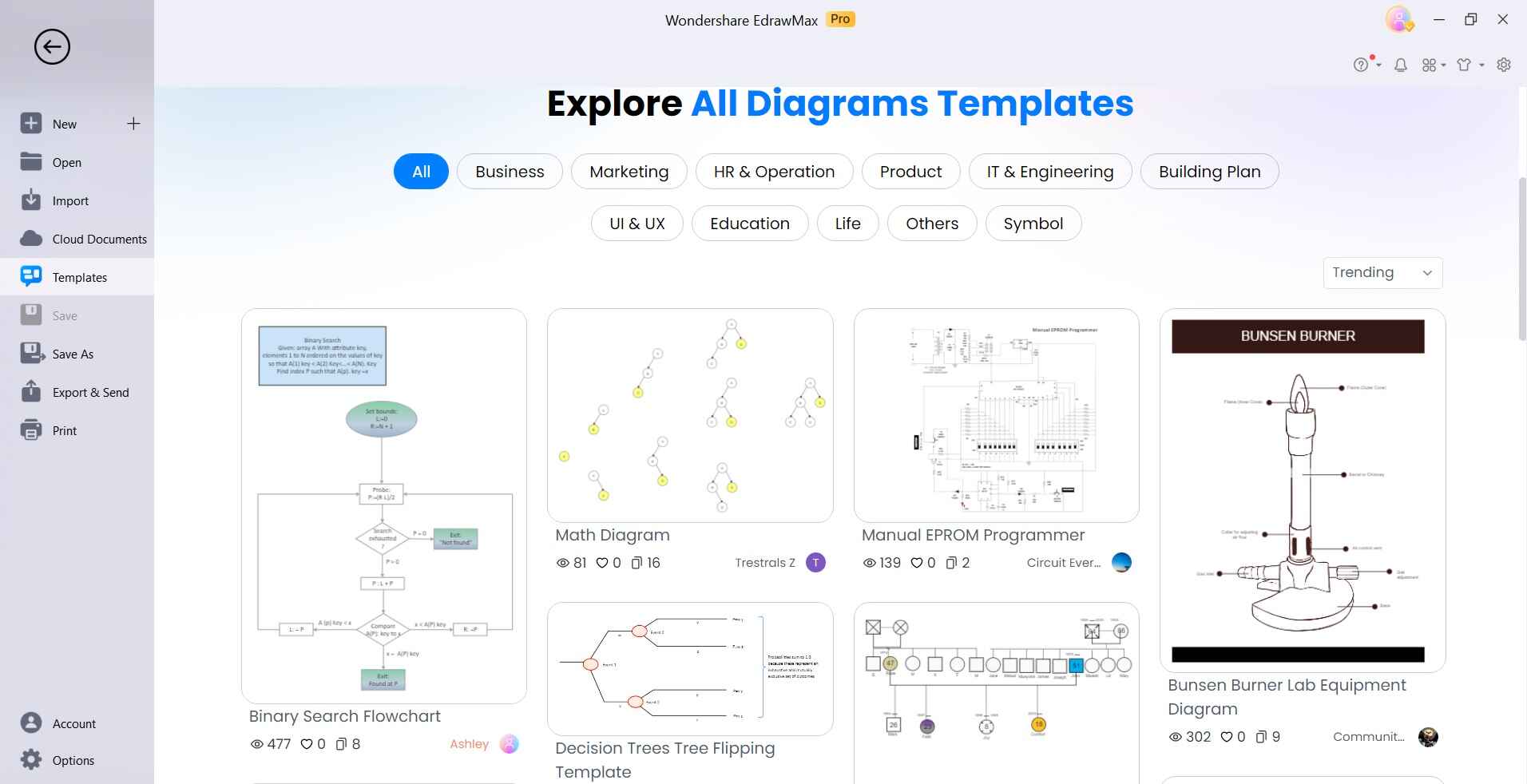
Step 2:
Drag and drop shapes for the flowchart elements. For a binary tree search, shapes like rectangles (for processes or steps), and arrows (for connections) will be essential.
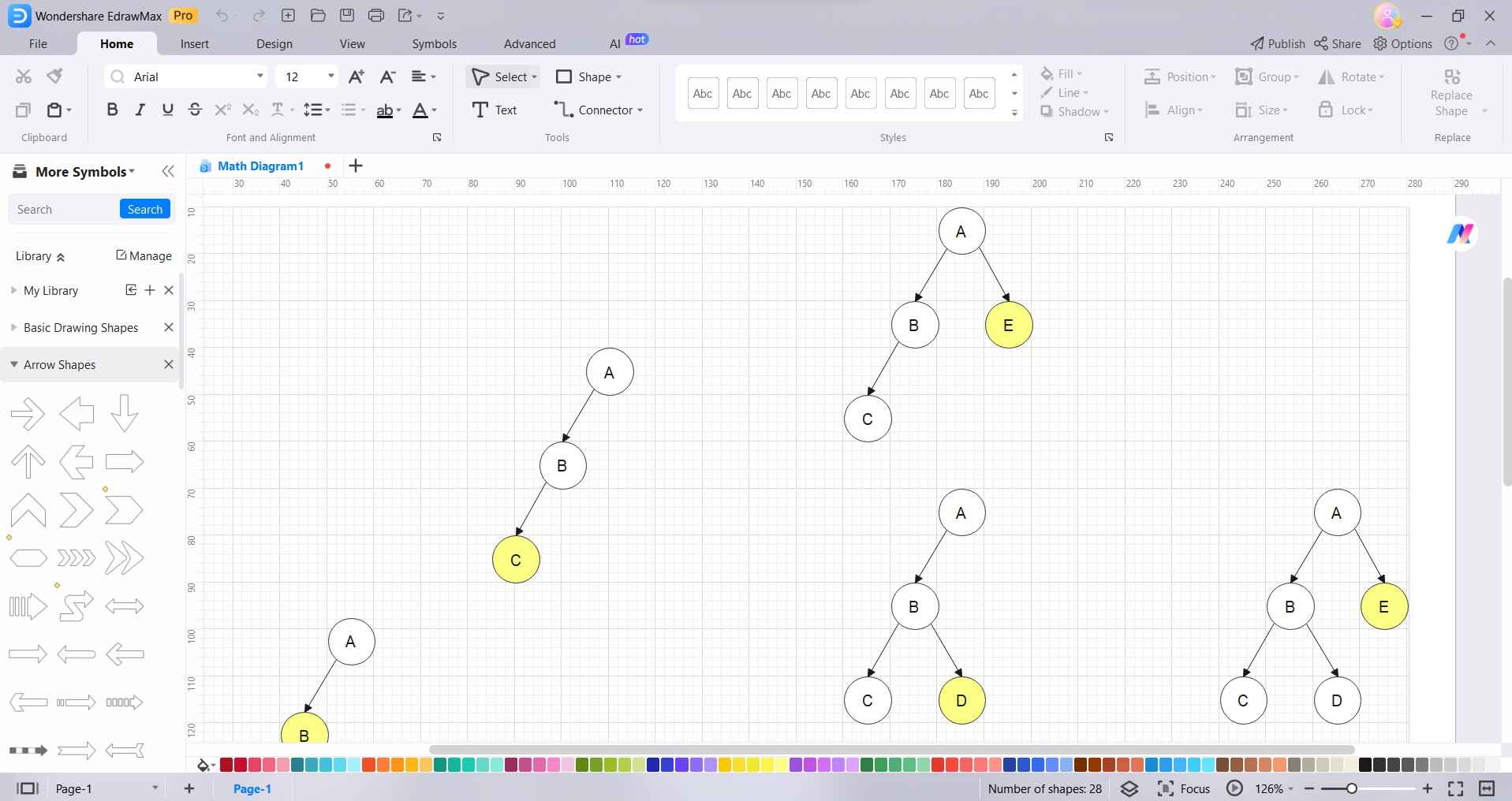
Step 3:
Use arrows or lines to connect the shapes in the logical sequence of the binary tree search process. Add text to label each shape or process.
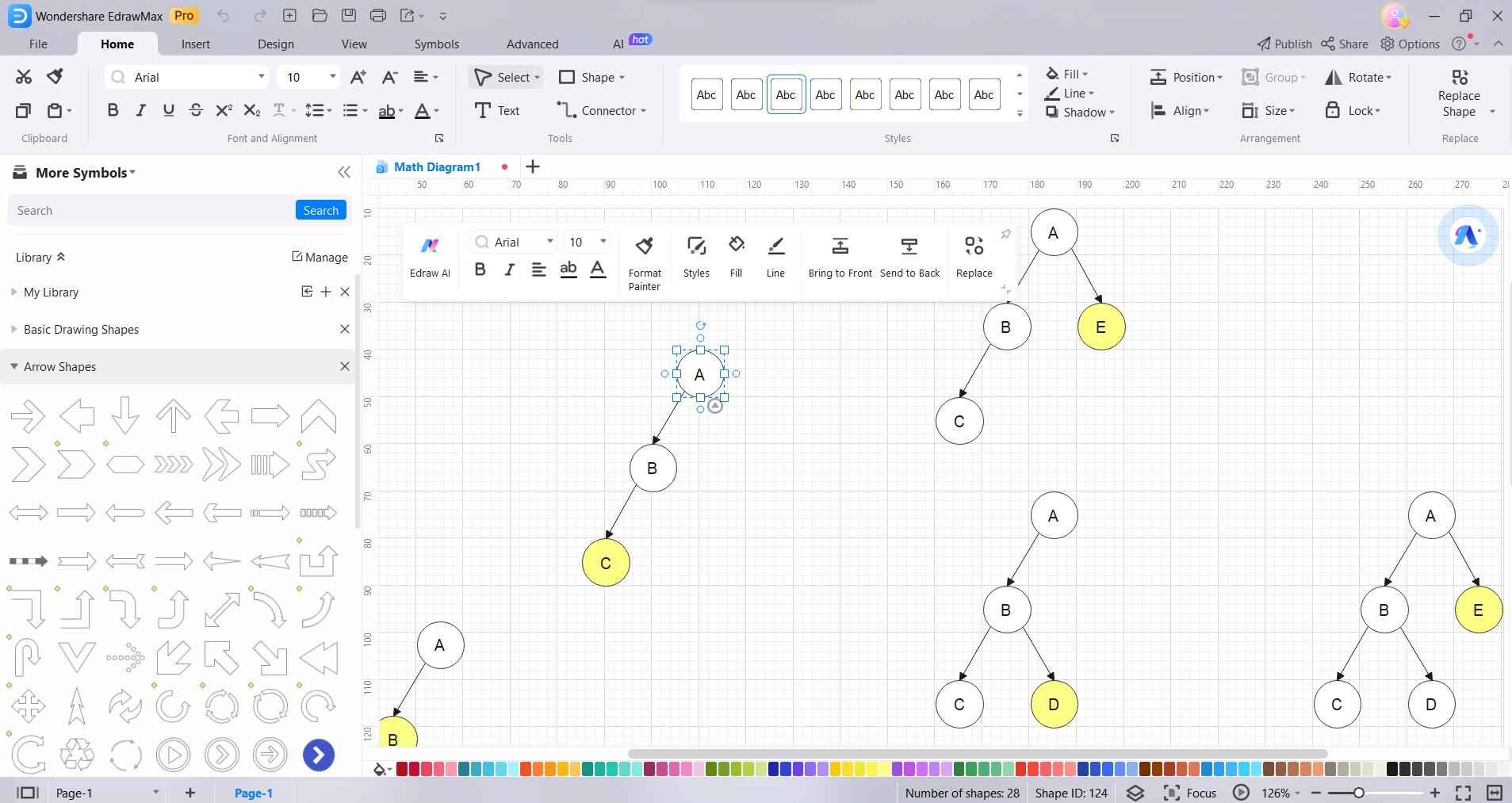
Step 4:
Click on any entity you wish to modify, and select “Styles”. EdrawMax offers various shapes, styles, and customization options to create detailed and visually appealing flowcharts.
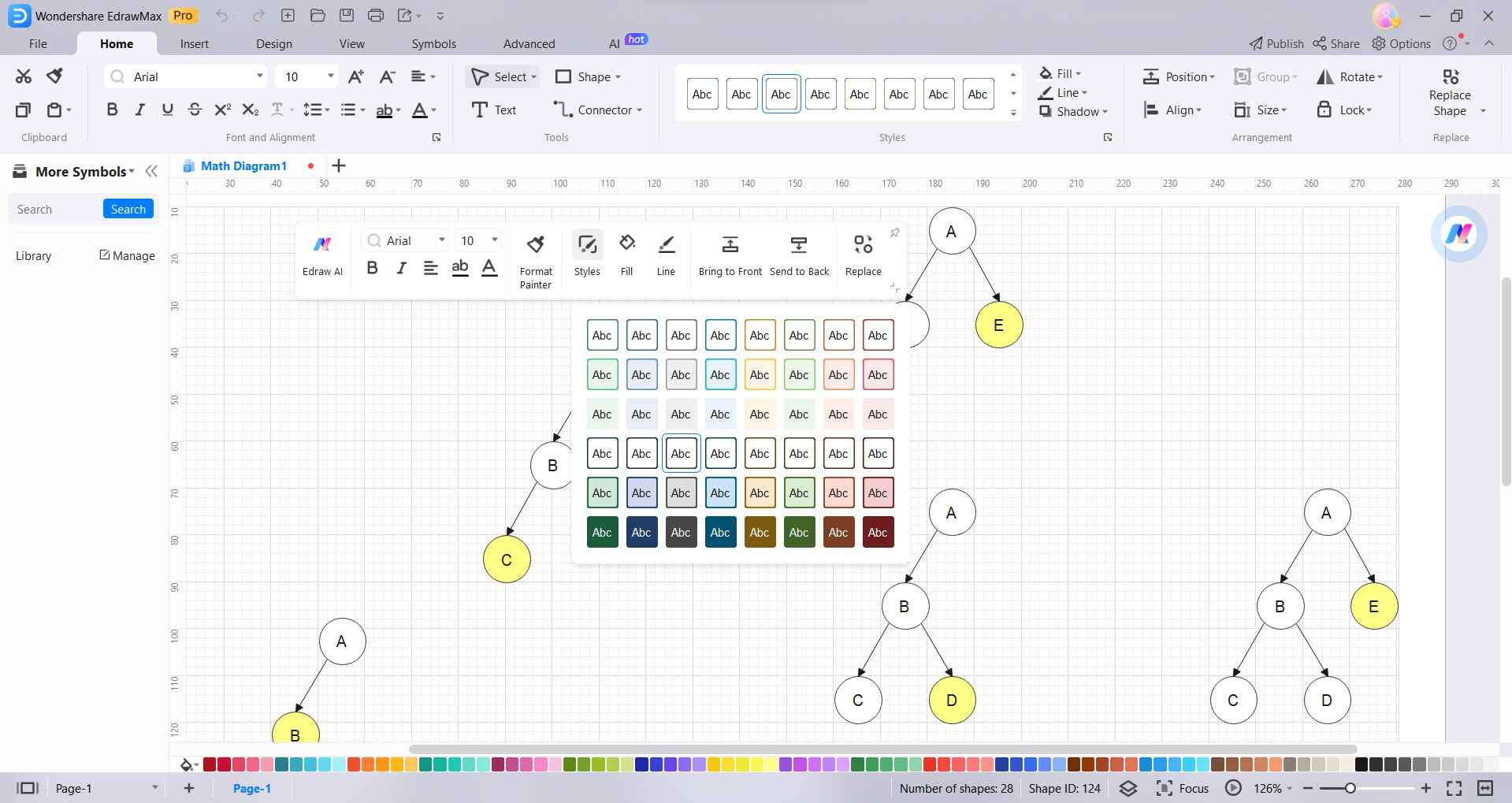
Step 5:
Once completed, head to File > Save to save your flowchart within EdrawMax or export it to the desired file format.
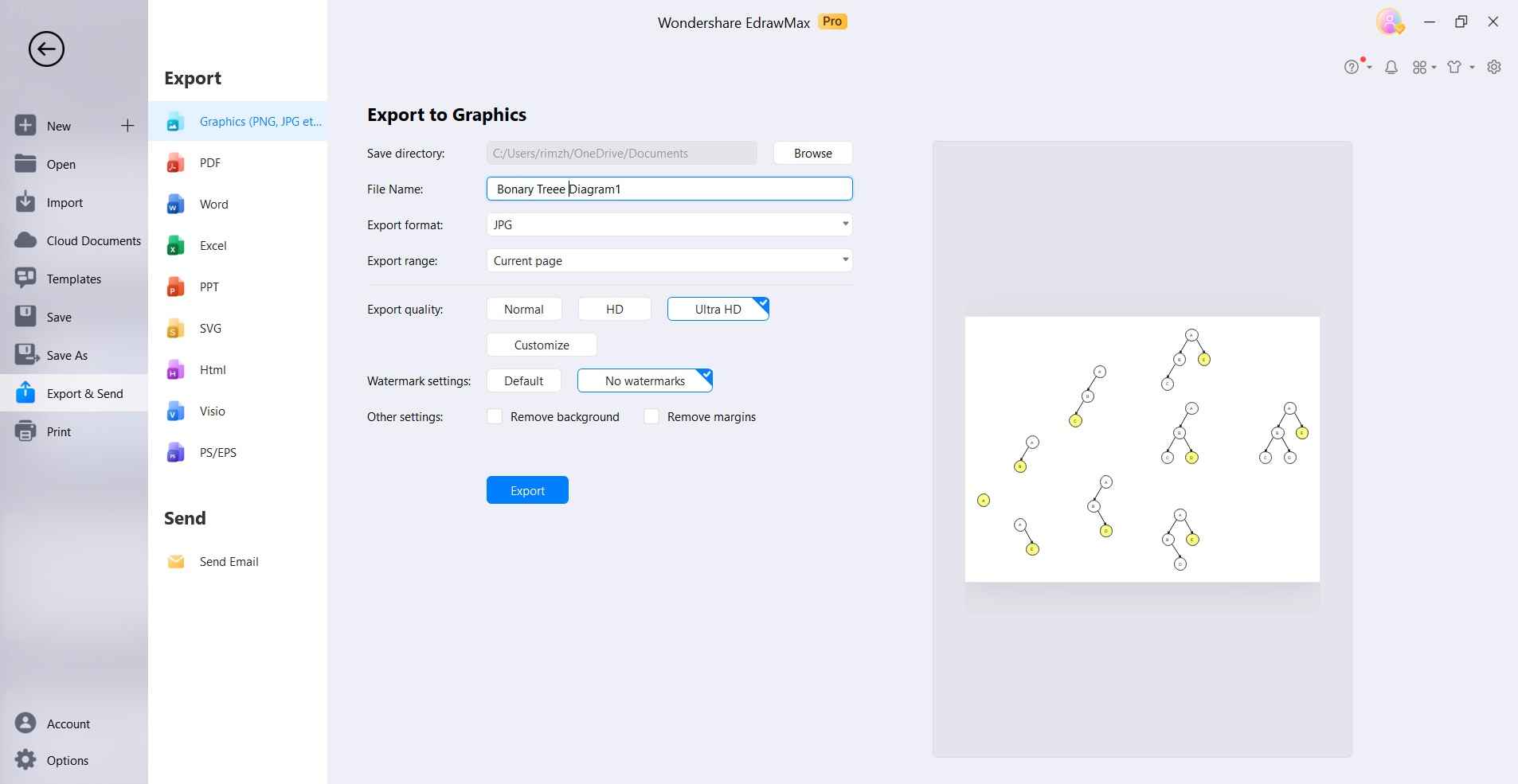
So, yes! EdrawMax is indeed a great tool for simplifying binary tree designs!
Conclusion
In this article, we covered fundamental aspects of binary trees, including their recursive structure, how to implement operations like search and insert in code, complex self-balancing variants, and how tools like EdrawMax can bolster comprehension through diagrams.
Whether just starting out learning or implementing sophisticated systems, internalizing these binary tree basics is essential for advancing programming prowess.
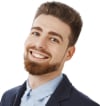