A class diagram is a static structure diagram essential in software engineering and object-oriented design. Class diagrams represent the structure of a system. They are crucial for designing robust and scalable software. It ensures components interact seamlessly.
In this article, I will explain a class diagram and present some typical examples. Also, I'll break down the components of a class diagram, including classes, attributes, operations, and relationships, to understand their roles. Additionally, we'll discuss the benefits of using class diagrams and present some typical examples in software development.
Ready to enhance your software design skills? Let’s examine UML class diagram examples and discover how they can streamline your development process.
In this article
Part 1. What is a Class Diagram?
A class diagram is a type of static structure diagram used in software engineering and object-oriented design. It visually represents the system's structure. It displays its classes, attributes, methods, and relationships. Class diagrams are a crucial part of the Unified Modeling Language (UML). It provides a standardized way to visualize the components of a software system.
A class diagram's primary purpose is to model an application's static view. This helps in understanding, designing, and documenting the software architecture. Class diagrams offer an organized blueprint by depicting the classes and their interconnections. These can guide developers throughout the software development process. They help identify the necessary classes, class data, and class operations.
Class diagrams show how different classes relate to each other. This includes relationships like inheritance, association, aggregation, and composition. These relationships explain how objects interact. Moreover, it creates a clear structure within the system.
Overall, class diagrams are a valuable tool for planning strong scalable software systems. They help team members communicate better. It also improves design quality and is an important part of software documentation. Developers can make sure that every part of the system works well together with it.
Part 2. 12 Class Diagram Examples
Class diagrams help us see the structure and flow of different systems clearly. They connect the dots between various parts, showing us how they work together. We'll explore twelve different class diagrams. They'll cover everything from healthcare to encryption and library systems. Each diagram is a practical tool for understanding how these systems organize and manage their operations effectively.
Class Diagram Example for Hotel Management
This is a class diagram for the hotel management system. It includes classes like Rooms, Receptionist, Accountant, Admin, Guest, and Booking. Each class has attributes and methods. For example, the Rooms class has attributes like room_id and methods like updateCondition. Relationships between classes show how they interact and form a hierarchy in the system.
Class Diagram Example for E-Commerce
The e-commerce system class diagram showcases different classes. It includes Customer, Account, Order, Address, and Shipment. Each class has its own attributes and methods. For instance, the Customer class has attributes like customerId and methods like OperationA. Relationships between classes show how they interact and form a clear structure within the system.
Class Diagram Example for Hospital Management
The class diagram for a hospital management system has several classes. These include Patient, Hospital, Doctor, and Nurse. Each class lists details like names for a Patient or tasks for a Doctor. It also shows how these classes connect. For instance, a Doctor looks after a Patient. This setup helps everyone understand their roles and how they work together.
Class Diagram Example for Encryption System
This class diagram shows the RSA Encryption system. It has three main classes: RSAEncryption, Plaintext, and Ciphertext. RSA Encryption handles key generation, encryption, and decryption. It uses integers like p, q, n, phi, e, and d. Plaintext holds the message to encrypt, while Ciphertext contains the encrypted data. The diagram illustrates data flow from plaintext creation to encryption.
Class Diagram Example for Shopping Management
The ABC Shop system class diagram has different parts. It includes abcShopSystem, personParentClass, CustomerFinal, Employee, and Supplier. The abcShopSystem class manages all store functions and data. The personParentClass is a basic class with common features like name and discount. Classes such as CustomerFinal, Employee, and Supplier come from personParentClass. They add special attributes like email and work hours for employees.
Class Diagram Example for Library Management
The Library UML Class Diagram includes several key components. Some are Librarian, Library Member, Student, Staff, Book, and Journal. The Librarian manages books and students. Library Members can issue and return books. Students and Staff are types of Library Members, with Staff able to issue and return journals. Books and Journals are linked to their management functions and attributes.
Class Diagram Example for Ticket Booking System
The Online Ticket Booking System class diagram includes classes. The classes are User, Ordering, Payment, Tickets, Database, Administrator, and LotterySystem. Users can sign up and update their profiles. The Ordering class takes care of ticket sales. The Payment class handles transactions. Administrators update the system and tickets. The LotterySystem class lets users search and buy tickets.
Class Diagram Example for App Management
The Reminders App class diagram shows how the app organizes reminders. MainGUI, FileManager, and ReminderList are key classes. MainGUI is the main interface. FileManager handles saving and loading data. ReminderList stores lists of reminders. Other classes like CreateReminderListWindow and EditReminderListWindow let users add or change reminders. Each part works together to make the app easy to use.
Class Diagram Example for Virtual Graden Management
The Garden class diagram shows various classes like Cat, Ant, AntHill, Mushroom, Main, and Background in a virtual garden. Each class has unique functions. For example, Cat and Ant have actions like move or render. The Main class connects all these parts, setting up and managing user interactions for a lively garden simulation.
Class Diagram Example for Online Eduation
This diagram shows an online education system much like Microsoft Teams. It has classes such as Participant, Student, Teacher, Attendance, File, Quiz, and Meeting. Students join meetings and check grades. Teachers start meetings and assign work. Quizzes use methods for adding and scoring. This setup makes managing classes and resources smoother.
Class Diagram Example for Airport Management
The structure of an airport management system is depicted in the UML class diagram which illustrates various entities and their relationships. Critical components include Airports, Airlines, Airplanes, Flights, Employees (which consists of Staff and Pilots), Customers, and Tickets. Attributes and methods for each class are outlined such as IDs and names while associations like an airport that hosts several airlines that operate numerous flights are shown. Employees are linked to airlines as well as personal details with identification numbers besides their roles. On the other hand, customers can book tickets establishing a clear interaction flow within the system.
Class Diagram Example for Parking lot Management
The Parking Lot Services class diagram features ParkingLot, Vehicle, Account, and ParkingTicket. The ParkingLot class handles ticket creation. Entrance and Exit Panels are in charge of printing tickets and calculating fees. The Vehicle and Account classes store details about cars and user accounts. This helps the parking service run smoothly.
Part 3. Components of Class Diagrams
Let's discuss the components of class diagrams.
Sections
The class diagram has three primary sections:
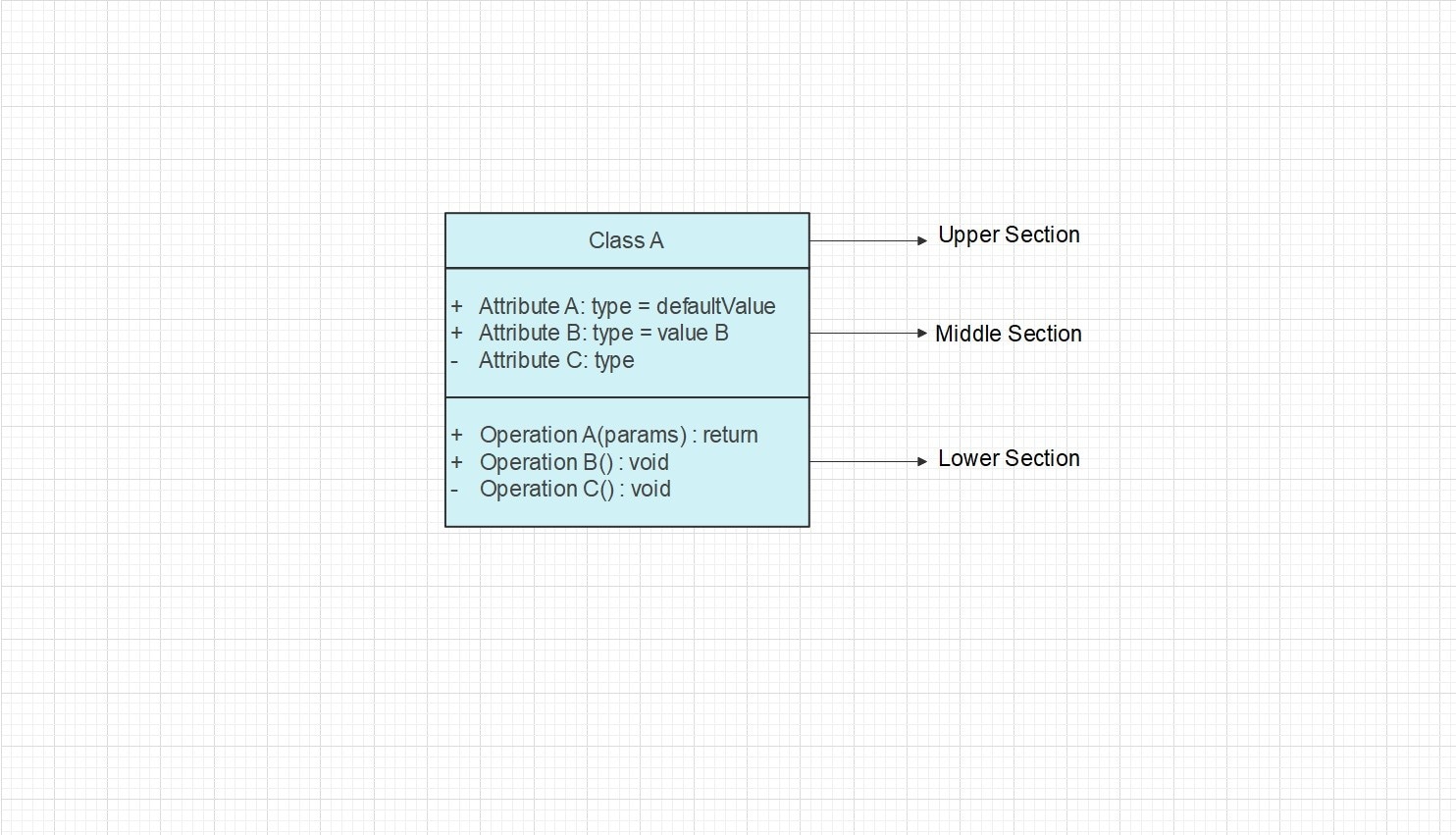
In the upper section, you'll find the class name, which should be bold and centered, starting with a capital letter. If the class is abstract, its name appears in italics. This naming helps clearly identify and differentiate the class.
The middle section details the class's attributes. Each attribute is marked with visibility indicators: public (+), private (-), protected (#), or package (~). These symbols reveal who can access the attributes. Each attribute name should be clear and descriptive, explaining its role within the class.
In the lower section, you'll see the methods or operations of the class. These are listed line by line, illustrating how the class manipulates data. Each method can include parameters and return types. It provides a snapshot of class functionalities.
This structure allows anyone reading the diagram to quickly understand the class's role, properties, and capabilities in the system.
Relationships
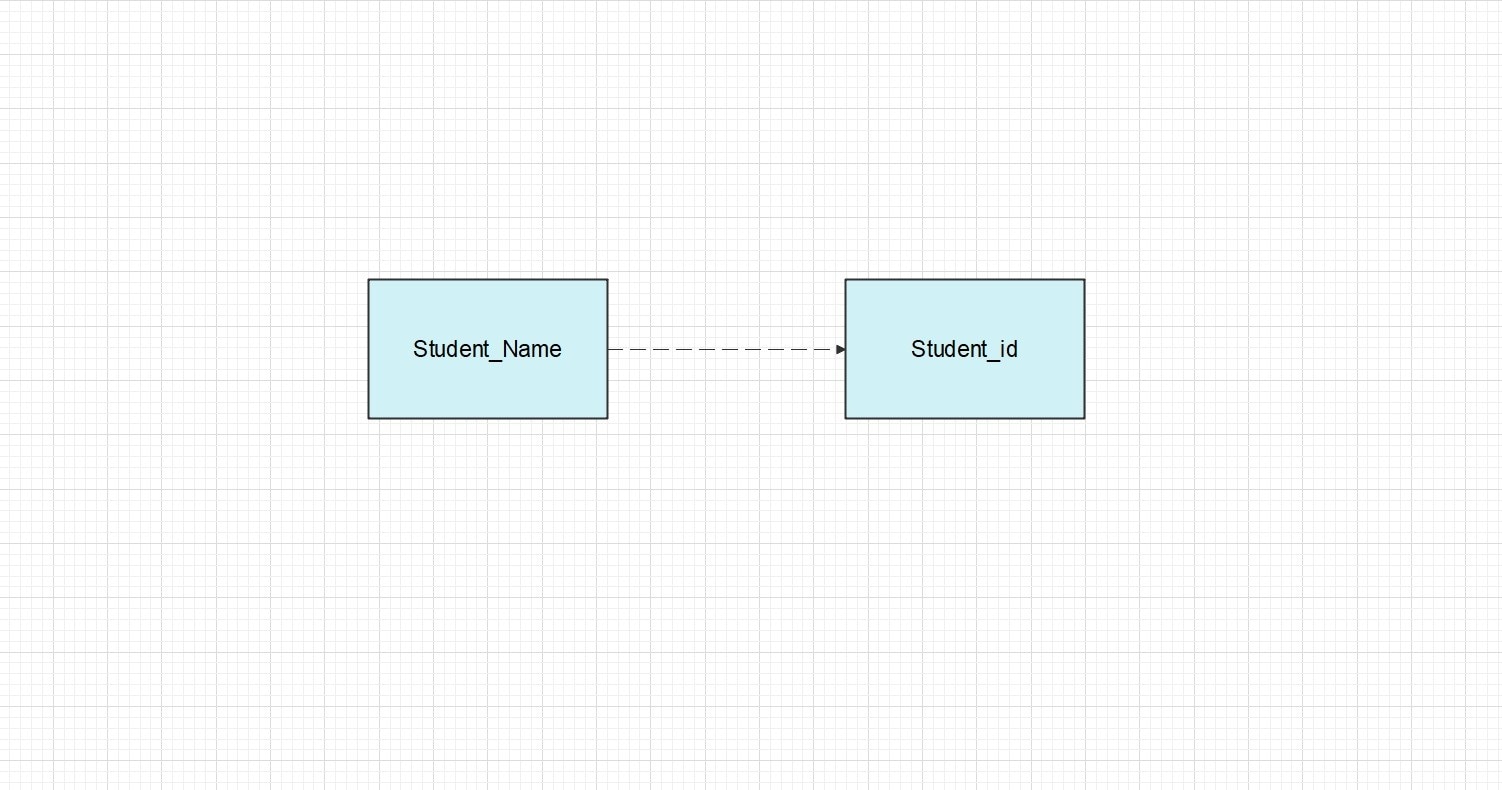
In class diagrams, Dependency signifies a situation where one class relies on another. This is shown with a dashed line. For example, the dashed line between Student_Name and Student_id suggests that operations in the Student_Name class may depend on the Student_id class. This setup highlights that the student’s name is connected to their specific identification number.
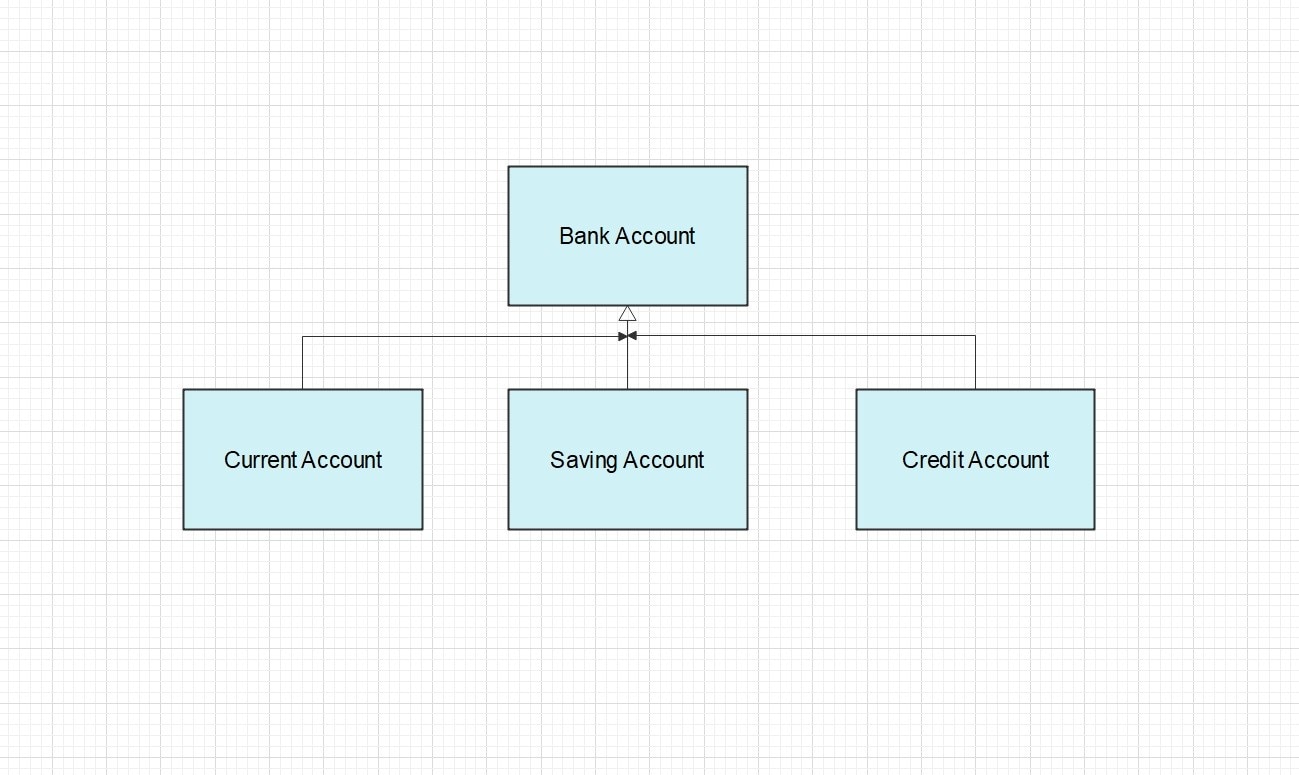
Generalization in a class diagram shows a hierarchy where one class is a special form of another. It is shown by a line that ends in a hollow triangle pointing at the more general class. For example, Current Account, Saving Account, and Credit Account are all derived from the Bank Account class. This setup demonstrates their inheritance of common features.
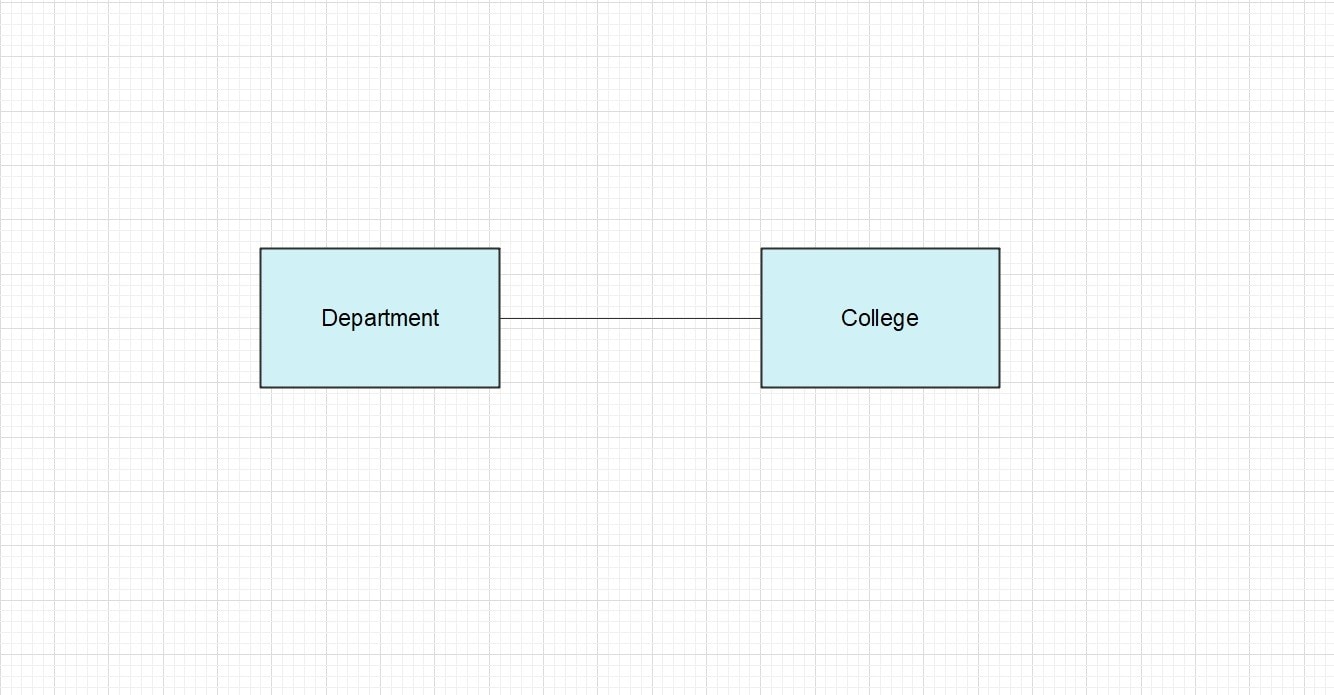
In class diagrams, Association shows how classes are linked. Each class works with another through their objects. For example: a Department linked to a College. This link means the department is part of the college, combining their educational roles.
Multiplicity
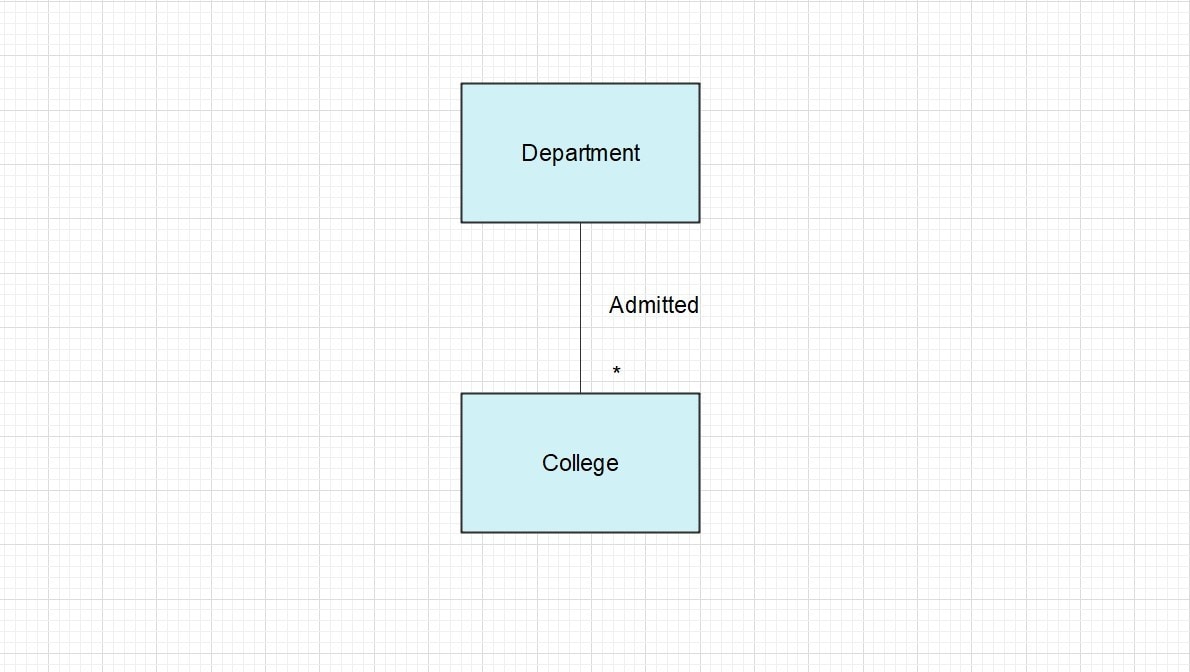
Multiplicity in class diagrams shows how many instances of one class can link to another. For example, the Admitted between Department and College means several departments can be part of one college. This is a one-to-many relationship from college to departments.
Aggregation
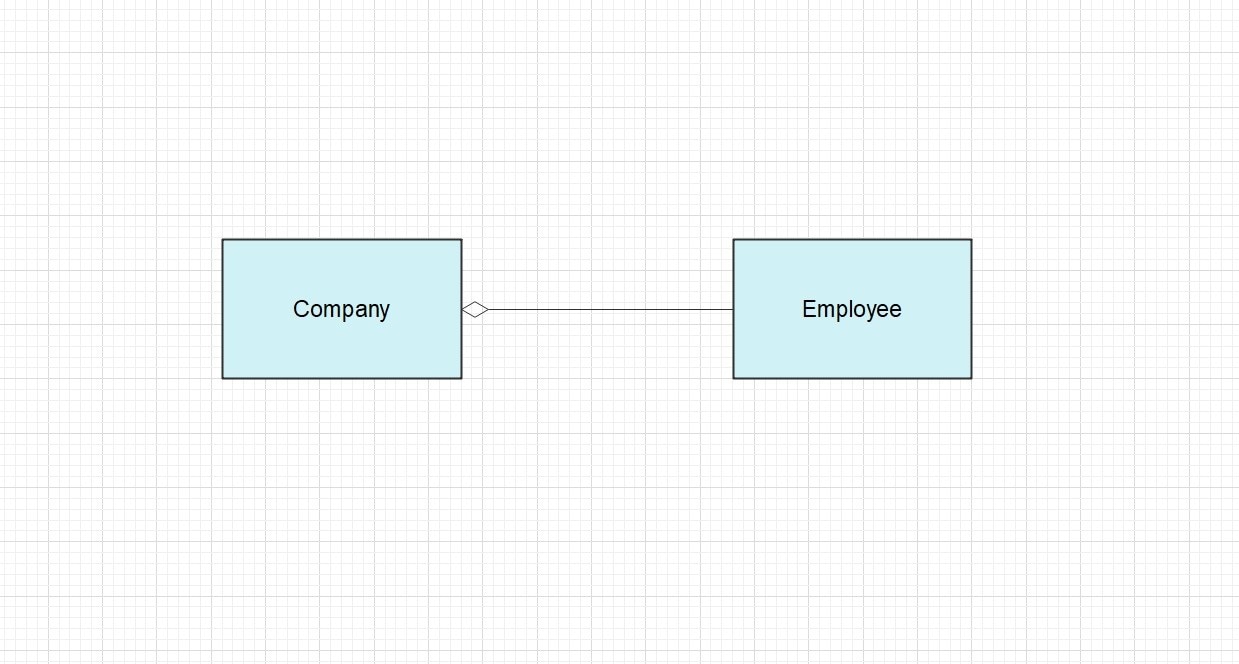
Aggregation in class diagrams represents a relationship where one class is a part of another but can exist independently. It shows a whole-part association. In the example, the Company and Employee diagram shows aggregation. The hollow diamond at the Company endpoints to the Employee. This indicates that while employees belong to a company, they can exist outside of it too.
Composition
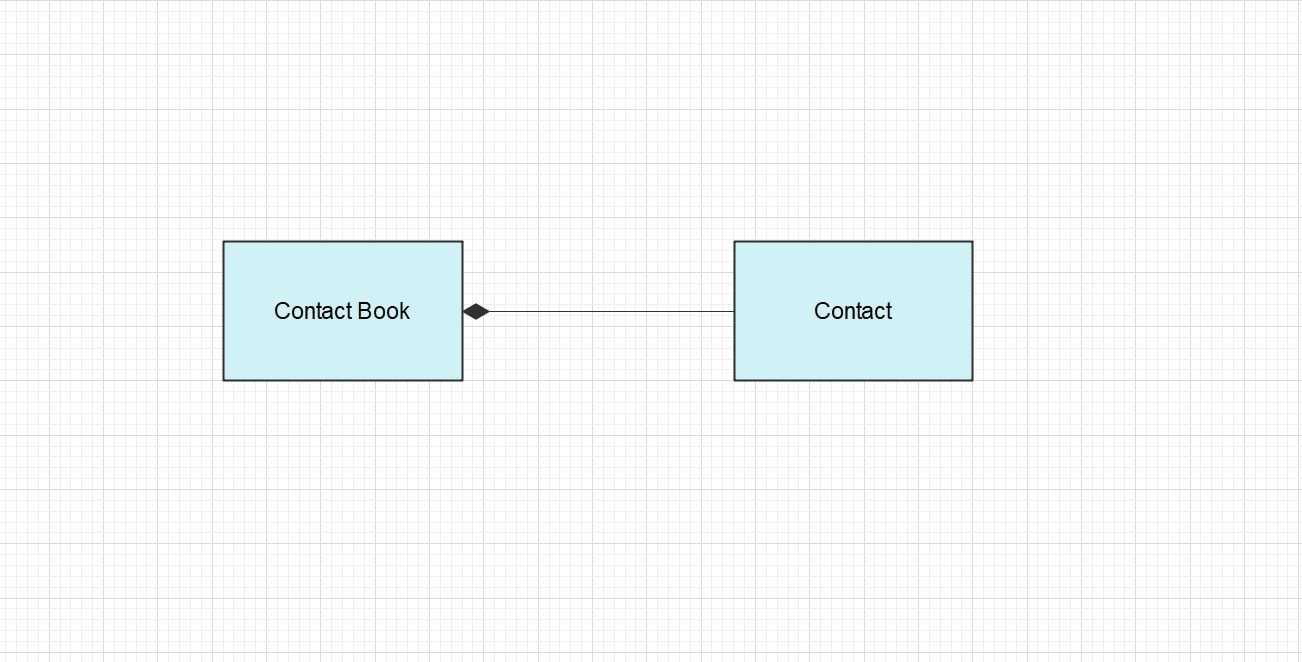
Composition in class diagrams represents a strong form of association where a part cannot exist without the whole. It shows a whole-part relationship, marked by a solid diamond. For instance, a Contact Book contains Contacts. The solid diamond at the Contact Book end indicates that contacts cannot exist without being part of the contact book.
Part 4. Benefits of Class Diagrams?
Class diagrams provide several key benefits in software development and systems analysis:
- Visual Clarity They offer a clear visualization of a system's structure, clarifying how components interact and relate to each other.
- Enhanced Communication These diagrams improve communication among team members, ensuring everyone has a shared understanding of the system's design.
- Facilitates Scalability Class diagrams make it easier to add new features or functionality while maintaining the integrity of the existing system.
- Early Issue Identification They help identify potential design issues early, allowing for adjustments before development progresses too far.
- Efficiency in Development By providing a clear blueprint, class diagrams can streamline the development process and reduce errors.
FAQ
Here are some frequently asked questions about using class diagrams:
-
Why use class diagrams?
Class diagrams are used to visually organize and model the classes in a software system, making it easier to understand, communicate, and maintain the system’s structure. -
How do class diagrams help in development?
They help developers see not only the static structure of the system but also how the various parts of a system interact with each other, which simplifies both the design and development processes. -
Can class diagrams be used for database design?
Yes, class diagrams can effectively represent the entities in a database and their relationships, serving as a blueprint for creating relational databases. -
What are the key elements of a class diagram?
Key elements include classes, attributes, operations, and different types of relationships such as associations, generalizations, and compositions.
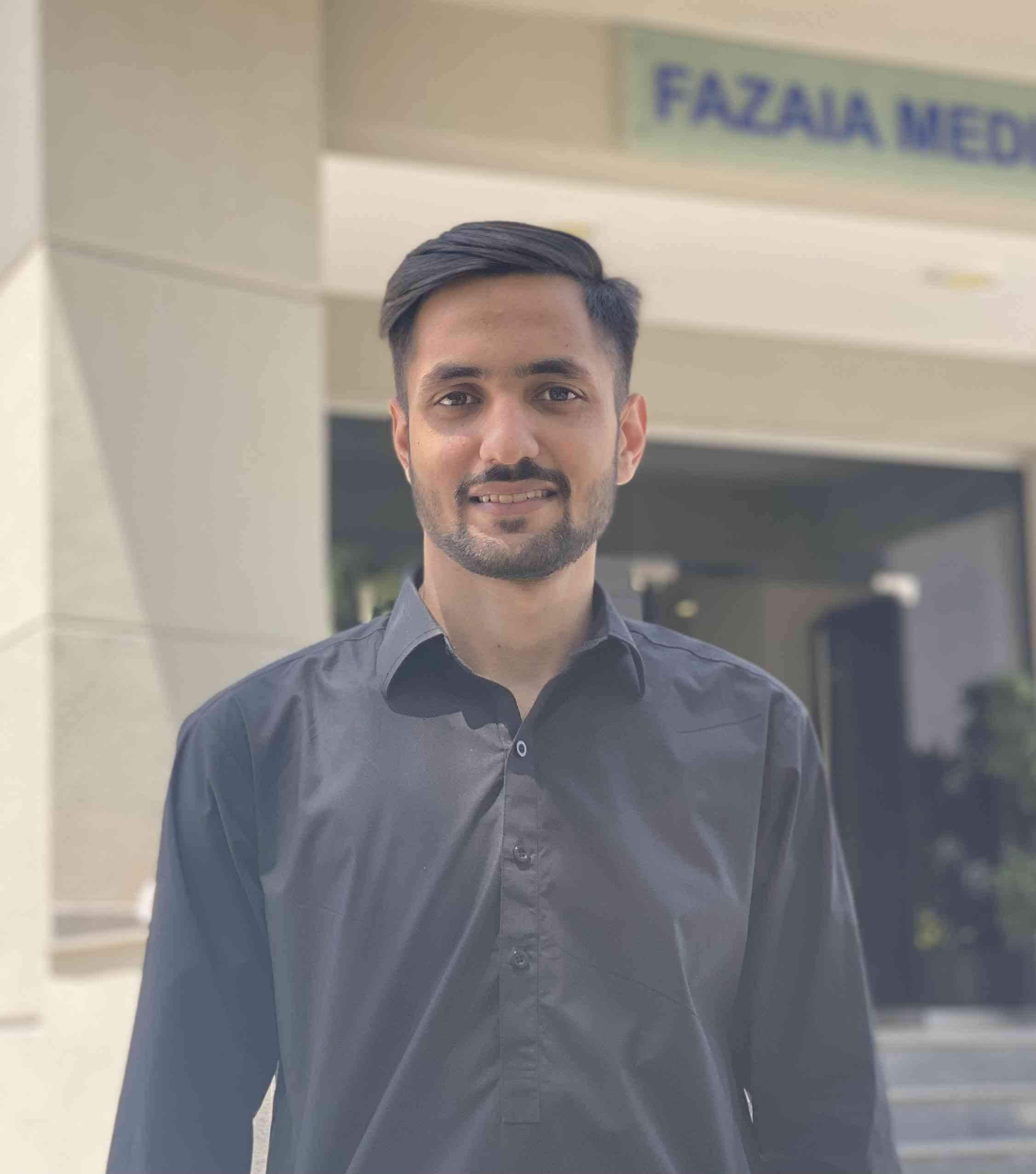