Structs are a pivotal data structure in programming that enables bundling related data together into custom types for organized storage. Learning the proper usage of structs to manage domain data effectively is a valuable skill for developers.
In this comprehensive tutorial about C++ create structs, we will provide all key details on structs for you to leverage for robust data modeling in your codebase. We will explain what exactly structs are, when they should be used, how to define and instantiate them, attaching methods, and more through beginner-friendly examples.
Whether you have no programming experience or have used other languages without structs, this guide will equip you with the knowledge to implement structs for clean data management. Let's get started!
In this article
Part 1: Understanding Structs
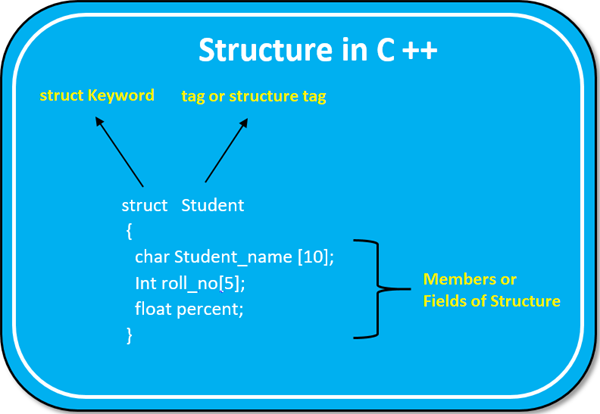
A struct at its core groups relevant data pieces into a single, coherent object. For instance, an e-commerce system may have a customer name, email, address, order history, etc. Structuring all that data into a Customer struct makes sense.
Structs have similarities with classes in that they encapsulate data and behavior. However, structs are focused exclusively on data storage and access without inheritance, polymorphism, etc. They also utilize value semantics rather than reference semantics.
Key traits and advantages offered by structuring data using structs:
- Custom types organize domain data.
- Related attributes bundled together.
- Value-based copies simplify assignment.
- Lightweight and efficient structure.
These characteristics directly translate to cleaner code and simplified data handling when leveraged effectively by knowing when and why structs are ideal in your program.
Part 2: Defining Structs in Different Programming Languages
While syntax varies across languages, structs generally adhere to this blueprint:
struct Name {
dataType attribute1;
dataType attribute2;
}
For example, in C# you would define a Customer struct with:
struct Customer {
string FullName;
string Email;
string[] Orders;
}
And Golang Create Struct example would look like this:
type Customer struct {
FullName string
Email string
Orders []string
}
Key components that make up a struct definition:
- struct keyword
- Name - PascalCase convention
- Curly braces encapsulating data
- Attributes typed individually
Carefully consider which data models your specific domain entities like Customer. Structuring related attributes is what makes structs valuable.
Part 3: Create Struct C++: Steps for Implementation
Here are the steps for creating a struct in C++ without using code:
- Conceptualize the Structure: Identify the elements or attributes that need to be grouped within the struct. For instance, if creating a struct for a person, consider attributes like name, age, and address.
- Define the Struct Name: Decide on a name that represents the collection of these attributes or elements. For example, a struct representing a person's details could be named Person.
- List the Members: Within the struct, list the various members or attributes that define its properties. Each member should represent an aspect or characteristic of the overall structure. For instance, a Person struct might include members like name, age, and address.
- Assign Data Types: Associate appropriate data types with each member to define the kind of information it will store. For example, the name could be a string, age an integer, and address another string or a custom data type representing an address.
- Consider Relationships: If the struct members have any relationships or dependencies, plan how they interact within the struct. For instance, a Person struct might have an address member that consists of multiple fields like street, city, and postal code.
- Plan Usage: Visualize how this struct will be utilized within your program. Determine where and how instances of this struct will be created, accessed, or modified to effectively organize and manage your data.
Following these steps will help in creating a well-defined struct in C++, which encapsulates related data and promotes better organization within your programs.
Part 4: Creating a Programming Diagram Using EdrawMax
Creating a programming diagram using EdrawMax holds immense significance in visualizing complex code structures and system architectures. This tool empowers programmers to illustrate the flow of logic, relationships between components, and the overall structure of their codebase.
With EdrawMax's intuitive interface and diverse templates, developers can efficiently communicate intricate coding concepts, facilitating better understanding among team members and stakeholders.
Here are the steps to create a programming diagram flowchart using EdrawMax:
Step 1:
Open the EdrawMax application on your computer. Head to File> New> Software and Database> Software Development. Choose a flowchart template that best suits your programming logic or start from scratch by selecting a blank canvas.
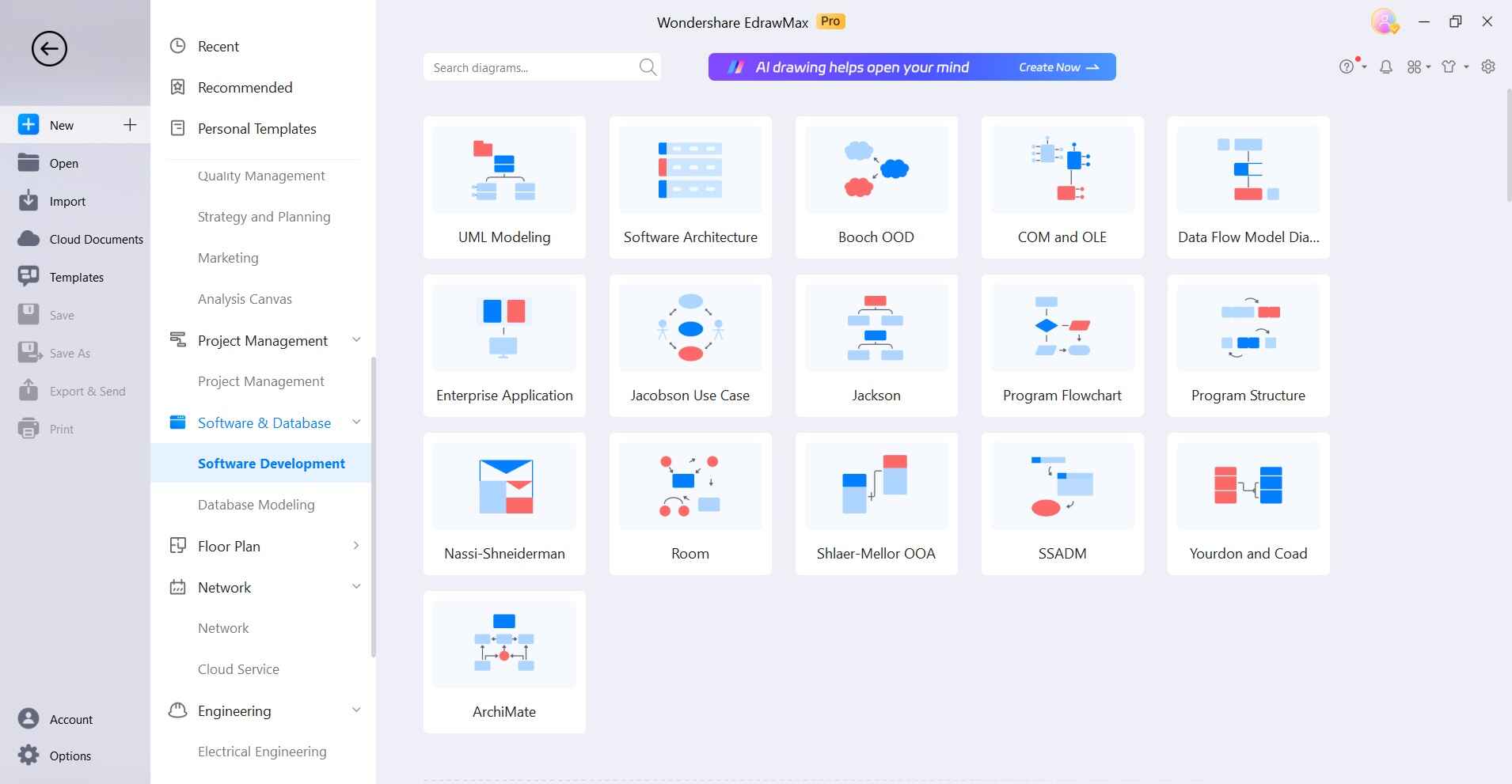
Step 2:
Utilize the flowchart shapes and symbols from the library. Drag and drop shapes onto the canvas to represent different programming elements like processes, decisions, loops, inputs, outputs, etc.
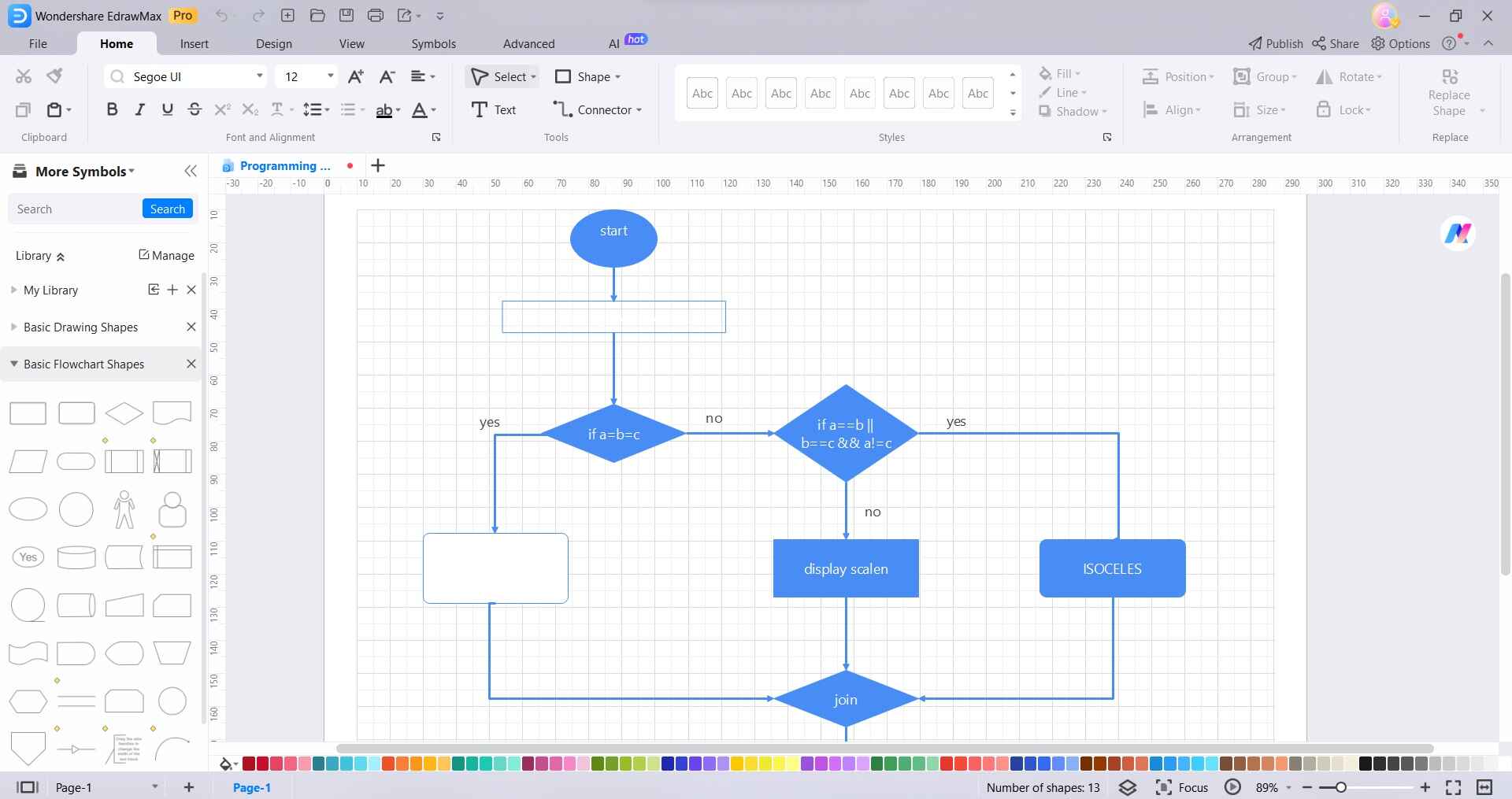
Step 3:
Label each shape or process with appropriate text to describe the functionality. This helps in clarifying the purpose of each element within the flowchart.
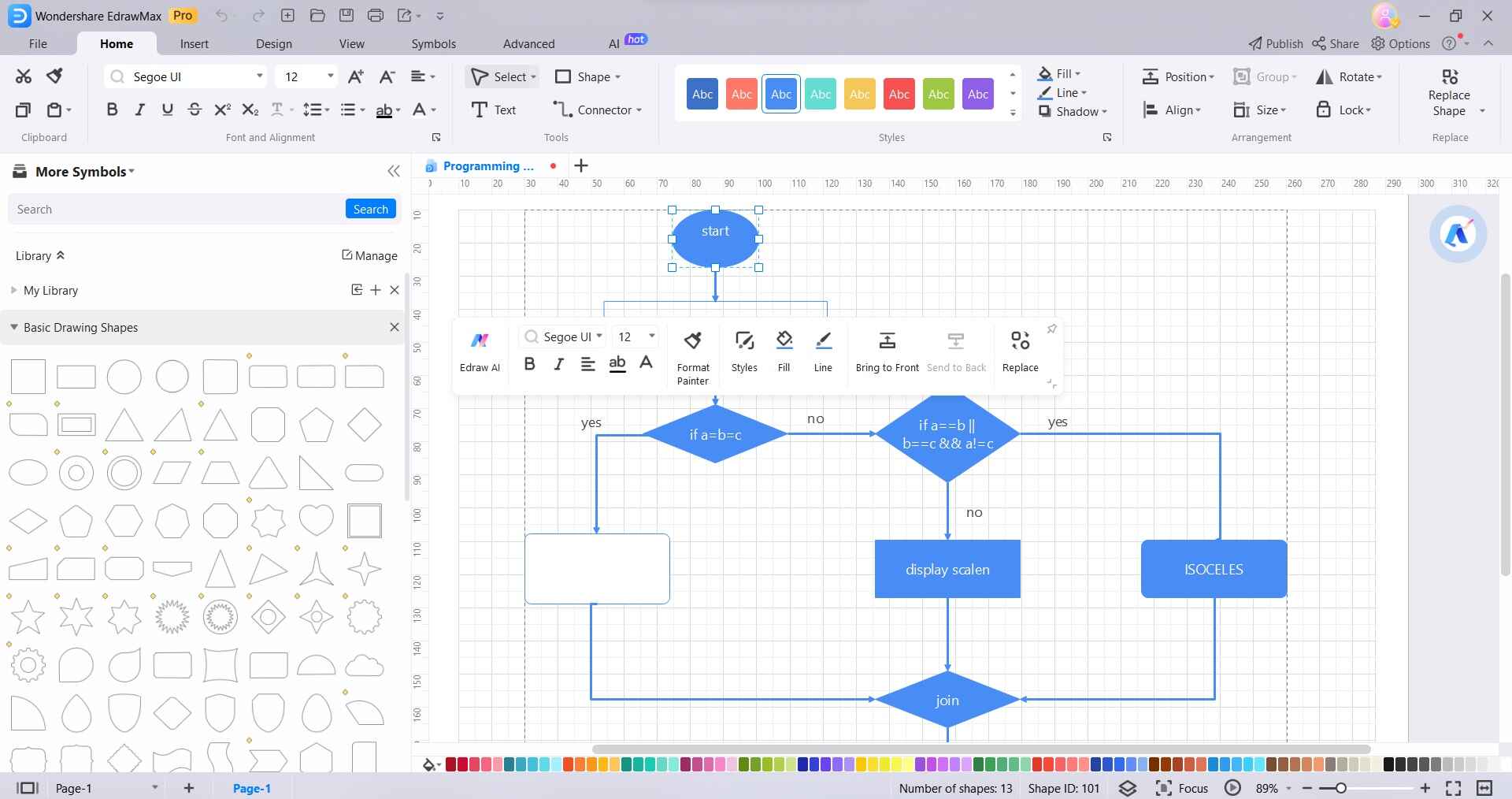
Step 4:
Modify the appearance of shapes, connectors, and text to enhance readability and visual appeal. Change colors, fonts, line styles, and sizes as needed.
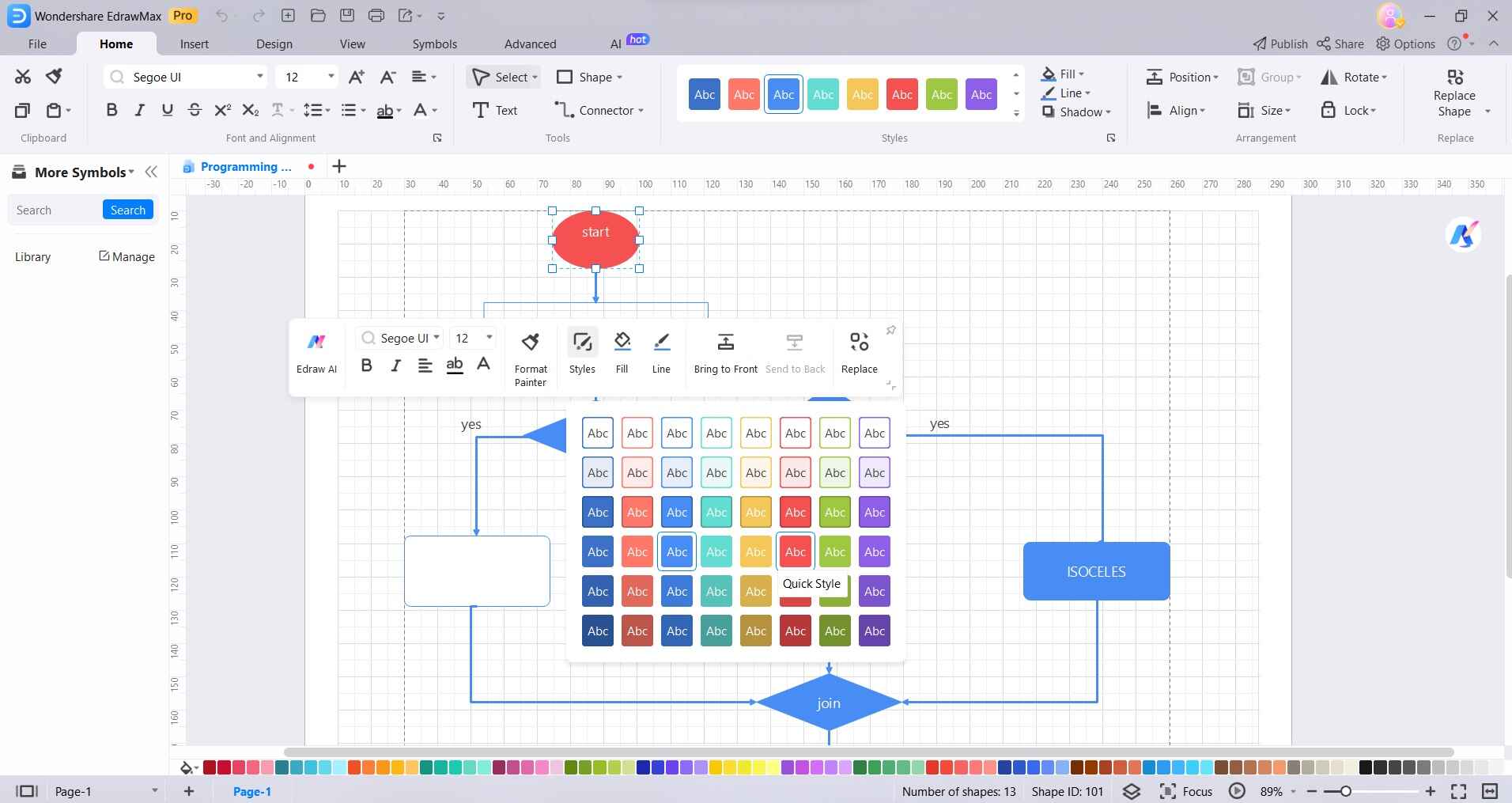
Step 5:
Save the completed programming diagram in your preferred format (PNG, JPEG, PDF, etc.) and share it with team members, and collaborators, or include it in project documentation.
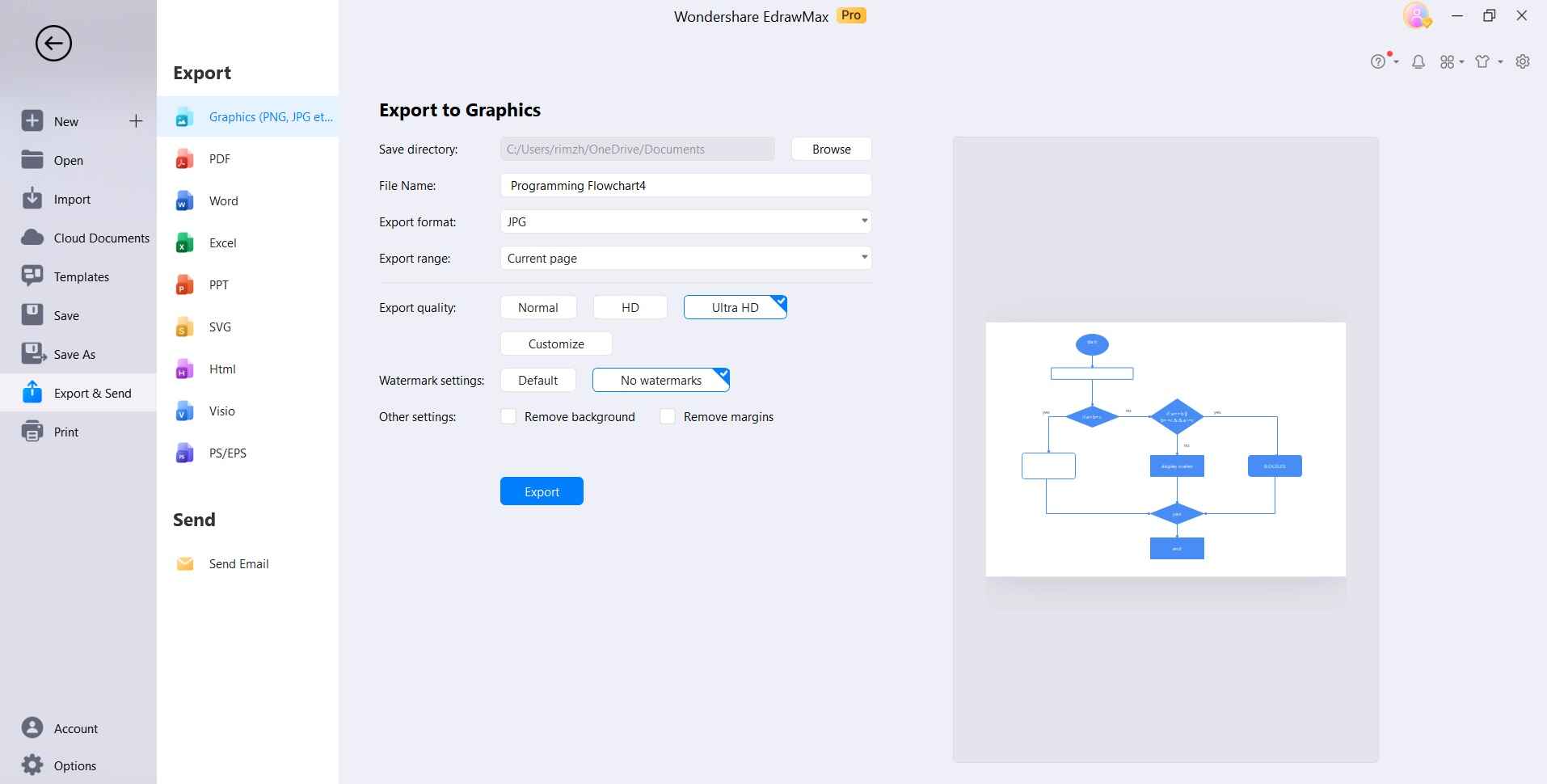
Following these steps using EdrawMax's intuitive interface and features can help create comprehensive and visually appealing programming diagrams and flowcharts for various programming projects.
Conclusion
Structs enable the creation of custom data types tuned for your specific use case by bundling relevant attributes and methods together. As covered in this tutorial, they have wide applicability and pronounced advantages for organizational data modeling.
Carefully considering which data groups together cohesively along with associated functionality during struct design will enable much cleaner, readable, scalable code. Thoughtful struct usage fundamentally leads to better overall program architecture.
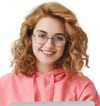