A linked list is a linear data structure that contains a sequence of connected nodes where each node stores a data element and a pointer or reference to the next node. Linked lists provide efficient insertion and removal of elements as compared to arrays.
In this article, we will learn how to create linked lists in C and Python programming languages.
In this article
Part 1: What is a Linked List?
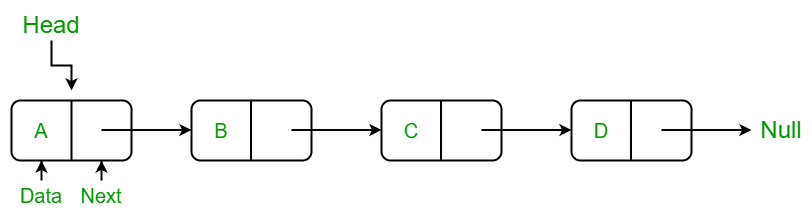
A linked list organizes items sequentially where each item points to the next one forming a chain. It consists of nodes where each node contains data and a pointer/reference to the next node. The last node of the list contains a null pointer indicating the end. This allows efficient dynamic memory allocation as the list can expand or shrink at runtime.
The main advantages of using linked lists over arrays are:
1. Dynamic size – Linked lists have no fixed size allowing dynamic expansion and contraction. Arrays have fixed pre-defined capacity.
2. Ease of insertion/deletion – We can easily insert and delete elements from any position of a linked list. However, insertion/deletion in arrays is expensive.
3. No memory wastage – Linked list allocation happens dynamically while arrays may have unused memory allocated.
4. Data access – Accessing an element in a linked list requires traversing sequentially from the head while arrays allow direct indexed access.
Part 2: Types of Linked Lists in C
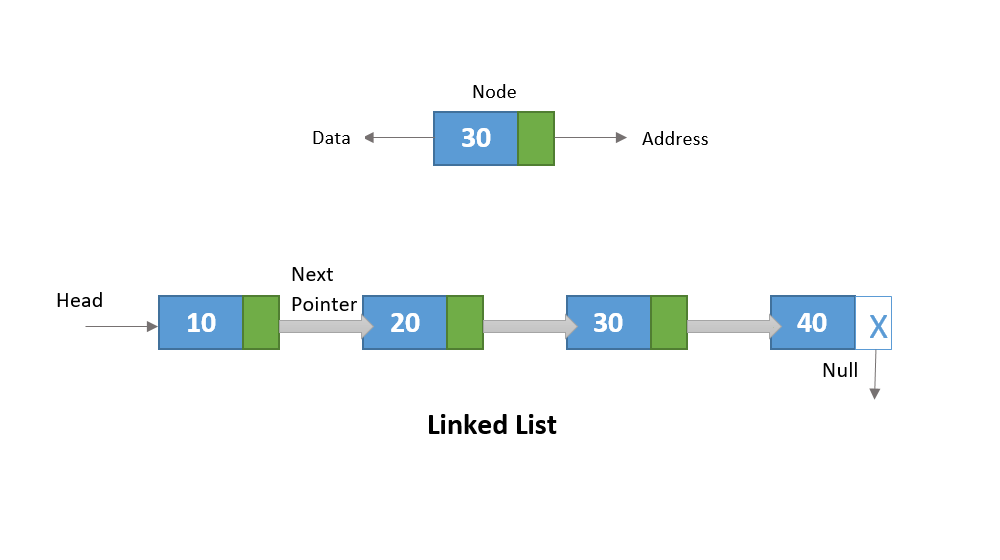
Here are the major types of linked lists in C:
- Singly Linked List - Each node stores data and a pointer to the next node.
- Doubly Linked List - Besides the next node pointer, each node also stores a pointer to the previous node allowing bi-directional traversal.
- Circular Linked List - The last node instead of having a null pointer stores a pointer to the first node forming a non-linear circular structure.
We will focus on creating singly linked lists in this article.
Part 3: Steps to Create Linked List in C
Here are the key steps to create a basic linked list data structure in C programming:
1. Include libraries:
#include
#include
2. Create a Node structure with data and the next pointer:
struct Node {
int data;
struct Node *next;
};
3. Create linked list functions:
- insert() - insert a node at the beginning
- print() - print linked list data
4. Define main() and test linked list:
int main() {
//insert nodes
insert(head, 1);
insert(head, 2);
//print list
print(head);
return 0;
}
Part 4: Example C Program to Create a Linked List
Here is an example C program demonstrating how to create a simple linked list:
#include
#include
struct Node {
int data;
struct Node *next;
};
void insert(struct Node** head, int data) {
//create a new node
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
//point new node next to head
newNode->next = *head;
//make a new node as head
*head = newNode;
}
void print(struct Node *head) {
struct Node *temp = head;
//traverse and print linked list
while(temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
}
int main() {
struct Node* head = NULL;
//insert nodes
insert(&head, 1);
insert(&head, 2);
insert(&head, 3);
//print list
print(head);
return 0;
}
In the above program, we first define a Node structure containing integer data and the next pointer. The insert() function allocates memory for each new node inserted at the head and adjusts the required links. Finally, the print() function sequentially accesses each node to output values.
Part 5: Advantages of Using Linked Lists
So, you have mastered how to create a linked list in C. Here are some benefits of using linked lists over other linear structures like arrays:
- Dynamic size allows expansion and contraction of the list at runtime.
- Easy insertion and removal of elements without reallocation.
- No limit or wastage of allocated memory.
- More efficient memory usage as it's allocated per node dynamically.
Part 6: Create a Programming Diagram Example Using EdrawMax
EdrawMax is handy software for visually creating programming diagrams like linked lists.
This nifty diagram creation tool makes it easy to generate programming visuals like linked lists, trees, flowcharts, etc automatically. The built-in templates help accelerate documentation.
Here are the steps to create a simple programming diagram example using EdrawMax:
Step 1:
Launch the EdrawMax software on your computer. Click on "New" or "File" and then select the "Software" or "Flowchart" category, depending on the specific programming diagram you want to create.
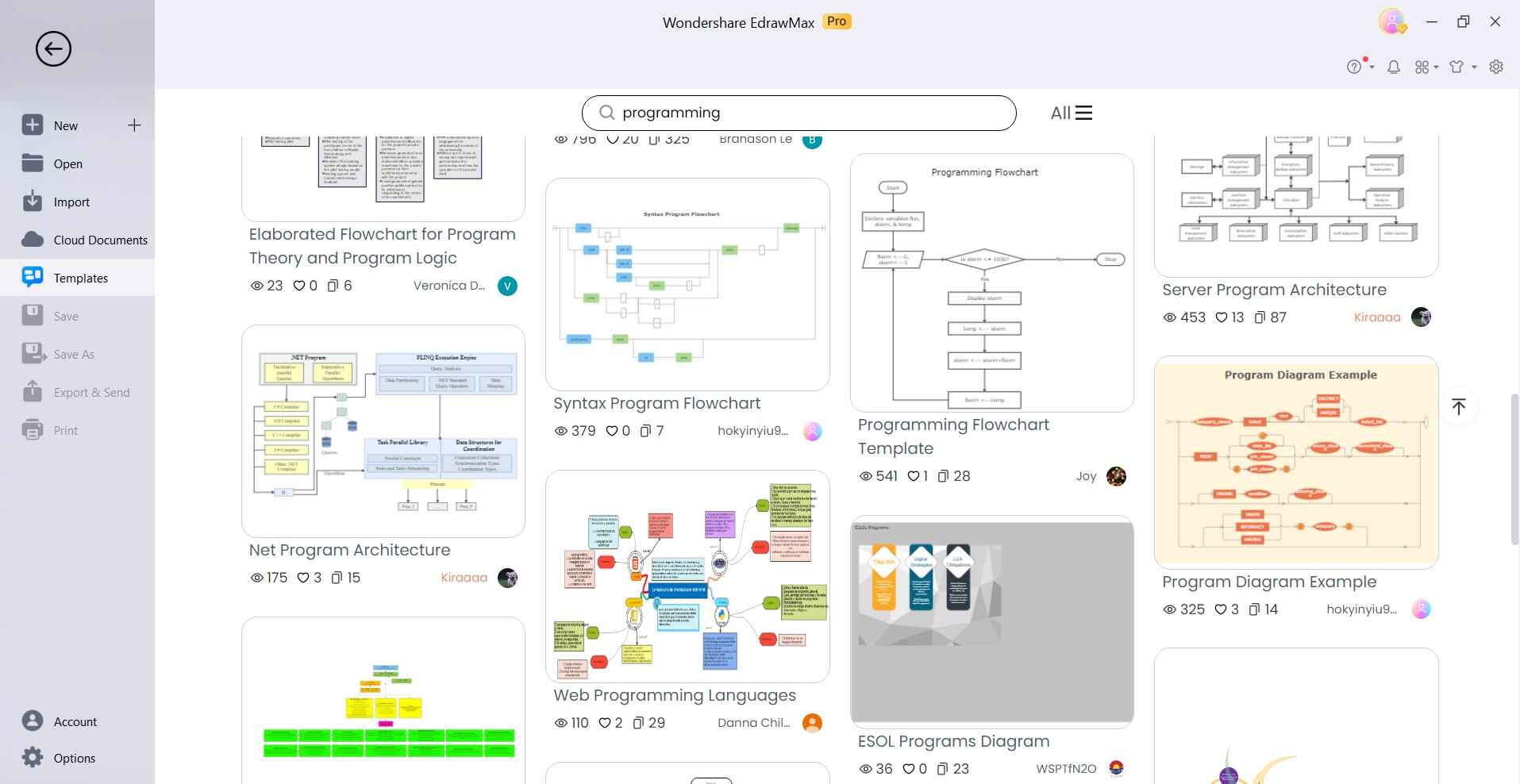
Step 2:
For a programming diagram, you might use shapes representing processes, decisions, inputs/outputs, loops, etc. Drag and drop shapes, symbols, and connectors from the libraries.
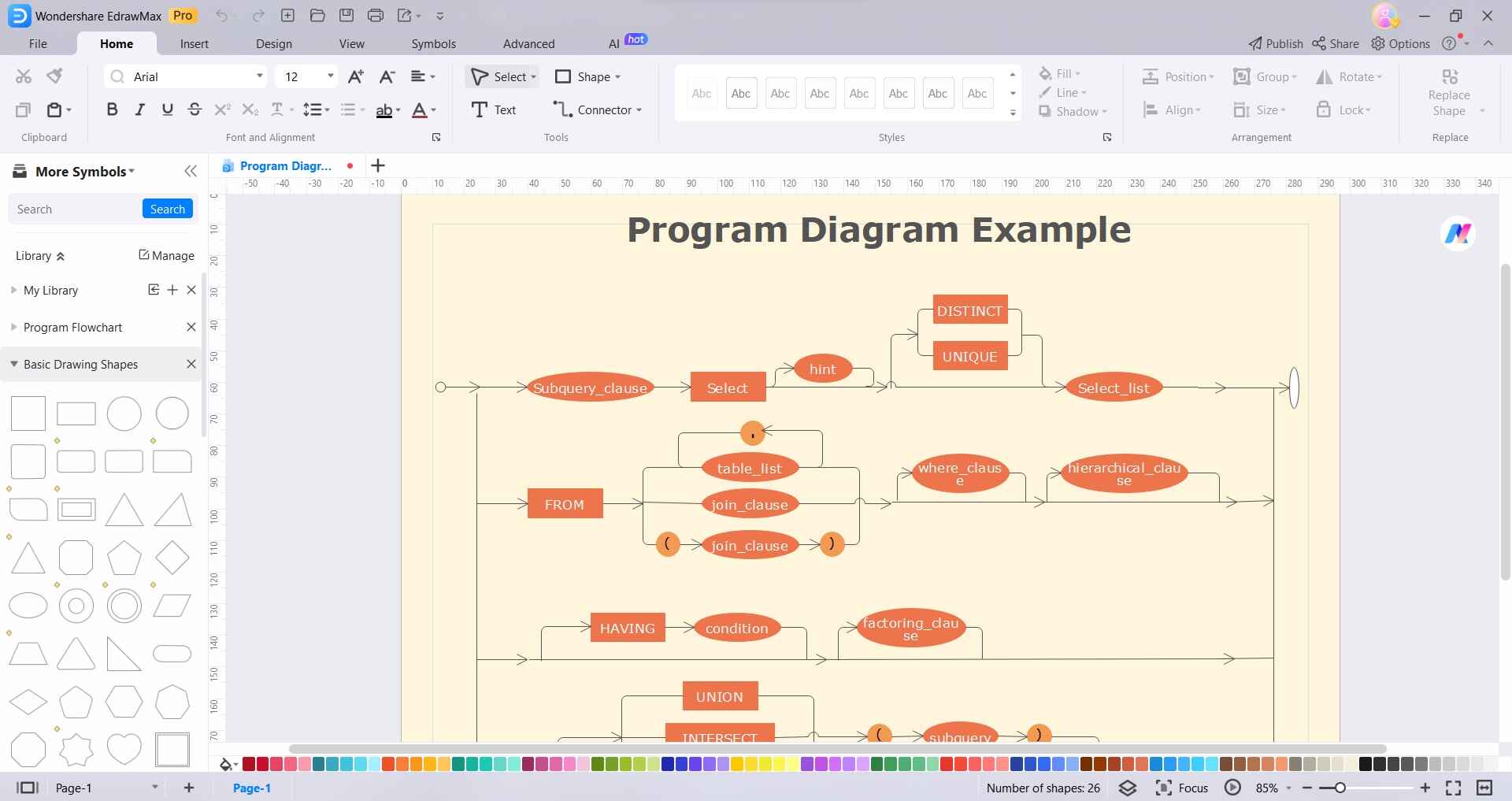
Step 3:
Modify the shapes, lines, and text to suit your requirements.
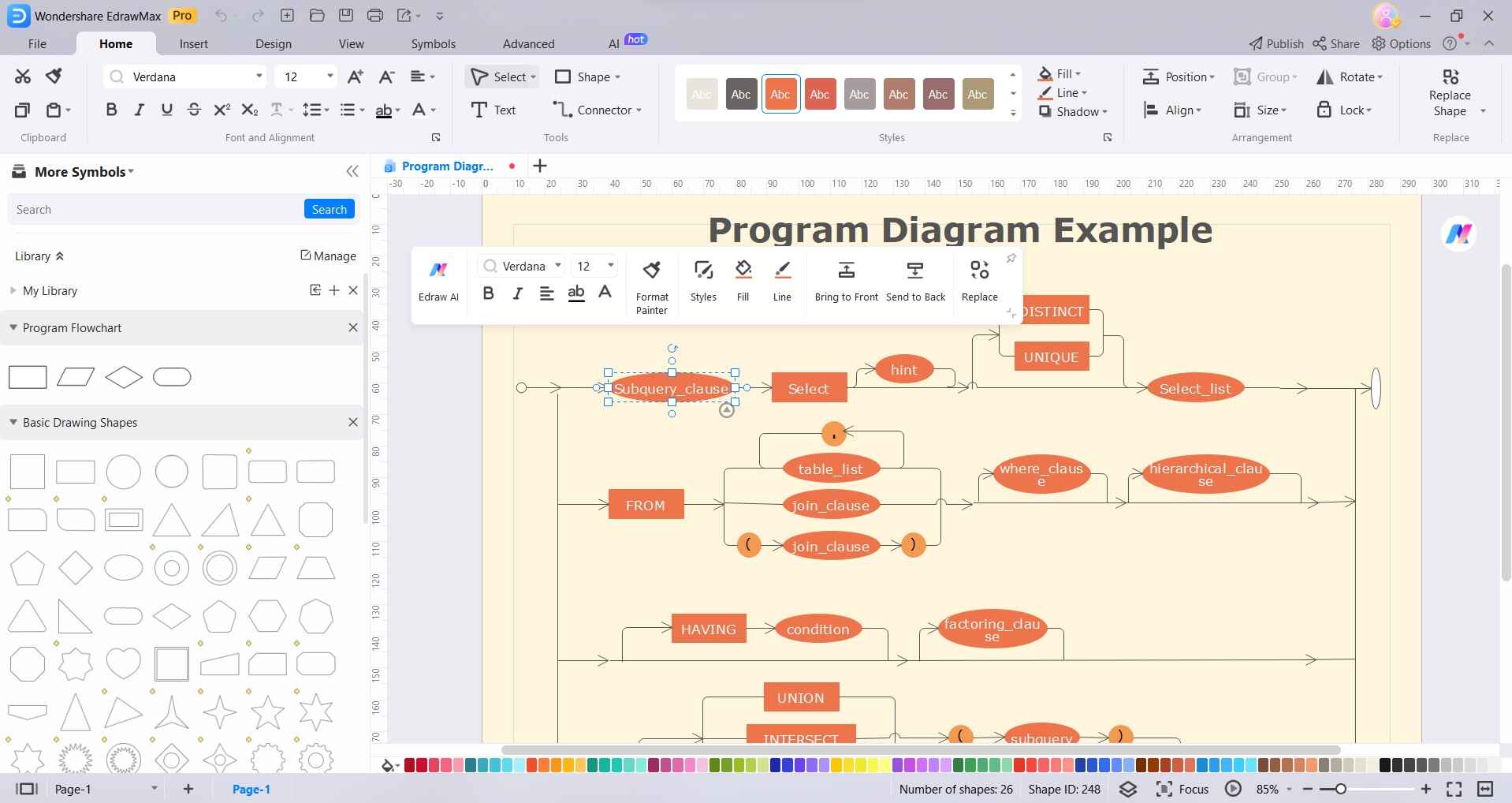
Step 4:
Change colors, add labels, and format text to enhance readability and clarity.
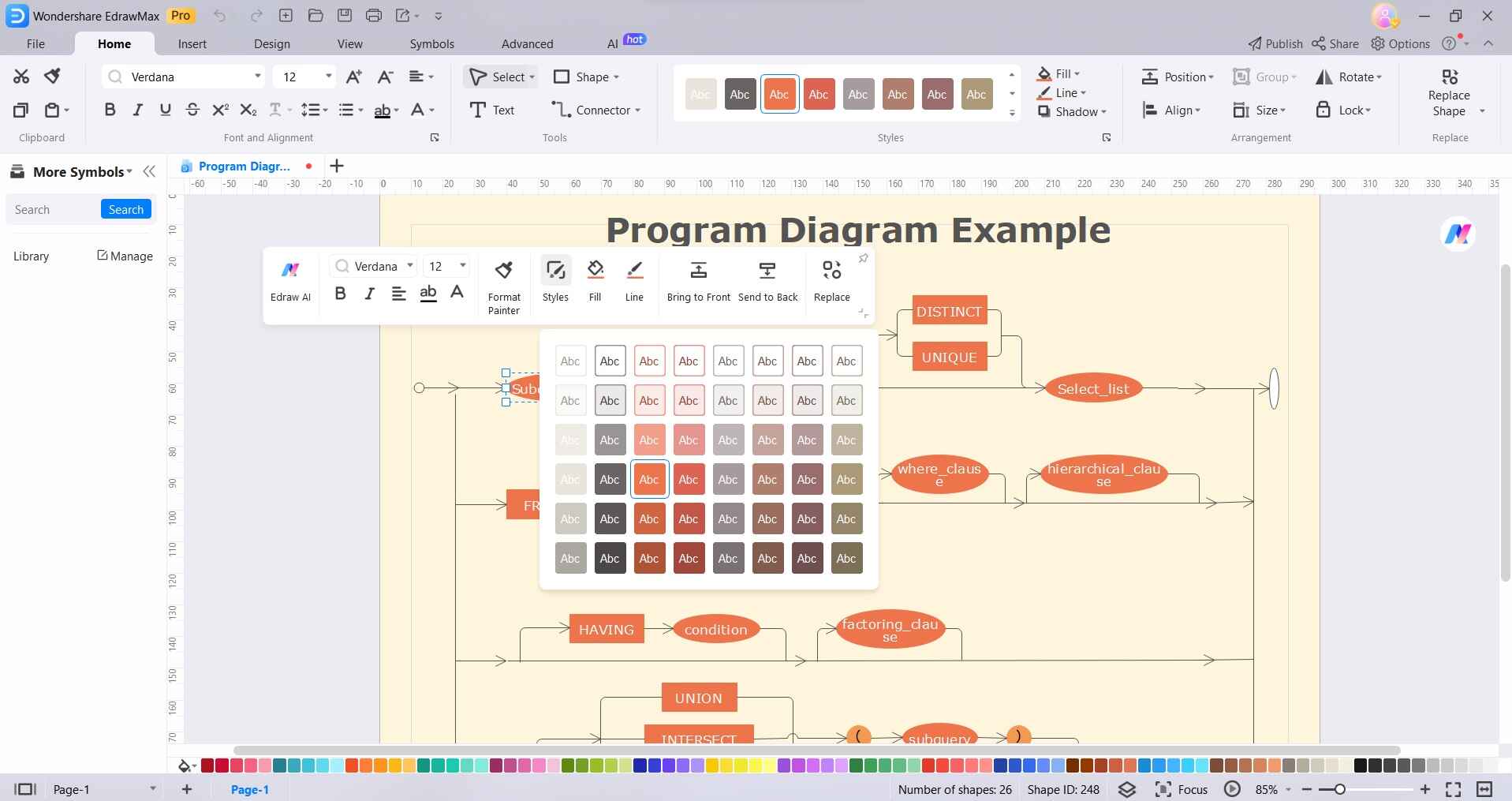
Step 5:
Save your work in the desired format (like .eddx for EdrawMax). You can also export it to various file types (PNG, JPEG, PDF, etc.) for sharing or presentation purposes.
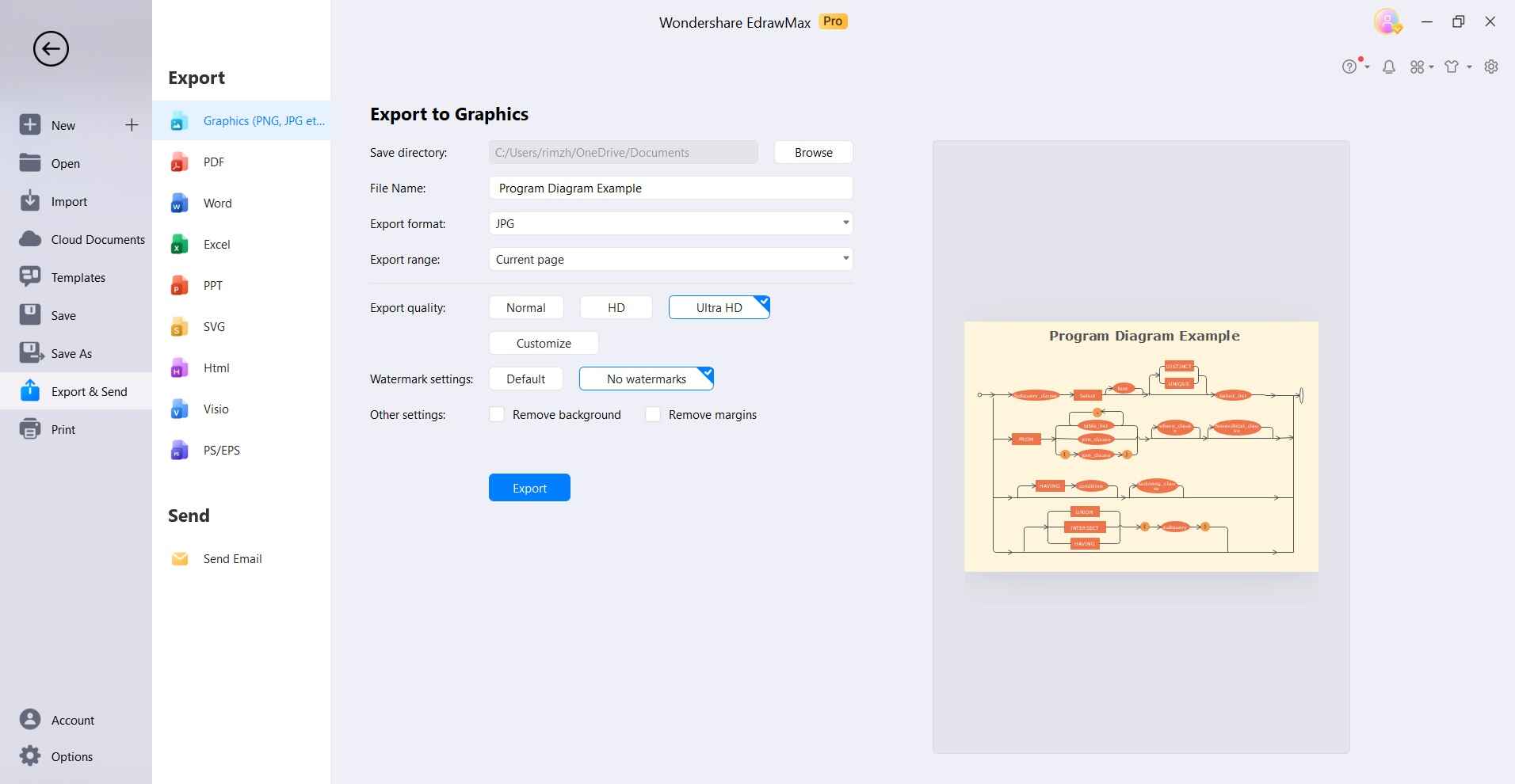
Following these steps in EdrawMax should help you create a clear and visually appealing programming diagram for your needs.
Conclusion
In this article, we went through the basics of linked lists - definition, types, advantage over arrays, and implementation in C and Python programming languages. We looked at practical example code as well as steps to visualize a linked list diagrammatically using the EdrawMax tool. Linked lists form an integral part of many algorithms and data structures.
Mastering linked list creation is key to effectively applying them in different problem-solving scenarios.
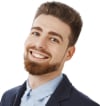