Classes and structures are fundamental building blocks in many programming languages, allowing developers to create reusable components with encapsulated data and behaviors. C# in particular implements classes and structures in an object-oriented manner, with some notable differences from other languages like Python or Swift.
In this article, we will dive into the details of C# class structure, contrasting it to other languages along the way. We’ll also look at specific class types like queue and stack classes, and explore creating flowcharts to design program logic and structure.
In this article
Part 1: What is a Python Class Structure
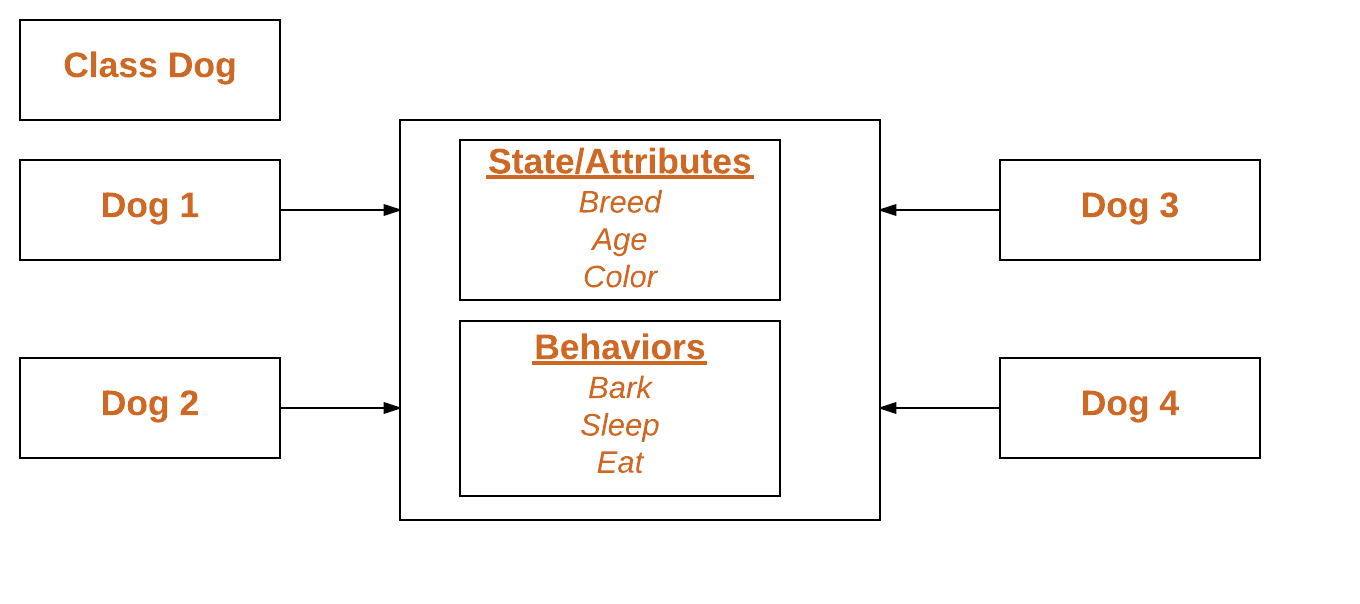
In Python, classes provide a means of bundling data and functionality together into reusable pieces of code. They encapsulate data in the form of fields or properties, and functionality in the form of methods. The basic Python class structure looks like this:
class MyClass:
# Fields
my_var = 1
# Constructor
def __init__(self):
self.my_var = 2
# Methods
def my_method(self):
print(self.my_var)
In this example, we created a class using the class
keyword, with indented blocks underneath to define the class body, consisting of fields, constructors, and methods. Objects get created from a class via instantiation.
Key characteristics of Python classes include:
- Inheritance: Child classes can inherit fields and methods.
- Encapsulation: Via access modifiers like private, and protected.
- Polymorphism: Using abstract base classes.
Overall, Python classes allow custom reusable components to be created that model complex real-world entities.
Part 2: Overview of C# Class Structure
Like Python, C# utilizes classes and OOP concepts like inheritance, encapsulation, and polymorphism. C# supports structures as a value type complement to classes, properties for encapsulated access to fields, no multiple inheritance with interfaces instead, and destructors for managing resources.
Additionally, C# supports concepts like generics, interfaces, delegates, and events to enable rich reusable component abstractions.
Part 3: Understanding Class and Structure in Swift
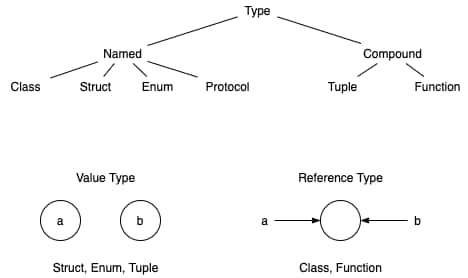
Swift classes and structures have some key differences. Structures are value types while classes are reference types. Structures do not support inheritance but have less overhead compared to classes and are preferred for simple immutable data models. Classes allow more complexity with inheritance, deinitializers, etc., whereas structures map to lightweight data models.
Choosing Between Classes and Structures:
- Use classes when you need reference semantics, inheritance, or complex object behavior.
- Use structures when you need value semantics or when dealing with simpler data types.
Understanding these differences helps in choosing the right tool for the job, ensuring efficient and effective code in Swift programming.
Part 4: What is a Queue Class Python?
A custom Queue class in Python allows encapsulation of queue operations like enqueue, dequeue, peek, and size. Benefits include reusable logic, hiding lower-level implementation, customizability through inheritance, and flexibility to store any object type.
Python's Queue class is typically implemented using the queue
module, which provides various implementations of queues like Queue
, PriorityQueue
, and Deque
. These classes offer functionalities such as adding elements (put), removing elements (get), checking if the queue is empty, and managing the ordering of elements as per the defined rules. They are often used in scenarios where elements need to be processed in the order they were added, like task scheduling, job processing, or event handling.
So a Queue class allows clean, reusable queuing behavior in an object-oriented manner.
Part 5: Difference Between Python Stack Class and Swift Class Struct
While Python utilizes classes for its core data structures, Swift opts for structures instead in many cases, including for stacks. The reasons for using a struct in Swift include that they avoid the overhead of classes, have value semantics that simplify usage, support mutability when needed, and inheritance is less important.
Overall, structures align well with immutable, value-oriented data models like stacks.
Part 6: Creating a Programming Flowchart Using EdrawMax
EdrawMax is professional diagramming software that makes it easy to create flowcharts to design program logic and structure. Its key features include drag-and-drop symbols, professional templates, an intuitive interface, multi-platform capabilities, and flexible export options.
EdrawMax enables programmers to quickly map out application flow, structure classes effectively, design database schemas, model software systems, and more. Its templates and export options facilitate software design workflows.
Here are the steps to create a simple programming flowchart using EdrawMax:
Step 1:
Launch the EdrawMax software on your computer. Look for the "Flowchart" category in the template selection panel. Choose a suitable template or start with a blank canvas.
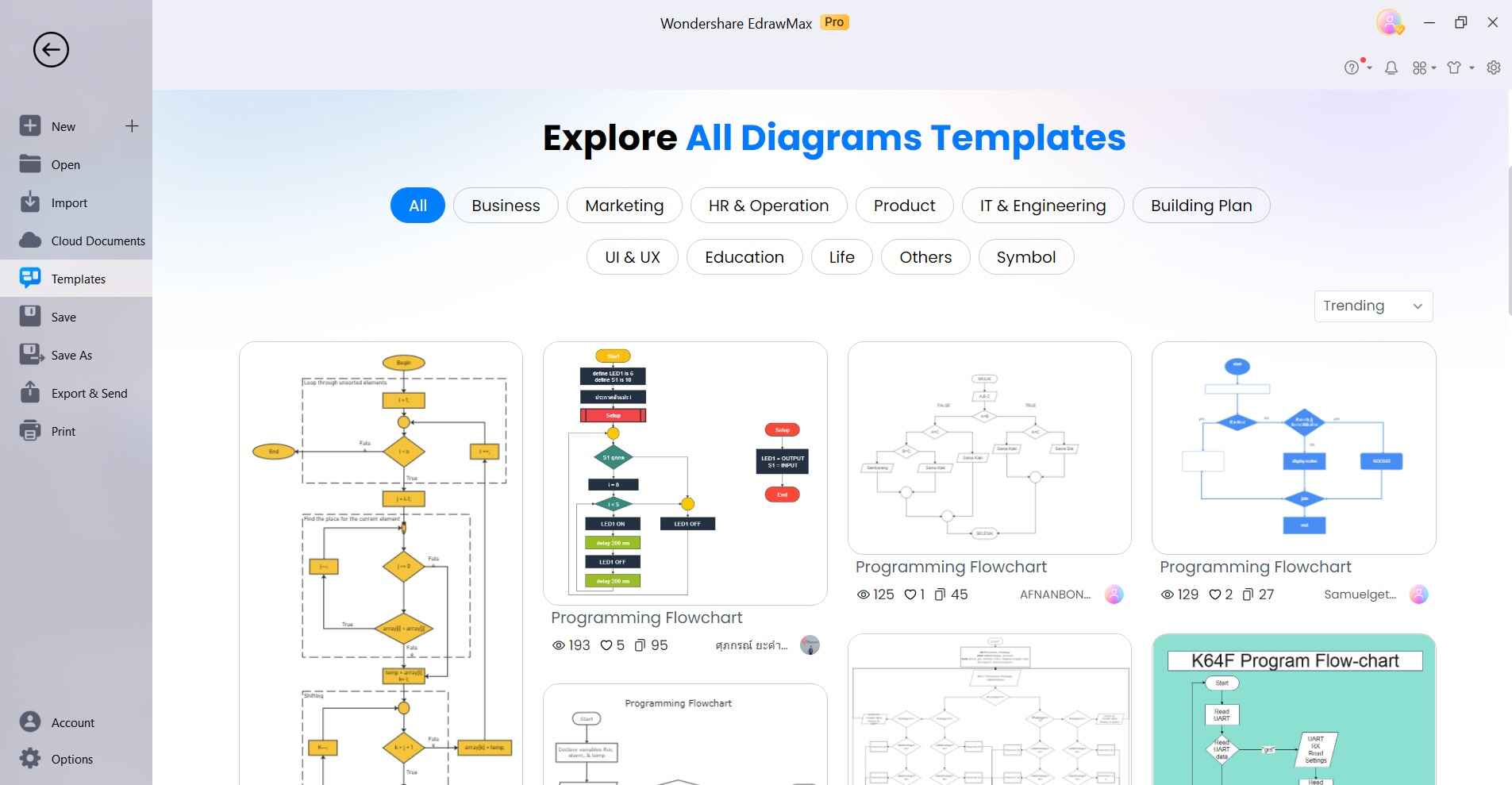
Step 2:
Drag and drop the required symbols onto the canvas from the symbol library.
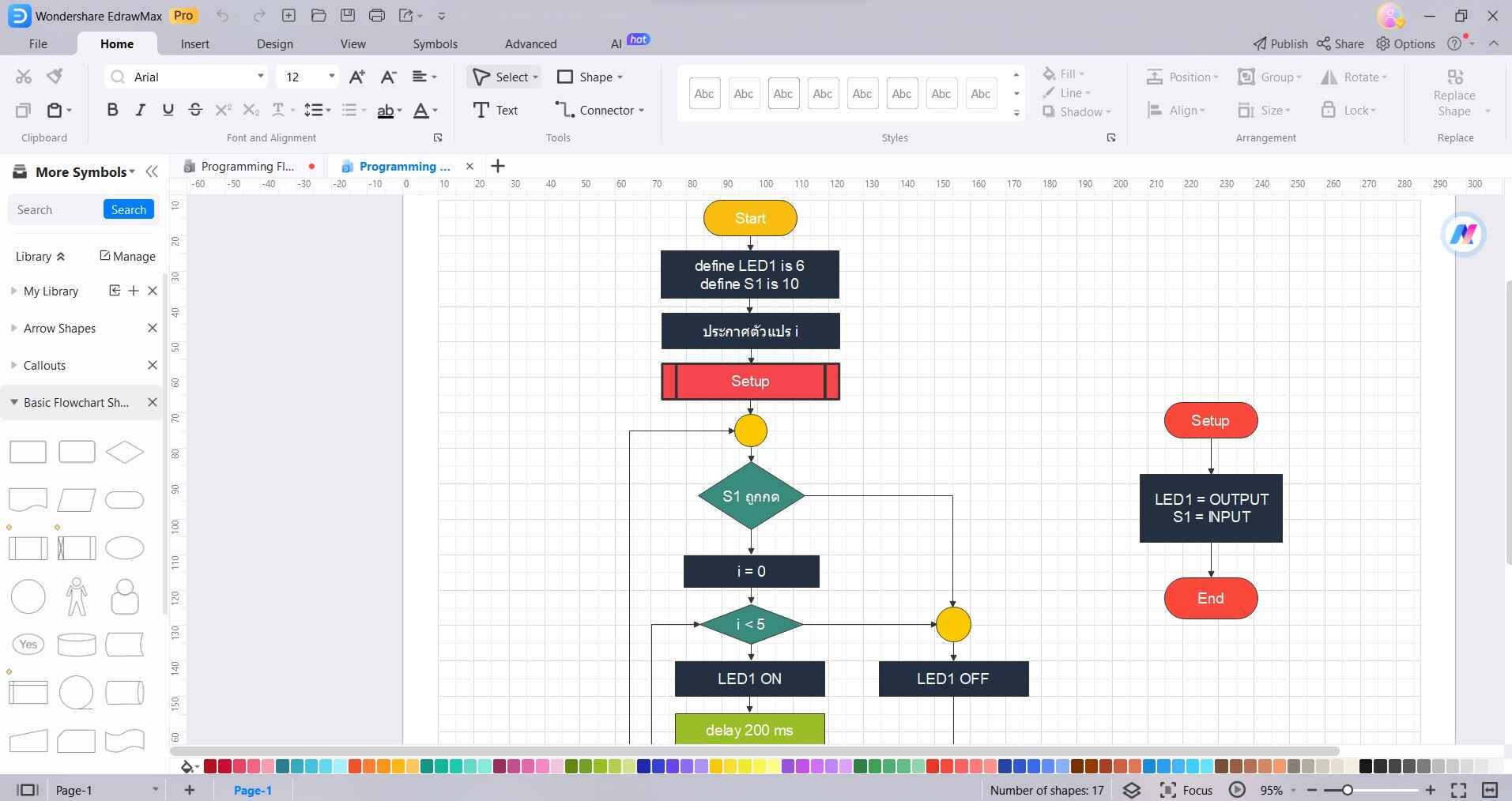
Step 3:
Double-click on the symbols to add text and labels indicating the actions or processes at each step.
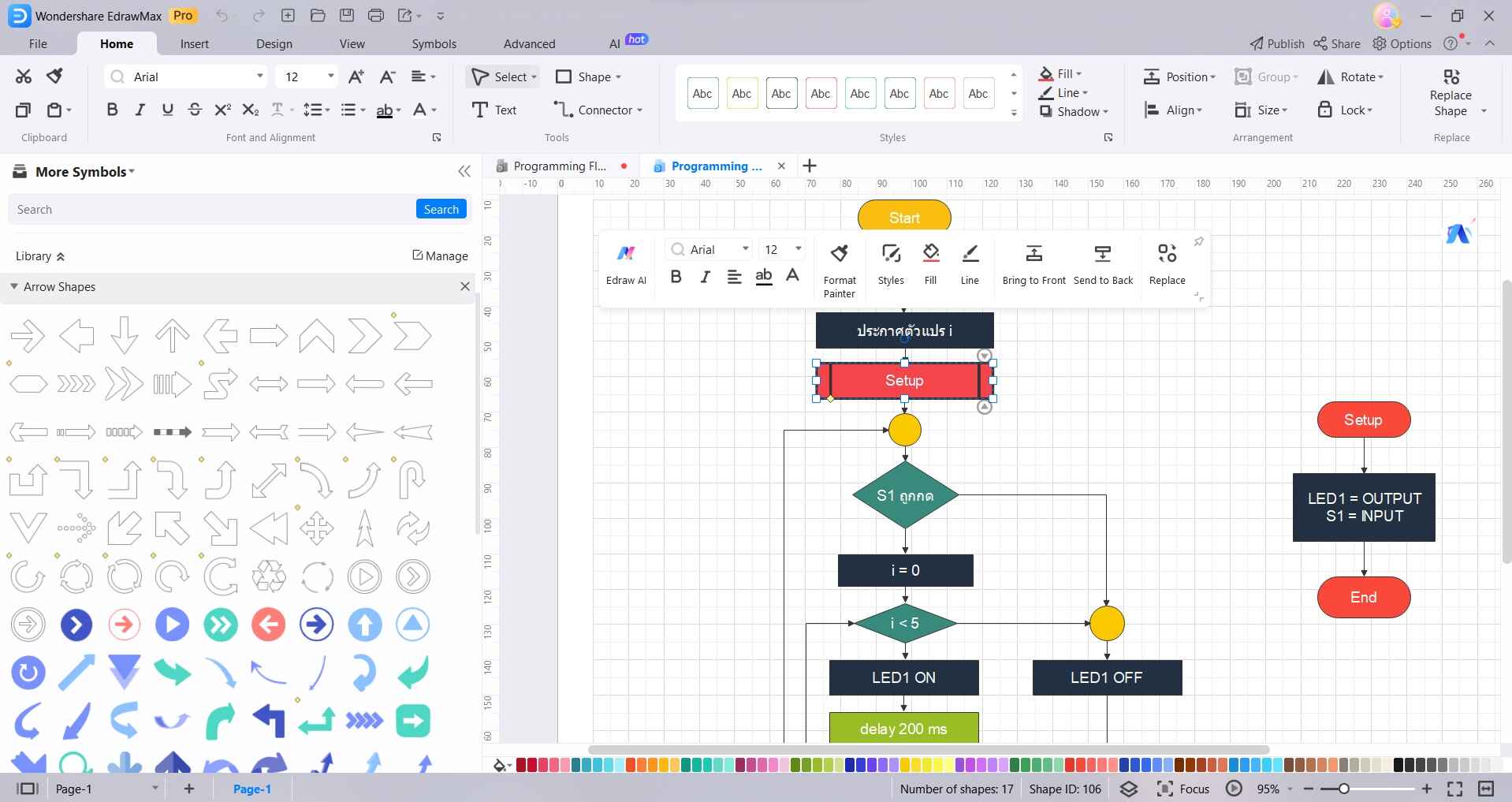
Step 4:
Modify the appearance of symbols, connectors, and text using formatting tools. Adjust colors, font sizes, line styles, and other visual elements to enhance readability and aesthetics.
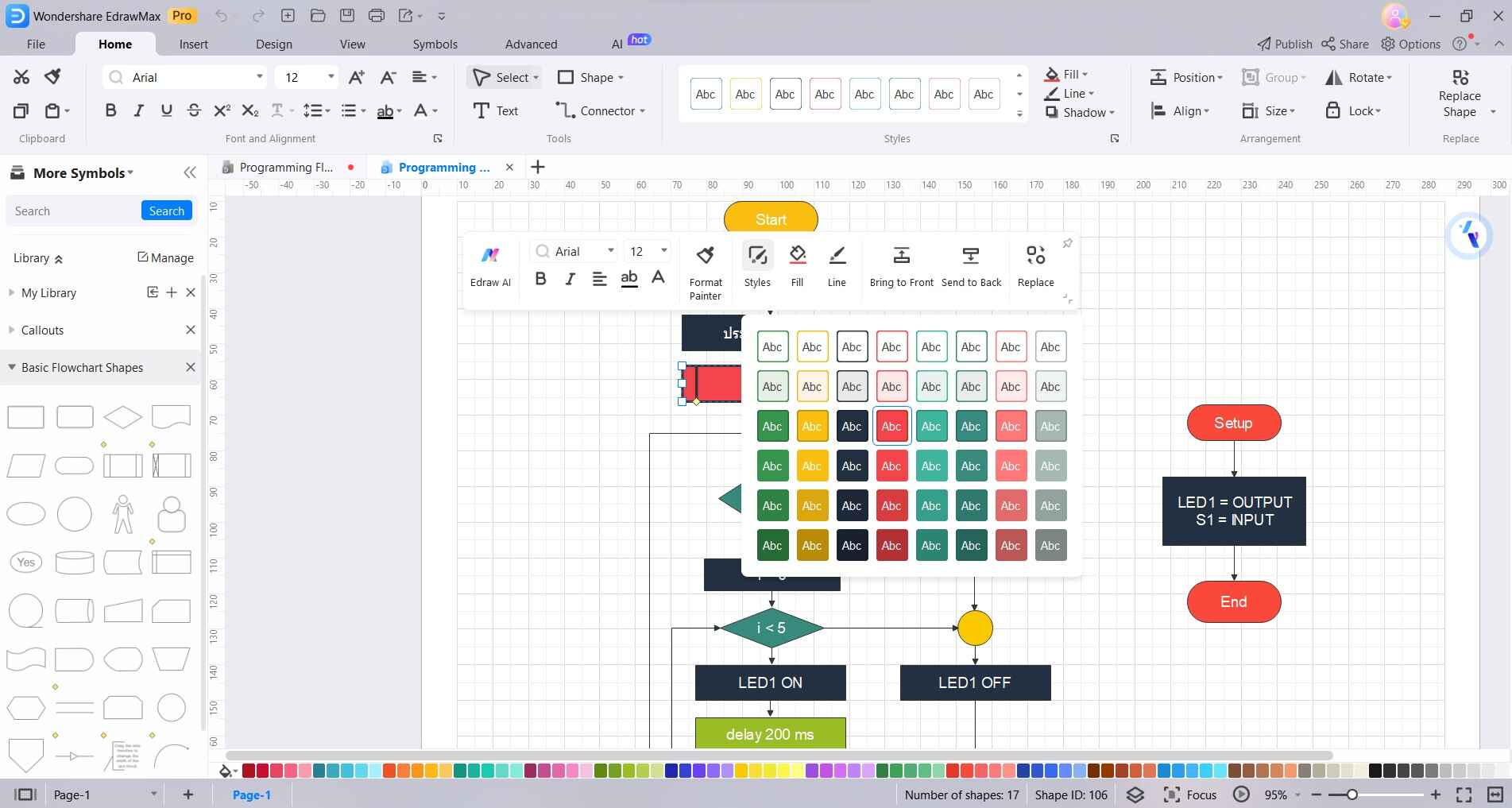
Step 5:
Save your work within EdrawMax for future editing. Export the flowchart to your desired format (such as PNG, JPG, PDF) for sharing or inclusion in documents or presentations.
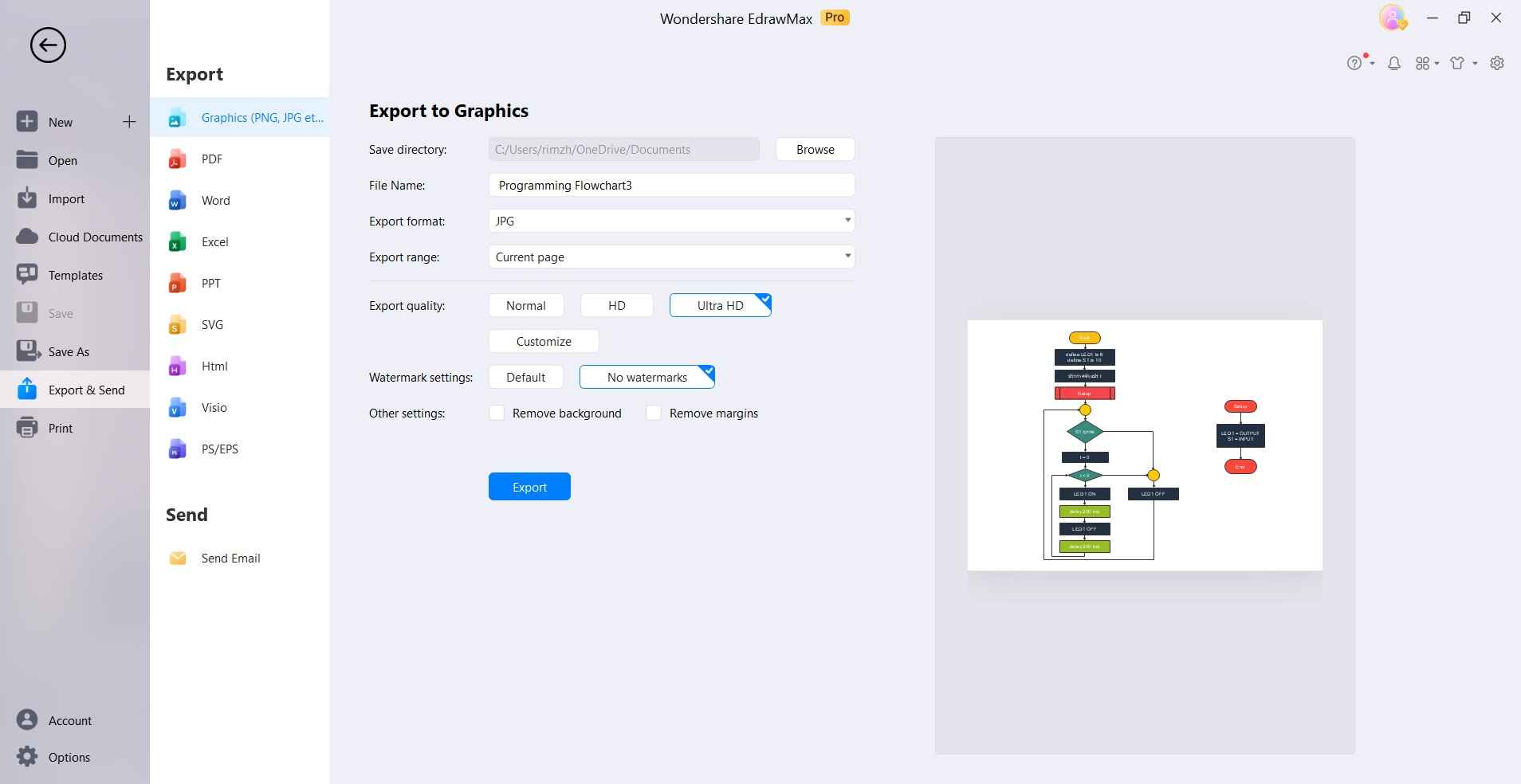
EdrawMax provides a user-friendly interface with drag-and-drop functionality, making it relatively intuitive to create flowcharts. Utilize the tool's features to visualize your programming logic effectively.
Conclusion
Understanding class structure is key across languages like C#, Python, and Swift. While OOP concepts translate broadly, some syntactical and structural differences exist between languages that impact how problems get modeled in code. By leveraging classes/structs judiciously, reusability and maintainability improve.
Tools like EdrawMax expedite software design via intuitive diagrams. As applications scale, classes provide the basis for extending, customizing, and managing complex systems.
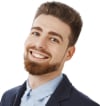