Structs in C++ allow developers to create custom data types by grouping related data into a single unit. Initializing structs properly is key to leveraging their full capabilities.
This article will provide a comprehensive overview of C++ struct initialization best practices. It starts with the fundamentals and builds up to more advanced examples and techniques for getting the most out of structs in C++ programming.
In this article
Part 1: What is a C++ Struct
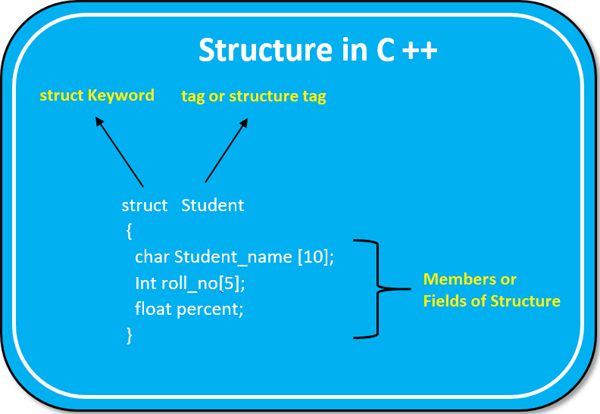
A struct in C++ is a user-defined data type that enables grouping different data types into a single data unit. Structs allow developers to represent complex data structures, acting as containers for diverse data types.
Key attributes that differentiate C++ structs from classes include:
- By default, members and base classes are made public.
- Structs can't define constructor or destructor member functions.
- Structs don't support inheritance between struct types.
In essence, structs trade features like encapsulation and inheritance for an emphasis on data aggregation and ease of access.
Part 2: Key Points about C++ Structs
Here are some key points worth noting about C++ structs:
- Value Semantics: C++ structs exhibit value semantic behavior rather than reference semantics. This means when struct instances are copied or assigned, the data is copied rather than having the instances point to the same data.
- Default Member Initialization: Members of a C++ struct get default-initialized if you don’t explicitly define a value. Value initialization rules apply based on the member data types.
- Stack Allocation: Struct instances get created on the program stack rather than the heap if they are local variables. This makes access very fast.
- Composition and Aggregation: Structs shine for composition/aggregation scenarios where you want to bundle related data for encapsulation.
- Compatible Data and Methods: You can define both data members and member functions within C++ structs. Methods can manipulate the data.
Part 3: Steps in C++ Struct Initialization
Properly initializing C++ structs is critical for leveraging their benefits. Here are the key steps:
- Define the structure with member fields.
- Declare an instance variable of the struct.
- Initialize members upon instantiation.
- Set values dynamically at runtime.
Choices for initialization include:
- Member initializer list
- Assignment after declaration
- Default member initialization
- Custom constructors
Proper initialization ensures that declared struct instances hold meaningful data as intended.
Part 4: Types of Structure and Classes in C++
C++ supports two main custom data types – native struct types and class types. Key differences include:
- Access controls: Members of structs are public by default while class members are private.
- Intended purpose: Structs emphasize data bundling while classes focus on encapsulation and methods.
You can initialize both types in similar ways. However, classes offer more flexibility and control through access modifiers and constructors.
In addition, C++ has class templates where you define the behaviors and members generically. This allows the creation of structs and classes of different concrete data types from a single template.
Part 5: Struct C++ Example for Better Understanding
Let’s look at an example C++ struct called Tree that bundles relevant data about a tree together:
struct Tree {
string name;
int heightFt;
int ageYrs;
void growHeight(int h) {
heightFt += h;
}
};
In this example, we defined data members to store the tree’s name, height, and age. We also defined a member function growHeight()
that takes a height value and increments the heightFt
field.
Usage could be:
Tree oak;
oak.name = "Northern Red Oak";
oak.heightFt = 5;
oak.ageYrs = 10;
oak.growHeight(3); // Height now 8
This demonstrates bundling relevant data together in a custom struct, encapsulating access through members while supporting value semantics.
Part 6: Create a Programming Flowchart Using EdrawMax
EdrawMax is professional diagramming software that makes it easy to create programming flowcharts to document code logic and structure.
Benefits of using EdrawMax for struct initialization flow include:
- Intuitive drag-and-drop interface.
- Multiple flowchart templates.
- Export flowcharts as images or PDF docs.
- Share and collaborate on diagrams.
By mapping out struct initialization in EdrawMax, you can visualize the member declaration order, understand initialization dependencies, catch issues early, and improve maintainability.
Here are the steps to create a programming flowchart using EdrawMax:
Step 1:
Launch the EdrawMax software on your computer. Click on "File" and select "New" to create a new document. Choose the "Flowchart" category or search for "Flowchart" in the search bar. Select a suitable flowchart template or start with a blank canvas.
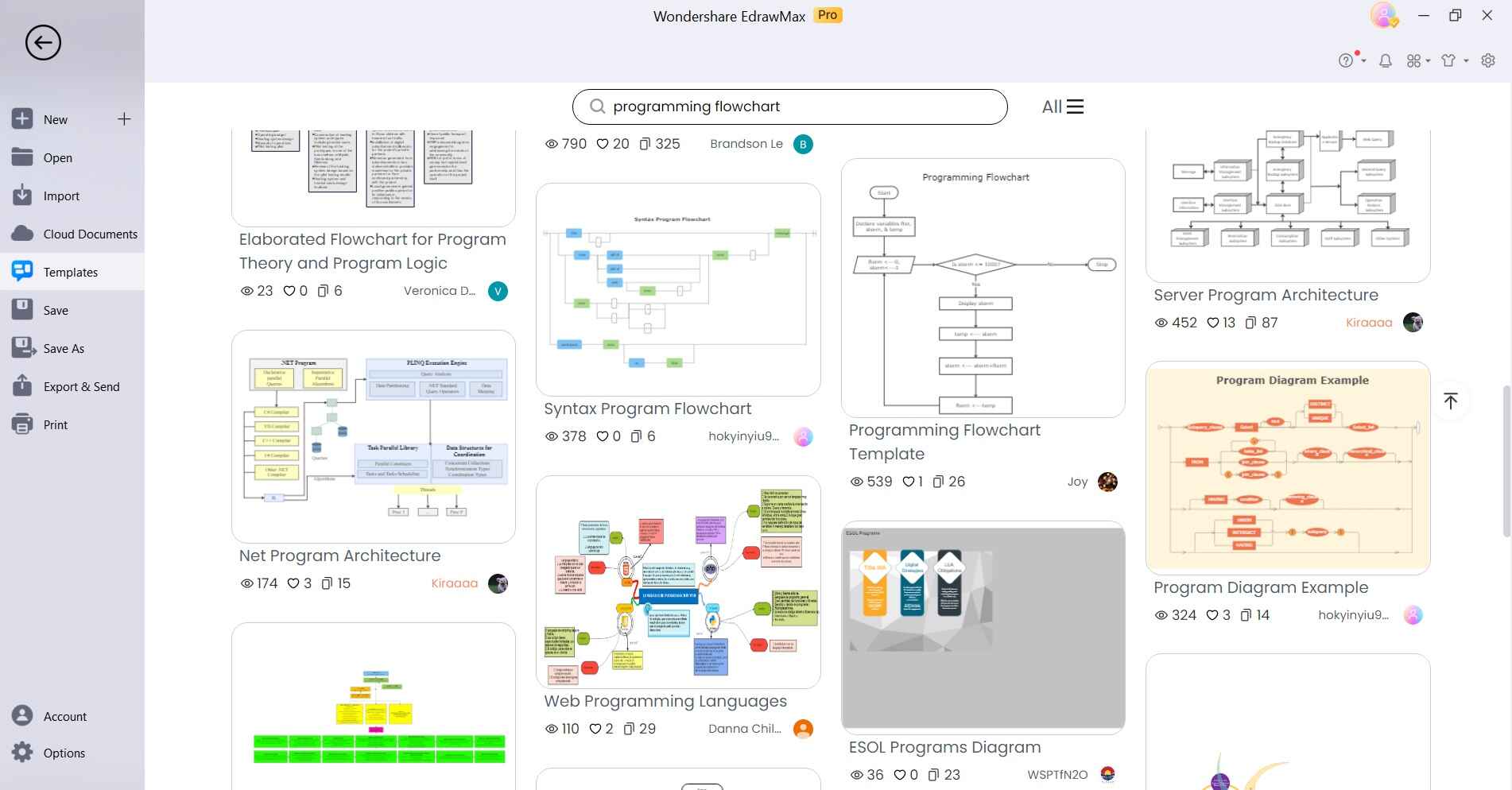
Step 2:
Drag and drop the necessary symbols from the left-hand sidebar onto the canvas.
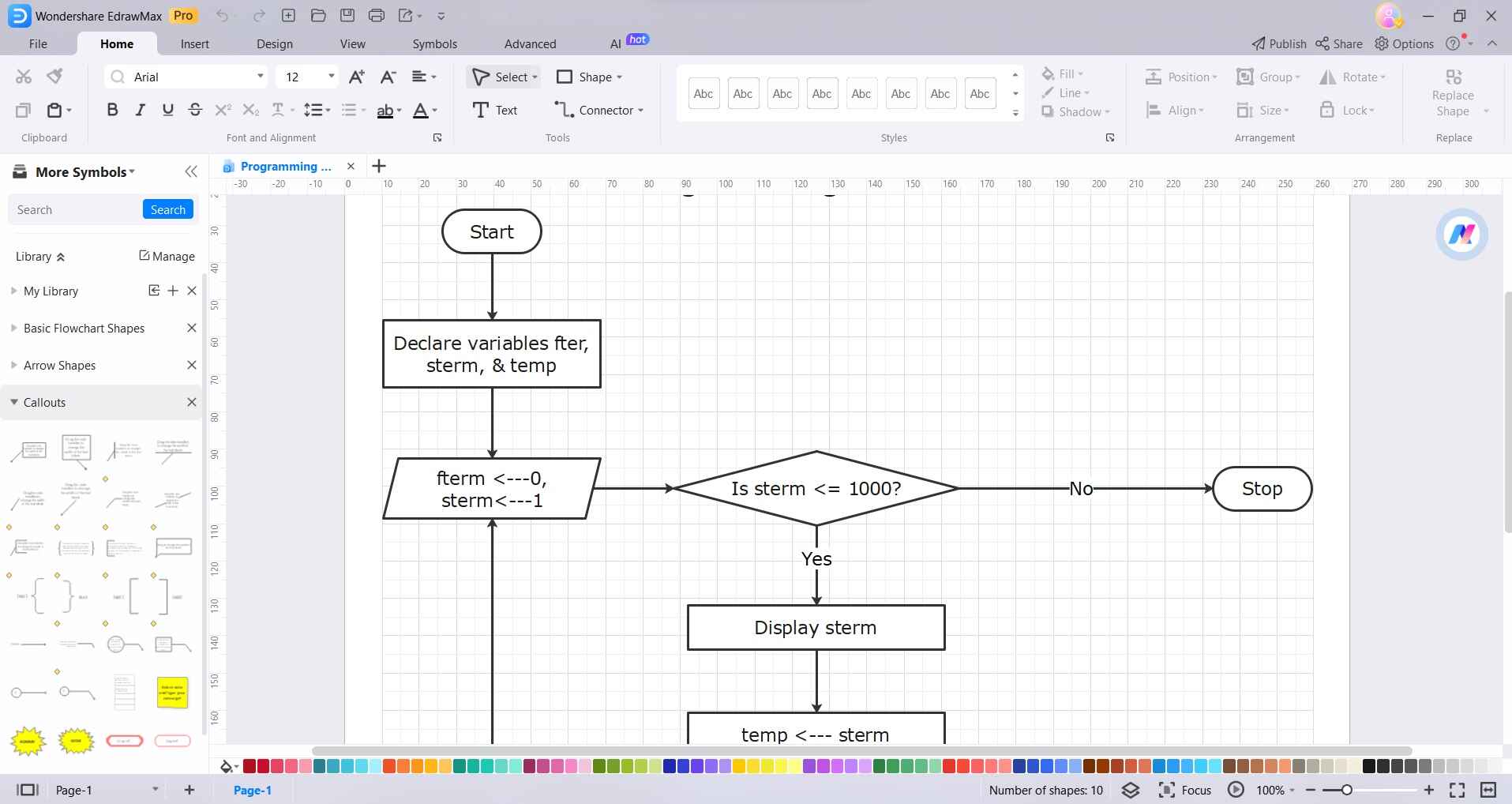
Step 3:
Use connector lines or arrows to link the symbols according to the logical flow of your program.
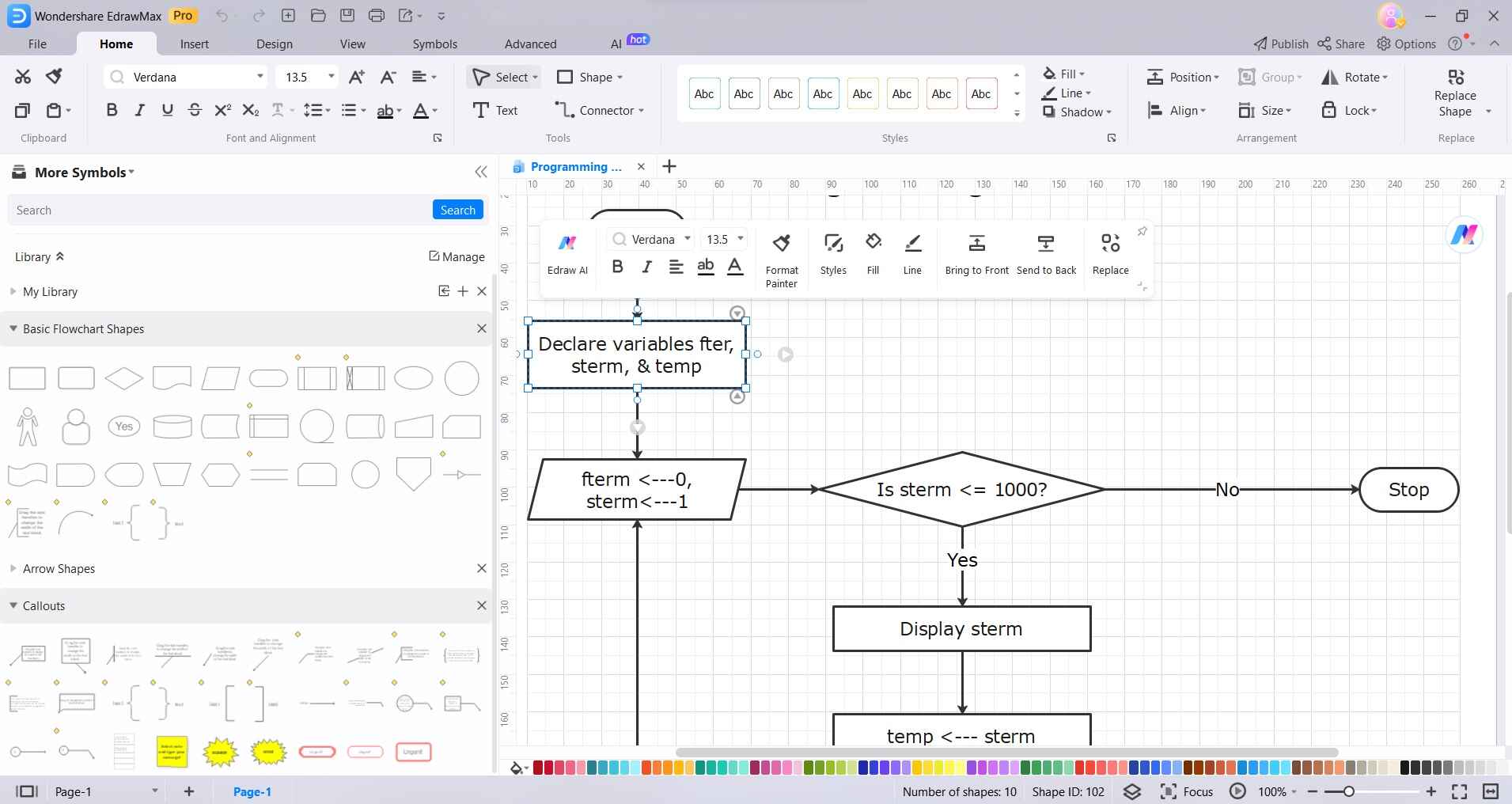
Step 4:
Customize the appearance of the flowchart by changing the colors, fonts, and styles of the symbols and connectors. EdrawMax offers various formatting options to make your flowchart visually appealing and easy to understand.
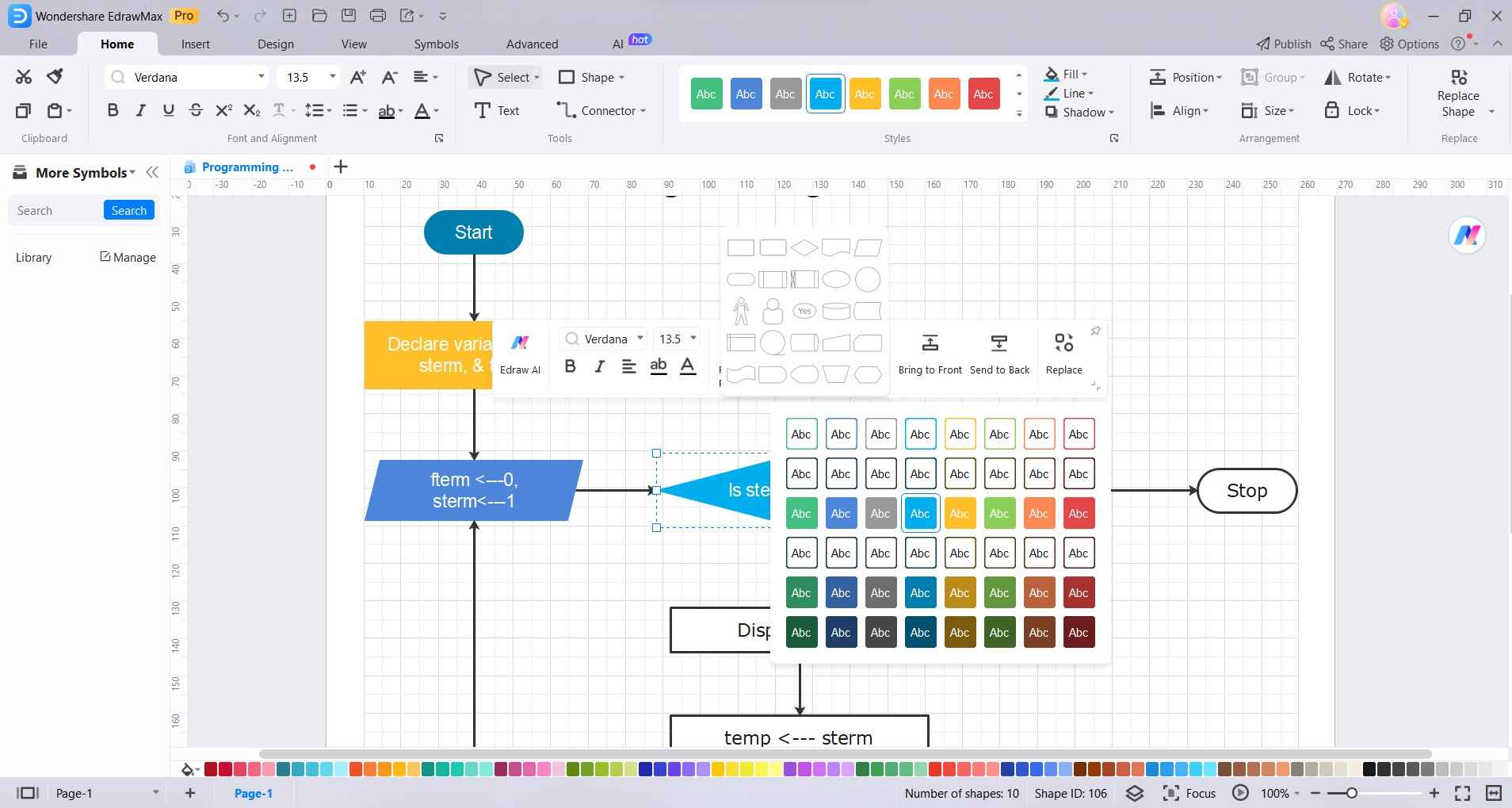
Step 5:
Once satisfied, save your flowchart in the desired format. EdrawMax allows you to save in multiple formats like PDF, PNG, or others suitable for your needs.
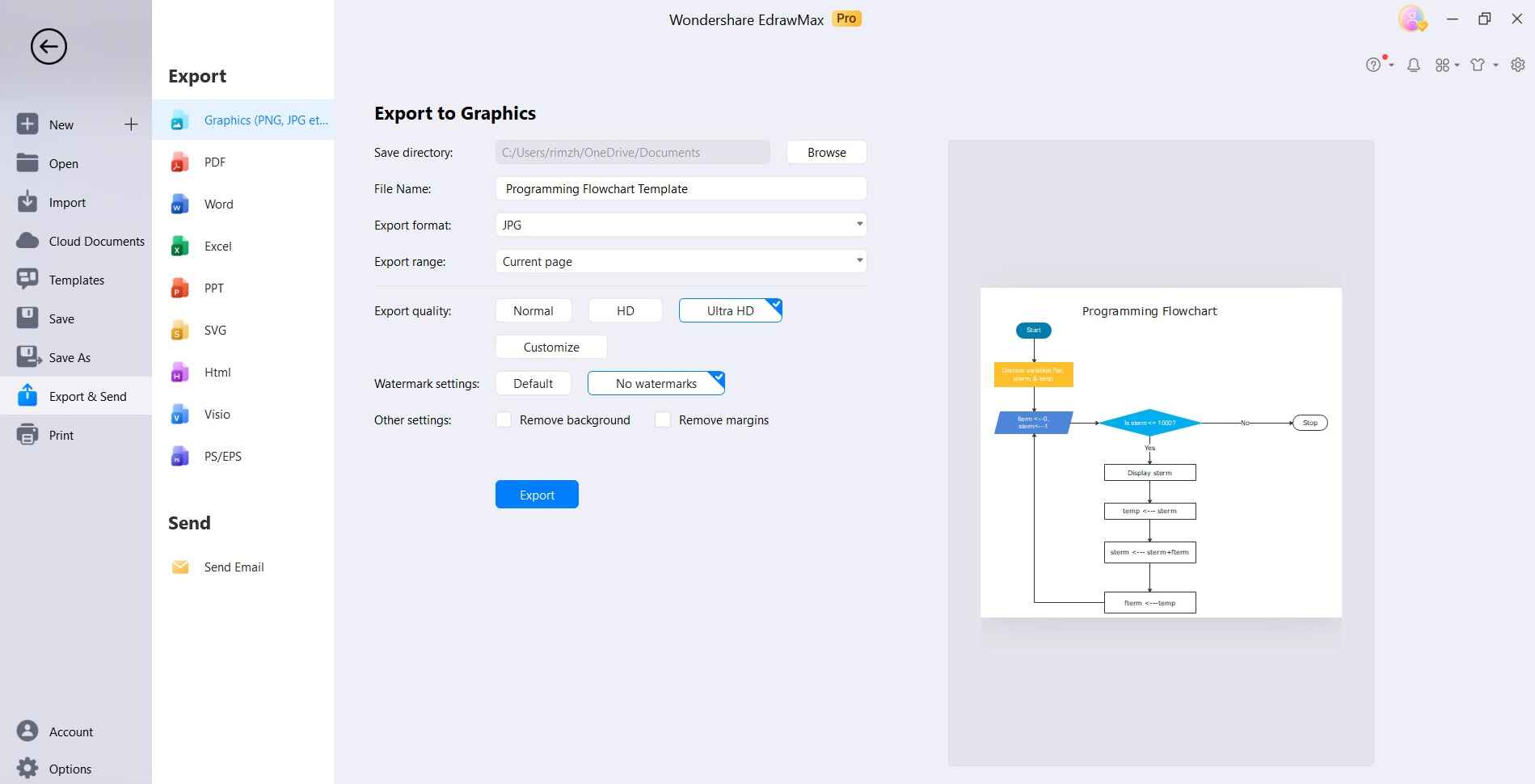
Following these steps should help you create a clear and concise programming flowchart using EdrawMax.
Conclusion
C++ structs allow the creation of custom data types tailored to specific programming needs. However, their full capabilities depend on proper initialization. By following best practices around member declaration order, initialization techniques, and purpose-built usage, developers can leverage structs effectively. Diagramming usage flows further bolsters maintainability.
With robust initialization and documentation, C++ structs become invaluable tools for managing complex application data needs.
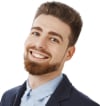