Linked lists are one of the fundamental data structures used in programming. They provide a way to store linear collections of data with efficient insertion and removal operations.
In this article, we will explore linked list programs in C++ implemented using classes and object-oriented principles.
Let's get started!
In this article
Part 1: What is a Linked List Program in C++?
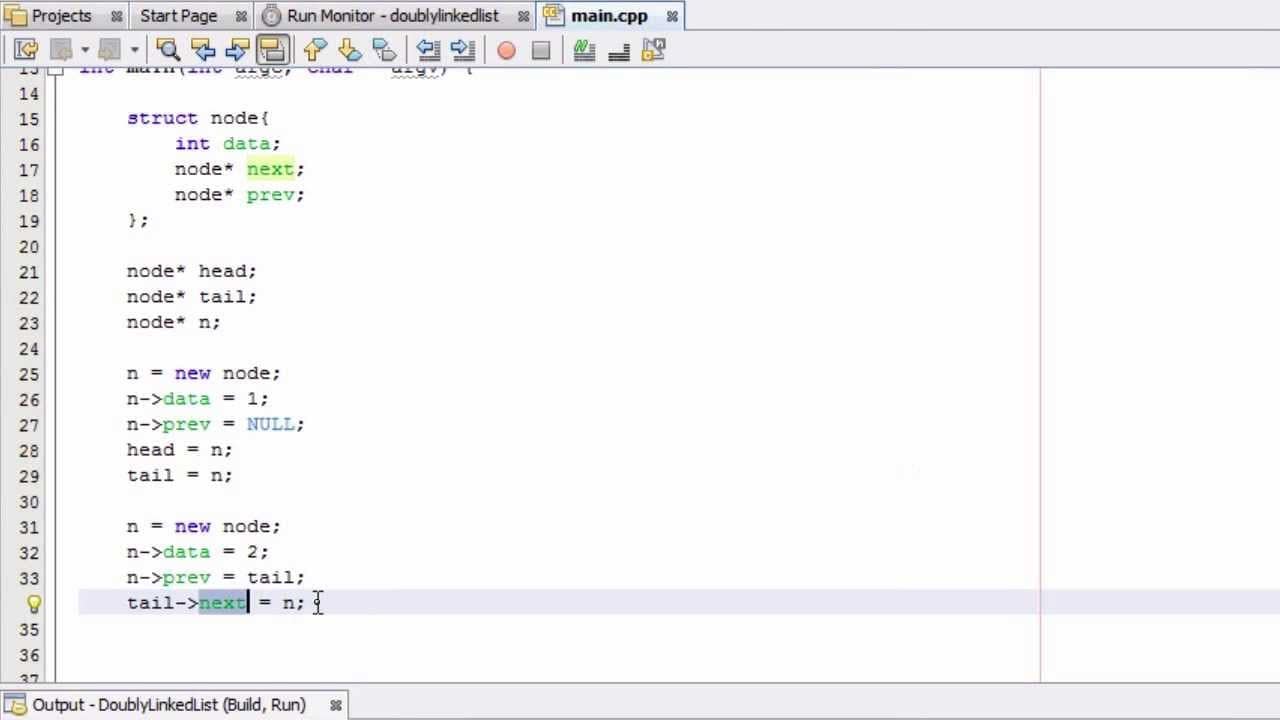
A linked list is a linear data structure consisting of nodes that are connected via pointers. Every node holds information along with a reference to the subsequent node within the sequence. The initial node is referred to as the head, and the final node directs to null or nothing, signifying the conclusion of the list.
In C++, we can implement linked lists using class or struct to represent each node. The class/struct will have members to store data and a pointer to refer to the next node.
Part 2: Types of Linked Lists in C++
There are three commonly used types of linked list programs in C++:
- Singly Linked List: In a singly linked list, every node only maintains a reference or pointer to the next node in the list. There are no pointers to the previous node. Singly-linked lists allow traversal in only one direction.
- Doubly Linked List: In a doubly linked list, each node has pointers to both the next node and the previous node.
- Circular Linked List: In a circular linked list, the last node's next pointer points to the first node forming a circular loop. This represents a repeating sequence allowing traversal in a loop.
Part 3: Steps for Implementing Linked List Program in C++ Using Class
Below are the key steps to build a basic linked list program in C++ using classes:
- Define a Node Class: This will represent each node in the linked list. It will have an integer data member to store node value and a pointer to the next node.
- Define Linked List Class: This class will represent the entire linked list. It will have a Node* variable to store the head node pointer. Member functions like addNode(), deleteNode(), etc can be added here.
- Create Node Objects Dynamically: For every new node, dynamic memory allocation using the 'new' keyword needs to be done.
- Update Next and Previous Pointers: The next and previous pointers of newly added nodes need to be correctly redirected to connect the nodes.
- Define Member Functions: Functions to add nodes at head/tail, delete nodes, print list, etc can be created.
- Use and Manipulate Linked List: We can now instantiate a Linked List class object and invoke functions like addNode(), deleteNode(), etc to build a linked list dynamically.
Part 4: Significance of Creating Linked List Program in C++ Using Class
Using a class to implement a linked list program has advantages over using primitive data types and structs in C++ due to the following reasons:
- Encapsulation: Member variables and functions are wrapped into a class allowing controlled access and abstraction.
- Data Security: Data can be made private or protected restricting direct access to class members.
- Reusability: Member functions written in class can be reused again and again by creating multiple objects.
- Maintainability: Code organized into classes is more maintainable than unstructured spaghetti code.
Thus, using object-oriented principles facilitates reusability, security, and modularity. This becomes more significant as the complexity of linked list programs increases in real-world applications.
Part 5: Creating an Algorithm Flowchart Using EdrawMax
EdrawMax is professional diagramming software that can help design algorithm flowcharts and data structure diagrams easily.
Some key benefits of using EdrawMax for programming and building linked lists are:
- Easy Drag-and-Drop Interface: Quickly draw flowcharts on canvas using icon symbols from vast libraries.
- Intuitive Organization of Complex Logic: Enables visualization of elaborate algorithms through flowcharts which is key for logical thinking.
- Customization for Data Structures: Specialized symbol sets can be designed for data structures like linked lists, trees, etc.
- Flexible Export Options: Flowcharts can be readily exported as images or coding languages like C++ further boosting productivity.
By incorporating tools like EdrawMax, programmers can save time, organize ideas methodically, and even auto-generate source code.
Here are the steps to create a simple programming algorithm flowchart using EdrawMax:
Step 1:
Launch the EdrawMax software on your computer. Choose a flowchart template or start with a blank canvas. Alternatively, switch to the “Template” section and search for “Algorithm Flowcharts”.
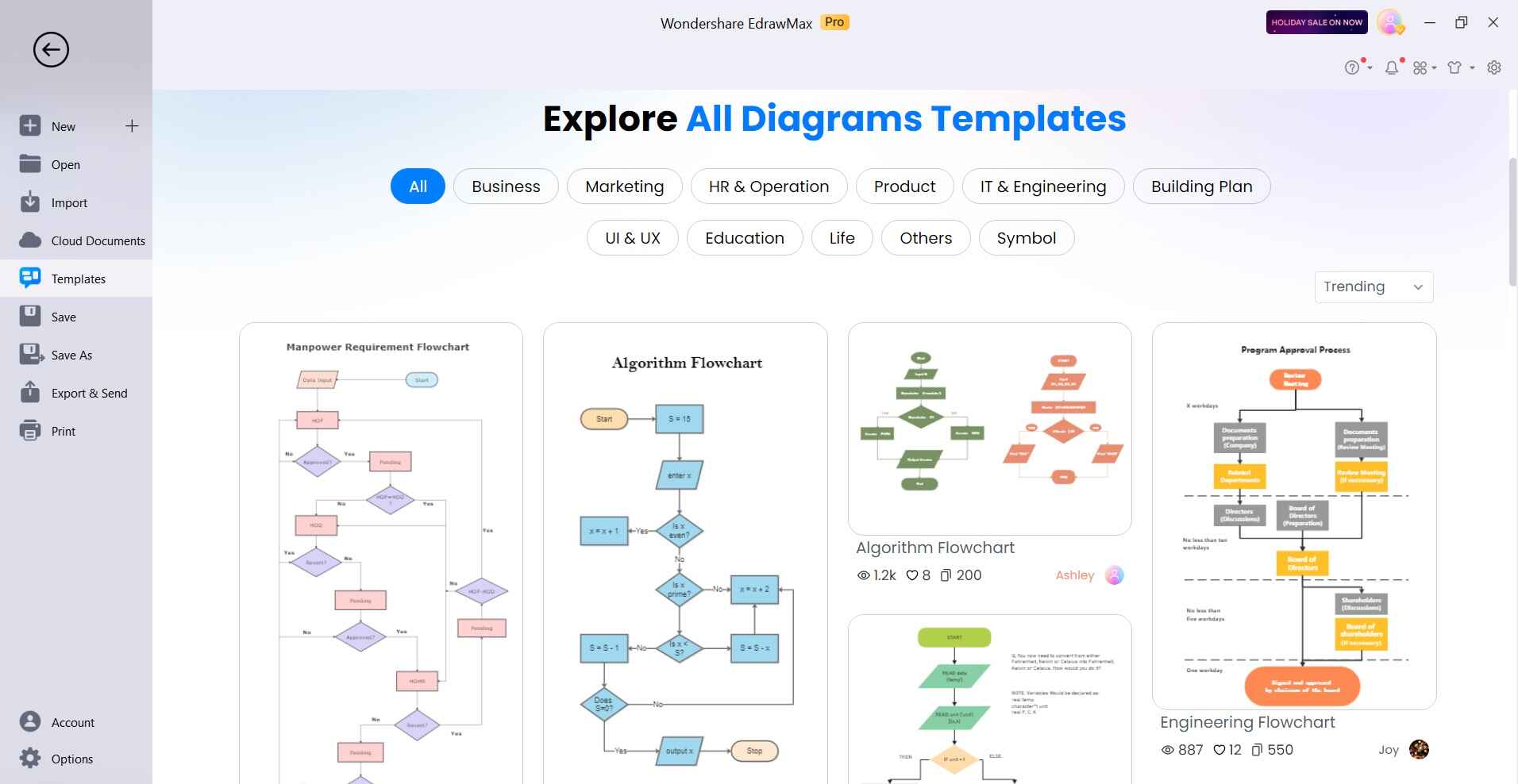
Step 2:
Drag and drop the Start and End symbols onto the canvas. These symbols typically resemble an oval or rounded rectangle.
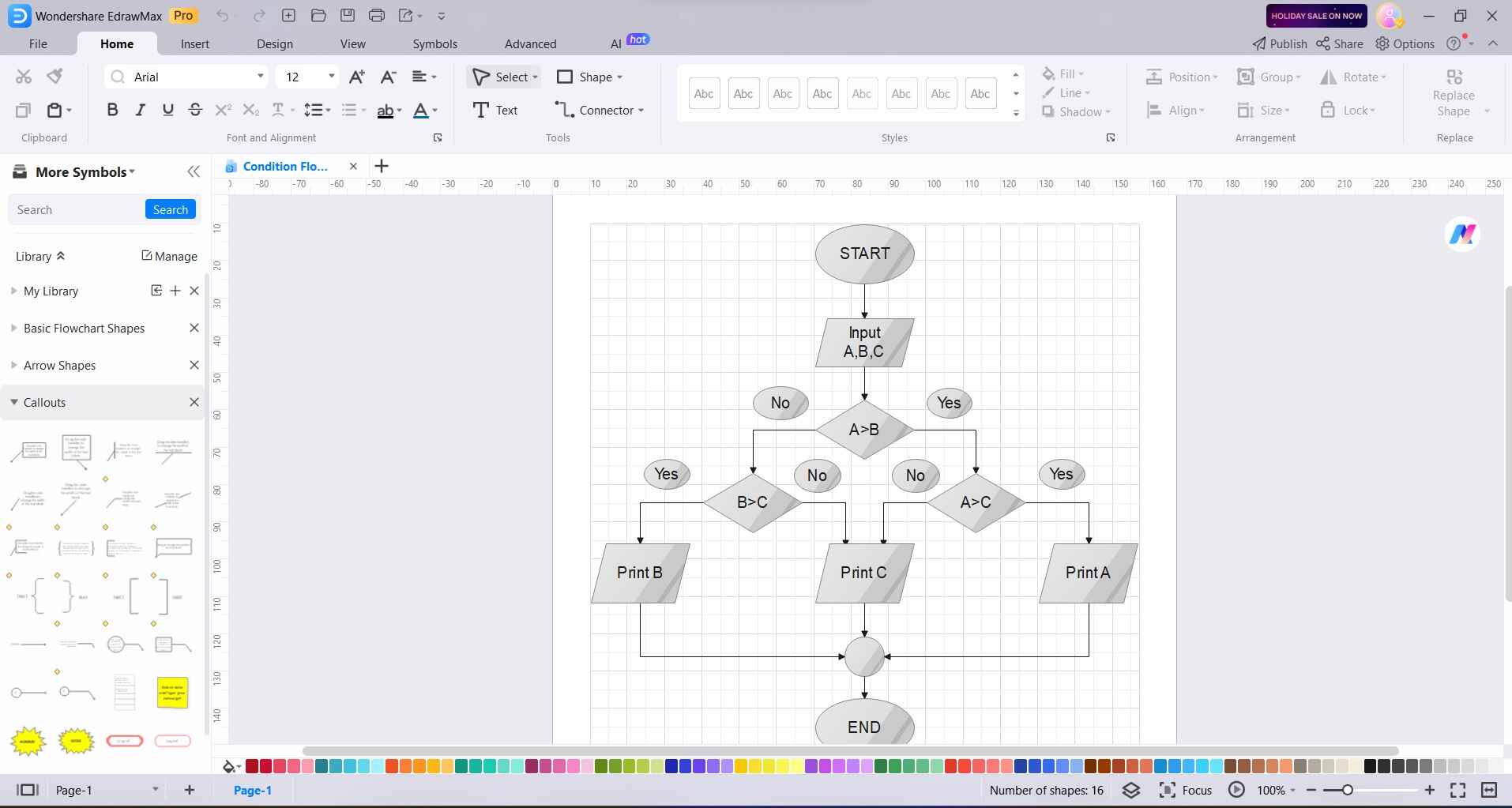
Step 3:
Add text to the arrows to describe the flow or indicate the conditions for decision symbols.
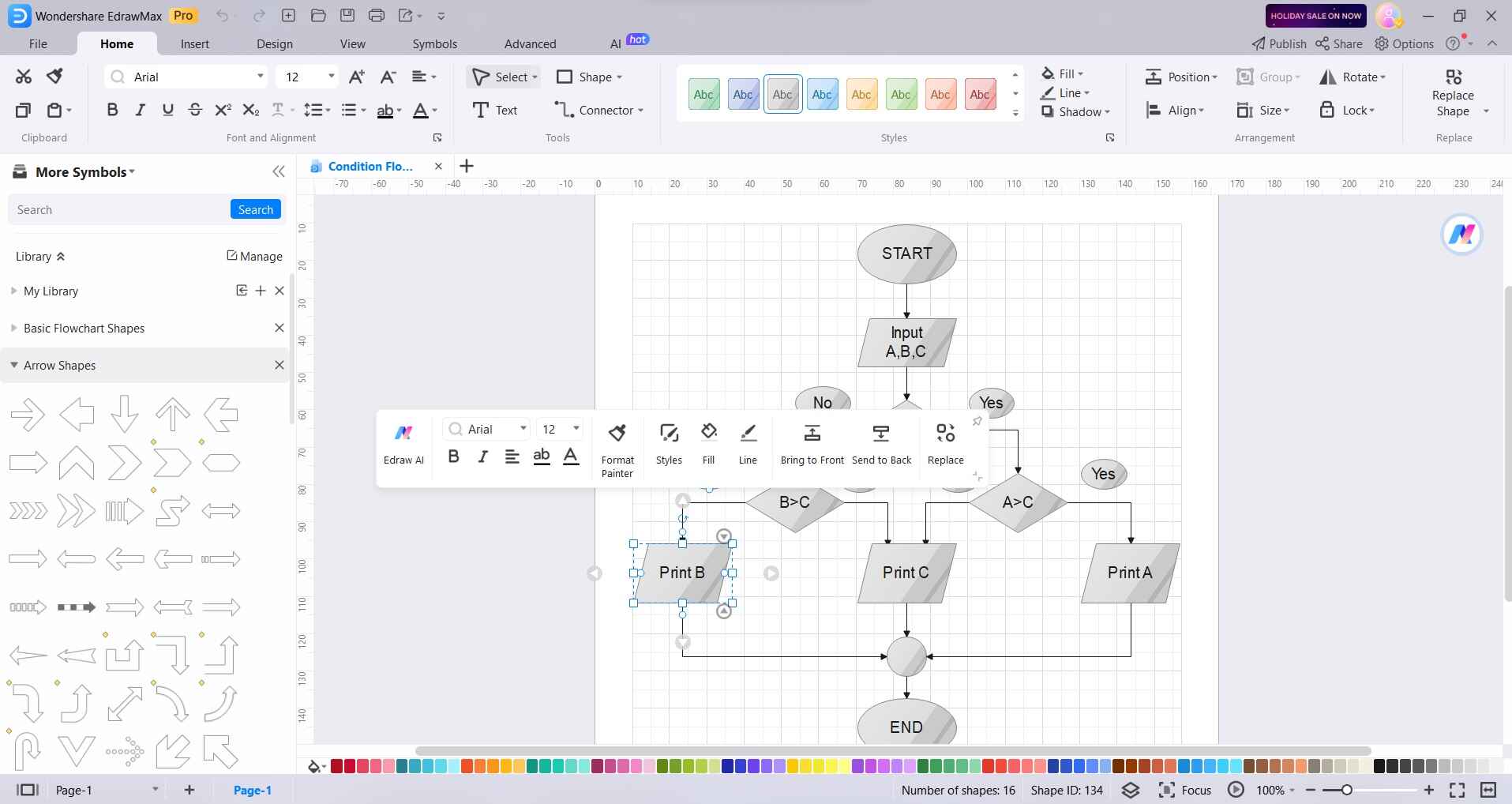
Step 4:
Adjust the layout, resize symbols, and format the text as needed to enhance readability. Click on any entity, and select “Styles” to make the chart more visually appealing.
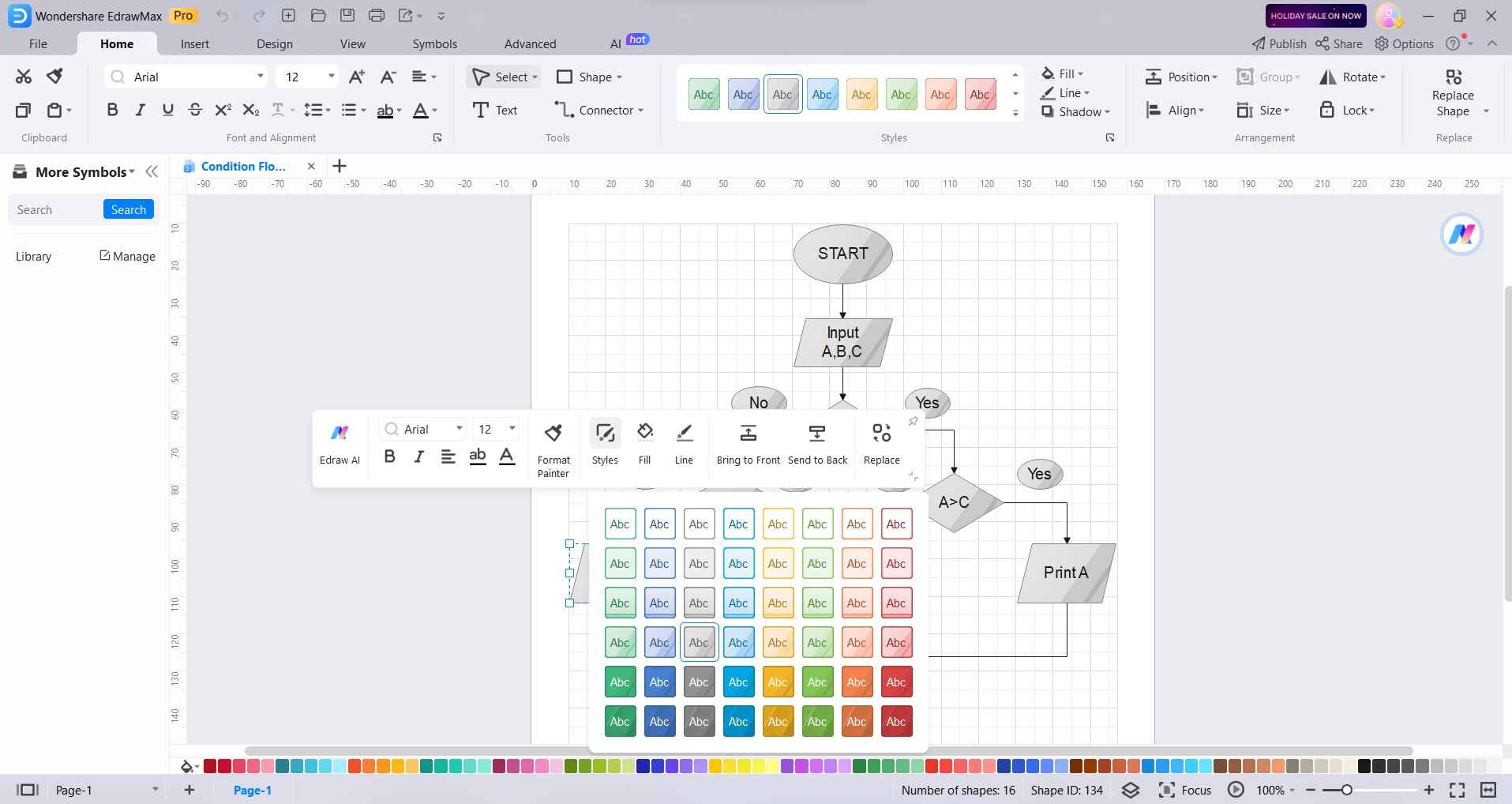
Step 5:
Once completed, save your flowchart in the desired format (e.g., JPG, PNG, PDF) and location on your computer.
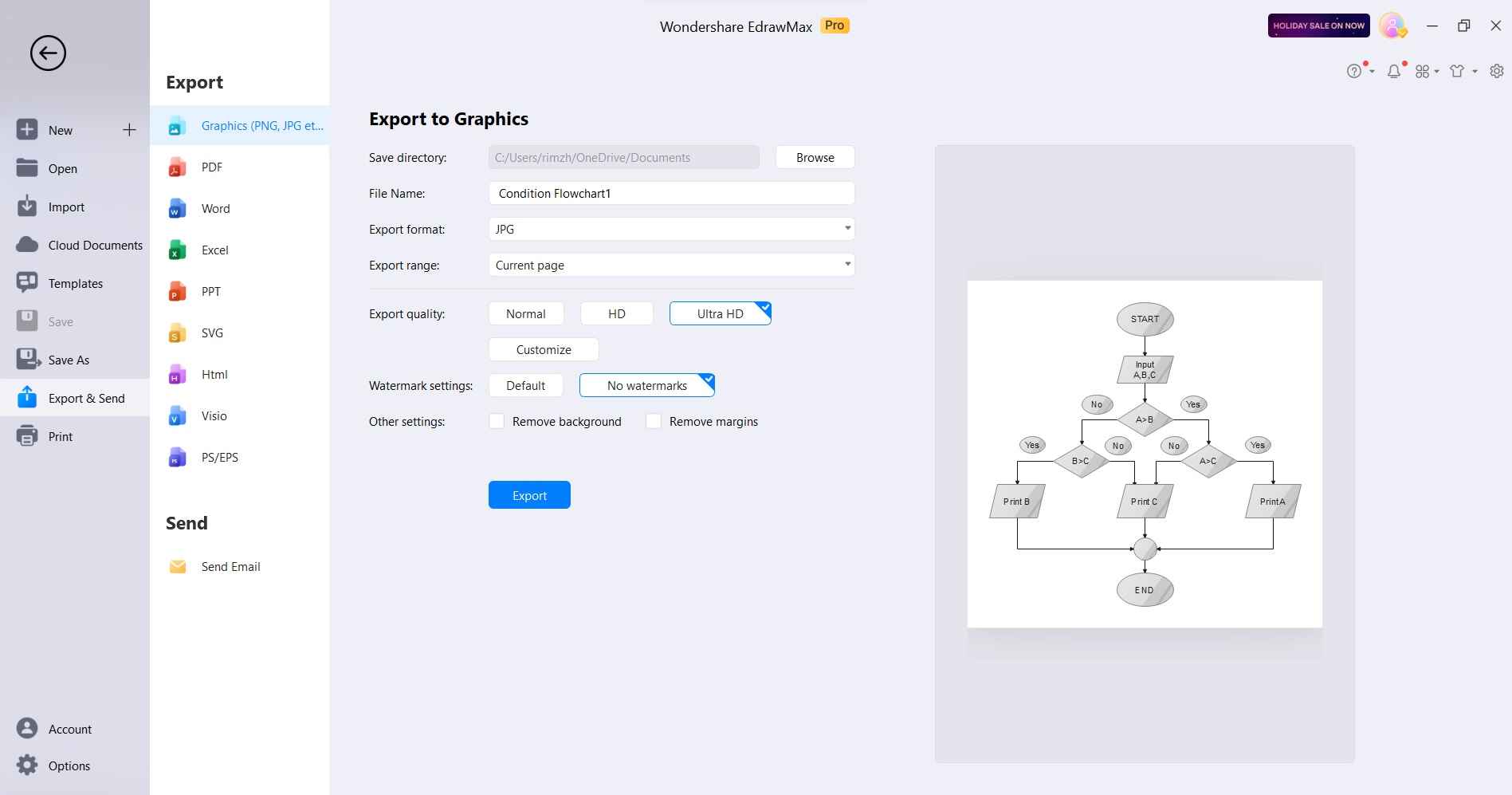
EdrawMax provides various symbols and tools to represent these steps visually in a flowchart.
Conclusion
We have explored key concepts like what linked lists are, different types of linked lists, object-oriented implementation using classes, and the utility of tools like EdrawMax for faster development and learning. Manipulating pointers to build linear dynamic data structures helps cement an understanding of memory management and reinforces algorithmic thinking.
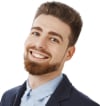